How to Use Vultr Managed Databases for Caching in Python
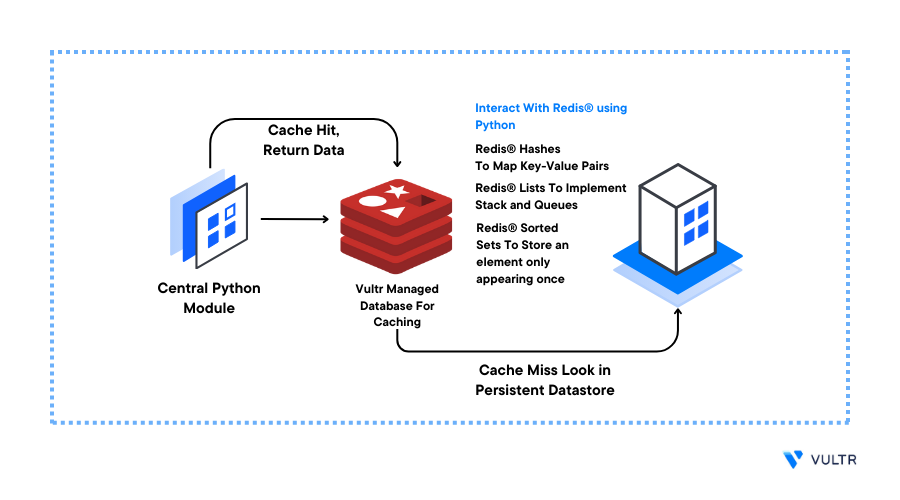
Introduction
Redis® is an in-memory data store that offers a flexible data structure, high performance, and on-demand scalability. A Vultr Managed Database for Caching offers high availability, high throughput, and low latency for mission-critical applications. This guide explains how to use a Vultr Managed Database for Caching in Python to create highly available applications.
Prerequisites
Before you begin:
- Deploy a Vultr Managed Database for Caching
- Deploy a Vultr Linux server to use as the development machine
- Use SSH to access the server as a non-root user
Set Up the Python Development Environment
To integrate a Vultr Managed Database for Caching to your Python application, install the Python redis
module and set up a sample project directory to store the application files.
Upgrade the Python
pip
package manager.$ pip install --upgrade pip
Install the Python
redis
driver to connect to the Redis® database.$ pip install redis
Create a new
project
directory.$ mkdir project
Navigate to the new
project
directory.$ cd project
Create the Redis® Gateway Class
To use your Vultr Managed Database for Caching connection in your Python application files, create a central Python module that connects to the database. You can then reuse the module by declaring the database module using the import
clause in your application. Follow the steps below to create the Redis® connection module
Using a text editor such as Vim, create a new
redis_gateway.py
file$ nano redis_gateway.py
Add the following code to the file. Replace the
vultr-prod-abcd.vultrdb.com
,16752
, andexample-password
values with your correct Vultr Managed Database for Caching detailsimport redis class RedisGateway: def __init__(self): r_host = 'vultr-prod-abcd.vultrdb.com' r_port = 16752 r_pass = 'example-password' self.r_client = redis.Redis( host = r_host, port = r_port, password = r_pass, ssl = 'true' )
Save and close the file
The above Python application code declares a
RedisGateway
class with an__init__(self)
method. This method executes every time you create an instance of theRedisGateway
class. Then, it connects to the Redis® database using theself.r_client = redis.Redis(...)
function.To import the Redis® database module to other Python source code files, use the following declarations:
import redis_gateway r_gateway = redis_gateway.RedisGateway() r_client = r_gateway.r_client
Implement Redis® Strings
In Redis®, a string is a sequence of bytes and it's the most used data type. You can use the string data type to store session IDs, user passwords, product information, and static HTML content. To create a key in a Redis® server, use the set
keyword. To retrieve a key value, use the get
keyword. In this section, create a Python application to implement Redis® strings as described below.
Create a new
redis_strings.py
file$ nano redis_strings.py
Add the following contents to the file
import redis_gateway r_gateway = redis_gateway.RedisGateway() r_client = r_gateway.r_client r_key = "john_doe" r_value = "example-password" r_client.set(r_key, r_value) print("...\r\n You've successfully set the Redis key.\r\n") if r_client.exists(r_key): r_value = r_client.get(r_key).decode("utf-8") print("The value for the " + r_key + " key is " + r_value + "\r\n ...")
Save and close the file.
The above application file imports the
redis_gateway
module you created earlier, then:r_key
: Defines the name of the string key you're setting in the Redis® databaser_value
: Defines the Redis® key valuer_client.set(r_key, r_value)
: Sets the key in the Redis® databaseif r_client.exists(r_key)
: Verifies whether the key exists in the Redis® database server before retrieving it to avoid run time errorsr_client.get(r_key).decode("utf-8")
: Retrieves the key from the Redis® database
Run the Redis® strings application file
$ python3 redis_strings.py
Output:
... You've successfully set the Redis key. The value for the john_doe key is example-password ...
As displayed in the above output, Python correctly connects to the Redis® database and sets the value
example-password
for the string keyjohn_doe
.
Implement Redis® Lists
A Redis® list is an ordered collection of strings used to implement queueing mechanisms. Redis® allows you to add elements to the head or tail of a list using the lpush
and rpush
commands. Create a new Python application to test the Redis® Lists functionality as described below.
Create a new
redis_lists.py
file$ nano redis_lists.py
Add the following contents to the file
import redis_gateway import json r_gateway = redis_gateway.RedisGateway() r_client = r_gateway.r_client r_list = "sample_customer_job_list" sample_job_1 = { "firstName": "JOHN", "lastName": "DOE", "email": "john_doe@example.com" } sample_job_2 = { "firstName": "MARY", "lastName": "SMITH", "email": "mary_smith@example.com" } r_list_value_1 = json.dumps(sample_job_1) r_list_value_2 = json.dumps(sample_job_2) r_client.lpush(r_list, r_list_value_1, r_list_value_2) print("...\r\n You've successfully added a customer registration jobs to the Redis list.\r\n...") while(r_client.llen(r_list) != 0): print("The values for the " + r_list + "\r\n...") print(r_client.lpop(r_list).decode("utf-8") + "\r\n...")
Save and close the file.
Below is what the above application code does:
- The
r_list
variable represents the key of your sample Redis® list. sample_job_1 = {...}
andsample_job_1 = {...}
are sample list values.- The
r_client.lpush(r_list, r_list_value_1, r_list_value_2)
function inserts the two sample jobs into the Redis® list - The
while(r_client.llen(r_list) != 0):
loop queries the Redis® server and iterates through ther_list
variable to print the list values
- The
Run the
redis_lists.py
application file$ python3 redis_lists.py
Output:
... You've successfully added a customer registration jobs to the Redis list. ... The values for the sample_customer_job_list ... {"firstName": "MARY", "lastName": "SMITH", "email": "mary_smith@example.com"} ... The values for the sample_customer_job_list ... {"firstName": "JOHN", "lastName": "DOE", "email": "john_doe@example.com"} ...
Implement Redis® Hashes
Redis® hashes are record types that map keys to value pairs. Implement Redis® hashes in the form of company data in a Python application as described below.
Create a new
redis_hash.py
file$ nano redis_hash.py
Add the following contents to the file
import redis_gateway import json r_gateway = redis_gateway.RedisGateway() r_client = r_gateway.r_client r_hash = "company_profile" r_client.hset(r_hash, "company_name", "XYZ COMPANY") r_client.hset(r_hash, "add_line_1", "123 SAMPLE STREET") r_client.hset(r_hash, "add_line_2", "APT BUILDING") r_client.hset(r_hash, "county", "3RD COUNTY") r_client.hset(r_hash, "city", "SUN CITY") r_client.hset(r_hash, "zip", "123456") print("...\r\nYou've successfully set a company profile.\r\n...") print("Company profile information \r\n" ) print(json.dumps(str(r_client.hgetall(r_hash)))) print("Company Name : " + r_client.hget(r_hash, "company_name").decode("utf-8"))
Save and close the file.
In the above application:
The
r_hash
variable represents the hash key in the Redis® database.The
r_client.hset(r_hash, "company_name", "XYZ COMPANY")
function sets a hash in the Redis server. This function is the same as:company_profile['company_name'] = "XYZ COMPANY"
r_client.hgetall(r_hash)
: Retrieves all key-value pairs for a given hashr_client.hget(r_hash, "company_name")
: Retrieves the value of a given key in the hash
Run the application
$ python3 redis_hash.py
Output:
... You've successfully set a company profile. ... Company profile information "{b'company_name': b'XYZ COMPANY', b'add_line_1': b'123 SAMPLE STREET', b'add_line_2': b'APT BUILDING', b'county': b'3RD COUNTY', b'city': b'SUN CITY', b'zip': b'123456'}" Company Name : XYZ COMPANY
Implement Redis® Sorted Sets
Sorted sets represent a collection of strings arranged by an associated score. An element can only appear once in a sorted set. Redis® offers the zadd
and zrange
functions for adding and retrieving sorted sets values. Implement these functions in a Python application as described below.
Create a new
redis_sorted_set.py
file$ nano redis_sorted_set.py
Add the following contents to the file
import redis_gateway import json r_gateway = redis_gateway.RedisGateway() r_client = r_gateway.r_client r_sorted_set = "database_ratings" database_scores = { 'MySQL': 1, 'PostgreSQl': 2, 'SQLite': 3, 'MongoDB': 4 } r_client.zadd(r_sorted_set, database_scores) print("...\r\nYou've successfully entered four database ratings.\r\n...") print("Database rating information: \r\n") print(r_client.zrange(r_sorted_set, 0, 3))
Save and close the file.
In the above application:
r_sorted_set
: Declares a key for the sorted setdatabase_scores = {...}
: Contains four elements with a matching scorer_client.zadd(r_sorted_set, database_scores)
: Adds the set elements to the Redis® serverr_client.zrange(r_sorted_set, 0, 3)
: Returns all sorted set values from the database
Run the application
$ python3 redis_sorted_set.py
Output:
... You've successfully entered four database ratings. ... Database rating information: [b'MySQL', b'PostgreSQl', b'SQLite', b'MongoDB']
Conclusion
In this guide, you have implemented different data types using a Vultr Managed Database for Caching with Python. Based on your application use case, implement the relevant Redis® data type to cache and store data in your database.
Next Steps
To integrate more solutions using your Vultr Managed Database for Caching, visit the following resources: