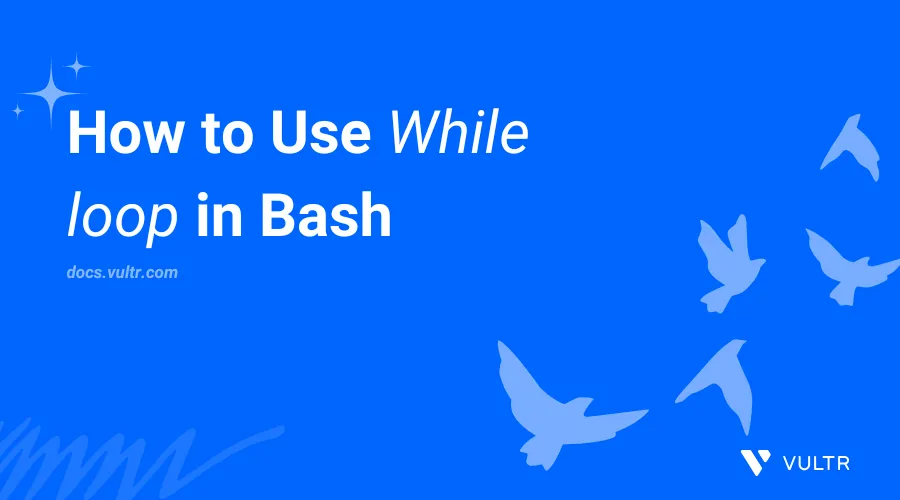
Introduction
Loops are programming constructs that continuously execute specific commands unless interrupted. For instance, the while
loop in Bash is a control structure that runs commands if a condition evaluates to true
. You can use while
loops to handle user input, read files, and execute a series of commands continuously.
This article explains how to use the while
loop in Bash for various tasks such as reading files, handling user input, and automating processes.
The while
Loop Command Syntax
The while
loop in Bash follows this syntax:
while [CONDITION]
do
# Commands
done
In the above command:
while
: Starts thewhile
loop.condition
: Sets the condition that you want to evaluate.do
: Marks the beginning of the loop's body including the commands to execute when the condition evaluates totrue
.done
: Marks the end of the loop and stops execution when the condition evaluates tofalse
.
Create while
Loops in Bash
Follow these steps to create a while loop
in Bash using a shell environment or script.
Create a new inline
while
loop to continuously increment a numeric variable's value up to a certain number, such as10
.console$ x=0; while [ $x -le 10 ]; do echo "The value of X is: $x"; ((x++)); done
Output:
The value of X is: 0 The value of X is: 1 The value of X is: 2 The value of X is: 3 The value of X is: 4 The value of X is: 5 The value of X is: 6 The value of X is: 7 The value of X is: 8 The value of X is: 9 The value of X is: 10
In the above inline
while
loop, the$x -le 10
condition evaluates totrue
if the variablex
is less than or equal to10
and outputs the current item's value while incrementing it using the((x++))
command. Inline while loops allow you to execute conditions in Bash without creating a script which is important when evaluating files or capturing real-time user input.Create a new
while.sh
script that tests if a variable is less than a number, such as10
, prints the current value, and increments the variable until the condition evaluates tofalse
.console$ nano while.sh
Add the following contents to the
while.sh
file.bash#!/bin/bash var=0 while [ $var -lt 10 ] do echo The current value: $var is less than 10 ((var++)) done
Save and close the file.
In this example, the
while loop
in Bash checks if the variable is less than10
, and outputs the current value.Run the script using Bash.
console$ bash while.sh
Output:
The current value: 0 is less than 10 The current value: 1 is less than 10 The current value: 2 is less than 10 The current value: 3 is less than 10 The current value: 4 is less than 10 The current value: 5 is less than 10 The current value: 6 is less than 10 The current value: 7 is less than 10 The current value: 8 is less than 10 The current value: 9 is less than 10
Create a new
while-script.sh
script.console$ nano while-script.sh
Add the following contents to the file to create a while loop that uses input redirection
<
instead of a condition to read the file contents line by line.bash#!/bin/bash while IFS= read -r line; do echo "$line" done < "/etc/passwd"
Save and close the file.
In the above script, the
while
loop uses the input redirection command<
to input the system user information file/etc/htpasswd
to theread
command and iterate over all lines in the file. TheIFS=
option keeps all whitespaces in the file.Run the script using Bash.
console$ bash while-script.sh
Output:
root:x:0:0:root:/root:/bin/bash daemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologin bin:x:2:2:bin:/bin:/usr/sbin/nologin sys:x:3:3:sys:/dev:/usr/sbin/nologin sync:x:4:65534:sync:/bin:/bin/sync games:x:5:60:games:/usr/games:/usr/sbin/nologin man:x:6:12:man:/var/cache/man:/usr/sbin/nologin lp:x:7:7:lp:/var/spool/lpd:/usr/sbin/nologin mail:x:8:8:mail:/var/mail:/usr/sbin/nologin news:x:9:9:news:/var/spool/news:/usr/sbin/nologin uucp:x:10:10:uucp:/var/spool/uucp:/usr/sbin/nologin proxy:x:13:13:proxy:/bin:/usr/sbin/nologin
Create Infinite while
Loops
Infinite While loops run indefinitely each time a condition evaluates to true
unless terminated or interrupted. The :
command creates an infinite loop that continuously executes a series of commands. Infinite loops are useful in logging and monitoring tasks that output continuous data. Follow the steps below to create an infinite while
loop that continuously runs unless you stop it using Ctrl + C.
Create a new
infinite.sh
script.console$ nano infinite.sh
Add the following contents to the
infinite.sh
file.bash#!/bin/bash while : do echo "This is an Infinite loop message, press <CTRL + C> to stop" sleep 1 done
Save and close the file.
In the above script, the
while
loop uses the:
option to create an infinite loop that outputs theThis is an Infinite loop message, press <CTRL + C> to stop
message. Thesleep 1
declaration instructs the loop to run every second.Run the script using Bash.
console$ bash infinite.sh
Output:
This is an Infinite loop message, press <CTRL + C> to stop This is an Infinite loop message, press <CTRL + C> to stop This is an Infinite loop message, press <CTRL + C> to stop This is an Infinite loop message, press <CTRL + C> to stop This is an Infinite loop message, press <CTRL + C> to stop
Create Nested while
Loops
Nested While loops execute multiple commands at different levels to perform complex iterative tasks. They allow you to use a loop inside another loop to handle complex tasks. Follow the steps below to create nested while
loops in Bash.
Create a new
nested.sh
script.console$ nano nested.sh
Add the following contents to the
nested.sh
file.bash#!/bin/bash app_name=("Apache" "Mysql" "Nginx" "Mysql" "PHP") var=0 while [ $var -lt ${#app_name[@]} ]; do j=0 while [ $j -lt ${#app_name[@]} ]; do if [ $var -ne $j ]; then echo "Pair: ${app_name[$var]} and ${app_name[$j]}" fi ((j++)) done ((var++)) done
Save and close the file.
In the above script, the nested
while
loop performs data pairing tasks by iterating over each element in theapp_name
array variable to pair non-identical package names. The outerwhile
loop iterates through all elements in the array, while the inner loop pairs each service with others using thej
variable value in the outer loop to compare the position of each element in the array.Run the script using Bash.
console$ bash nested.sh
Output:
Pair: Apache and Mysql Pair: Apache and Nginx Pair: Apache and Mysql Pair: Apache and PHP Pair: Mysql and Apache Pair: Mysql and Nginx Pair: Mysql and Mysql Pair: Mysql and PHP Pair: Nginx and Apache Pair: Nginx and Mysql Pair: Nginx and Mysql Pair: Nginx and PHP Pair: Mysql and Apache Pair: Mysql and Mysql Pair: Mysql and Nginx Pair: Mysql and PHP Pair: PHP and Apache Pair: PHP and Mysql Pair: PHP and Nginx Pair: PHP and Mysql
To control the flow of a while loop, conditional statements like if-else are essential. Visit the if-else in Bash guide for more information.
Use the break
Statement in while
Loops
The break
statement terminates and exits a loop when a condition evaluates to true
. In the following steps, use the break
statement to control the flow of a while
loop.
Create a new
break.sh
script.console$ nano break.sh
Add the following contents to the
break.sh
file.bash#!/bin/bash var=0 while [ $var -lt 10 ] do echo "Updated Value is: $var" ((var++)) if [[ "$var" == '10' ]]; then echo "The value is equal to 10, ending..." break fi done echo 'The loop test is complete!'
Save and close the file.
In the above script, the
break
statement terminates the loop when thevar
variable reaches10
and outputs theThe loop test is complete!
message when the loop stops.Run the script using Bash.
console$ bash break.sh
Output:
Updated Value is: 0 Updated Value is: 1 Updated Value is: 2 Updated Value is: 3 Updated Value is: 4 Updated Value is: 5 Updated Value is: 6 Updated Value is: 7 Updated Value is: 8 Updated Value is: 9 The value is equal to 10, ending... The loop test is complete!
Use the continue
Statement in while
Loops
The continue
statement stops the current iteration in a while
loop and starts the next iteration when a specific condition evaluates to true
. You can combine the continue
and if
statements to test for a specific result in a loop to start the next iteration without stopping the loop. Follow the steps below to use the continue
statement in a while
loop.
Create a new
continue.sh
script.console$ nano continue.sh
Add the following contents to the
continue.sh
file.bash#!/bin/bash var=0 while [ $var -lt 10 ] do echo "Updated Value is: $var" ((var++)) if [[ "$var" == '5' ]]; then echo "The value is equal to 5, skipping..." continue fi done
Save and close the file.
In the above script, the
while
loop uses anif
conditional statement to compare if the current value is equal to5
. Thecontinue
statement starts the next iteration and ignores other commands in the loop.Run the script using Bash.
console$ bash continue.sh
Output:
Updated Value is: 0 Updated Value is: 1 Updated Value is: 2 Updated Value is: 3 Updated Value is: 4 The value is equal to 5, skipping... Updated Value is: 5 Updated Value is: 6 Updated Value is: 7 Updated Value is: 8 Updated Value is: 9
Use while
Loops with Conditional Statements
Conditional statements (if
, case
, or if-else
) control a loop's flow by using the results of a loop's iteration to execute specific commands. You can also combine conditional statements with break
and continue
commands. In the following steps, use the if
statement to control a while
loop.
Create a new
if-while.sh
script.console$ nano if-while.sh
Add the following contents to the
if-while.sh
file.bash#!/bin/bash var=0 while [ $var -le 10 ]; do if (( var % 2 == 0 )); then echo "The value of $var is EVEN." else echo "The value of $var is ODD." fi ((var++)) done
Save and close the file.
The above script uses the
if-then
conditional statement in awhile
loop to check if the currentvar
variable value is divisible by2
to evaluate the result as odd or even.Run the script using Bash.
console$ bash if-while.sh
Output:
The value of 0 is EVEN. The value of 1 is ODD. The value of 2 is EVEN. The value of 3 is ODD. The value of 4 is EVEN. The value of 5 is ODD. The value of 6 is EVEN. The value of 7 is ODD. The value of 8 is EVEN. The value of 9 is ODD. The value of 10 is EVEN.
Use while
Loops with Functions
You can use while
loops with functions to create reusable commands and perform different tasks for each condition. Functions work with conditional statements within a loop to execute reusable code or commands at different intervals. Follow the steps below to use a while
loop with multiple functions to perform different actions whenever a condition evaluates to true
.
Create a new
functions-while.sh
script.console$ nano functions-while.sh
Add the following contents to the
functions-while.sh
file.bash#!/bin/bash read -p "Enter your application names (apache,nginx,mysql,php or help|-h): " -a app_name help_page() { echo "Usage: ./functions-while.sh [options]" echo echo "Options:" echo "apache" echo "nginx" echo "mysql" echo "php" echo " -h, --help Display this help page" } var=0 while [ $var -lt ${#app_name[@]} ]; do service="${app_name[$var]}" if [[ $service == "help" || $service == "-h" ]]; then help_page else case $service in "apache") echo "$service: works as the Web server" ;; "mysql") echo "$service: works as a Database server" ;; "nginx") echo "$service: works as a Reverse proxy or Web server" ;; "php") echo "$service: works as a Hypertext processor" ;; *) echo "$service: Unknown service" ;; esac fi ((var++)) done
Save and close the file.
In the above script, the
app_name
array stores elements from the user's input, and thewhile
loop iterates over each element in the indexed array that is less than thevar
variable's value. The loop uses anif-then
statement to evaluate if the current list item ishelp
or execute the matching pattern using acase
conditional statement. The((var++))
command increments the currentvar
variable value to iterate over all array elements.Run the script using Bash.
console$ bash functions-while.sh
Enter one or more application names, such as
apache
ormysql
when prompted.Enter your application names (apache,nginx,mysql,php or help|-h):
Output:
Enter your application names (apache,nginx,mysql,php or help|-h): apache mysql apache: works as the Web server mysql: works as a Database server
Conclusion
You have used the while loop in Bash to evaluate specific conditions and continuously execute tasks or commands. The while
loops are important when evaluating user inputs, variables, or multiple conditions to achieve a specific result. You can use while
loops with other Bash features, such as for
loops, conditional statements, arrays, and variables to run continuous tasks.
No comments yet.