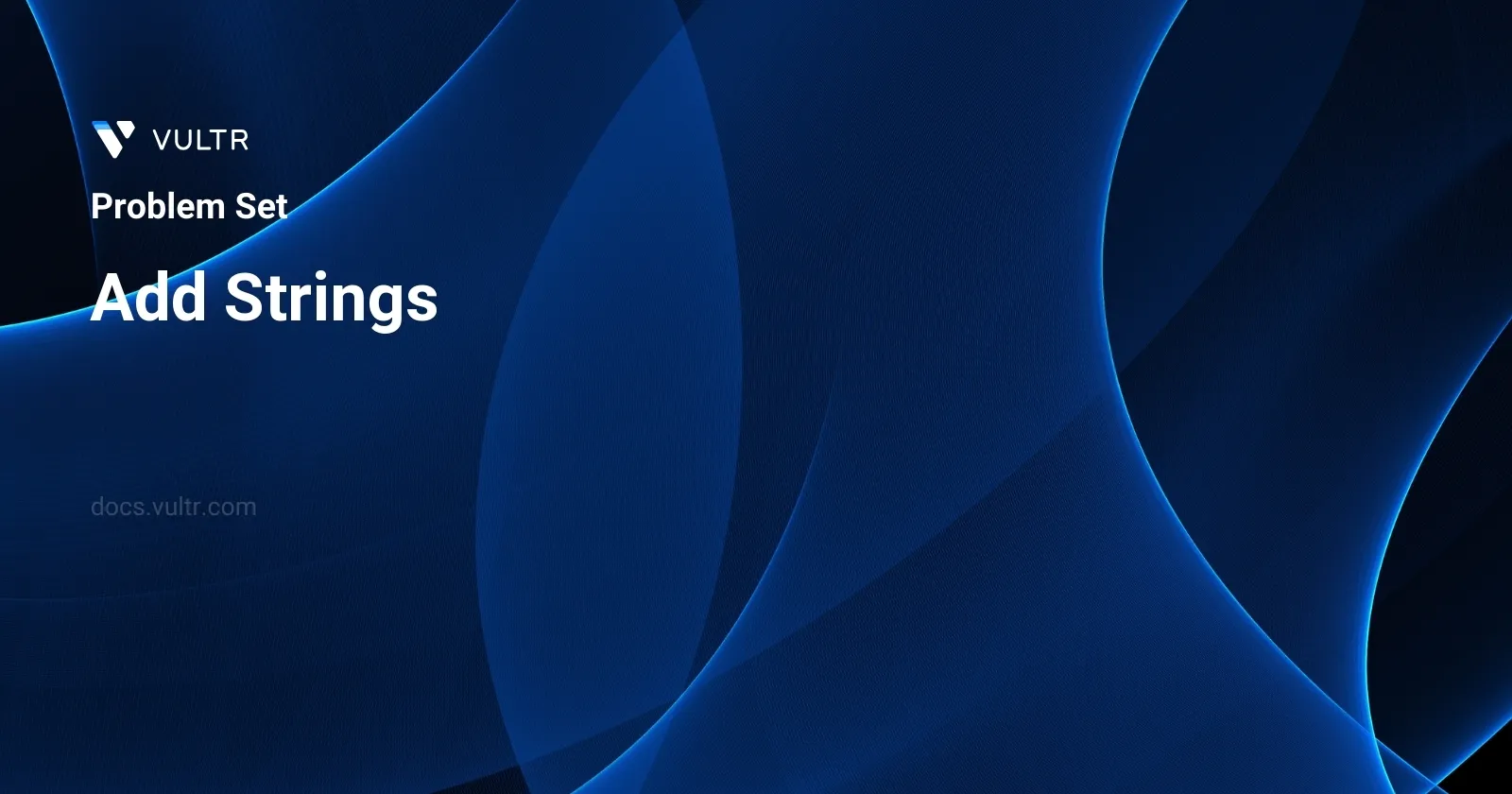
Problem Statement
The challenge presented requires adding two non-negative integers that are given as string representations and returning the result as a string. This must be accomplished without converting the strings directly to integer data types or utilizing any library designed to handle large integers such as BigInteger
. The solution demands a more fundamental approach to addition, likely necessitating an algorithm similar to how manual addition is performed, digit by digit, while managing carry-over values across digit boundaries.
Examples
Example 1
Input:
num1 = "11", num2 = "123"
Output:
"134"
Example 2
Input:
num1 = "456", num2 = "77"
Output:
"533"
Example 3
Input:
num1 = "0", num2 = "0"
Output:
"0"
Constraints
1 <= num1.length, num2.length <= 104
num1
andnum2
consist of only digits.num1
andnum2
don't have any leading zeros except for the zero itself.
Approach and Intuition
To sum two large numbers represented as strings without converting them directly into integers, we simulate the traditional column-by-column addition method taught in basic arithmetic. The process follows these logical steps:
- Initialize an empty result string and a variable to keep track of carry.
- Align the strings by their rightmost digits. This may involve padding the shorter string with leading zeros.
- Iterate through the digits of both strings from right to left (i.e., from least significant digit to most significant digit):
- Sum the digits at the current position along with any carry from the previous position.
- Compute the digit of the result by taking the modulo of the sum by 10.
- Update the carry for the next iteration as the division of the sum by 10.
- After processing both strings, check if there is any remaining carry and, if so, append it to the result.
- Since the result has been built from the least significant to the most significant digit, reverse the result string before returning it to get the correct order.
Considerations Based on Constraints:
- The lengths of both strings can vary widely, so the solution should cater to the situation where one string is significantly shorter than the other.
- Only digits are involved; therefore, no checks for illegal characters are required.
- We do not have to handle negative numbers or decimals, simplifying our task slightly.
- Solutions must handle arbitrary large lengths efficiently since the upper constraint allows for strings of length up to 104.
Through this method, we efficiently handle the addition of two arbitrary-length numbers represented as strings, respecting the constraints and requirements set forth.
Solutions
- Java
- Python
class Solution {
public String concatenateNumbers(String number1, String number2) {
StringBuilder resultBuilder = new StringBuilder();
int carryOver = 0;
int index1 = number1.length() - 1;
int index2 = number2.length() - 1;
while (index1 >= 0 || index2 >= 0) {
int digit1 = index1 >= 0 ? number1.charAt(index1) - '0' : 0;
int digit2 = index2 >= 0 ? number2.charAt(index2) - '0' : 0;
int sum = (digit1 + digit2 + carryOver) % 10;
carryOver = (digit1 + digit2 + carryOver) / 10;
resultBuilder.append(sum);
index1--;
index2--;
}
if (carryOver != 0)
resultBuilder.append(carryOver);
return resultBuilder.reverse().toString();
}
}
This Java solution defines a method concatenateNumbers
to add two numbers represented as strings without converting them to integers. The approach utilizes a StringBuilder
to efficiently build the resulting string. The algorithm works backwards from the least significant digit (rightmost) of both strings, adding corresponding digits along with any carry from the previous digit sum.
Here is a step-by-step breakdown of the algorithm:
- Initialize a
StringBuilder
calledresultBuilder
to store the resulting digits. - Initialize an integer
carryOver
to keep track of any carry obtained during the addition of two digits. - Use two index pointers,
index1
andindex2
, to traverse from the end of both number strings. - Enter a loop that continues until both index pointers have traversed all the digits of both strings.
- Determine the current digit of
number1
andnumber2
. If the index is below 0, use 0. - Calculate the sum of the two digits and the
carryOver
. - Compute the new carry by dividing the current sum by 10.
- Append the remainder of the current sum divided by 10 to the
resultBuilder
. - Decrement both
index1
andindex2
pointers.
- Determine the current digit of
- After exiting the loop, check if there is any remaining
carryOver
. If so, append it to theresultBuilder
. - Since digits have been appended in reverse order, reverse the contents of
resultBuilder
to obtain the correct order. - Return the resulting string which represents the sum.
This method accurately handles the addition of large numbers, bypassing any limitations on integer size, making it efficient and reliable for string-based arithmetic operations.
class Calculator:
def sum_numbers_as_strings(self, num1: str, num2: str) -> str:
result_list = []
carry_over = 0
pointer1 = len(num1) - 1
pointer2 = len(num2) - 1
while pointer1 >= 0 or pointer2 >= 0:
digit1 = ord(num1[pointer1]) - ord('0') if pointer1 >= 0 else 0
digit2 = ord(num2[pointer2]) - ord('0') if pointer2 >= 0 else 0
total = (digit1 + digit2 + carry_over) % 10
carry_over = (digit1 + digit2 + carry_over) // 10
result_list.append(total)
pointer1 -= 1
pointer2 -= 1
if carry_over:
result_list.append(carry_over)
return ''.join(str(x) for x in result_list[::-1])
This solution involves a Python class named Calculator
that features a method sum_numbers_as_strings
to add two numerical strings. The method accepts two string inputs, num1
and num2
, representing the numbers to be added. Here's how the solution operates step-by-step:
- Initialize
result_list
to store each computed digit of the final result andcarry_over
to keep track of any carry that needs to be added to the next digit. - Set
pointer1
andpointer2
to point to the last characters ofnum1
andnum2
respectively. - Use a
while
loop to iterate as long as there is a remaining character in either num1 or num2.- If the pointer is within the bounds of the string, convert the character at that position to its integer equivalent; otherwise, use zero.
- Calculate the sum of the current digits and the
carry_over
. Compute the digit to append toresult_list
and updatecarry_over
for the next iteration. - Decrease both pointers.
- After exiting the loop, check if there is a remaining
carry_over
and, if so, append it toresult_list
. - Generate the final result by reversing
result_list
and joining its elements into a single string.
This algorithm successfully handles the addition of large numbers represented as strings without the need for direct numeric conversion, ensuring it works efficiently even with very large number representations.
No comments yet.