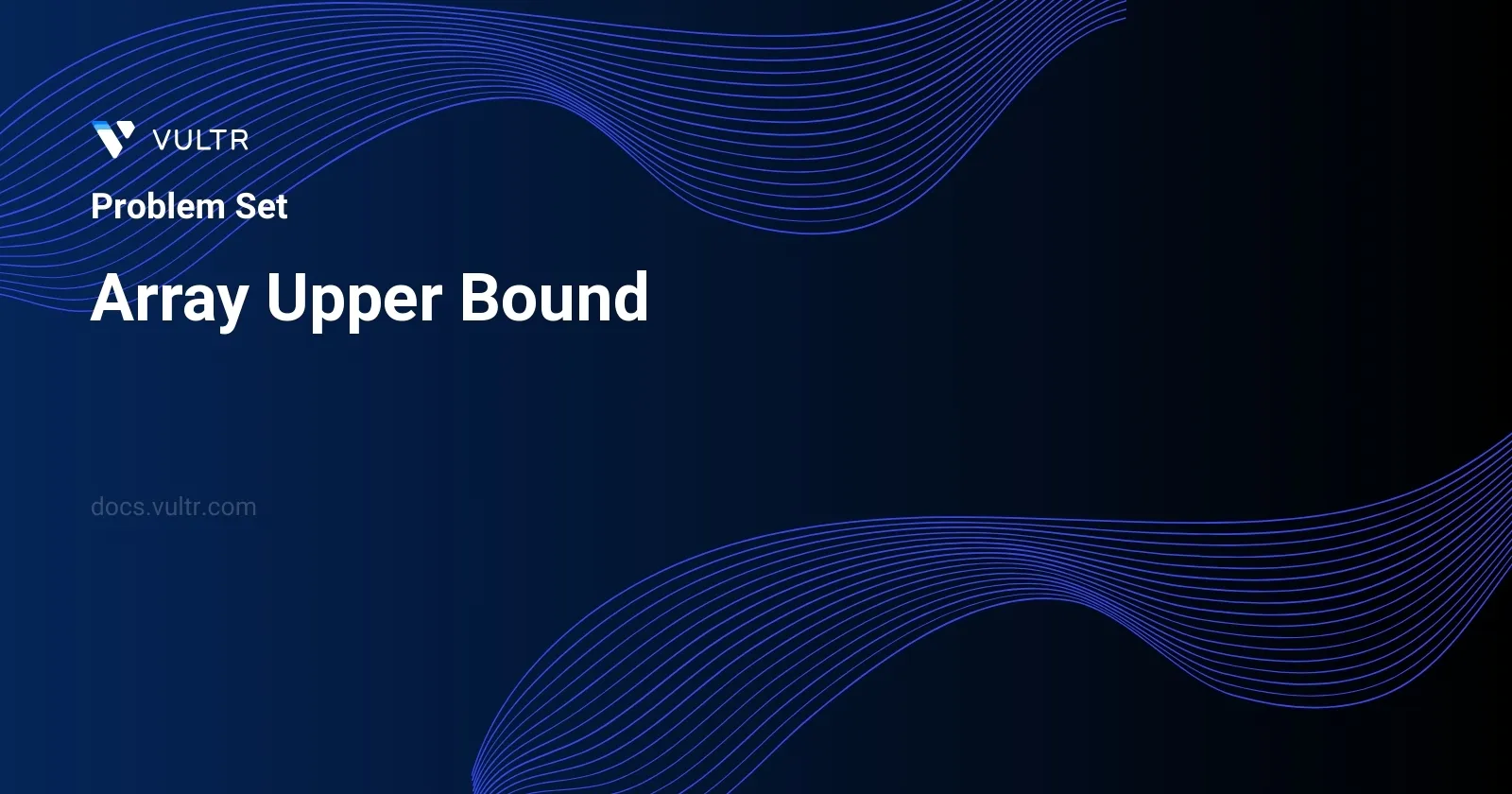
Problem Statement
The task is to implement a method named upperBound()
for arrays which efficiently finds the last index of a specified target number within an array. The arrays in question, nums
, are sorted in ascending order and may contain repeated elements. The upperBound()
method should return the last position of the target number if it is present in the array. However, if the target number does not exist in the array, the function should return -1
. The primary challenge here is implementing this search functionality in an optimized way, considering the possible large size of the input arrays and leveraging the sorted property of the arrays.
Examples
Example 1
Input:
nums = [3,4,5], target = 5
Output:
2
Explanation:
Last index of target value is 2
Example 2
Input:
nums = [1,4,5], target = 2
Output:
-1
Explanation:
Because there is no digit 2 in the array, return -1.
Example 3
Input:
nums = [3,4,6,6,6,6,7], target = 6
Output:
5
Explanation:
Last index of target value is 5
Constraints
1 <= nums.length <= 104
-104 <= nums[i], target <= 104
nums
is sorted in ascending order.
Approach and Intuition
The functionality of upperBound()
is to locate the last occurrence of the target value in a sorted array. Understanding and using the properties of the sorted array can make this search efficient:
Binary Search Approach:
- Using a modified binary search algorithm provides a logarithmic time complexity, which is suitable for constraints where the array length can go up to 10,000 elements. Instead of searching for the first occurrence of the target, we adapt the binary search to continue searching to the right, even after finding the target, to ensure we get the last occurrence.
- Initialize
low
to 0 andhigh
tonums.length - 1
. Whilelow
is less than or equal tohigh
, calculate themid
index. - If
nums[mid]
is less than the target, move thelow
pointer tomid + 1
. Ifnums[mid]
equals the target, set an answer variable tomid
and move thelow
pointer tomid + 1
to continue searching on the right side. - If
nums[mid]
is greater than the target, move thehigh
pointer tomid - 1
. - If the search completes and the target was found, return the position stored in the answer variable. If the target was not found at all, return
-1
.
Edge Cases Handling:
- When the array has only one element, directly check if it is equal to the target.
- If the target is outside the minimum and maximum bounds of the array, immediately return
-1
, thereby improving the efficiency by avoiding unnecessary search. - Ensure that the method efficiently handles arrays where all elements are the same as the target or none of the elements is the target.
This upperBound()
method leveraging a modified binary search exploits the fact that the input array is sorted, providing both efficient and clear handling of various scenarios detailed by the constraints.
Solutions
- JavaScript
Array.prototype.findUpperBound = function(value) {
return this.lastIndexOf(value);
};
The given JavaScript function extends the Array prototype to include a findUpperBound
method, which aims to find the upper bound of a specific value within an array. Specifically, this method utilizes the lastIndexOf()
function to return the highest index at which the specified value can be found in the array. This implementation assumes the array might contain multiple instances of the value, and the goal is to identify the last occurrence.
To utilize this method, follow these steps:
Ensure the array to which this method will be applied contains only sortable elements, like numbers or strings.
Call the
findUpperBound
function, passing the value for which the upper bound is to be determined.
Here's an example:
let numbers = [1, 2, 4, 4, 4, 5, 6];
console.log(numbers.findUpperBound(4)); // Output: 4
In this example, the findUpperBound
method successfully finds the last occurrence of the value 4
in the array, which is at index 4.
Remember, if the value does not exist in the array, Array.prototype.lastIndexOf()
returns -1
, indicating that the value is not found.
No comments yet.