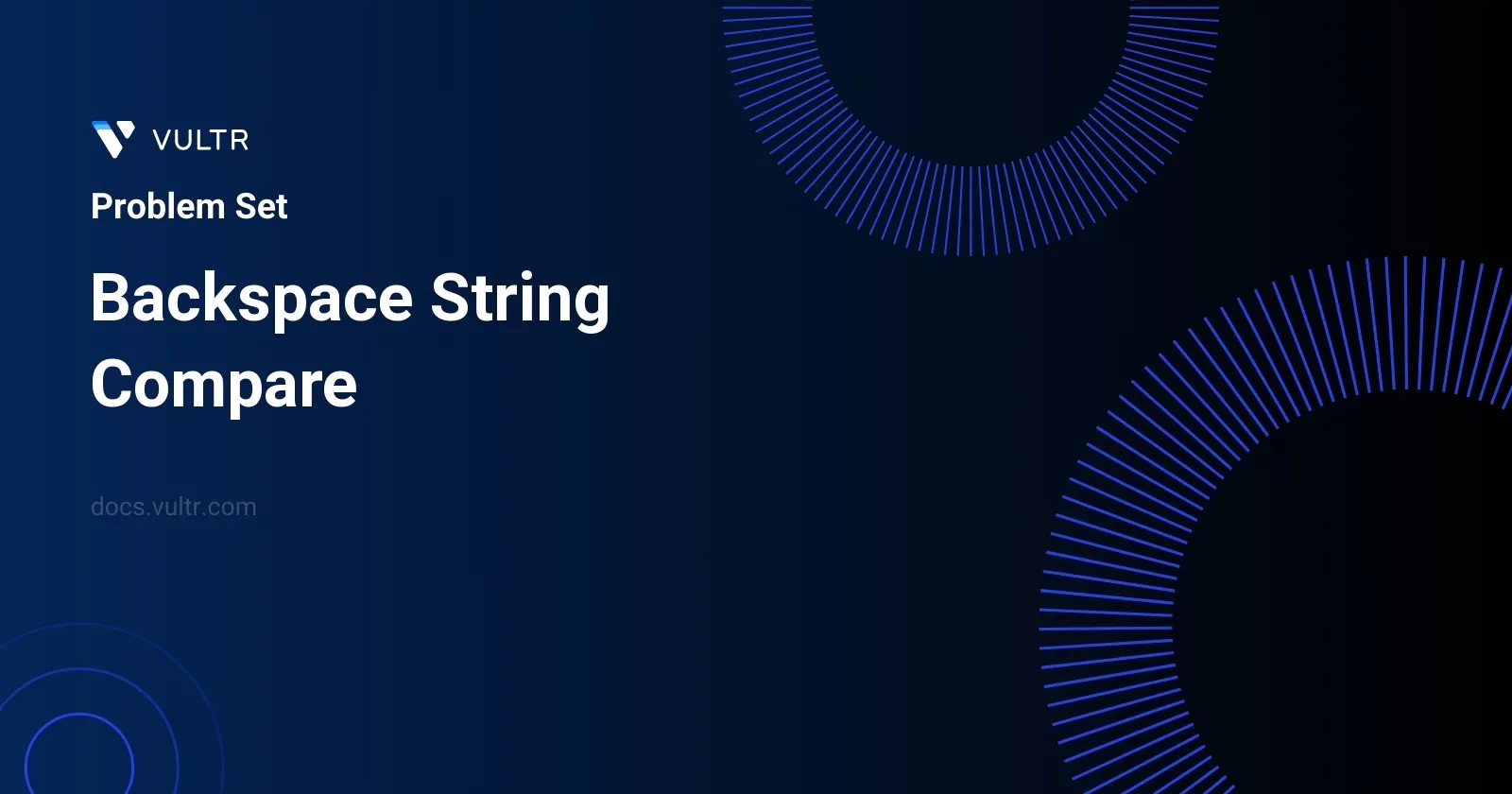
Problem Statement
The problem involves two strings, s
and t
, both of which simulate textual input into a text editor that supports the backspace character. Our task is to determine if, after fully processing both strings with all backspace characters taking effect, the resulting output from both strings is the same.
A backspace character, represented here as '#'
, results in the deletion of the character immediately preceding it, if there is one. If there is no character to delete (i.e., the string is empty or there have been multiple backspaces in succession), the backspace has no effect.
The challenge is to process these strings according to the rules of typing and backspacing in a text editor and to then compare the final forms of both strings to see if they are identical.
Examples
Example 1
Input:
s = "ab#c", t = "ad#c"
Output:
true
Explanation:
Both s and t become "ac".
Example 2
Input:
s = "ab##", t = "c#d#"
Output:
true
Explanation:
Both s and t become "".
Example 3
Input:
s = "a#c", t = "b"
Output:
false
Explanation:
s becomes "c" while t becomes "b".
Constraints
1 <= s.length, t.length <= 200
s
andt
only contain lowercase letters and'#'
characters.
Approach and Intuition
Based on the problem and examples provided, constructing the resulting string from the given input string while respecting the deletion caused by '#'
characters is crucial. The core intuition revolves around simulating this typing and backspacing process effectively. Here’s a basic approach to handle this:
Initialize Stack for Each String: Use a stack data structure to simulate the effect of typing and backspacing on both strings
s
andt
. Stacks are ideal for this as they easily allow us to add and remove characters at the end of the string.Process Each Character of Both Strings:
- For every character in the string:
- If the character is not
'#'
, push it onto its respective stack. - If the character is
'#'
and the stack is not empty, pop the top character of the stack. This simulates the backspace operation.
- If the character is not
- For every character in the string:
Convert Stack to String: Once all characters have been processed through the stack, convert the stack back to a string for both
s
andt
.Compare the Two Resulting Strings: If the strings obtained from both stacks are identical, return
true
as they represent the same text after all backspaces are taken into account. Otherwise, returnfalse
.
By leveraging a stack to process each string, we ensure that we manage the backspace operations efficiently, effectively modeling the behavior of a text editor. This approach is straightforward and ensures that our solution respects the constraints provided, specifically the bounds on the length of s
and t
and their character content. And this simulative approach fundamentally ensures that each character and backspace is processed as it would be in a real-time typing scenario.
Solutions
- Java
class Solution {
public boolean compareStrings(String str1, String str2) {
int index1 = str1.length() - 1, index2 = str2.length() - 1;
int backCount1 = 0, backCount2 = 0;
while (index1 >= 0 || index2 >= 0) {
while (index1 >= 0) {
if (str1.charAt(index1) == '#') {backCount1++; index1--;}
else if (backCount1 > 0) {backCount1--; index1--;}
else break;
}
while (index2 >= 0) {
if (str2.charAt(index2) == '#') {backCount2++; index2--;}
else if (backCount2 > 0) {backCount2--; index2--;}
else break;
}
if (index1 >= 0 && index2 >= 0 && str1.charAt(index1) != str2.charAt(index2))
return false;
if ((index1 >= 0) != (index2 >= 0))
return false;
index1--; index2--;
}
return true;
}
}
The "Backspace String Compare" program in Java provides a method to determine if two strings are equivalent after processing backspaces. This solution effectively simulates real-time string editing by comparing two strings character by character, accounting for the backspace character #
.
The function, compareStrings
, operates with time complexity O(N + M) where N and M are the lengths of the two input strings. It achieves this by traversing each string from the end to the beginning, which allows it to handle the backspaces appropriately.
- Initialize pointers for each string (
index1
andindex2
) set at their last characters. - Track the number of backspaces needed using
backCount1
andbackCount2
.
The core of the function involves looping through both strings simultaneously using the following logic:
- Decrement the index pointers appropriately when a
#
character is encountered, incrementing the respective backspace counter. - If a non-
#
character is encountered and the backspace count is more than zero, decrement the backspace counter and the index pointer. - If characters from both strings (at the current indices after adjusting for backspaces) do not match, or if one string is exhausted before the other, return
false
. - The loop exits when both strings have been completely traversed, taking all backspaces into account. If all corresponding characters matched, the function returns
true
.
This approach efficiently compares the intended final forms of the two strings despite any interspersed backspace characters, providing a clear and intuitive way to determine backspace-affect string equality.
No comments yet.