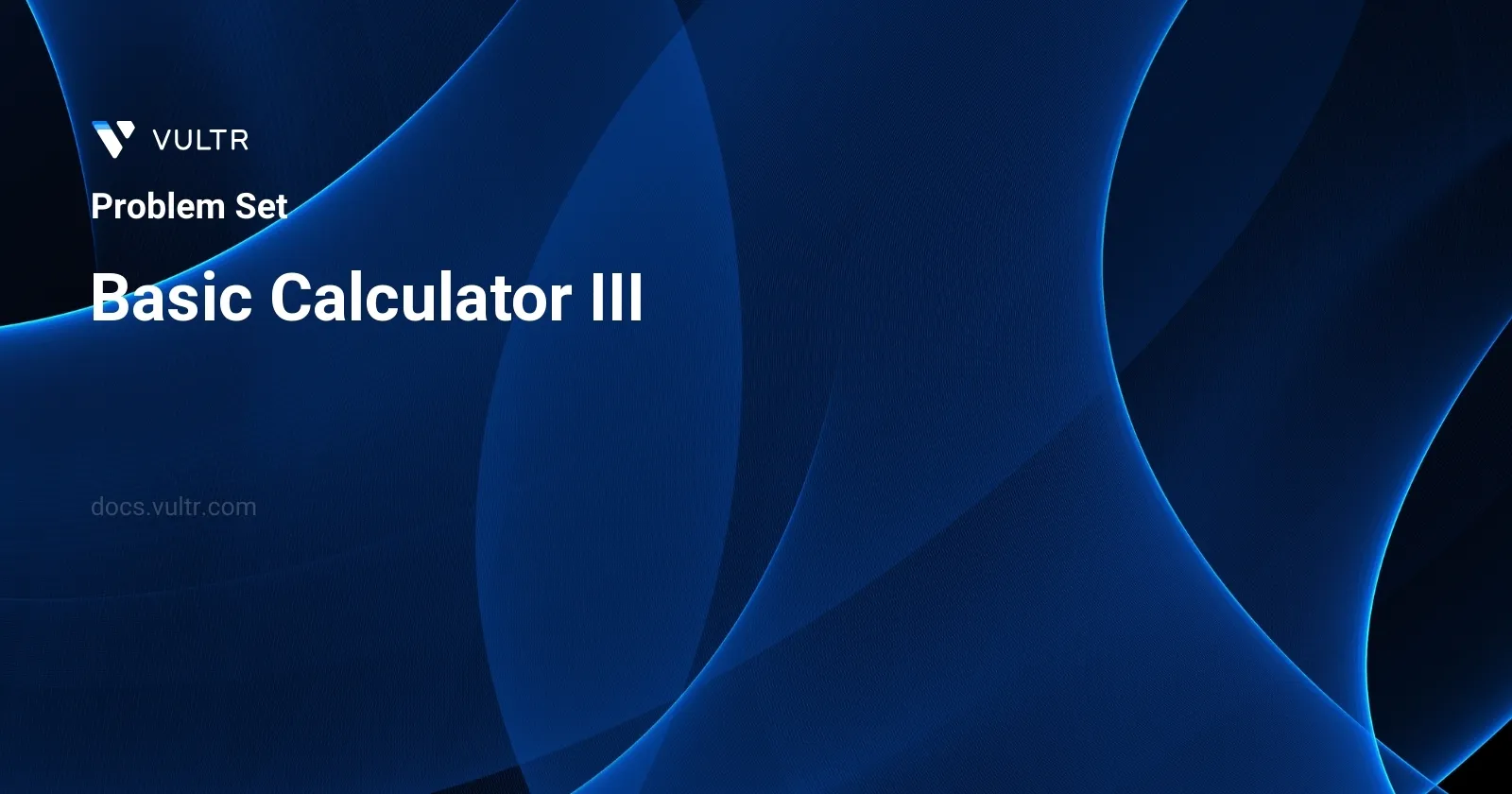
Problem Statement
In this task, we are required to develop a basic calculator that can evaluate simple mathematical expression strings. The expressions can include:
- Non-negative integers
- The four fundamental arithmetic operators: addition (
+
), subtraction (-
), multiplication (*
), and division (/
) - Parentheses for grouping (
(
and)
)
The division operation in these expressions should truncate any decimal part towards zero. This means that any resulting value from a division will be brought down to the nearest whole number towards zero.
It is important to highlight that the input expression will always be valid, which simplifies our task by not having to deal with syntax errors or invalid input scenarios. Additionally, the results of any intermediate calculations will fit within the signed 32-bit integer range.
The solution must be derived without the use of any built-in functions that automatically evaluate strings as mathematical expressions, such as the eval()
function in many programming environments.
Examples
Example 1
Input:
s = "1+1"
Output:
2
Example 2
Input:
s = "6-4/2"
Output:
4
Example 3
Input:
s = "2*(5+5*2)/3+(6/2+8)"
Output:
21
Constraints
1 <= s <= 104
s
consists of digits,'+'
,'-'
,'*'
,'/'
,'('
, and')'
.s
is a valid expression.
Approach and Intuition
Given the problem constraints and requirements, here is a general step-by-step approach to solving the expression:
Initialize a Stack Structure:
- Use a stack to manage operators and values as you parse through the string. This is crucial for honoring the precedence of operators and for properly evaluating expressions enclosed in parentheses.
Parse Through the String:
- Iterate over each character in the string. For numbers, you might need to read more than one character if the number has multiple digits.
- Handle the occurrence of spaces if any (usually, trimming the input string can be an initial step to simplify parsing).
Operation Handling:
- When an operator is encountered, decide whether to immediately evaluate the expression or push it onto the stack based on the operator's precedence and whether there are parentheses influencing the order of operations.
Implementing Operator Precedence and Associativity:
- Multiplication and division have higher precedence than addition and subtraction.
- Use the stack to adjust the calculation order. For example, if the current operator is
*
or/
, you might want to immediately evaluate it against the last number in the stack.
Handling Parentheses:
- Upon encountering a
(
, push it to the stack to signify a boundary. - Upon encountering a
)
, keep processing (popping and applying operations) until you reach a(
.
- Upon encountering a
Final Calculation:
- After parsing the entire string, there might still be some operators and operands in the stack that need to be processed in order to reach the final result.
Edge Handling
- Since the input guarantees valid strings, we don't need elaborate error handling for syntax. However, care must be taken to correctly handle the edge cases in mathematical operations, especially the integer division truncation towards zero.
By following these guidelines, one can construct an algorithm that evaluates a string expression respecting mathematical precedence and operator behavior without resorting to native expression evaluation functions.
Solutions
- Java
- Python
class Solution {
private int calculateValue(char op, int operand1, int operand2) {
if (op == '+') {
return operand1;
} else if (op == '-') {
return -operand1;
} else if (op == '*') {
return operand1 * operand2;
}
return operand1 / operand2;
}
private int processExpression(String expression, int[] index) {
Stack<Integer> numbers = new Stack<>();
int currentNumber = 0;
char lastOperator = '+';
while (index[0] < expression.length()) {
char character = expression.charAt(index[0]);
if (character == '(') {
index[0]++;
currentNumber = processExpression(expression, index);
} else if (Character.isDigit(character)) {
currentNumber = currentNumber * 10 + Character.getNumericValue(character);
} else {
if (lastOperator == '*' || lastOperator == '/') {
numbers.push(calculateValue(lastOperator, numbers.pop(), currentNumber));
} else {
numbers.push(calculateValue(lastOperator, currentNumber, 0));
}
if (character == ')') {
break;
}
currentNumber = 0;
lastOperator = character;
}
index[0]++;
}
int result = 0;
for (int value : numbers) {
result += value;
}
return result;
}
public int calculate(String s) {
s += "@";
int[] idx = new int[1];
return processExpression(s, idx);
}
}
This Java solution implements a calculator that can handle basic mathematical operations including addition, subtraction, multiplication, and division, as well as properly dealing with nested expressions enclosed in parentheses. The primary method calculate
prepares the input string and initiates the parsing of the expression by calling processExpression
, which recursively evaluates the string.
- Start by using a stack to manage numbers as they are processed.
- As characters are consumed from the expression:
- Detect and handle nested expressions recursively when encountering an opening parenthesis '('.
- Accumulate numeric values when a digit is found.
- Calculate intermediate results using basic arithmetic operations, depending on the last encountered operator which is stored and updated. Operators are properly managed with respect to their precedence and associativity.
- Handle closing parenthesis ')' to properly exit sub-expressions and continue evaluating the parent expression.
- The solution utilizes helper function
calculateValue
to perform the arithmetic operations depending on the last operator encountered. - After processing the entire expression, sum all values contained in the stack to produce the final result.
The solution ensures that operations are processed in the correct order, particularly handling the precedence of multiplication and division over addition and subtraction. This implementation effectively addresses the requirement of parsing and evaluating complex string-based arithmetic expressions with nested operations.
class Solver:
def compute(self, expr: str) -> int:
def calc(num1, num2, op):
if op == "+":
return num1
if op == "-":
return -num1
if op == "*":
return num1 * num2
return int(num1 / num2)
def process(index):
numbers = []
current_num = 0
last_op = "+"
while index[0] < len(expr):
char = expr[index[0]]
if char == "(":
index[0] += 1
current_num = process(index)
elif char.isdigit():
current_num = current_num * 10 + int(char)
else:
if last_op in "*/":
numbers.append(calc(numbers.pop(), current_num, last_op))
else:
numbers.append(calc(current_num, 0, last_op))
if char == ")":
break
current_num = 0
last_op = char
index[0] += 1
return sum(numbers)
expr += "@"
return process([0])
The provided Python code implements a class Solver
with a method compute()
to evaluate a mathematical expression passed as a string. This function can handle integers and the operators "+", "-", "*", and "/", including handling of parentheses for prioritizing operations.
You calculate expressions using the following approach:
Define a nested function
calc()
that takes two numbers and an operator, performing the basic arithmetic operation based on the given operator.Define another nested function
process()
which uses recursion for evaluating expressions, particularly for handling nested expressions within parentheses. The function iterates over the characters of the expression, evaluating sub-expressions recursively whenever opening parentheses are encountered.Within
process()
, manage a listnumbers
to store intermediate results, and usecurrent_num
to construct numbers digit by digit as parsed from the string.Use
last_op
to remember the last operator encountered, applying it tocurrent_num
and the previous results innumbers
, depending on whether the operator is a multiplication/division or addition/subtraction.The loop within
process()
deals with the characters one by one, updating the listnumbers
based on the operators. If a closing parenthesis or the end of the string is reached, break the loop and sum up all values innumbers
which holds the final result of the current (sub-)expression.Append a non-numeric character (e.g., "@") to the end of the expression to ensure the last number or operation is processed.
The main function call starts the processing with
process([0])
where0
is the initial index of the string.
With this implementation, ensure correct formation and ordering of operations, especially for expressions involving multiple types of operators and nested expressions. This solution will handle the parsing and computation of the input string as an arithmetic expression, returning the correct integer result.
No comments yet.