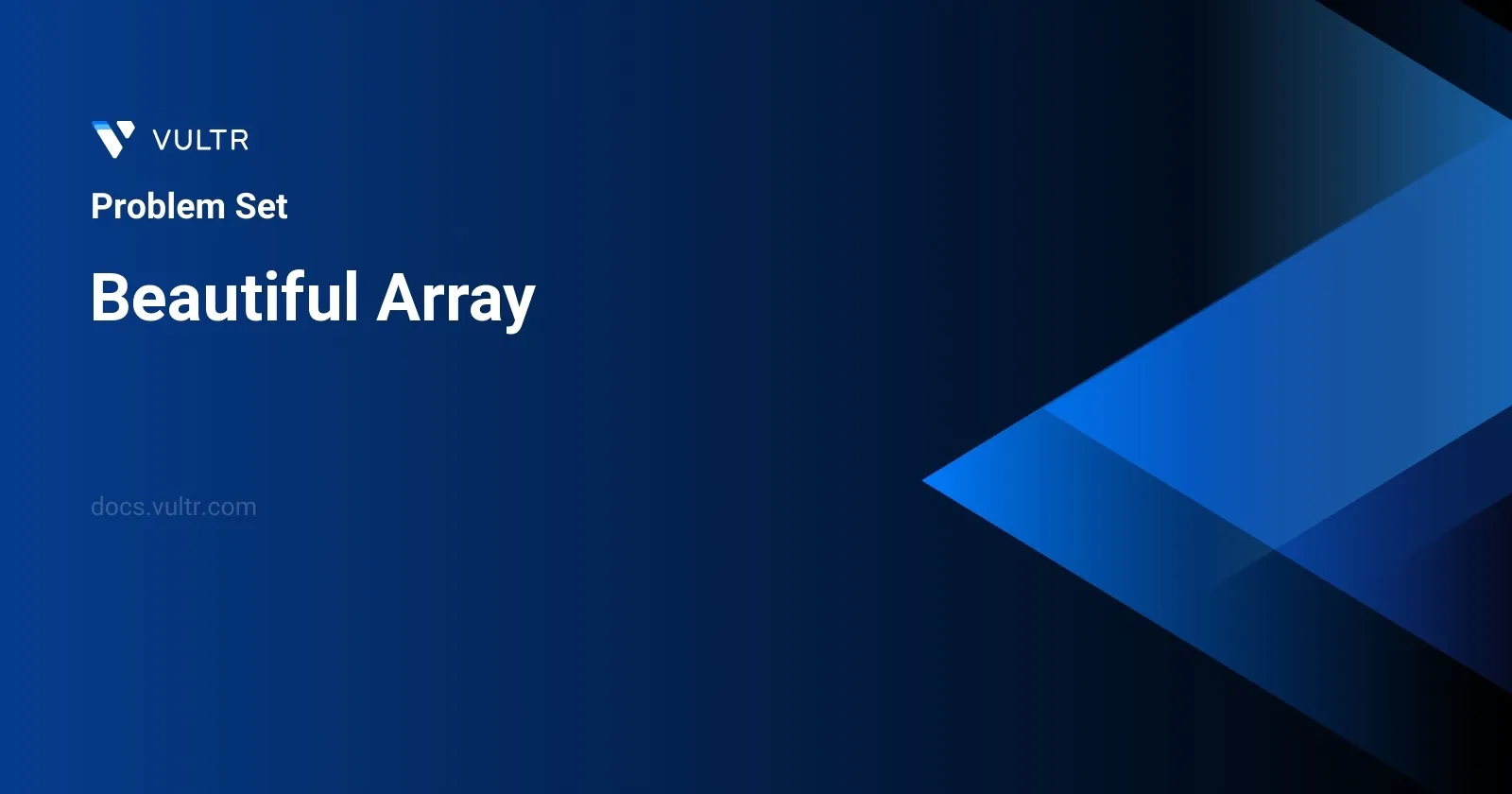
Problem Statement
An array nums
of a specific length n
is considered beautiful if it meets two primary conditions:
- The array is a permutation of the integers
1
ton
. In other words,nums
contains all integers from1
up ton
exactly once. - For any pair of indices
i
andj
such that0 <= i < j < n
, no intermediate indexk
(wherei < k < j
) satisfies the equation2 * nums[k] == nums[i] + nums[j]
. This requirement ensures that no middle element in the array is the strict average of two other elements that are on either side of it.
Given the integer n
, the task is to find and return any beautiful array nums
of length n
. There will always be at least one valid configuration for the specified n
.
Examples
Example 1
Input:
n = 4
Output:
[2,1,4,3]
Example 2
Input:
n = 5
Output:
[3,1,2,5,4]
Constraints
1 <= n <= 1000
Approach and Intuition
Understanding the approach to generate a beautiful array can be facilitated by the provided examples. Here's a breakdown based on the conditions:
Examples
Example 1
Input:
n = 4
Output:
[2,1,4,3]
Example 2
Input:
n = 5
Output:
[3,1,2,5,4]
Constraints
1 <= n <= 1000
Approach
The approach to constructing a beautiful array can be achieved by leveraging the divide and conquer strategy. Here's a breakdown of the steps:
- Start by considering two separate sequences, one containing all odd numbers and the other containing all even numbers within the range
[1, n]
. - Recursively apply the method to each sequence. A smaller instance of a beautiful array of odds or evens can itself be beautiful.
- Concatenate the results from the odd and even sequences to build the full beautiful array. This approach works because any conditions that might disrupt the "beautiful" arrangement are inherently avoided by separating odds and evens—the sums of an even and an odd number can never be exactly twice another number completely from odd or even subsets.
- For instance, if you split an array into [odd numbers] and [even numbers], the condition
2 * nums[k] == nums[i] + nums[j]
gets inherently sidestepped. Owing to the nature of evenness and oddness, adding two odds or two evens results in an even number, while doubling any single element from one set would always result in an even number too, hence the equation cannot be satisfied.
Intuition
- Permuting and checking every combination until the conditions are satisfied would be computationally expensive, especially as
n
grows. - The separation technique ensures there can be no
k
for which the condition2 * nums[k] == nums[i] + nums[j]
will hold, given how summing and doubling works within odds and evens. - The method is not just efficient but guarantees the fulfillment of conditions without needing extensive checking after array construction.
Solutions
- Java
class Solution {
Map<Integer, int[]> cache;
public int[] beautifulArray(int N) {
cache = new HashMap<>();
return generateArray(N);
}
public int[] generateArray(int N) {
if (cache.containsKey(N))
return cache.get(N);
int[] result = new int[N];
if (N == 1) {
result[0] = 1;
} else {
int idx = 0;
for (int element : generateArray((N + 1) / 2)) // odd numbers
result[idx++] = 2 * element - 1;
for (int element : generateArray(N / 2)) // even numbers
result[idx++] = 2 * element;
}
cache.put(N, result);
return result;
}
}
The provided Java solution implements a method to generate a "Beautiful Array." An array is termed beautiful if for every triplet (i, j, k)
in the array where i < j < k
, the condition nums[k] * 2 != nums[i] + nums[j]
is satisfied.
Here's an outline of how the solution works:
- The
beautifulArray
function initializes aHashMap
calledcache
to store intermediate results and calls thegenerateArray
function. generateArray
is a recursive function that:- Checks if the result for the given size
N
is already computed and stored incache
. If yes, it retrieves and returns the result fromcache
. - Initializes an array
result
to hold the elements of the currentN
-sized beautiful array. - If
N
equals 1, it returns an array[1]
since a single-element array is trivially beautiful. - Distributes the process of creating the beautiful array into generating arrays for odd and even indexed elements by recursively calling itself with adjusted sizes:
- The array of odd indexed elements is derived by calculating
2 * element - 1
for each element in the recursive call(N + 1) / 2
. - Similarly, the array for even indexed elements is generated by computing
2 * element
for each in the recursive callN / 2
.
- The array of odd indexed elements is derived by calculating
- Both results are combined, and the cumulative array
result
is stored incache
before being returned.
- Checks if the result for the given size
Using memoization via the cache
map optimizes the function by avoiding redundant calculations for previously encountered values of N
, thus making the function efficient for larger inputs. This approach leverages the property that a perfect mix of odds and evens, derived from smaller beautiful arrays, yields a larger beautiful array.
No comments yet.