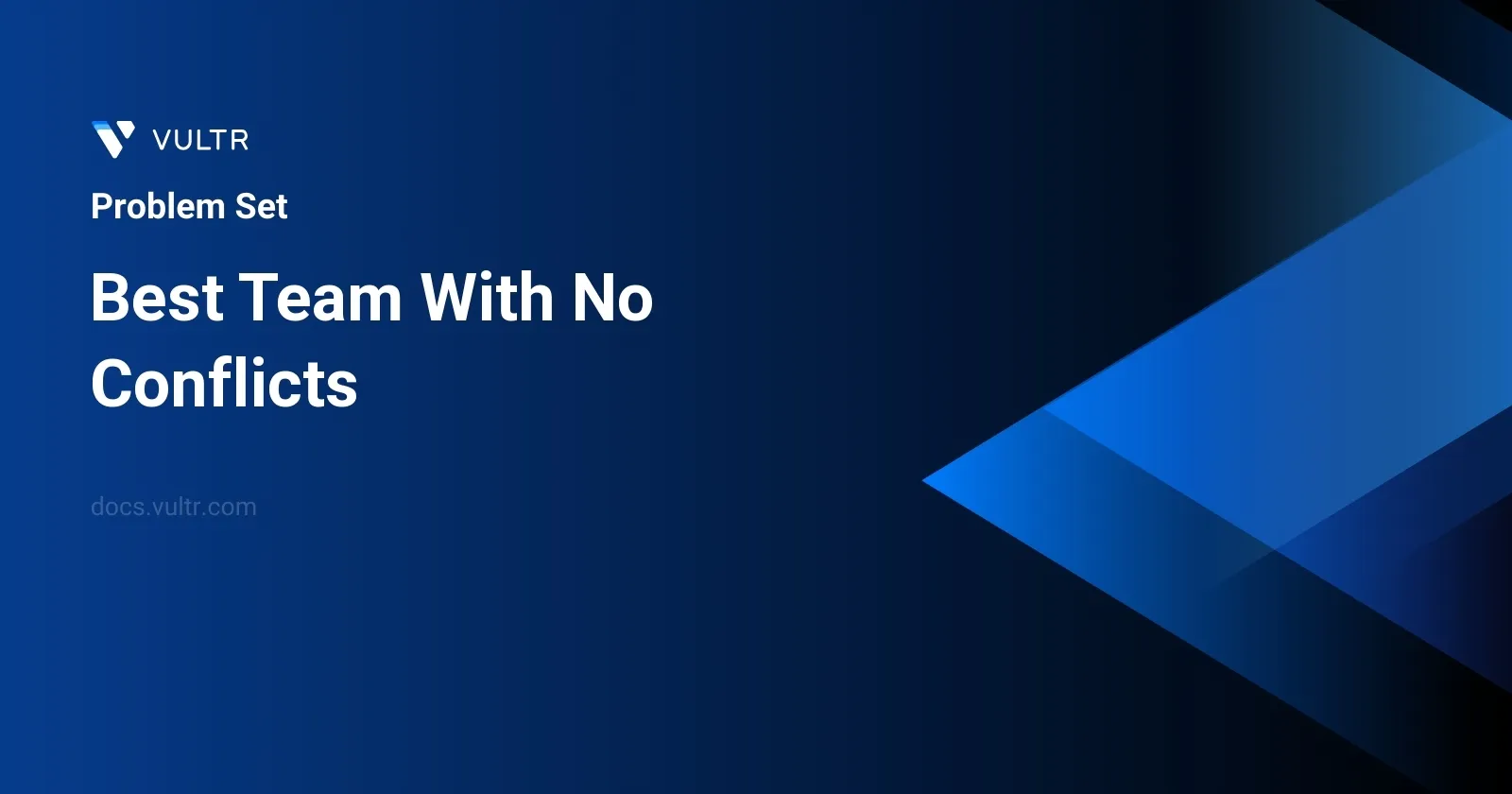
Problem Statement
In this problem, you are tasked with forming a basketball team with the highest possible score for an upcoming tournament. Each player on the team has a corresponding age and score. The challenge lies in selecting players such that the total score of the team is maximized while ensuring the team does not have any conflicts. A conflict arises if a younger player has a higher score than an older player. Players of the same age can be selected together without any conflict issue.
Given two lists, scores
and ages
, with each element representing the respective score and age of a player, you are to determine the maximum score achievable by any team that can be formed under these conditions.
Examples
Example 1
Input:
scores = [1,3,5,10,15], ages = [1,2,3,4,5]
Output:
34
Explanation:
You can choose all the players.
Example 2
Input:
scores = [4,5,6,5], ages = [2,1,2,1]
Output:
16
Explanation:
It is best to choose the last 3 players. Notice that you are allowed to choose multiple people of the same age.
Example 3
Input:
scores = [1,2,3,5], ages = [8,9,10,1]
Output:
6
Explanation:
It is best to choose the first 3 players.
Constraints
1 <= scores.length, ages.length <= 1000
scores.length == ages.length
1 <= scores[i] <= 106
1 <= ages[i] <= 1000
Approach and Intuition
When considering the solution for forming the optimal team, keep in mind the following insights and approach derived from the examples and constraints:
Firstly, pair up each player's score with their age. This linkage helps maintain a clear relationship between a player's age and their score, which is crucial for the solution.
Order or sort these pairs primarily by age and then by scores. This sorting will help in easy comparison and decision-making in subsequent steps as it naturally places older players before younger ones and within the same age, the better scores first.
Use a dynamic programming technique to determine the maximum score you can achieve when considering up to the i-th player. Define an array dp where
dp[i]
represents the maximum score possible with the first i players sorted as described.For each player, consider two scenarios:
- Including this player in the current team and check scores of all previous valid arrangements to ensure there's no conflict in terms of age and score.
- Not including this player in the team which means the score remains the same as the previous player's computation.
The transition for the dp would be to either take the score of the current player and add it to the best possible score of all previous players who do not create a conflict or to simply take the best score achieved by the previous player.
For a visual illustration using the given examples:
- For players sorted by age and score:
- Example 1: Easiest scenario, where each subsequent player is both older and better, means adding up all the scores gives the highest.
- Example 2: Selecting players of the same age or players who don't create a reverse situation in terms of scoring hierarchy is optimal.
- Example 3: Players need to be selected to ensure no younger player with a lower index in the sorted list has a higher score than an older.
Applying a thoughtful dynamic programming approach based on these observations will lead to an efficient solution that respects the constraint limits.
Solutions
- C++
- Java
class Solution {
public:
int optimalTeamScore(vector<int>& scores, vector<int>& ages) {
vector<pair<int, int>> combinedInfo;
for (int i = 0; i < scores.size(); i++) {
combinedInfo.push_back({scores[i], ages[i]});
}
// Sort pairs by scores then ages.
sort(combinedInfo.begin(), combinedInfo.end());
int maxAge = 0;
for (int age : ages) {
maxAge = max(maxAge, age);
}
vector<int> binaryIndexedTree(maxAge + 1, 0);
int maxScore = INT_MIN;
for (auto &info : combinedInfo) {
// Get the current maximum score achievable up to this age.
int currentScore = info.first + getMax(binaryIndexedTree, info.second);
// Update the binary indexed tree with this age's best score.
updateBIT(binaryIndexedTree, info.second, currentScore);
maxScore = max(maxScore, currentScore);
}
return maxScore;
}
// Function to retrieve the maximum score up to the specified age
int getMax(vector<int>& BIT, int age) {
int bestScore = INT_MIN;
for (int i = age; i > 0; i -= i & (-i)) {
bestScore = max(bestScore, BIT[i]);
}
return bestScore;
}
// Function to update the binary indexed tree
void updateBIT(vector<int>& BIT, int age, int score) {
for (int i = age; i < BIT.size(); i += i & (-i)) {
BIT[i] = max(BIT[i], score);
}
}
};
The solution focuses on finding the best team score without conflicts based on team members' scores and ages using a binary indexed tree (BIT) for efficient computation. Here's how the solution progresses:
- Combine each player's score and age into a pair and store these in a list.
- Sort this list to handle the team selection in an ordered manner.
- Determine the highest age to set up the appropriate data structure (BIT) with adequate size.
- Use the BIT to process each player optimally. For each player, based on their age, retrieve the maximum score achievable up to that age (using the
getMax
function). Update the BIT according to this new potential score using theupdateBIT
function. - Continuously track the maximum score found during these updates.
- Additional Functions:
- getMax: Uses age as the index and iterates backward over the BIT to find the maximum score up to that age.
- updateBIT: Updates the BIT with the potential maximum score for player's age and adjusts subsequent values accordingly.
By sorting players and employing a BIT for efficient maximum range queries and updates, this approach efficiently manages the optimal team selection problem. The utilization of C++ and its standard library functions helps streamline complex operations, like sorting and maximizing data efficiently.
class Solution {
public int maximumTeamScore(int[] scores, int[] ages) {
int len = ages.length;
int[][] pairs = new int[len][2];
for (int i = 0; i < len; i++) {
pairs[i][0] = scores[i];
pairs[i][1] = ages[i];
}
// Sort by scores first, then by ages.
Arrays.sort(pairs, (x,y) -> x[0] == y[0] ? x[1]-y[1] : x[0]-y[0]);
int maxAge = 0;
for (int age : ages) {
maxAge = Math.max(maxAge, age);
}
int[] fenwickTree = new int[maxAge + 1];
int maxScore = Integer.MIN_VALUE;
for (int[] pair : pairs) {
// Current best score including this player.
int scoreWithCurrent = pair[0] + getScoreUpToAge(fenwickTree, pair[1]);
// Update Fenwick Tree with the best score for this age.
updateFenwickTree(fenwickTree, pair[1], scoreWithCurrent);
maxScore = Math.max(maxScore, scoreWithCurrent);
}
return maxScore;
}
private int getScoreUpToAge(int[] tree, int age) {
int bestScore = Integer.MIN_VALUE;
while (age > 0) {
bestScore = Math.max(bestScore, tree[age]);
age -= age & (-age);
}
return bestScore;
}
private void updateFenwickTree(int[] tree, int age, int score) {
while (age < tree.length) {
tree[age] = Math.max(tree[age], score);
age += age & (-age);
}
}
}
The solution to the problem of forming the best team with no conflicts involves using dynamic programming with a Fenwick Tree (Binary Indexed Tree) for efficient computation of maximum scores. Here's a breakdown of the approach used in the Java solution:
- Start by extracting pairs of scores and ages from the input arrays. These pairs help in sorting and further processing.
- Sort these pairs primarily by scores and if there's a tie, by ages. This helps in ensuring that younger players with the same score aren't unfairly prioritized.
- Use a Fenwick Tree to keep track of the best scores up to a certain age, essentially enabling dynamic calculation of scores including the constraints imposed by ages.
- Iterate over each player, represented by a pair, and calculate the potential maximum score considering the players before them who do not conflict in terms of age and score.
- Update the Fenwick Tree as you compute scores for each player. This involves setting the score for a specific age only if it exceeds the previously stored score for that age, which is crucial in ensuring that the dynamic programming approach efficiently keeps track of the best scoring path.
- Extract the maximum score from these calculations as your result. This represents the highest possible team score given the constraints.
The use of a Fenick Tree is key as it facilitates quick updates and queries of the best scores up to certain ages, which are otherwise computationally expensive operations if done in simpler iterative manners. By leveraging both sorting and Fenwick Tree, the solution efficiently tackles the problem ensuring that both age and score conditions are respected leading to an optimal outcome.
No comments yet.