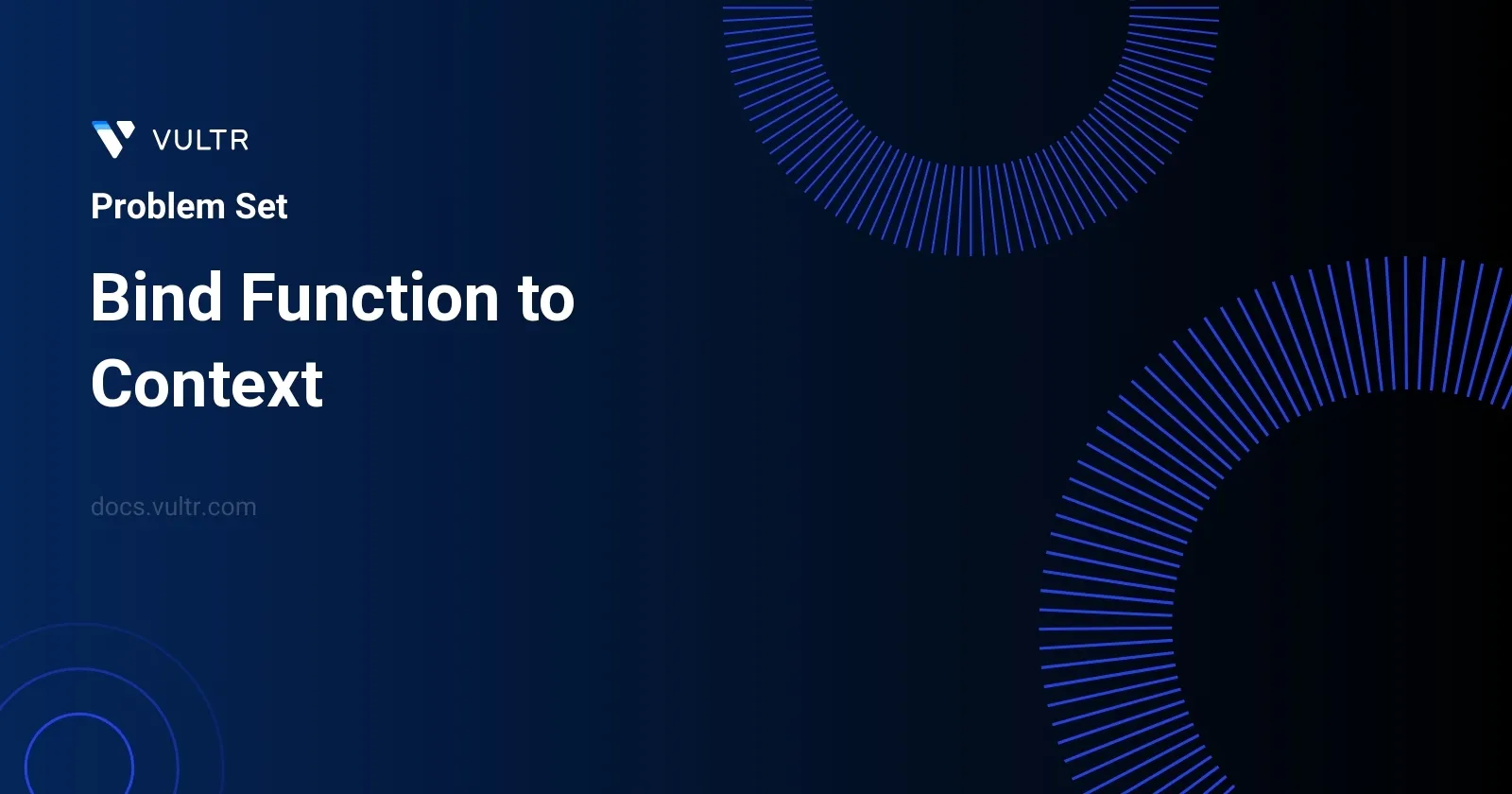
Problem Statement
The goal is to enhance the functionality of all JavaScript functions by adding a custom method named bindPolyfill
. This method allows attaching a specific object as the this
context to a function. When bindPolyfill
is invoked with an object obj
, this object is set as the new context (this
) of the function where bindPolyfill
is called.
Consider a function f
that uses this
to access one of its properties:
function f() {
console.log('My context is ' + this.ctx);
}
f();
Normally, calling f()
without any context binding would result in an output showing undefined
because this.ctx
is not defined. However, through our bindPolyfill
method:
const boundFunc = f.bindPolyfill({ "ctx": "My Object" });
boundFunc();
This changes the output to "My context is My Object"
by setting this
to the object { "ctx": "My Object" }
. Implementing bindPolyfill
must be done without using the existing Function.bind
built-in method.
Examples
Example 1
Input:
fn = function f(multiplier) { return this.x * multiplier; } obj = {"x": 10} inputs = [5]
Output:
50
Explanation:
const boundFunc = f.bindPolyfill({"x": 10}); boundFunc(5); // 50 A multiplier of 5 is passed as a parameter. The context is set to {"x": 10}. Multiplying those two numbers yields 50.
Example 2
Input:
fn = function speak() { return "My name is " + this.name; } obj = {"name": "Kathy"} inputs = []
Output:
"My name is Kathy"
Explanation:
const boundFunc = f.bindPolyfill({"name": "Kathy"}); boundFunc(); // "My name is Kathy"
Constraints
obj
is a non-null object0 <= inputs.length <= 100
Approach and Intuition
To tackle this problem effectively, let us consider the operation of bindPolyfill
step-by-step:
bindPolyfill
is a method added toFunction.prototype
so that it is available to all functions.When a function calls
bindPolyfill
, it should take an objectobj
and optionally further arguments to be passed. The core operation is that it will return a new function.Within this new function, the original function should be called using
Function.prototype.apply()
orFunction.prototype.call()
, which allows explicitly setting thethis
value.apply()
will be beneficial as it can take an array of arguments, fitting well with the possibility of the original function accepting multiple parameters, as noted in the constraints (0 <= inputs.length <= 100
).
The examples provided illustrate typical scenarios where:
In the first example, a method expecting a multiplier uses a custom context to multiply a property value (
this.x
). After binding, calling the function with a parameter correctly computes the result using the bound context.In the second example, a simple method returns a string that includes a property from its context. Once bound with an object, it accurately reflects the new bound context when called.
These examples emphasize understanding how context (this
keyword) manipulation works in JavaScript and how bound functions can be created to operate with designated contexts, enhancing modularity and reusability of functions.
Solutions
- JavaScript
Function.prototype.myBind = function(context) {
return (...args) => this.apply(context, args);
}
This JavaScript code snippet addresses how to bind a function to a specific context manually. It involves adding a method myBind
to the Function.prototype
, making it available to all function objects.
- Start by defining
Function.prototype.myBind
which takes a single parameter:context
. Thiscontext
refers to the object that the function should be associated with when called. - The method returns a new function. This returned function is created using an arrow function, which inherently does not have its own
this
context; instead, it captures thethis
context of the enclosing function scope. - Within the arrow function, use the function's
apply
method. Theapply
method calls the original function settingthis
to the provided context (context
) and accepting an array of arguments (...args
). - By calling
.myBind(context)
, any function can be attached dynamically to the specified objectcontext
. When the binded function is then called, it executes in the scope ofcontext
with all passed arguments.
This enhancement mirrors the functionality of the native JavaScript bind
function, providing flexibility in function context management in user-defined methods or scenarios where modifying function scope is necessary.
No comments yet.