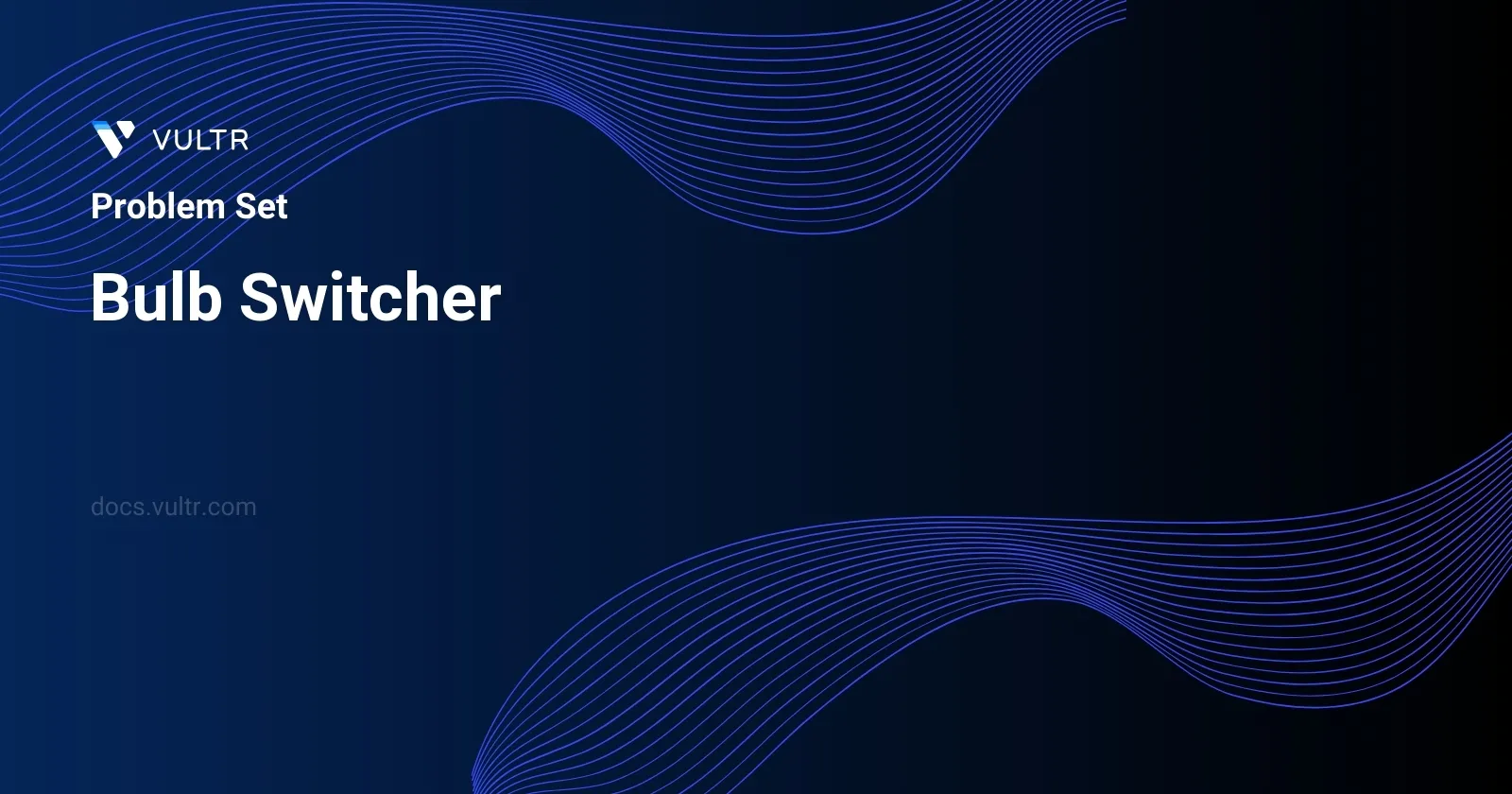
Problem Statement
The bulb toggling puzzle consists of n
bulbs lined up in a row, all initially turned off. The state of these bulbs is altered over a series of rounds, specifically n
rounds in total. Each round, i
, involves toggling the state of every i
th bulb. A bulb's state is toggled by switching it from off to on or from on to off. Thus, for a given round i
:
- First round: every bulb is toggled (all bulbs turn on).
- Second round: every second bulb is toggled (i.e., bulbs 2, 4, 6, …).
- Third round: every third bulb is toggled (i.e., bulbs 3, 6, 9, …).
- This process continues up to the
n
th round where only then
th bulb is toggled.
The challenge is to determine how many bulbs are left in the "on" position after all n
rounds are completed.
Examples
Example 1
Input:
n = 3
Output:
1
Explanation:
At first, the three bulbs are [off, off, off]. After the first round, the three bulbs are [on, on, on]. After the second round, the three bulbs are [on, off, on]. After the third round, the three bulbs are [on, off, off]. So you should return 1 because there is only one bulb is on.
Example 2
Input:
n = 0
Output:
0
Example 3
Input:
n = 1
Output:
1
Constraints
0 <= n <= 109
Approach and Intuition
The pattern of bulbs being toggled in multiple rounds is quite systematic, leading us to some interesting observations particularly about the factors of numbers:
- In every round
i
, thei
th bulb's status is toggled. - For any bulb
j
, it will be toggled for each divisor it has. For example, bulb 12 is toggled in rounds 1, 2, 3, 4, 6, and 12. - Bulbs that are toggled an odd number of times will end up being on, and those toggled an even number of times will be off.
Observing patterns in factors:
- A bulb ends up on only if it has an odd number of total divisors. The numbers with an odd number of divisors are perfect squares. This is because divisors usually come in pairs, except when the number is a perfect square.
Consequently:
- The number of bulbs that remain on after all rounds can be determined by counting how many numbers up to
n
are perfect squares.
Given this understanding:
- To find how many bulbs are on, identify all numbers up to
n
that are perfect squares. The count of these perfect squares gives the answer. - Mathematically, the count of perfect squares up to
n
is the integer part of the square root ofn
.
Thus, the problem simplifies to calculating how many perfect squares exist up to n
, which can be done efficiently without simulating all the rounds.
Solutions
- C++
- Java
- C
- JavaScript
- Python
class Solution {
public:
int switchBulbs(int bulbs) {
return sqrt(bulbs);
}
};
When designing a solution for the "Bulb Switcher" problem in C++, note that the optimal approach involves leveraging the properties of squares to determine how many bulbs remain on. The core insight rests on the observation that bulbs toggled an odd number of times remain on, which happens only for bulbs corresponding to perfect square positions since divisors are paired except for squares with a single middle divisor.
Here's a concise breakdown of implementing this solution:
- Define a function
switchBulbs
in a solution class which accepts one integer parameter,bulbs
. - The function simply returns the integer part of the square root of
bulbs
.
This square root essentially counts the number of perfect square numbers from 1 to bulbs
because each perfect square bulb is the only type that will be toggled an odd number of times (i.e., will remain on).
Remember to include the cmath
library in your code to use the sqrt
function, ensuring the calculation accurately provides the integer square root.
This approach covers:
- Efficient calculation using a mathematical understanding of the problem.
- Minimal computation, with a time complexity of O(1) thanks to the direct utilization of the
sqrt
function.
class Solution {
public int switchBulb(int bulbs) {
return (int) Math.sqrt(bulbs);
}
}
The solution summary for the "Bulb Switcher" problem is straightforward. This Java solution employs a simple mathematical approach to determine the number of bulbs that remain on after some operations based on their initial state.
The switchBulb
method takes an integer bulbs
as the parameter, representing the total number of bulbs. The essence of the solution involves calculating the square root of the total bulbs, and then returning that value. The square root calculation helps determine the count of bulbs that will remain on due to the perfect square toggling effect where bulbs are toggled during every factor of their position number, causing bulbs at perfect square positions to end toggled only an odd number of times, thus remaining on.
The key components of the method include:
- Using the
Math.sqrt()
function to calculate the square root. - Casting the result of
Math.sqrt()
toint
to ensure that the function returns a valid integer value fitting the method's return type.
This concise method effectively and efficiently solves the problem using mathematical properties related to factors and toggles associated with perfect squares.
int lightBulbSwitcher(int total) {
return sqrt(total);
}
The solution presented focuses on a problem commonly referred to as the "Bulb Switcher". This involves determining the number of bulbs left in the "on" position after performing a series of toggle operations according to specific rules. The solution is implemented in C.
The function lightBulbSwitcher(int total)
calculates the number of bulbs that remain on by applying the square root to the total number of bulbs. This operation directly corresponds to identifying the number of perfect squares up to total
, since only bulbs at positions that are perfect square numbers will remain on after all toggles are complete. Here's a breakdown of the function:
- The function takes an integer
total
, which represents the total number of bulbs. - It returns the integer value of the square root of
total
. This is achieved using thesqrt
function, which computes the square root.
This method efficiently determines the result without iterating over all the bulbs, leveraging mathematical properties instead for a quick computation.
var toggleBulb = function(count) {
return Math.floor(Math.sqrt(count));
};
This solution pertains to the "Bulb Switcher" problem where the objective is to determine the number of bulbs that remain on after n toggles on n bulbs. The solution is implemented in JavaScript.
The function named toggleBulb
receives an input parameter count
, which represents the total number of bulbs. The function calculates the number of bulbs that remain on by taking the square root of count
and then applying the Math.floor()
function to round down to the nearest whole number. This calculation directly determines the number of bulbs that will still be on after all bulbs have been toggled.
Key observations for understanding this approach:
- Each bulb is toggled in rounds that correspond to their number factors.
- A bulb ends up being on if it is toggled an odd number of times.
- Bulbs that have an odd number of total divisors (which happens when the number is a perfect square) will remain on, hence taking the square root gives the count of such bulbs up to
count
.
This concise implementation effectively solves the problem by leveraging mathematical properties, maximizing efficiency with minimal computational steps.
class Solution:
def switchBulbs(self, total: int) -> int:
return int(total**0.5)
This summary guides you through the solution for the Bulb Switcher problem using Python. The problem involves determining the number of bulbs that remain on after all bulbs have been toggled following a specific sequence. The solution takes advantage of a mathematical insight related to perfect squares to efficiently compute the outcome.
In the given Python code:
- Define a class named
Solution
. - Within this class, define a method
switchBulbs
that accepts an integertotal
representing the total number of bulbs. - The method calculates the square root of the total number of bulbs, converts it to an integer, and returns this value. This integer represents the count of bulbs that remain on after the toggle process.
The key to understanding this approach lies in recognizing that only bulbs at the positions of perfect squares will be toggled an odd number of times (hence remain on), because they have an odd number of divisors. Other bulbs are toggled an even number of times, turning them off. Thus, simply computing the square root of the total number of bulbs yields the count of bulbs that stay on, as each square from 1 to the integer part of the square root corresponds uniquely to a bulb that remains on.
No comments yet.