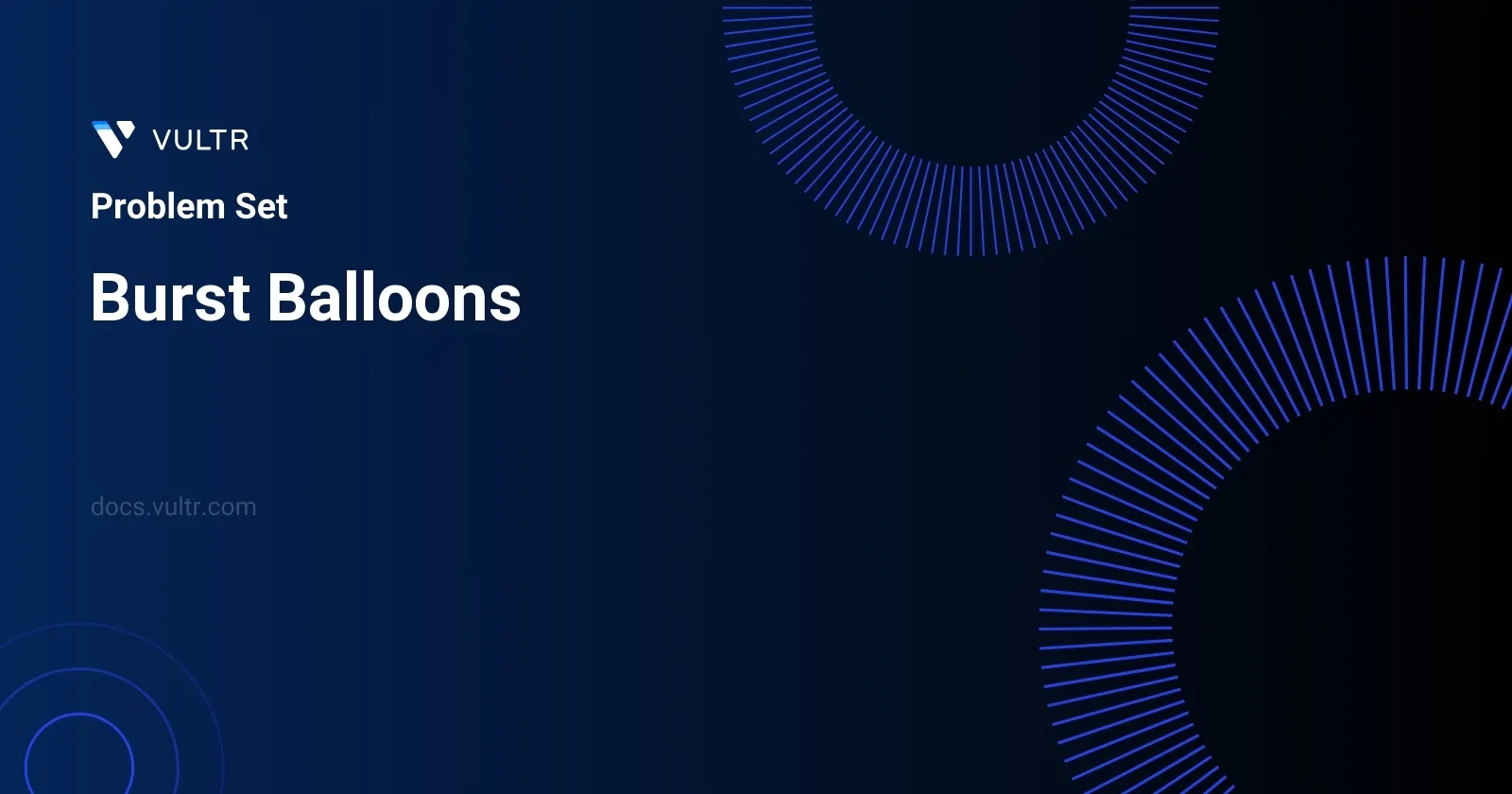
Problem Statement
In this task, you are equipped with n
balloons, each sequentially indexed from 0
to n - 1
. Every balloon is distinctly marked with a numeral on it, captured within an array termed nums
. The ultimate goal here extends beyond just bursting these balloons; it is to strategize the bursting sequence to maximize scoring potential. Each time a balloon is burst (let's say the i
th balloon), you earn coins equivalent to the product of the numbers on three balloons: the current balloon i
, and its immediate neighbors i-1
and i+1
. Importantly, if these neighboring positions exceed the array boundary, a default value of 1
should be considered for the calculations. The challenge intensifies as you need to determine the sequence that yields the highest coin accumulation by the end of all bursts.
Examples
Example 1
Input:
nums = [3,1,5,8]
Output:
167
Explanation:
nums = [3,1,5,8] --> [3,5,8] --> [3,8] --> [8] --> [] coins = 3*1*5 + 3*5*8 + 1*3*8 + 1*8*1 = 167
Example 2
Input:
nums = [1,5]
Output:
10
Constraints
n == nums.length
1 <= n <= 300
0 <= nums[i] <= 100
Approach and Intuition
Game Plan
- Understanding and visualizing the problem as a dynamic programming scenario, where a subproblem corresponds to maximizing the coins from bursting a subsequence of balloons.
- Recognizing that if we burst a balloon last in a sub-interval, the coins obtained for bursting it would not be affected by the outside. Its left and right neighbors in the sub-interval become the effective neighbors, simplifying the problem for every sub-interval.
- Implementing a table
dp
wheredp[i][j]
will store the maximum coins obtained by bursting all the balloons between indexi
andj
, inclusive.
Principle Details
- Initialization: For balloons with no neighbors, earning is simply the product of neighboring values treated as
1
if no actual neighbors exist. These are base cases for our dynamic programming approach. - Transition: For each interval
[i, j]
, consider every balloonk
within this interval as the last balloon to burst. Compute the coinsnums[i-1] * nums[k] * nums[j+1]
adding the results from subproblemsdp[i][k-1]
anddp[k+1][j]
. - Result Retrieval: After evaluating all subintervals and their burst sequences,
dp[0][n-1]
yields the maximum coins that can be gathered.
Example Walkthrough
- For
nums = [3,1,5,8]
, the interval assessment would compute values for smaller lengths and gradually build up. Bursting1
(nums[1]
) first followed by5
and then3
ends with8
to gather a sum of167
. - This strategy ensures optimal score accumulation by strategically choosing which balloon to burst last in every possible subarray of balloons.
By tackling this problem with a dynamic approach, focusing on smaller subproblems and gradually building up to the entire problem size, one can efficiently determine the best strategy to burst balloons for maximum score.
Solutions
- C++
- Java
- Python
class Solution {
public:
int maxBurstEarn(vector<int>& balloons) {
balloons.insert(balloons.begin(), 1);
balloons.push_back(1);
vector<vector<int>> profit(balloons.size(), vector<int>(balloons.size(), 0));
for (int start = balloons.size() - 2; start >= 1; --start) {
for (int end = start; end <= balloons.size() - 2; ++end) {
for (int last = start; last <= end; ++last) {
int earned = balloons[start - 1] * balloons[last] * balloons[end + 1];
int sum = profit[start][last - 1] + profit[last + 1][end];
profit[start][end] = max(profit[start][end], earned + sum);
}
}
}
return profit[1][balloons.size() - 2];
}
};
The provided C++ program is a solution to the problem of maximizing earnings from bursting balloons. The balloons are represented as an integer array where each integer denotes the earnings if that particular balloon is burst.
The primary method in the Solution
class, maxBurstEarn
, utilizes dynamic programming to calculate the maximum earnings. The approach involves inserting dummy balloons with a value of 1
at both the beginning and the end of the array. This facilitates the calculation without having to handle special cases at the array boundaries.
Here's an explanation of the key steps and the dynamic programming table used (profit
):
Initialization:
- Start by modifying the
balloons
vector to include1
at both ends. - Define a 2D vector,
profit
, initialized with zeros. The dimensions ofprofit
are the size of theballoons
vector by the size of theballoons
vector. This table is used to store the maximum earnings possible by bursting all balloons between indicesi
andj
.
- Start by modifying the
Dynamic Programming Table Filling:
- Traverse the
balloons
array from the second last index down to the second index (start
index from last to first actual balloon). - For each
start
, iterateend
fromstart
to the second last index. - Inside the nested loop, determine the best balloon (
last
) to burst last in the range[start, end]
. - Calculate the potential earned value if
last
is the final balloon to burst in[start, end]
which includes:- Precomputed maximum profits from segments
[start, last-1]
and[last+1, end]
. - The product of the values of the balloons at
start-1
,last
, andend+1
.
- Precomputed maximum profits from segments
- Maintain the maximum earnings in the
profit
table.
- Traverse the
Result Extraction:
- At the end of the iterations,
profit[1][balloons.size()-2]
stores the maximum earnings by bursting all balloons (except the dummy ones).
- At the end of the iterations,
This efficient dynamic programming solution ensures that you carefully evaluate each possible scenario of bursting balloons to maximize earnings from the sequence, reducing the complexity significantly compared to naive methods.
class Solution {
public int maximumCoins(int[] balloons) {
int len = balloons.length + 2;
int[] paddedBalloons = new int[len];
System.arraycopy(balloons, 0, paddedBalloons, 1, len - 2);
paddedBalloons[0] = 1;
paddedBalloons[len - 1] = 1;
int[][] burstMemo = new int[len][len];
for (int start = len - 2; start >= 1; start--) {
for (int end = start; end <= len - 2; end++) {
for (int last = start; last <= end; last++) {
int coins = paddedBalloons[start - 1] * paddedBalloons[last] * paddedBalloons[end + 1];
int totalCoins = burstMemo[start][last - 1] + burstMemo[last + 1][end];
burstMemo[start][end] = Math.max(burstMemo[start][end], totalCoins + coins);
}
}
}
return burstMemo[1][len - 2];
}
}
The provided Java solution aims at solving a dynamic programming problem where the goal is to burst all balloons and maximize the number of coins collected. Each balloon, when burst, gives coins based on the product of its value and the values of the neighboring balloons still intact (left and right). The strategy involves using a padded balloons array where two dummy balloons (value of 1) are added at both ends of the array to simplify calculations.
The approach utilizes a 2D dynamic programming table, burstMemo
, where burstMemo[start][end]
represents the maximum coins that can be collected by bursting all balloons between indices start
to end
. The algorithm proceeds by iterating over all possible subarrays of paddedBalloons
, starting with the smallest and expanding outwards. For each subarray, it iteratively attempts to burst balloons one by one and calculates the maximum coins that can be obtained by considering coins collected from previously burst subarrays.
- Initialize
len
as the length of theballoons
array plus two. - Create
paddedBalloons
with additional boundary balloons set to 1. - Copy the original balloons into the
paddedBalloons
, positioned between the two boundary balloons. - Implement a nested loop where:
- Outer loops calculate values for progressively larger subarrays.
- Innermost loop iterates over each balloon as the last balloon to be burst, calculating coins collected from this decision.
- The maximum coins collected by bursting all balloons is stored in
burstMemo[1][len-2]
as it represents the subarray excluding the boundary balloons.
The solution effectively breaks the problem into smaller sub-problems, using previously solved outcomes to build up the answer for a larger set of balloons, making it a quintessential application of dynamic programming for optimal substructure problems.
class Solution:
def maximumCoins(self, nums: List[int]) -> int:
# Handling a special repetitive case
if len(nums) > 1 and len(set(nums)) == 1:
return (nums[0]**3) * (len(nums) - 2) + (nums[0]**2) + nums[0]
# Padding nums array with ones on both sides
padded_nums = [1] + nums + [1]
length = len(padded_nums)
# Dynamic Programming table initialization
coin_dp = [[0] * length for _ in range(length)]
# Burst simulations
for start in range(length - 2, 0, -1):
for end in range(start, length - 1):
for k in range(start, end + 1):
coins_gained = padded_nums[start - 1] * padded_nums[k] * padded_nums[end + 1]
dp_sum = coin_dp[start][k - 1] + coin_dp[k + 1][end]
coin_dp[start][end] = max(coin_dp[start][end], coins_gained + dp_sum)
return coin_dp[1][length - 2]
The provided solution in Python addresses the problem of finding the maximum coins one can collect by bursting balloons strategically. The balloons are represented by an array nums
, where each element represents the number of coins inside that balloon.
The approach utilizes dynamic programming. Here's a brief rundown of how the solution works:
- First, the solution checks if the input list
nums
contains more than one element and if all the elements are the same. If yes, a specific formula calculates the maximum coins directly. - The
nums
array is then padded with1
on both ends. This is a strategic move to simplify the boundary conditions during the dynamic programming iterations. - A 2D list,
coin_dp
, is initialized to store the maximum coins that can be collected from bursting all balloons between any two indices. - The solution then iterates over subarrays of
padded_nums
, considering each possible end point for the subarray. For each subarray, it iterates over each possible last balloon to be burst (denoted byk
), calculates the coins gained by bursting it as the last one, and updates thecoin_dp
matrix accordingly. - The maximum coins collected by bursting all balloons, barring the added ones, is found in
coin_dp[1][length - 2]
.
This strategically layered dynamic programming solution efficiently calculates the maximum possible coins by choosing the optimal balloons to burst at each step.
No comments yet.