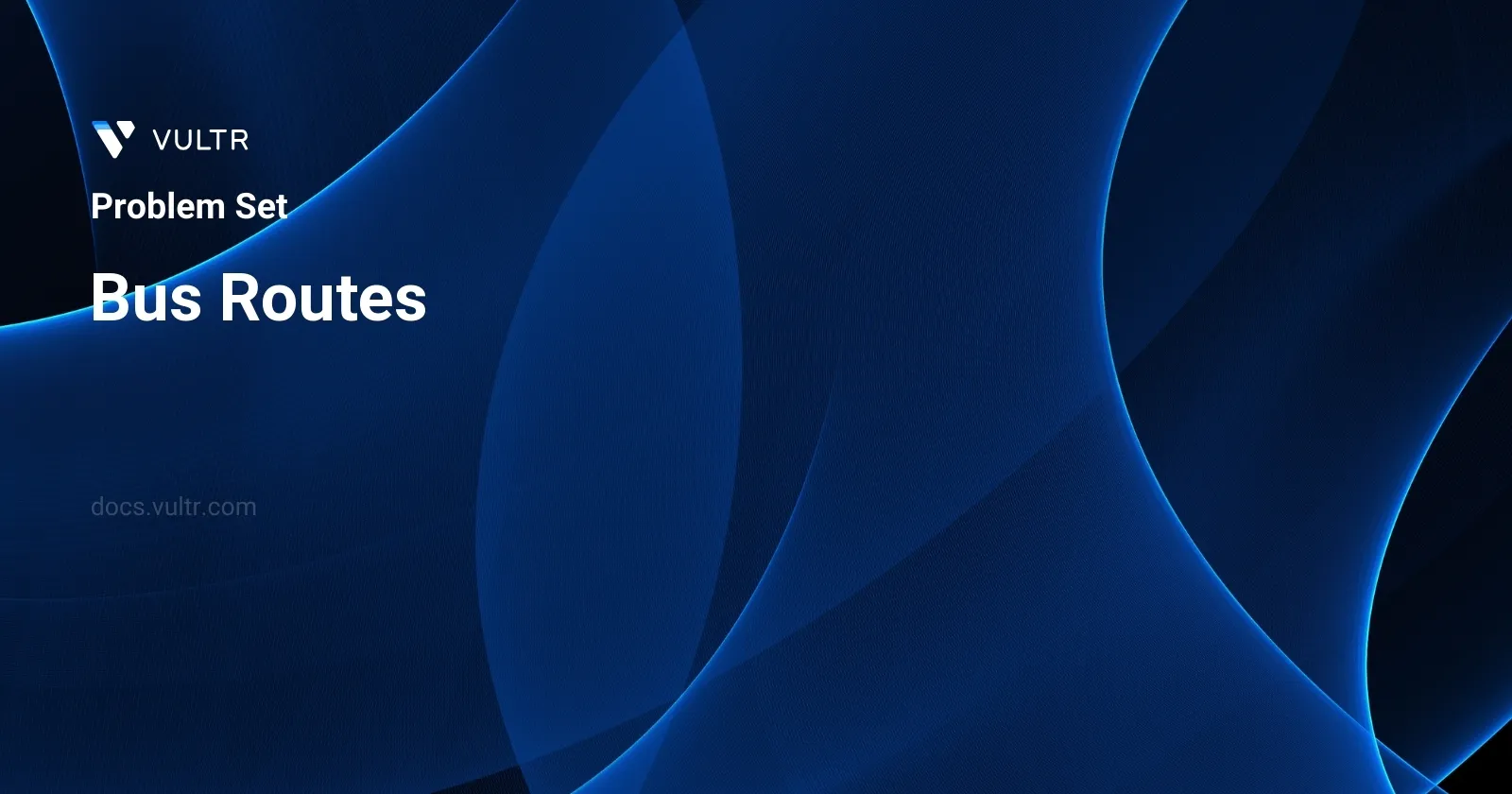
Problem Statement
In this task, you are working with a set of bus routes where each bus embarks on a specified sequence of bus stops continuously and indefinitely. Every route in the array routes
signifies a unique bus route where each bus circles through its stops over and over. Each route is represented by a list of bus stops. For instance, routes[0] = [1, 5, 7]
indicates that bus number 0 continuously travels through stops 1, 5, and 7 in that sequence.
Starting from a bus stop named source
, where no immediate bus is available (i.e., you're not aboard any bus from the start), your objective is to travel to a bus stop named target
using as few bus transfers as possible. The function should return the minimum number of bus rides required to reach the target. If it's impossible to reach the target from the source using the available routes, the function should return -1
.
Examples
Example 1
Input:
routes = [[1,2,7],[3,6,7]], source = 1, target = 6
Output:
2
Explanation:
The best strategy is take the first bus to the bus stop 7, then take the second bus to the bus stop 6.
Example 2
Input:
routes = [[7,12],[4,5,15],[6],[15,19],[9,12,13]], source = 15, target = 12
Output:
-1
Constraints
1 <= routes.length <= 500
.1 <= routes[i].length <= 105
- All the values of
routes[i]
are unique. sum(routes[i].length) <= 105
0 <= routes[i][j] < 106
0 <= source, target < 106
Approach and Intuition
To solve this problem, a graph traversal algorithm is generally an efficient method, possibly a breadth-first search (BFS) that explores the shortest path first:
Understanding the problem with the graph perspective: Consider each bus stop as a node and each bus route as an edge connecting the stops it services. Your task is to find the shortest path (minimum edges traversed) from the source node to the target node.
Building the graph: Map each bus stop to the list of buses that pass through it. This way, you can navigate from one stop to other stops on the same route efficiently and determine possible transfers.
Generating the BFS algorithm:
- Utilize a queue to explore each stop, starting from the
source
. - Track visited stops to prevent processing the same stop multiple times.
- For each stop, examine the bus routes that service it, and then explore all other stops serviced by those buses, marking them as visited and adding them to the queue.
- If you reach the target stop, return the number of buses (or edges) taken to get there.
- Utilize a queue to explore each stop, starting from the
Optimization considerations:
- As the number of bus stops and routes can be quite large, aim to keep the space and time complexity manageable by only storing necessary information like visited stops and bus-route connectivity.
Early termination: If the
source
is the same astarget
, the result is immediately 0 as no travel is needed.
this approach ensures that we utilize each bus route effectively and navigate through the least number of bus changes, leveraging the unique routes provided and large possible values within the constraints. It handles non-direct routes and cases where some stops might be isolated or far away in terms of bus transfers from the target.
Solutions
- C++
- Java
class Solution {
public:
vector<int> adjacencyList[501];
void buildGraph(vector<vector<int>>& routes) {
for (int i = 0; i < routes.size(); i++) {
for (int j = i + 1; j < routes.size(); j++) {
if (shareCommonStop(routes[i], routes[j])) {
adjacencyList[i].push_back(j);
adjacencyList[j].push_back(i);
}
}
}
}
bool shareCommonStop(vector<int>& route1, vector<int>& route2) {
int i = 0, j = 0;
while (i < route1.size() && j < route2.size()) {
if (route1[i] == route2[j]) {
return true;
}
route1[i] < route2[j] ? i++ : j++;
}
return false;
}
void enqueueRoutes(queue<int>& q, vector<vector<int>>& routes, int source) {
for (int i = 0; i < routes.size(); i++) {
if (containsStop(routes[i], source)) {
q.push(i);
}
}
}
bool containsStop(vector<int>& route, int stop) {
for (int j = 0; j < route.size(); j++) {
if (route[j] == stop) {
return true;
}
}
return false;
}
int numBusesToDestination(vector<vector<int>>& routes, int source, int target) {
if (source == target) {
return 0;
}
for (int i = 0; i < routes.size(); ++i) {
sort(routes[i].begin(), routes[i].end());
}
buildGraph(routes);
queue<int> q;
enqueueRoutes(q, routes, source);
vector<int> visited(routes.size(), 0);
int buses = 1;
while (!q.empty()) {
int sz = q.size();
while (sz--) {
int node = q.front();
q.pop();
if (containsStop(routes[node], target)) {
return buses;
}
for (int adj : adjacencyList[node]) {
if (!visited[adj]) {
visited[adj] = 1;
q.push(adj);
}
}
}
buses++;
}
return -1;
}
};
This solution implements an algorithm to determine the minimum number of buses required to reach a target destination from a given source using bus routes described as a vector of vectors.
- First, the solution makes use of an adjacency list for graph representation of the bus routes, where each index represents a bus (bus route) and the list at that index contains other buses (bus routes) that have a common stop.
- The
buildGraph
function constructs this graph. It checks for common stops between each pair of bus routes using theshareCommonStop
function, which uses a two-pointer technique to find common elements in two sorted lists. - The algorithm ensures all routes are sorted for efficient common stop detection.
- The
numBusesToDestination
function initiates the process if the source is the same as the target returning 0, as no bus needs to be taken. - It uses a breadth-first search (BFS) approach starting from the source. This is implemented by first enqueuing all buses that include the source stop into a queue.
- During the BFS, for each bus route dequeued, it checks if the target stop is in the current bus route. If found, it returns the current count of buses taken.
- Each neighboring bus route that is not yet visited is then added to the queue to explore further.
- If the queue exhausts and the target is not reached, the function returns -1, indicating the destination is not reachable.
The solution effectively uses graph theory coupled with BFS, ensuring it works efficiently even for a larger number of routes due to the adjacency list representation and systematic searching.
class GraphSolver {
List<List<Integer>> connectionGraph = new ArrayList();
void buildGraph(int[][] routes) {
for (int i = 0; i < routes.length; i++) {
for (int j = i + 1; j < routes.length; j++) {
if (nodesIntersect(routes[i], routes[j])) {
connectionGraph.get(i).add(j);
connectionGraph.get(j).add(i);
}
}
}
}
boolean nodesIntersect(int[] route1, int[] route2) {
int index1 = 0, index2 = 0;
while (index1 < route1.length && index2 < route2.length) {
if (route1[index1] == route2[index2]) {
return true;
}
if (route1[index1] < route2[index2]) {
index1++;
} else {
index2++;
}
}
return false;
}
void enqueueInitialNodes(Queue<Integer> queue, int[][] routes, int start) {
for (int i = 0; i < routes.length; i++) {
if (containsStop(routes[i], start)) {
queue.add(i);
}
}
}
boolean containsStop(int[] route, int stop) {
for (int stopIndex = 0; stopIndex < route.length; stopIndex++) {
if (route[stopIndex] == stop) {
return true;
}
}
return false;
}
public int findBusRoutes(int[][] routes, int source, int target) {
if (source == target) {
return 0;
}
for (int i = 0; i < routes.length; ++i) {
Arrays.sort(routes[i]);
connectionGraph.add(new ArrayList());
}
buildGraph(routes);
Queue<Integer> queue = new LinkedList<>();
enqueueInitialNodes(queue, routes, source);
Set<Integer> visited = new HashSet<Integer>(routes.length);
int edgesCrossed = 1;
while (!queue.isEmpty()) {
int levelSize = queue.size();
while (levelSize-- > 0) {
int currentNode = queue.remove();
if (containsStop(routes[currentNode], target)) {
return edgesCrossed;
}
for (int adjacent : connectionGraph.get(currentNode)) {
if (!visited.contains(adjacent)) {
visited.add(adjacent);
queue.add(adjacent);
}
}
}
edgesCrossed++;
}
return -1;
}
}
The solution presented implements an approach to solve the "Bus Routes" problem using graph-based techniques with Java. In this problem, buses are represented as nodes, and the existence of a common bus stop between two different bus routes creates an edge between those nodes. The primary objective is to find the minimum number of transitions from one bus to another required to travel from a source stop to a destination stop.
The core classes and methods include:
GraphSolver
- A class where the main logic for handling the graph and the problem resides.buildGraph(int[][] routes)
- Constructs the graph by creating edges between nodes (routes) that share at least one bus stop.nodesIntersect(int[] route1, int[] route2)
- Helper method to determine if two routes intersect based on their stops.enqueueInitialNodes(Queue<Integer> queue, int[][] routes, int start)
- Identifies and enqueues all routes that include the starting bus stop.containsStop(int[] route, int stop)
- Checks whether a specific bus stop id is present in a route.findBusRoutes(int[][] routes, int source, int target)
- Implements a Breadth-First Search (BFS) to explore the shortest path from the start stop to the target stop. The method also handles cases where the source and target are the same.
Key aspects in this method include:
- Initialization of a
connectionGraph
where each node is a list representing bus routes that can be reached from that starting node. - Each route is sorted to optimize the search process in intersect check.
- Use of a queue to implement the BFS where the routes containing the initial bus stop are enqueued as starting points.
- A set
visited
tracks which nodes have been visited in the BFS to prevent redundant processing. - The BFS explores levels of routes, trying to reach the target stop with the fewest bus transitions, incrementing the count
edgesCrossed
as it spans out.
Edge cases considered are:
- Direct reach from source to target without any transitions.
- No possible route found, in which case the function returns -1.
This solution efficiently models the problem using graph theory concepts combined with BFS for minimum path traversal, which is suitable given the nature of the problem with potential large numbers of bus stops and routes.
No comments yet.