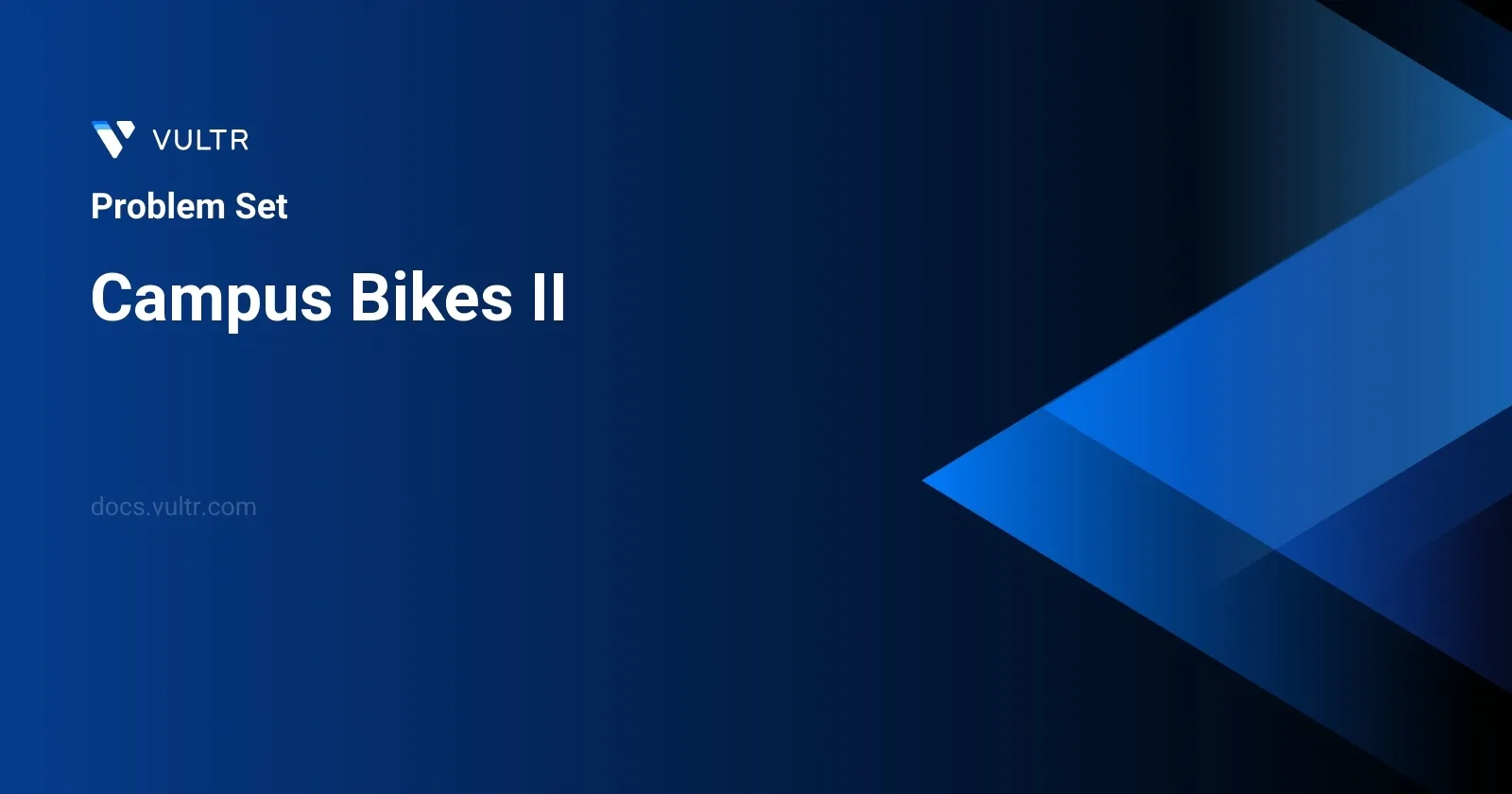
Problem Statement
In a scenario where a campus is depicted as a 2D grid, there exist n
workers and m
bikes, with the relationship n <= m
. Both the workers and bikes are represented as two-dimensional coordinates on the grid. The main objective is to allocate exactly one unique bike to each worker. This pairing must be done such that the collective sum of the Manhattan distances between each worker and their assigned bike is as minimized as possible. The Manhattan distance between two points, say p1
and p2
, is calculated using the formula: Manhattan(p1, p2) = |p1.x - p2.x| + |p1.y - p2.y|
. The task is to determine and return the minimal possible sum of these distances for the optimal assignments.
Examples
Example 1
Input:
workers = [[0,0],[2,1]], bikes = [[1,2],[3,3]]
Output:
6
Explanation:
We assign bike 0 to worker 0, bike 1 to worker 1. The Manhattan distance of both assignments is 3, so the output is 6.
Example 2
Input:
workers = [[0,0],[1,1],[2,0]], bikes = [[1,0],[2,2],[2,1]]
Output:
4
Explanation: We first assign bike 0 to worker 0, then assign bike 1 to worker 1 or worker 2, bike 2 to worker 2 or worker 1. Both assignments lead to sum of the Manhattan distances as 4.
Example 3
Input:
workers = [[0,0],[1,0],[2,0],[3,0],[4,0]], bikes = [[0,999],[1,999],[2,999],[3,999],[4,999]]
Output:
4995
Constraints
n == workers.length
m == bikes.length
1 <= n <= m <= 10
workers[i].length == 2
bikes[i].length == 2
0 <= workers[i][0], workers[i][1], bikes[i][0], bikes[i][1] < 1000
- All the workers and the bikes locations are unique.
Approach and Intuition
The problem at hand requires an optimal assignment of bikes to workers such that the total Manhattan distance is minimized. Here's an intuitive approach based on the examples and constraints provided:
Understanding Distance Calculation:
- The Manhattan distance is chosen because it effectively measures the grid-based path between any two points in a linear city-like block layout.
Example Walk-throughs:
- For Example 1, with two workers and two bikes:
- Calculate distance from each worker to each bike.
- The optimal assignment by manual inspection or algorithmic design leads to each worker paired such that total distance is minimized to 6.
- For Example 2, the scenario of three workers and three bikes again shows:
- Calculation of possible distances and then deciding on distribution optimizing the sum of distances. A few assignment trials provide a minimal sum of 4.
- Example 3 demonstrates distance minimization with aligned workers and far distant bikes, yielding a straightforward but large distance sum of 4995.
- For Example 1, with two workers and two bikes:
Constraint Utilization:
- Given that the number of workers and bikes can be at most 10, an exhaustive approach or an optimized algorithm using backtracking or the Hungarian algorithm (also known as the Munkres or Kuhn-Munkres algorithm) could be feasible. These methods work by ensuring each potential assignment's feasibility and optimality under the given constraints.
- Unique positions of workers and bikes simplify the calculation as there won't be duplicate positions which could potentially complicate the pairing mechanism, ensuring that each worker-bike pair is distinctly calculated without overlaps.
This task, though computationally intensive for large numbers, remains computationally feasible for the upper constraints specified, by leveraging combinatorial optimization techniques particularly suited to such assignment problems.
Solutions
- C++
- Java
class Solution {
public:
int calcDistance(vector<int>& coordsA, vector<int>& coordsB) {
return abs(coordsA[0] - coordsB[0]) + abs(coordsA[1] - coordsB[1]);
}
int bitCount(int bitmask) {
int total = 0;
while (bitmask != 0) {
bitmask &= (bitmask - 1);
total++;
}
return total;
}
int assignBikes(vector<vector<int>>& workers, vector<vector<int>>& bikes) {
int totalBikes = bikes.size();
int totalWorkers = workers.size();
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> minHeap;
unordered_set<int> processed;
minHeap.push({0, 0});
while (!minHeap.empty()) {
int distSum = minHeap.top().first;
int mask = minHeap.top().second;
minHeap.pop();
if (processed.find(mask) != processed.end())
continue;
processed.insert(mask);
int currentWorker = bitCount(mask);
if (currentWorker == totalWorkers)
return distSum;
for (int bikeIdx = 0; bikeIdx < totalBikes; bikeIdx++) {
if (!(mask & (1 << bikeIdx))) {
int newDistSum = distSum +
calcDistance(workers[currentWorker], bikes[bikeIdx]);
int newMask = mask | (1 << bikeIdx);
minHeap.push({newDistSum, newMask});
}
}
}
return -1;
}
};
The solution implements an approach to assign bikes to workers such that the sum of the Manhattan distances between each worker and their assigned bike is minimized. This problem is tackled using a priority queue to facilitate the selection of minimum distances, and a bitmask to represent the assignment state of bikes, optimizing the approach further with an unchecked state tracking via a set.
The
calcDistance
function calculates the Manhattan distance between two points. It takes two vectors,coordsA
andcoordsB
, representing the coordinates of a worker and a bike, respectively.The
bitCount
function counts the number of set bits in an integer. This represents the number of bikes that have been assigned to workers.assignBikes
is the core function where:- It initializes a priority queue which is used to always extend the pair of current total distance and the bitmask representing bike assignments that has the smallest distance.
- A set named
processed
is maintained to avoid reprocessing the same bitmask. - Continuously, it pops elements from the priority queue, checks and processes them. If the bitmask indicates all workers have been assigned bikes, the current distance sum is returned as the result.
- For each unassigned bike, the function calculates the new distance if that bike were assigned to the current worker and then pushes this new distance and updated bitmask back into the priority queue.
This solution leverages bit manipulation and a min-heap to effectively and efficiently solve the problem by considering each possible allocation of bikes to workers, ensuring that each combination is processed in an order that first considers the lowest cumulative distance.
class Solution {
private int calculateDistance(int[] worker, int[] bike) {
return Math.abs(worker[0] - bike[0]) + Math.abs(worker[1] - bike[1]);
}
private int bitCount(int number) {
int count = 0;
while (number != 0) {
number &= (number - 1);
count++;
}
return count;
}
public int assignBikes(int[][] workers, int[][] bikes) {
int totalBikes = bikes.length, totalWorkers = workers.length;
PriorityQueue<int[]> priorityQueue = new PriorityQueue<>((a, b) -> a[0] - b[0]);
Set<Integer> visitedMasks = new HashSet<>();
priorityQueue.add(new int[]{0, 0});
while (!priorityQueue.isEmpty()) {
int[] top = priorityQueue.poll();
int distSum = top[0], mask = top[1];
if (visitedMasks.contains(mask))
continue;
visitedMasks.add(mask);
int workerID = bitCount(mask);
if (workerID == totalWorkers) {
return distSum;
}
for (int bikeID = 0; bikeID < totalBikes; bikeID++) {
if ((mask & (1 << bikeID)) == 0) {
int newDistSum = distSum +
calculateDistance(workers[workerID], bikes[bikeID]);
int newMask = mask | (1 << bikeID);
priorityQueue.add(new int[]{newDistSum, newMask});
}
}
}
return -1;
}
}
This program in Java solves the problem of assigning bikes to workers in such a way that the total distance traveled by all workers is minimized. It efficiently uses a bit manipulation technique to represent combinations of bike assignments to workers, combined with a priority queue to ensure the smallest distance sum is prioritized for expansion. Here's how the solution is structured:
Helper Methods:
calculateDistance
: Computes the Manhattan distance between a worker and a bike.bitCount
: Counts the number of 1s in the binary representation of the number, representing how many bikes have been assigned.
Core Method -
assignBikes
:- Initializes a
PriorityQueue
to store and retrieve states by minimum distance sum using a comparator. - Uses a
Set
to avoid revisiting already checked combinations of bike assignments, exploiting properties of bit masks to represent these combinations. - Continuously expands the state with the smallest distance sum by trying to assign unassigned bikes to the next worker. Each state is a pair of the current distance sum and the bitmask representing which bikes have been assigned.
- Initializes a
Efficiency Considerations:
- By stopping the expansion as soon as all workers have bikes assigned (
workerID == totalWorkers
), the algorithm ensures an optimal solution is found as soon as possible. - Using bit manipulation (via masks) provides a compact and efficient way to handle the combinations of assignments.
- By stopping the expansion as soon as all workers have bikes assigned (
This approach ensures a focused, step-by-step examination of possible assignments, using both bit-level operations for efficiency and a priority-based exploration to ensure the minimum distance configuration is found swiftly.
No comments yet.