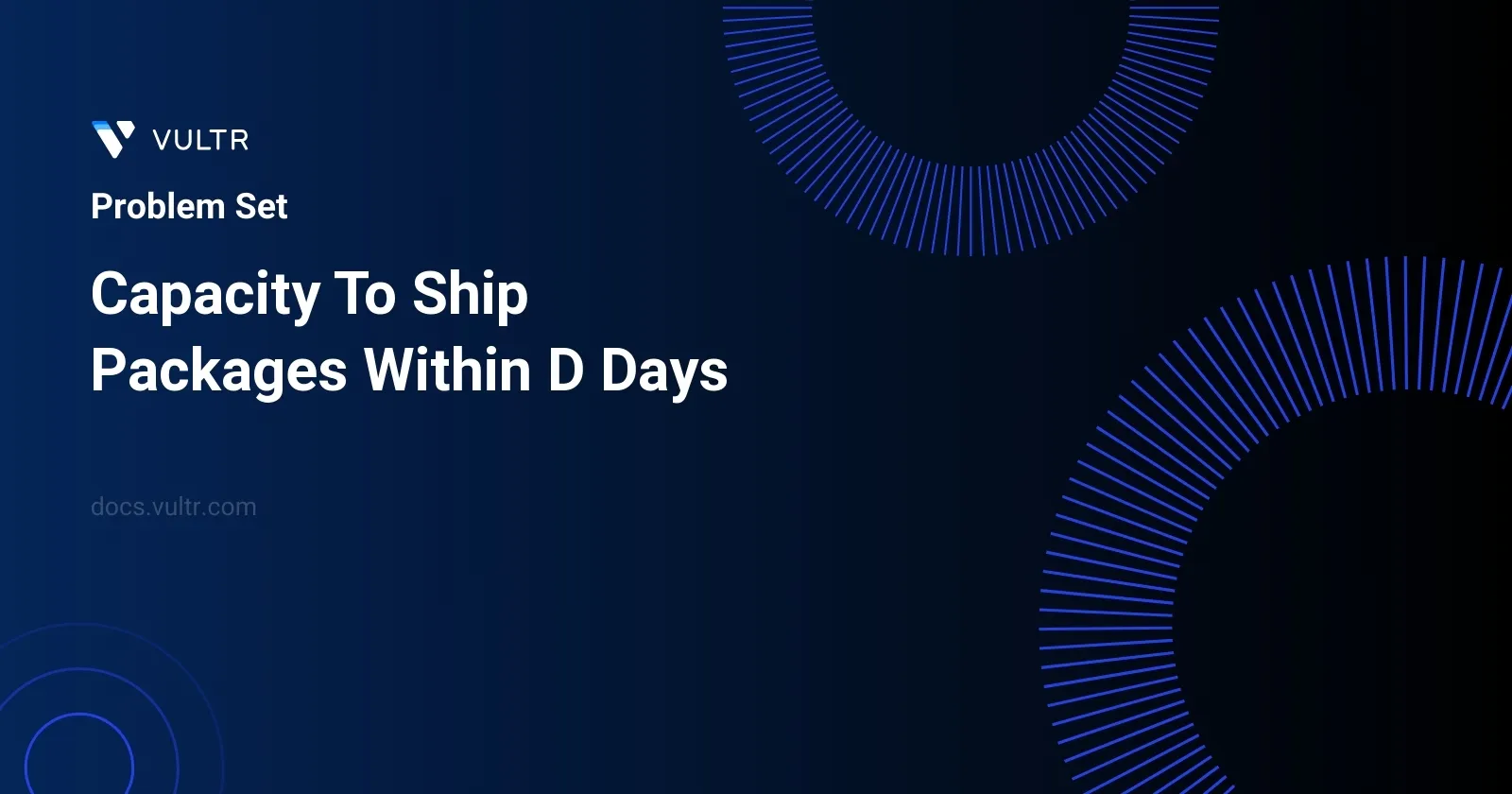
Problem Statement
The objective is to determine the minimum capacity of a ship required to transport all packages placed sequentially on a conveyor belt to another port within a specified number of days. Each package has a predefined weight. The ship can load multiple packages daily but cannot exceed a certain weight capacity. It is mandated that the order of the packages on the conveyor belt is preserved when loading them onto the ship. Therefore, the challenge involves calculating the least weight capacity that would enable the complete transfer of packages within the given timeframe, taking into account the constraints imposed by the package weights and the number of days available for shipment.
Examples
Example 1
Input:
weights = [1,2,3,4,5,6,7,8,9,10], days = 5
Output:
15
Explanation:
A ship capacity of 15 is the minimum to ship all the packages in 5 days like this: 1st day: 1, 2, 3, 4, 5 2nd day: 6, 7 3rd day: 8 4th day: 9 5th day: 10 Note that the cargo must be shipped in the order given, so using a ship of capacity 14 and splitting the packages into parts like (2, 3, 4, 5), (1, 6, 7), (8), (9), (10) is not allowed.
Example 2
Input:
weights = [3,2,2,4,1,4], days = 3
Output:
6
Explanation:
A ship capacity of 6 is the minimum to ship all the packages in 3 days like this: 1st day: 3, 2 2nd day: 2, 4 3rd day: 1, 4
Example 3
Input:
weights = [1,2,3,1,1], days = 4
Output:
3
Explanation:
1st day: 1 2nd day: 2 3rd day: 3 4th day: 1, 1
Constraints
1 <= days <= weights.length <= 5 * 104
1 <= weights[i] <= 500
Approach and Intuition
The problem outlined involves determining the minimal ship capacity to distribute packages over a number of days without violating the sequential order of the weights
array. Given the constraints and the necessity to maintain the order of shipment, a greedy or straightforward approach is inefficient. Instead, we turn towards a binary search combined with a checking mechanism that efficiently validates the potential capacity.
Here's how the approach and solution can be conceptualized:
- Identify the basic boundaries for the binary search:
- Lower Bound: The maximum value in
weights
. At minimum, the ship's capacity should be able to carry the heaviest single package. - Upper Bound: The sum of all items in
weights
. This represents the scenario where all packages are shipped in one day.
- Lower Bound: The maximum value in
- Utilize binary search between these bounds:
- Calculate the middle point (
mid
) which represents a potential ship capacity. - Check if using this capacity can distribute all packages within the given
days
:- Start accumulating weights day-by-day.
- If adding another package exceeds the
mid
(current capacity under test), start a new day and continue accumulating from the next package. - Keep track of the number of days needed for this capacity.
- Based on the number of days calculated:
- If the days required with
mid
capacity is less than or equal todays
, it implies that it might be possible to reduce the capacity. Hence, adjust the upper bound of the binary search tomid - 1
and repeat. - If the days exceed, it signifies that the
mid
capacity is too small, and therefore, adjust the lower bound tomid + 1
.
- If the days required with
- Calculate the middle point (
- Continue the binary search process until the lower bound exceeds the upper bound. The optimal solution (minimal ship capacity) is found at the lowest
mid
for which the shipment schedule fits within thedays
provided.
By employing this precise and logical strategy of binary search and day-by-day capacity checking, we can efficiently find the minimal ship capacity needed to meet the shipment schedule requirement.
Solutions
- C++
- Java
class Solution {
public:
bool canShip(vector<int>& weights, int capacity, int maxDays) {
int requiredDays = 1, totalWeight = 0;
for (int w : weights) {
totalWeight += w;
if (totalWeight > capacity) {
requiredDays++;
totalWeight = w;
}
}
return requiredDays <= maxDays;
}
int shipWithinDays(vector<int>& weights, int days) {
int minimumLoad = 0, maximumWeight = 0;
for (int weight : weights) {
minimumLoad += weight;
if(weight > maximumWeight) maximumWeight = weight;
}
int low = maximumWeight, high = minimumLoad;
while (low < high) {
int mid = low + (high - low) / 2;
if (canShip(weights, mid, days)) {
high = mid;
} else {
low = mid + 1;
}
}
return low;
}
};
The provided C++ solution deals with determining the minimum capacity required to ship packages within a specified number of days. Here's an explanation of how the solution implements this functionality:
The solution uses the binary search technique on the range of possible ship capacities, which efficiently narrows down the minimum possible capacity required.
The function
canShip
checks whether a given capacity can be used to ship all packages within the allowed days. It iterates over package weights, grouping them together until their total exceeds the current capacity, in which case a new shipment day is started.In the
shipWithinDays
function:Calculate the minimal possible load that must be able to be shipped in a day, which is the weight of the heaviest package among
weights
, and accumulate the total of all weights as the maximal boundary (minimumLoad
).Perform a binary search between
maximumWeight
andminimumLoad
. For each middle value (mid
), usecanShip
to test if it's feasible to ship with this capacity in the specified number of days. Adjust the low or high boundary based on the feasibility.The loop continues adjusting low and high limits until an optimal minimum capacity (
low
) is identified that satisfies the conditions.
This efficient approach optimizes the handling of large data inputs and ensures that the minimum shipping capacity is found with precision, adhering to the constraints of the given days.
class Solution {
Boolean canShip(int[] packages, int capacity, int maxDays) {
int requiredDays = 1, load = 0;
for (int packageWeight : packages) {
load += packageWeight;
if (load > capacity) {
requiredDays++;
load = packageWeight;
}
}
return requiredDays <= maxDays;
}
public int shipWithinDays(int[] weights, int days) {
int sumWeights = 0, maxWeight = 0;
for (int weight : weights) {
sumWeights += weight;
maxWeight = Math.max(maxWeight, weight);
}
int left = maxWeight, right = sumWeights;
while (left < right) {
int mid = (left + right) / 2;
if (canShip(weights, mid, days)) {
right = mid;
} else {
left = mid + 1;
}
}
return left;
}
}
The problem "Capacity To Ship Packages Within D Days" involves determining the minimum capacity of a ship so that all packages can be shipped within a given number of days. A binary search approach is applied to solve the problem efficiently in Java.
The solution class contains two methods:
- canShip: Checks if it's possible to ship all packages within the given days and capacity.
- shipWithinDays: Determines the minimum capacity using a binary search strategy.
Here's a breakdown of the implementation:
canShip method:
- Initialize
requiredDays
to 1 andload
to 0 to track the total weight loaded on the ship for the current day. - Iterate over the package weights, adding them to the current load.
- When the accumulated load exceeds the capacity, increment the required days and reset the load to the current package's weight.
- The method returns true if the total required days are within the given limit.
- Initialize
shipWithinDays method:
- Calculate the sum of all weights and determine the maximum single weight.
- Set the binary search range between this maximum weight and the sum of all weights.
- Use a binary search loop to find the minimum possible shipping capacity:
- Calculate the midpoint of the range as a potential shipping capacity.
- If
canShip
returns true for this midpoint, narrow the search to the lower half by adjusting the right end of the range. - Otherwise, adjust the left end of the range to the higher half.
- Return the left boundary of the range as this represents the minimum capacity needed.
This setup ensures that each check for feasibility (canShip
) is optimized and minimizes the capacity required to meet the specified days constraint. The utilization of binary search provides a significant efficiency boost, especially useful when dealing with large data sets or tight performance requirements.
No comments yet.