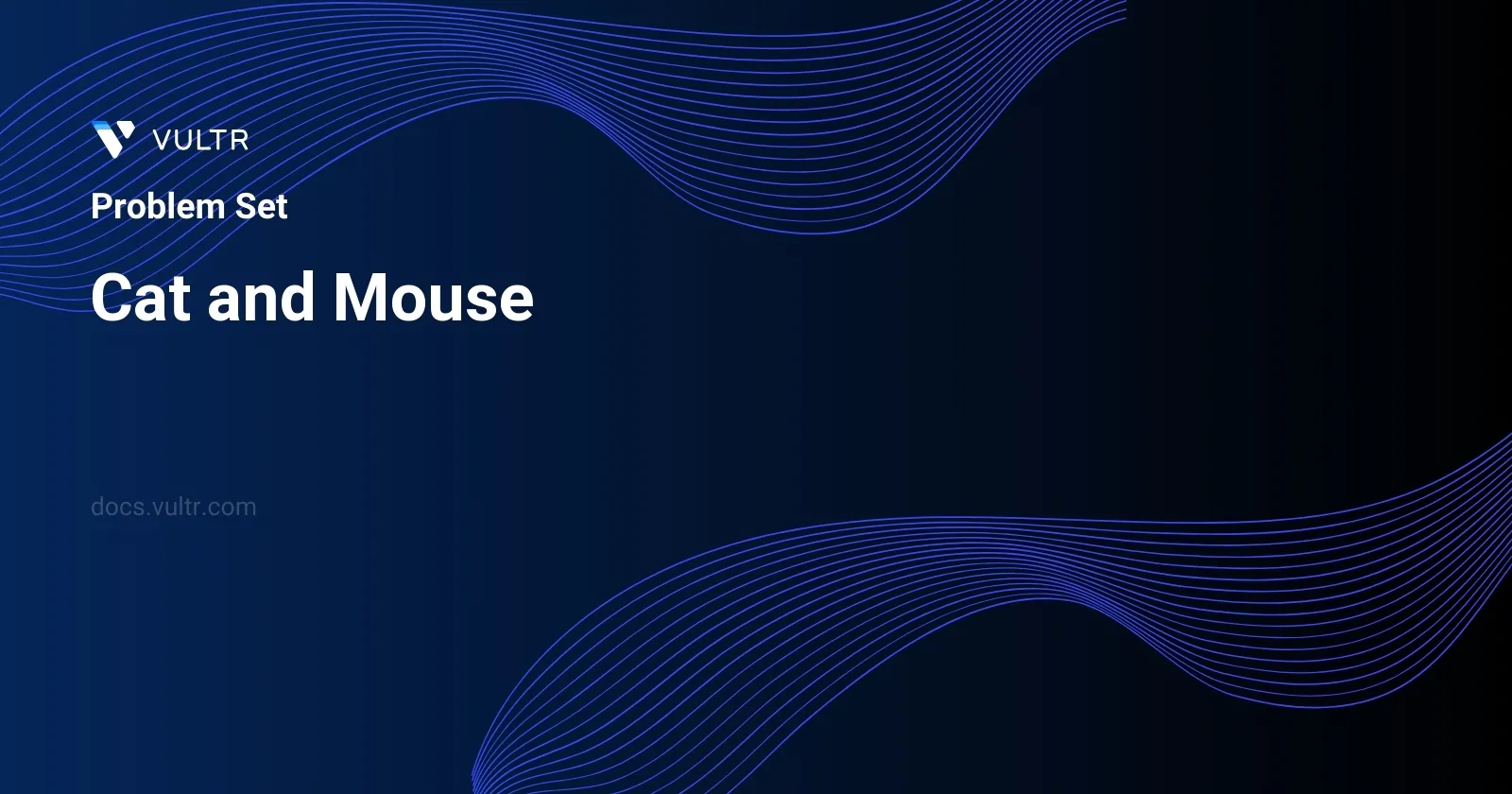
Problem Statement
The game is structured around a simple, yet fascinating concept involving two players — a Mouse and a Cat, maneuvering through an undirected graph. The layout of the game involves player positions and movement rules dictated by the graph's structure. Each node in the graph represents a position that the Mouse or the Cat can occupy. A unique dynamic is introduced with the 'Hole' at node 0
, which is pivotal as a potential escape or winning point for the Mouse. The Cat, however, is restricted from entering this node.
Players alternate turns starting with the Mouse at node 1
and the Cat at node 2
. On each player's turn, they must move from their current node to a connected node. The game can conclude in three possible ways:
- The Cat wins if it lands on the same node as the Mouse.
- The Mouse wins if it reaches the Hole (node
0
). - The game ends in a draw if any position repeats with the same player’s turn.
Given the graph's configuration, and assuming both players are using optimal strategies, the challenge is to predict the outcome. Will the game favor the Mouse, the Cat, or end in a stalemate? The solution should effectively interpret the graph to deliver one of three results: 1
for a Mouse win, 2
for a Cat win, or 0
for a draw.
Examples
Example 1
Input:
graph = [[2,5],[3],[0,4,5],[1,4,5],[2,3],[0,2,3]]
Output:
0
Example 2
Input:
graph = [[1,3],[0],[3],[0,2]]
Output:
1
Constraints
3 <= graph.length <= 50
1 <= graph[i].length < graph.length
0 <= graph[i][j] < graph.length
graph[i][j] != i
graph[i]
is unique.- The mouse and the cat can always move.
Approach and Intuition
To tackle this problem, it’s essential to understand the implications of each move within the context of the graph. The objective includes not just moving but also strategically positioning oneself to either escape (by the Mouse) or trap (by the Cat). Here’s a step-by-step approach:
Game Representation: First, represent the game using the graph where nodes depict positions connected by possible moves. Recognize the role of specific nodes like the Hole (node
0
), the starting position of the Mouse (node1
), and the Cat (node2
).Movement Rules: Understand that each move from a node must adhere to the graph’s defined pathways and restrictions (e.g., Cat cannot move to node
0
).Winning Conditions: Analyze paths and predetermine sequences that quickly lead to the Mouse reaching the hole or the Cat capturing the Mouse.
Simulation of Optimal Gameplay: Use techniques such as depth-first search (DFS) or breadth-first search (BFS) to simulate each possible move sequence. Given that nodes have unique adjacency lists and graph sizes are moderate, such algorithms are feasible.
Handling Cycles and Repeated States: Implement mechanisms to detect and handle cycles or repeated states (draw conditions). This can involve maintaining a history of game states to detect repetitions, thereby declaring a draw if a state recurs with the same player to move.
By simulating every possible outcome from the given starting conditions, leveraging the structure of the graph, and considering optimal strategies for both players, one can predict the end result accurately. Analyzing the paths and strategic points in the graph where the game can pivot from one outcome to another is crucial. This strategic examination combined with algorithmic simulations forms the backbone of solving this intriguing and challenging problem.
Solutions
- Java
class GameStrategy {
public int determineWinner(int[][] connections) {
int nodeCount = connections.length;
final int TIE = 0, MOUSE_WIN = 1, CAT_WIN = 2;
int[][][] stateColor = new int[50][50][3];
int[][][] connectedDegrees = new int[50][50][3];
for (int mouse = 0; mouse < nodeCount; ++mouse)
for (int cat = 0; cat < nodeCount; ++cat) {
connectedDegrees[mouse][cat][1] = connections[mouse].length;
connectedDegrees[mouse][cat][2] = connections[cat].length;
for (int next: connections[cat]) if (next == 0) {
connectedDegrees[mouse][cat][2]--;
break;
}
}
Queue<int[]> processingQueue = new LinkedList();
for (int k = 0; k < nodeCount; ++k)
for (int turn = 1; turn <= 2; ++turn) {
stateColor[0][k][turn] = MOUSE_WIN;
processingQueue.add(new int[]{0, k, turn, MOUSE_WIN});
if (k > 0) {
stateColor[k][k][turn] = CAT_WIN;
processingQueue.add(new int[]{k, k, turn, CAT_WIN});
}
}
while (!processingQueue.isEmpty()) {
int[] current = processingQueue.remove();
int m = current[0], c = current[1], turn = current[2], winState = current[3];
for (int[] progenitor: findParents(connections, m, c, turn)) {
int pm = progenitor[0], pc = progenitor[1], pt = progenitor[2];
if (stateColor[pm][pc][pt] == TIE) {
if (pt == winState) {
stateColor[pm][pc][pt] = winState;
processingQueue.add(new int[]{pm, pc, pt, winState});
} else {
connectedDegrees[pm][pc][pt]--;
if (connectedDegrees[pm][pc][pt] == 0) {
stateColor[pm][pc][pt] = 3 - pt;
processingQueue.add(new int[]{pm, pc, pt, 3 - pt});
}
}
}
}
}
return stateColor[1][2][1];
}
public List<int[]> findParents(int[][] graph, int m, int c, int turn) {
List<int[]> predecessors = new ArrayList();
if (turn == 2) {
for (int prevMouse: graph[m])
predecessors.add(new int[]{prevMouse, c, 3-turn});
} else {
for (int prevCat: graph[c]) if (prevCat > 0)
predecessors.add(new int[]{m, prevCat, 3-turn});
}
return predecessors;
}
}
This Java program implements a game strategy to determine a winner in a simulation where a Cat and a Mouse move through connected nodes in a game graph. The determineWinner
method within the GameStrategy
class uses multi-dimensional arrays to track game states and moves, applying a strategic algorithm to predict the outcome based on move possibilities and state changes.
Here's how the solution approaches the problem:
- Defines constants for tie, mouse win, and cat win states to clarify outcomes.
- Initializes 'stateColor' to manage the current state (win or tie) for different positions of cat and mouse, and 'connectedDegrees' to count unprocessed moves.
- Sets base state conditions: the mouse wins if located at the start node without the cat, and the cat wins if both are at the same node.
- Starts from base conditions and processes all possible game states and moves through a queue to propagate outcomes:
- Extracts each condition and evaluates potential moves or 'parents'.
- Updates states and move possibilities based on the results of possible moves.
- Applies a breadth-first search strategy using a queue which manages the state evaluation in a structured manner.
- The final outcome for starting positions of mouse and cat is extracted from the array.
Key elements used include:
- Multi-dimensional arrays for dynamic tracking of game states.
- Queue to process states in order and deal with dependencies among moves.
- Breadth-first search strategy to explore all possible outcomes from initial to final states.
This approach ensures that all possible game configurations are considered and the result accurately reflects the strategic outcome of the game based on predefined moves and rules. The method returns the winner for a specific starting condition of the cat at node 2 and the mouse at node 1.
No comments yet.