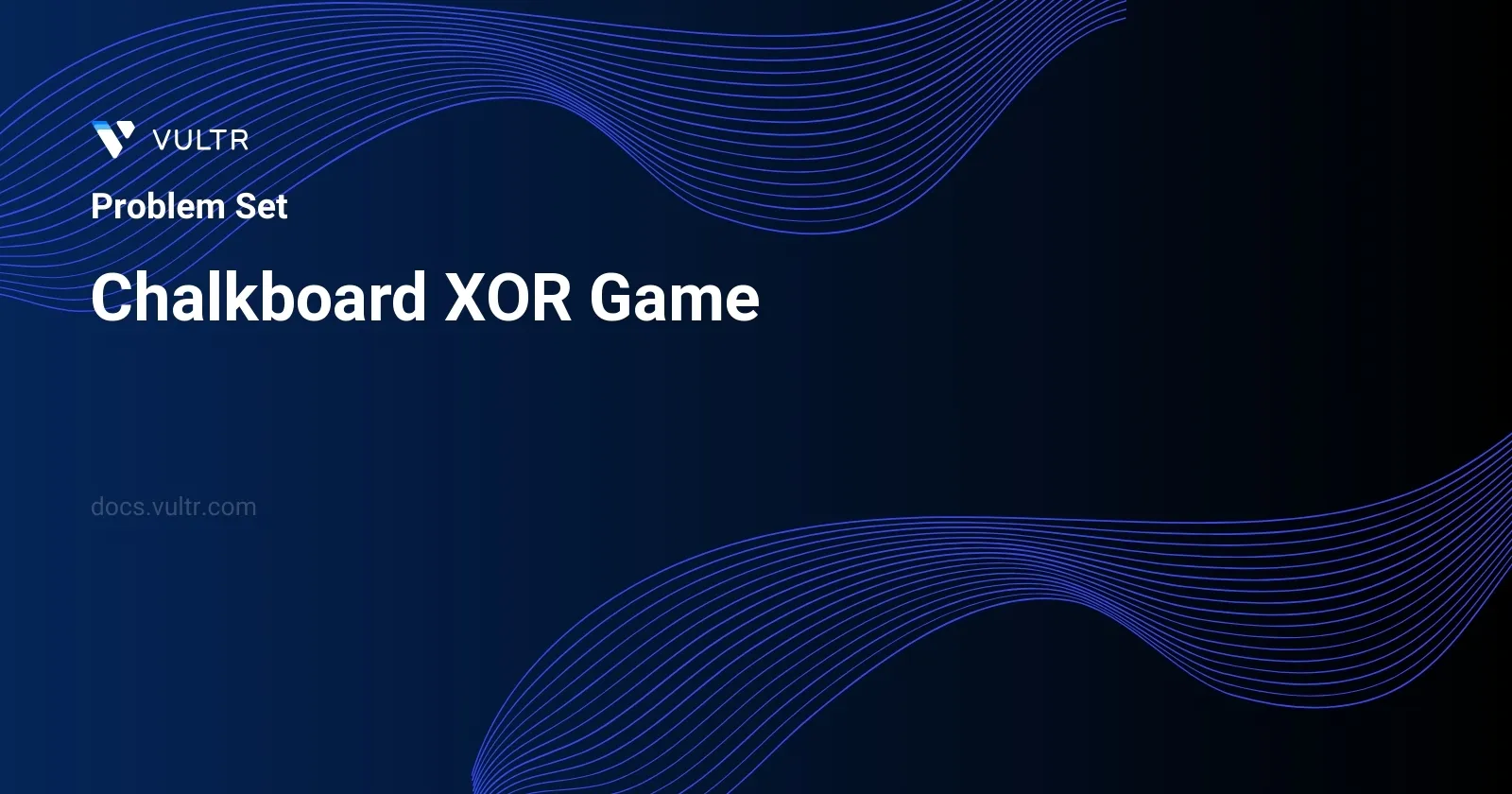
Problem Statement
In the given problem, an array of integers nums
represents the set of numbers written on a chalkboard. Alice and Bob are in a competition where they alternately erase one number from the chalkboard, starting with Alice. The challenge lies in the effect of the bitwise XOR operation applied to the numbers left on the board after each move. A player loses if their move results in the XOR of all remaining numbers to be zero. Additionally, a player immediately wins if the XOR result of all the chalkboard numbers at the start of their turn is zero. The objective is to determine if Alice can win the game, given that both players are using their best strategies throughout the game.
Examples
Example 1
Input:
nums = [1,1,2]
Output:
false
Explanation:
Alice has two choices: erase 1 or erase 2. If she erases 1, the nums array becomes [1, 2]. The bitwise XOR of all the elements of the chalkboard is 1 XOR 2 = 3. Now Bob can remove any element he wants, because Alice will be the one to erase the last element and she will lose. If Alice erases 2 first, now nums become [1, 1]. The bitwise XOR of all the elements of the chalkboard is 1 XOR 1 = 0. Alice will lose.
Example 2
Input:
nums = [0,1]
Output:
true
Example 3
Input:
nums = [1,2,3]
Output:
true
Constraints
1 <= nums.length <= 1000
0 <= nums[i] < 216
Approach and Intuition
Understanding Bitwise XOR for Gameplay:
The XOR (exclusive OR) operation is a fundamental concept utilized here:
- XOR of a number with itself is zero.
- XOR of a number with zero is the number itself.
Thus, the game's outcome can be dictated by the initial condition of the chalkboard numbers.
Strategy Analysis Using Examples:
Example 1 Analysis (
nums = [1,1,2]
):- Alice’s options are:
- If she removes a
1
, the remaining numbers are[1, 2]
. Their XOR equals 3, which isn't zero. However, Alice eventually loses after more moves, since removing any number would leave a situation for Bob to respond optimally and force Alice into a losing position. - If she removes the
2
, the remaining numbers are[1, 1]
. Their XOR is 0, thus Alice immediately loses.
- If she removes a
- Conclusion: Regardless of the approach, Alice ends up losing.
- Alice’s options are:
Example 2 Analysis (
nums = [0, 1]
):- Initially, the XOR of
[0, 1]
is1
, which isn’t zero. Alice can remove0
, leading to a XOR remaining of[1]
which is 1. On Bob's turn, any move leads to zero, causing Bob to lose. - Conclusion: With optimal play, Alice wins.
- Initially, the XOR of
Example 3 Analysis (
nums = [1, 2, 3]
):- Initial XOR is
0
when computed for all integers, meaning if Alice beginnings with such a scenario, she wins immediately with no move required. - In any nuanced plays, strategic removals keep the scenario mostly favorable to the starter, which in every game case, is Alice.
- Initial XOR is
Strategy for Winning:
- Alice will win if the XOR of all numbers in
nums
at the start of her turn isn’t zero. She then should play strategically to either maintain non-zero XOR conditions or manipulate the board such that Bob faces a zero-XOR scenario on his turn. - If the game starts with a XOR sum of zero naturally, Alice wins automatically without making any move.
Considering these examples and the application of bitwise operations, the game heavily relies on the cumulative XOR of the starting array and the ongoing strategic interaction between Alice and Bob. Understanding the XOR conditions after each potential move is key to determining the optimal strategy and predicting the game's outcome, fundamentally benefiting Alice if she begins optimally and adapts as the board evolves.
Solutions
- Java
class Solution {
public boolean playXORGame(int[] elements) {
int result = 0;
for (int element : elements) result ^= element;
return result == 0 || elements.length % 2 == 0;
}
}
The given Java solution addresses the XOR game problem, where the aim is to determine the winning strategy based on the initial array of integers, elements
.
In the playXORGame
method:
- A variable
result
initializes at 0. It's used to store the accumulative XOR of all array elements. - Loop through each integer in
elements
and apply the XOR operation. This effectively gives the cumulative XOR result of all elements in the array. - The method concludes by returning
true
if either of the following conditions is met:- The cumulative XOR result (
result
) is 0. - The number of elements in the array is even.
- The cumulative XOR result (
The XOR operation has a key property: x ^ x = 0
for any integer x
. Thus, the game strategy hinges on these conditions to determine if it’s possible to win the game based on the initial configuration of the board.
No comments yet.