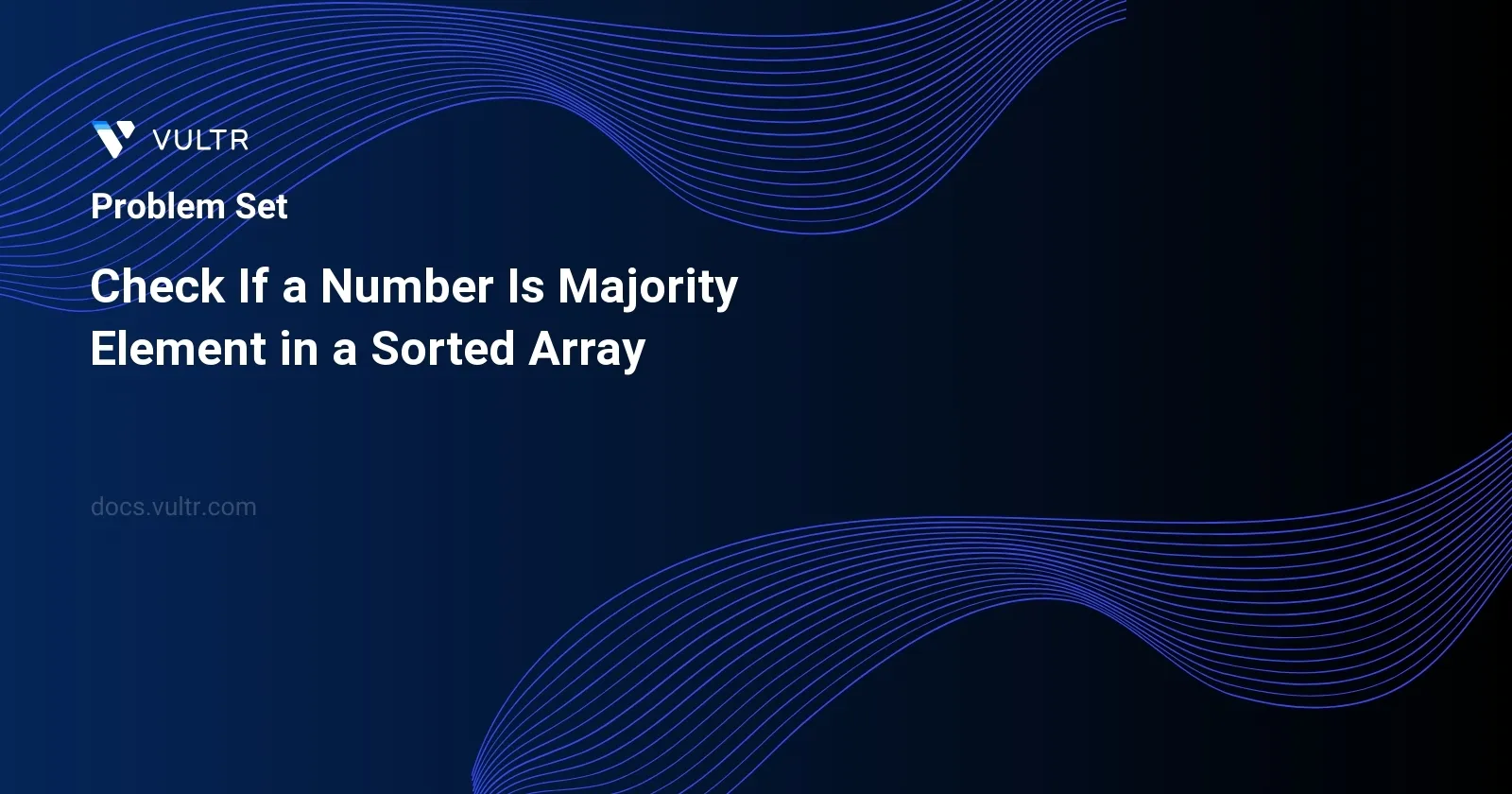
Problem Statement
The problem requires determining if a given integer target
is the majority element in a given sorted array nums
. A majority element is defined as an element that appears more than half the number of times in the list, that is, more than nums.length / 2
times. The objective is to return more true
if the target
is a majority element, otherwise return false
.
Examples
Example 1
Input:
nums = [2,4,5,5,5,5,5,6,6], target = 5
Output:
true
Explanation:
The value 5 appears 5 times and the length of the array is 9. Thus, 5 is a majority element because 5 > 9/2 is true.
Example 2
Input:
nums = [10,100,101,101], target = 101
Output:
false
Explanation:
The value 101 appears 2 times and the length of the array is 4. Thus, 101 is not a majority element because 2 > 4/2 is false.
Constraints
1 <= nums.length <= 1000
1 <= nums[i], target <= 109
nums
is sorted in non-decreasing order.
Approach and Intuition
To determine if target
is a majority element in nums
, consider the following steps:
- Calculate the required count for the majority, which is more than
nums.length / 2
. - Since the array is sorted, binary search can be efficiently used to find the first and last occurrence of
target
innums
, which will help in determining the total number of timestarget
appears in the array. - Check if the count of
target
surpasses the majority count calculated in step 1. If it does, returntrue
; else, returnfalse
.
From the examples:
- In Example 1,
target
is 5, and it appears 5 times innums
, which is more than9/2
, thus 5 is a majority element. - In Example 2,
target
is 101, appearing only 2 times innums
. Since2
is not more than4/2
, 101 is not a majority element.
Constraints Analysis
- The constraints ensure that even a straightforward approach that involves counting the number of times
target
appears will be within acceptable performance limits. - The sorted nature of
nums
provides a crucial insight that optimized searching techniques like binary search can be directly applied.
By taking advantage of the array's sorted property, effective search algorithms can significantly prune down the search space, making the solution both efficient and easy to understand.
Solutions
- C++
- Java
class Solution {
public:
int find_lower_bound(vector<int>& arr, int val) {
int left = 0;
int right = arr.size() - 1;
int pos = arr.size();
while (left <= right) {
int middle = (left + right) / 2;
if (arr[middle] >= val) {
right = middle - 1;
pos = middle;
} else {
left = middle + 1;
}
}
return pos;
}
bool checkMajorityElement(vector<int>& arr, int val) {
int firstPos = find_lower_bound(arr, val);
return (firstPos + arr.size() / 2 < arr.size()) && (arr[firstPos + arr.size() / 2] == val);
}
};
This C++ code implements a solution to determine if a number is the majority element in a sorted array. The provided C++ class Solution
includes two main functions:
find_lower_bound:
- This function computes the first position in the array where the value is greater than or equal to the target value
val
using a binary search algorithm. It adjusts theleft
,right
, andmiddle
indices based on the comparison between the middle element of the array andval
. The function returns the lowest indexpos
wherearr[pos] >= val
.
- This function computes the first position in the array where the value is greater than or equal to the target value
checkMajorityElement:
- This function first retrieves the lower bound position of
val
in the array usingfind_lower_bound
. - Then, it checks if the element at the position half the size of the array away from this starting point is still equal to
val
. This verification along with the comparison of indices determines ifval
is the majority element in the array. The function returnstrue
ifval
is the majority element, otherwisefalse
.
- This function first retrieves the lower bound position of
The essence of the solution is in efficiently locating the start occurrence of the potential majority element using binary search and then verifying its dominance by checking its occurrence at the calculated majority position.
This implementation is optimized for a sorted array, ensuring that the check operation is done in logarithmic time. This is significantly faster than a linear scan, especially for large arrays.
class Solution {
int findLowerBound(int[] array, int key) {
int left = 0;
int right = array.length - 1;
int result = array.length;
while (left <= right) {
int mid = (left + right) / 2;
if (array[mid] >= key) {
right = mid - 1;
result = mid;
} else {
left = mid + 1;
}
}
return result;
}
public boolean isMajorityElement(int[] array, int key) {
int lowerIndex = findLowerBound(array, key);
return lowerIndex + array.length / 2 < array.length && array[lowerIndex + array.length / 2] == key;
}
}
The provided Java program checks if a given number is the majority element in a sorted array. A number is considered a majority element if it appears more than half the times in the sorted array. Here is the explanation and breakdown of how this validation is achieved:
findLowerBound Method: Implements a binary search algorithm to find the lowest index (
lowerIndex
) of the givenkey
within thearray
. It keeps adjusting the search range (left
andright
) and updates the result to the mid-point where thekey
is found or supposed to be inserted. This method returns the lowest index where thekey
could be inserted without disturbing the order of the sorted array, hence effectively giving the first occurrence of thekey
.isMajorityElement Method: Utilizes the
findLowerBound
method to get thelowerIndex
ofkey
inarray
. It checks whether adding half the length of the array tolowerIndex
results in an index that still contains thekey
and compares whether this index doesn't exceed the boundary. If these conditions are true,key
is the majority element and the method returnstrue
. Otherwise, it returnsfalse
.
These methods together allow efficient checking of majority element presence by minimizing the need to iterate through the entire array, leveraging the property of binary search.
No comments yet.