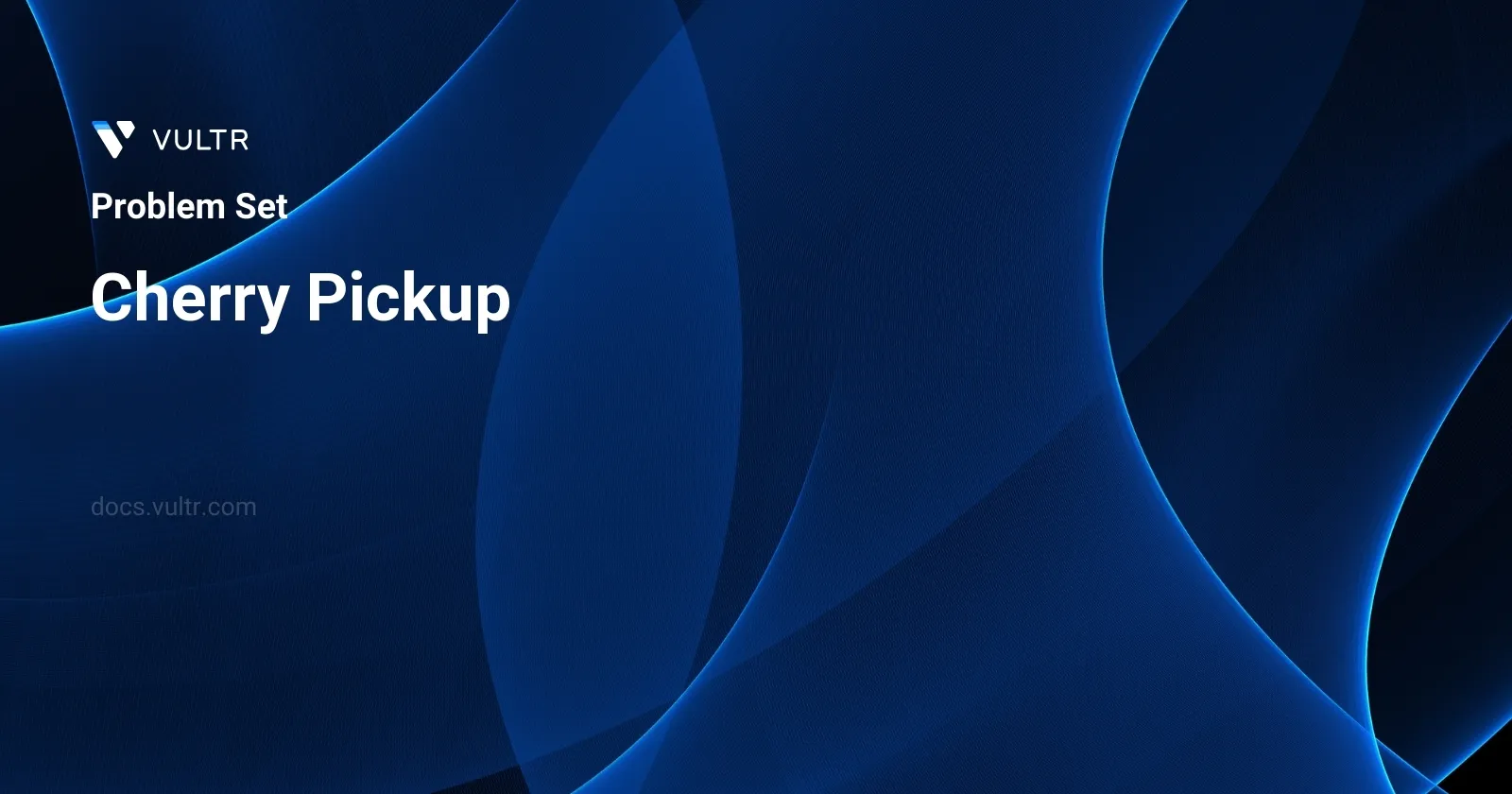
Problem Statement
In this scenario, you have a square grid of size n x n
where each cell holds one of three possible integer values:
0
representing an empty cell that you can navigate through,1
indicating a cherry is present, which you can collect and then the cell becomes empty,-1
signaling a thorn that blocks your path and prevents movement through that cell.
The main objective is to determine the maximum number of cherries you can collect by navigating the grid under specific rules. The journey consists of two major steps:
- Starting at the top-left corner of the grid
(0, 0)
, you need to travel to the bottom-right corner(n - 1, n - 1)
using valid pathways (i.e., moving through cells containing0
or1
only). - Once reaching
(n - 1, n - 1)
, you must return to(0, 0)
again only through valid cells.
During this journey, whenever you pass through a cell containing a cherry (1
), it is collected thus turning the cell into a 0
. The task is to return the maximum cherries collected following these rules. If for some reason, a valid path does not exist between (0, 0)
and (n - 1, n - 1)
, then the result should be zero since no cherries can be collected.
Examples
Example 1
Input:
grid = [[0,1,-1],[1,0,-1],[1,1,1]]
Output:
5
Explanation:
The player started at (0, 0) and went down, down, right right to reach (2, 2). 4 cherries were picked up during this single trip, and the matrix becomes [[0,1,-1],[0,0,-1],[0,0,0]]. Then, the player went left, up, up, left to return home, picking up one more cherry. The total number of cherries picked up is 5, and this is the maximum possible.
Example 2
Input:
grid = [[1,1,-1],[1,-1,1],[-1,1,1]]
Output:
0
Constraints
n == grid.length
n == grid[i].length
1 <= n <= 50
grid[i][j]
is-1
,0
, or1
.grid[0][0] != -1
grid[n - 1][n - 1] != -1
Approach and Intuition
To solve this problem efficiently, consider the following approach and underlying thought process:
Dynamic Programming Strategy:
- Use a memoization table to avoid redundant calculations. The table can store the maximum cherries collected for specific combinations of cell positions.
- The solution incorporates the aspect of two simultaneous journeys, one going towards
(n-1, n-1)
and another returning to(0, 0)
. Because of overlapping routes, there's an opportunity to optimize cherry collection.
Dual traversal technique:
- Travel not only from the top-left to the bottom-right but simultaneously consider the reversed route. This dual perspective helps in evaluating the optimal path and maximizes cherry collection.
- Ensure that thorny cells (
-1
) are avoided in both paths as they are impassable.
Recursive Exploration:
- Begin the recursive exploration from
(0,0)
, considering all potential paths to(n-1, n-1)
, and measure cherry collection. - From
(n-1, n-1)
, recursively calculate the number of cherries that can be collected on the way back to(0, 0)
.
- Begin the recursive exploration from
By maintaining a dynamic programming matrix or utilizing memoized recursion, the solution keeps track of the maximum cherries collectable from every cell when paired with its mirrored cell on the reverse journey. This technique directly considers the overlapping of paths during both trips, drastically increasing the efficiency of the cherry collection process.
In terms of constraints, make sure the movement is within grid bounds and does not cross over thorns, maintaining valid paths throughout. Given the grid sizes limitation (1 <= n <= 50
), this approach manages the potential complexity in a feasible manner, aiming at an optimal solution.
Solutions
- Java
class Solution {
public int collectCherries(int[][] matrix) {
int size = matrix.length;
int[][] cherryDP = new int[size][size];
for (int[] line: cherryDP) {
Arrays.fill(line, Integer.MIN_VALUE);
}
cherryDP[0][0] = matrix[0][0];
for (int step = 1; step <= 2*size - 2; ++step) {
int[][] tempDP = new int[size][size];
for (int[] line: tempDP) {
Arrays.fill(line, Integer.MIN_VALUE);
}
for (int i = Math.max(0, step - (size - 1)); i <= Math.min(size - 1, step); ++i) {
for (int j = Math.max(0, step - (size - 1)); j <= Math.min(size - 1, step); ++j) {
if (matrix[i][step - i] == -1 || matrix[j][step - j] == -1) {
continue;
}
int value = matrix[i][step-i];
if (i != j) {
value += matrix[j][step - j];
}
for (int previousI = i - 1; previousI <= i; ++previousI) {
for (int previousJ = j - 1; previousJ <= j; ++previousJ) {
if (previousI >= 0 && previousJ >= 0) {
tempDP[i][j] = Math.max(tempDP[i][j], cherryDP[previousI][previousJ] + value);
}
}
}
}
}
cherryDP = tempDP;
}
return Math.max(0, cherryDP[size - 1][size - 1]);
}
}
In the given Java solution for the "Cherry Pickup" problem, the primary strategy is to use dynamic programming to determine the maximum number of cherries that can be collected from a grid. This grid is represented by a 2D matrix where each cell either contains cherries (represented by positive integers), is an empty cell (represented by 0), or has thorns which make the cell impassable (represented by -1).
Here are the main steps and features of the implemented solution:
Initialize a 2D array 'cherryDP' of the same size as the input matrix to store the maximum cheries that can be collected up to each point in the grid. Start by setting all values in 'cherryDP' to a very low number (Integer.MIN_VALUE) to effectively represent unvisitable cells, except for the starting point [0][0], which is set to the value of matrix[0][0].
Use a nested loop to simulate the movement through the matrix using a 'step' variable, which combines both row and column indices (i, j being the current positions in the paths of two simultaneously moving individuals). Calculate steps from 1 to
2*size - 2
to cover possible movements within the bounds of the matrix.For each step, initialize a temporary DP array 'tempDP' similar to 'cherryDP' to store the current step's computation results.
Consider all possible positions (i, j) at each step and ensure that neither point is on a thorn (-1 value in the matrix). If not impassable, compute the potential maximum cherries to be collected at both points. If the two positions are not the same, sum the cherries from both cells; otherwise, just take the cherries from one.
Look back at all possible positions that could have been moved from to reach the current cell (i, j), i.e., (i-1, j), (i, j-1), and (i-1, j-1), making sure to stay within the grid bounds and that previous cells were accessible. Update 'tempDP' based on these values to reflect the maximum cherries collectable up to that point.
After processing all possible positions for a step, replace 'cherryDP' with 'tempDP'.
Finally, return the maximum value found at 'cherryDP[size-1][size-1]', which represents the maximum cherries collectable at the bottom-right corner of the grid. Ensure this value is at least zero, considering paths that might not collect any cherries.
Use this approach to efficiently solve the problem while managing edge cases like impassable cells. The solution ensures that both optimal path calculations and impassability are checked at every move, utilizing dynamic programming to build on solutions from previous steps.
No comments yet.