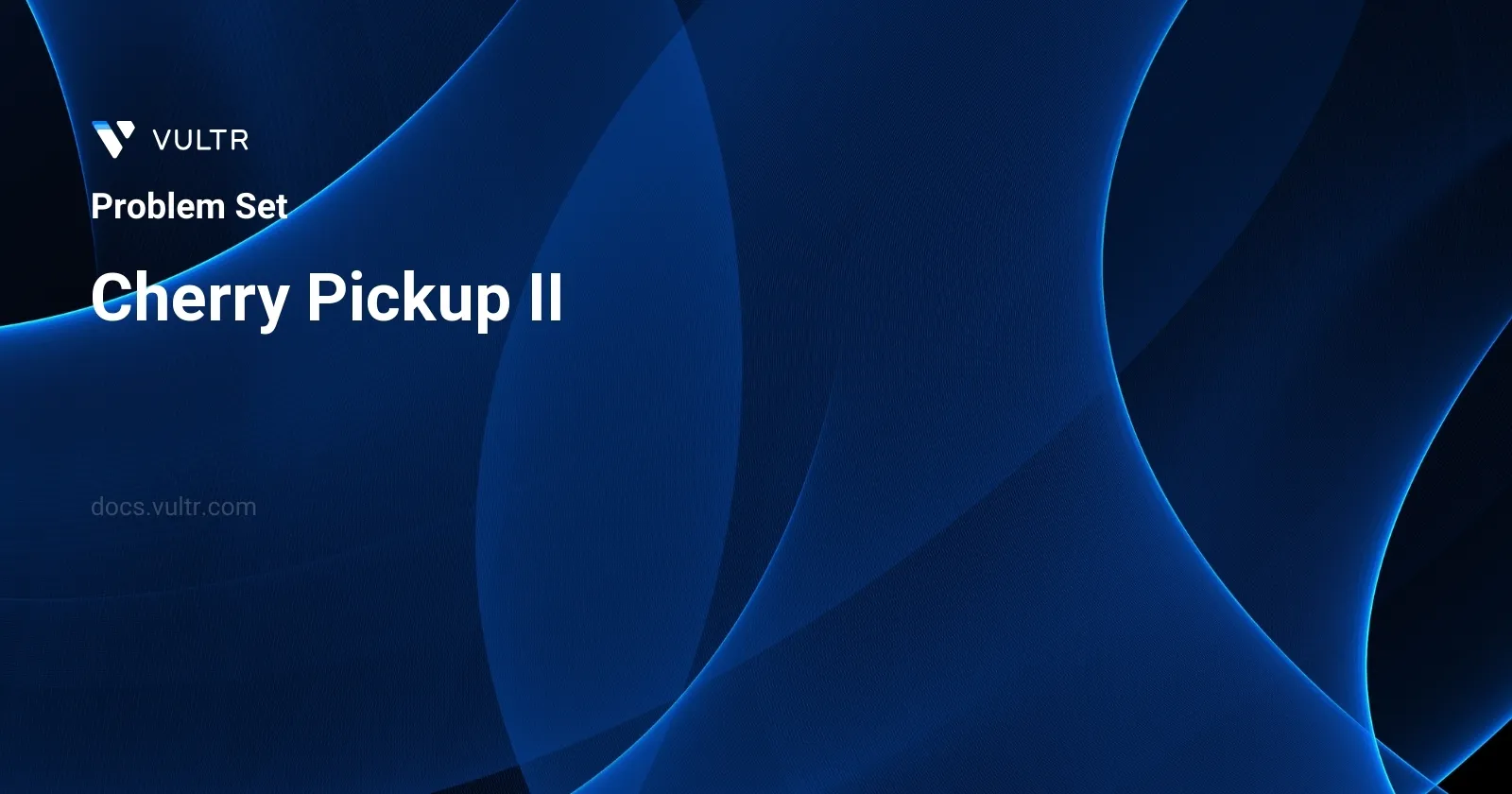
Problem Statement
In this problem, you are given a matrix called grid
which represents a cherry field. Each element in the grid indicates the number of cherries that can be collected from that specific cell. You have two robots to help you collect cherries:
- Robot #1 starts at the top-left corner of the matrix
(0, 0)
. - Robot #2 starts from the top-right corner
(0, cols - 1)
wherecols
is the number of columns in the matrix.
The objective is to calculate the maximum number of cherries both robots can collect by the time they reach the bottom row of the grid. Both robots can only move downwards to either the next left diagonal cell, the cell directly below, or the next right diagonal cell. Each time a robot visits a cell, all cherries in that cell are collected, and the cell becomes empty. If both robots land on the same cell, they still only collect the cherries once. They are not allowed to move outside the boundaries of the grid at any time.
Examples
Example 1
Input:
grid = [[3,1,1],[2,5,1],[1,5,5],[2,1,1]]
Output:
24
Explanation:
Path of robot #1 and #2 are described in color green and blue respectively. Cherries taken by Robot #1, (3 + 2 + 5 + 2) = 12. Cherries taken by Robot #2, (1 + 5 + 5 + 1) = 12. Total of cherries: 12 + 12 = 24.
Example 2
Input:
grid = [[1,0,0,0,0,0,1],[2,0,0,0,0,3,0],[2,0,9,0,0,0,0],[0,3,0,5,4,0,0],[1,0,2,3,0,0,6]]
Output:
28
Explanation:
Path of robot #1 and #2 are described in color green and blue respectively. Cherries taken by Robot #1, (1 + 9 + 5 + 2) = 17. Cherries taken by Robot #2, (1 + 3 + 4 + 3) = 11. Total of cherries: 17 + 11 = 28.
Constraints
rows == grid.length
cols == grid[i].length
2 <= rows, cols <= 70
0 <= grid[i][j] <= 100
Approach and Intuition
The above problem can be approached by considering how to maximize the collective harvesting as both robots progress through their paths from the top to the bottom of the grid. The two key dynamics here are optimizing the path for each robot such that they collect maximum cherries independently, and managing places where their paths overlap to ensure efficiency. Here's a breakdown of how the solution can be approached:
- Dynamic Programming (DP):
- A DP array can be used to store maximum cherries collected up to every possible position pair
(i, j1, j2)
of robot #1 and robot #2, wherei
is the row index, andj1
andj2
are the respective column indices for robots #1 and #2.
- A DP array can be used to store maximum cherries collected up to every possible position pair
- State Transition:
- For every position
(i, j1, j2)
calculate and update the cherries collected by considering all moves from the previous row that could lead to this state which includes:- Moving from above left, above, or above right for both robots.
- For every position
- Managing Overlapping Positions:
- Special attention is needed for the case where both robots end up on the same cell
(i, j)
. Essentially, only one of the robots’ collections can count in such cases to avoid double counting.
- Special attention is needed for the case where both robots end up on the same cell
- Initialization and Boundary Conditions:
- Start with the initial position where robot #1 is at
(0, 0)
and robot #2 is at(0, cols-1)
. As robots can only move within the boundary of the matrix, ensure the states respect these boundaries.
- Start with the initial position where robot #1 is at
- Result Computation:
- After populating the DP matrix, the bottom row will display the maximum cherries collectible when both robots reach any of the columns in the last row. The maximum of these values will be the desired result.
Given the constraints of the grid size (up to 70x70), this dynamic programming approach should computationally feasible in terms of time complexity, even though it involves a three-dimensional DP array and multiple state transitions.
Solutions
- Java
- Python
class Solution {
public int collectCherries(int[][] field) {
int rows = field.length;
int cols = field[0].length;
int cache[][][] = new int[rows][cols][cols];
for (int r = rows - 1; r >= 0; r--) {
for (int c1 = 0; c1 < cols; c1++) {
for (int c2 = 0; c2 < cols; c2++) {
int sum = 0;
// Collect cherries from current positions
sum += field[r][c1];
if (c1 != c2) {
sum += field[r][c2];
}
// Calculate maximum possible from next rows
if (r != rows - 1) {
int maxVal = 0;
for (int nextC1 = c1 - 1; nextC1 <= c1 + 1; nextC1++) {
for (int nextC2 = c2 - 1; nextC2 <= c2 + 1; nextC2++) {
if (nextC1 >= 0 && nextC1 < cols && nextC2 >= 0 && nextC2 < cols) {
maxVal = Math.max(maxVal, cache[r + 1][nextC1][nextC2]);
}
}
}
sum += maxVal;
}
cache[r][c1][c2] = sum;
}
}
}
return cache[0][0][cols - 1];
}
}
The provided Java solution for the Cherry Pickup II problem uses dynamic programming to determine the maximum number of cherries that can be collected by two robots moving on a grid. The method collectCherries
is designed to handle a grid (int[][] field
) where rows represent the different positions from top to bottom of the grid and columns represent the positions from left to right.
Key concepts of the solution:
- Initialize a 3D array
cache
to store maximum cherries collected up to each position for robot1 at columnc1
and robot2 at columnc2
for every rowr
. - Iterate from the bottom of the grid (
rows - 1
) to the top (0
), considering all possible positions of the two robots (c1
andc2
for columns). - For each position combination of
c1
andc2
, compute the sum of cherries collected by both robots. Robot located at the same column collects cherries only once. - If not at the last row, update the sum by adding the maximum possible cherries collected from the next row for any valid move of robot1 and robot2 (both can move to their current, left, or right adjacent columns if within grid boundary).
- Update the
cache
array with the calculated cherry sum for that position pair. - The result, maximum cherries collected starting from the top row with robots starting at the first and last column, is stored in
cache[0][0][cols - 1]
.
Implementation Steps:
- Initialize the
cache
3D array to store intermediary results. - Use nested loops to iterate over each row from bottom to top and each possible pair of column positions for the two robots.
- Inside the innermost loop, calculate the initial sum of cherries from the current column positions of robots, taking care not to double-count if both robots are in the same column.
- If not in the last row, loop through all possible next positions of both robots and update the sum with the maximum collected cherries from valid positions.
- Store the maximum collectible cherries for each combination of positions in the
cache
array. - Return the maximum cherries collectible with starting positions at the first and last columns of the top row.
This approach ensures time-efficient cherry collection computation for each possible robot position as they traverse the grid, making optimal use of dynamic programming to avoid redundant calculations.
class Solution:
def collectCherries(self, matrix: List[List[int]]) -> int:
rows = len(matrix)
cols = len(matrix[0])
memo = [[[0]*cols for _ in range(cols)] for __ in range(rows)]
for level in reversed(range(rows)):
for first in range(cols):
for second in range(cols):
cherries = 0
# pick cherries from current position
cherries += matrix[level][first]
if first != second:
cherries += matrix[level][second]
# transition to the next row
if level != rows-1:
cherries += max(memo[level+1][next_first][next_second]
for next_first in [first, first+1, first-1]
for next_second in [second, second+1, second-1]
if 0 <= next_first < cols and 0 <= next_second < cols)
memo[level][first][second] = cherries
return memo[0][0][cols-1]
Cherry Pickup II is a problem where you aim to maximize the number of cherries collected by two robots moving through a grid from the top to the bottom row. Each cell in the grid contains a certain number of cherries that the robots can collect. The objective is achieved using dynamic programming to store and compute maximum cherries that can be collected at each step, with both robots starting from different positions.
In the provided Python3 solution:
- Start by defining a function
collectCherries
in theSolution
class that takes a 2D listmatrix
as input. - Determine the number of rows and columns in the matrix.
- Initialize a 3D list
memo
to store the cherry picks for every position and state of the robots. - Begin from the last row and iterate upwards, considering all possible positions of the two robots (axes
first
for the first robot andsecond
for the second robot). - For each position, compute the number of cherries collected if both robots collect cherries from their respective positions (avoid double counting if both robots end up in the same column).
- Additionally, factor in the cherries collected from potential positions in the next row where the robots could move: directly below, one step to the left, or one step to the right, ensuring the positions are within the grid bounds.
- Return the maximum cherries collected starting from the first row, first and last columns as the initial positions of the two robots.
This approach ensures that by the time you compute the values for the top row in memo
, you have already considered all possible paths and accumulated cherry counts for the robots moving through all rows of the grid. This method returns the maximum number of cherries that can be collected by both robots when they traverse the grid optimally from top to bottom.
No comments yet.