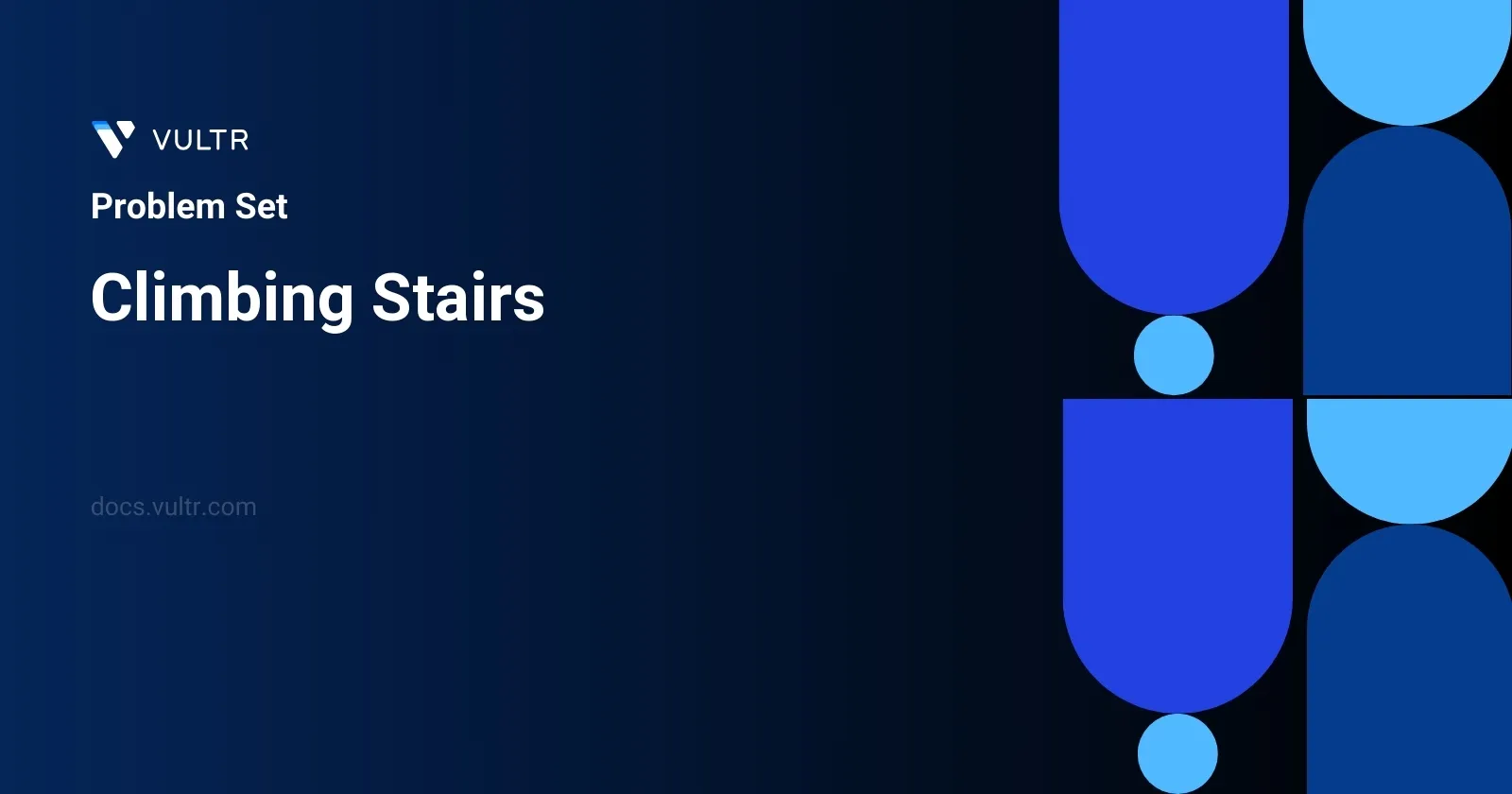
Problem Statement
The problem presents a scenario where you are attempting to climb a staircase that requires n
steps to reach the top. Each attempt to ascend the staircase allows you to climb either one step or two steps at a time. The challenge is to determine the total number of distinct methods by which you can reach the top of the staircase. The concept closely mimics the ways in which combinations of step sequences can be arranged to complete the series that sums precisely to n
.
Examples
Example 1
Input:
n = 2
Output:
2
Explanation:
There are two ways to climb to the top. 1. 1 step + 1 step 2. 2 steps
Example 2
Input:
n = 3
Output:
3
Explanation:
There are three ways to climb to the top. 1. 1 step + 1 step + 1 step 2. 1 step + 2 steps 3. 2 steps + 1 step
Constraints
1 <= n <= 45
Approach and Intuition
Given the problem description and the constraints, we can intuitively think of the solution in terms of dynamic programming or recursion because the problem has an optimal substructure and overlapping subproblems. Here's a step-by-step breakdown of the logic:
Base Cases:
- If
n = 1
, the only way to reach the top is by taking a single step. - If
n = 2
, there are two possible ways to reach the top: one step at a time (1+1), or all at once (2).
- If
Recursive Relation:
- For any step
n
, you can reach it fromn-1
by taking a single step or fromn-2
by taking two steps. Hence, the number of ways to reach stepn
can be derived from the sum of ways to reach the stepsn-1
andn-2
.
This leads to the recurrence relation f(n) = f(n-1) + f(n-2), which closely relates to the Fibonacci sequence.
- For any step
Implementation:
- Use either a recursive approach with memoization (to save previously computed results and prevent redundant calculations) or an iterative approach utilizing dynamic programming to build up solutions for all numbers up to
n
. - A straightforward approach can involve using an array where the indices represent the step numbers and the values represent the number of ways to reach those steps.
- Use either a recursive approach with memoization (to save previously computed results and prevent redundant calculations) or an iterative approach utilizing dynamic programming to build up solutions for all numbers up to
The complexity analysis would reveal that a dynamic programming solution where each state depends only on the previous two states can be very efficient, especially given the constraint where 1 <= n <= 45
. This problem setup ensures that our approach will run in optimal time without facing issues of stack overflow or excessive computational overhead that recursive solutions might face at higher values of n
.
Solutions
- C++
- Java
- C
- JavaScript
- Python
class Solution {
public:
int climbStairs(int steps) {
double sqrtOf5 = sqrt(5);
double golden = (1 + sqrtOf5) / 2;
double inverseGolden = (1 - sqrtOf5) / 2;
return (int)((pow(golden, steps + 1) - pow(inverseGolden, steps + 1)) / sqrtOf5);
}
};
The problem "Climbing Stairs" involves calculating the number of distinct ways to climb a given number of stairs where you can advance one or two steps at a time. The given C++ solution utilizes a mathematical approach involving the Fibonacci sequence due to the nature of the problem, where the number of ways to reach the nth step is the sum of the ways to reach the (n-1)th and (n-2)th steps.
Here's a breakdown of what the code does:
- Calculate values needed from the Fibonacci formula:
sqrtOf5
: the square root of 5.golden
: Phi (the golden ratio), calculated as(1 + sqrtOf5) / 2
.inverseGolden
: The conjugate of the golden ratio,(1 - sqrtOf5) / 2
.
- For the given number of steps, the function
climbStairs
determines the number of ways to climb using the formula derived from Binet's Fibonacci number formula. - It returns the result by calculating
((pow(golden, steps + 1) - pow(inverseGolden, steps + 1)) / sqrtOf5)
which effectively calculates the nth Fibonacci number where n issteps + 1
to account for starting on the ground (0th step).
The approach ensures efficient calculation leveraging the closed-form expression of Fibonacci, often called Binet's Formula, which allows for direct computation without iterative or recursive methods that could be less efficient for large inputs.
public class Solution {
public int climbStairs(int steps) {
double squareRootOf5 = Math.sqrt(5);
double goldenRatio = (1 + squareRootOf5) / 2;
double inverseGoldenRatio = (1 - squareRootOf5) / 2;
return (int) ((Math.pow(goldenRatio, steps + 1) - Math.pow(inverseGoldenRatio, steps + 1)) / squareRootOf5);
}
}
The "Climbing Stairs" solution in Java leverages the Fibonacci series to determine the number of ways to reach the top of a stairwell given a number of steps. The method climbStairs
uses a formula derived from Binet's Fibonacci number formula:
- Calculate the square root of 5.
- Define the golden ratio and its inverse.
- Use these values to compute the Fibonacci sequence formula that applies to climbing stairs:
(Math.pow(goldenRatio, steps + 1) - Math.pow(inverseGoldenRatio, steps + 1)) / squareRootOf5
.
This formula directly calculates the correct Fibonacci value for the number of ways to climb the stairs using a combination of exponentiation and natural constants to achieve an efficient and clear solution. This method handles the input steps
effectively, offering a mathematically sound approach to solving the problem without iterative or recursive methods that are often less efficient.
int calculateSteps(int steps) {
double root5 = sqrt(5);
double golden = (1 + root5) / 2;
double silver = (1 - root5) / 2;
return (int)((pow(golden, steps + 1) - pow(silver, steps + 1)) / root5);
}
The provided C function calculateSteps
computes the number of ways to climb a given number of stairs using a mathematical approach based on the Fibonacci sequence. Each step can be calculated with the formula involving the golden ratio (approximately 1.618) and its conjugate, the silver ratio.
The function accepts an integer
steps
as its parameter, representing the total number of steps.It starts by computing the square root of 5 and storing it in
root5
.It then calculates the golden ratio (
golden
) and the silver ratio (silver
).The result is achieved using a direct application of Binet's Formula where:
- It calculates the power of
golden
andsilver
raised to the parametersteps+1
. - The difference of these powers divided by
root5
gives the result.
- It calculates the power of
The function finally casts this result to an integer and returns it.
This efficient approach ensures that you can compute the number of ways to climb stairs without iterative or recursive calculations, making it computationally efficient even for large numbers of steps.
var countWays = function (steps) {
var root5 = Math.sqrt(5);
var goldenRatio = (1 + root5) / 2;
var silverRatio = (1 - root5) / 2;
return Math.floor((Math.pow(goldenRatio, steps + 1) - Math.pow(silverRatio, steps + 1)) / root5);
};
The provided JavaScript function countWays
solves the problem of determining the number of ways to climb a staircase with a given number of steps, utilizing the Fibonacci sequence.
- The function receives an integer
steps
as its parameter, representing the total number of steps in the staircase. - It initially calculates the square root of 5 and stores this value in
root5
. - The function defines
goldenRatio
which symbolizes the (1 + root5)/2, commonly related to the positive part of the Fibonacci closed-form expression. - Similarly,
silverRatio
is calculated as (1 - root5)/2, representing the negative part of the Fibonacci formula. - Lastly, the function computes the number of ways by using the formula of the Fibonacci sequence applied directly to the staircase problem:
(goldenRatio^(steps + 1) - silverRatio^(steps + 1)) / root5
. Math.floor
is used to ensure that the result is returned as an integer, which is the expected format for counting distinct ways.
By using the Fibonacci sequence's properties, this function efficiently calculates the number of distinct ways to climb the stairs with complexity essentially linear in terms of power calculation time, making it very efficient for large numbers of steps. The Fibonacci-based approach is both elegant and effective for the climbing stairs problem, exploiting the natural pattern in which climbing configurations grow.
class Solution:
def countWays(self, steps: int) -> int:
root5 = 5 ** 0.5
golden_ratio = (1 + root5) / 2
inv_golden_ratio = (1 - root5) / 2
return int((golden_ratio ** (steps + 1) - inv_golden_ratio ** (steps + 1)) / root5)
The provided Python solution addresses the problem of determining the number of ways to climb stairs, using the Fibonacci sequence formula. The method countWays
in the Solution
class calculates the number of distinct ways to reach the top of a staircase with a given number of steps, referred to as steps
.
The solution utilizes the mathematical concept of the golden ratio and its inverse to compute the Fibonacci number that corresponds to the provided number of steps plus one. This is achieved through the following process:
- Calculate the square root of 5.
- Derive the golden ratio as
(1 + root5) / 2
. - Calculate the inverse of the golden ratio as
(1 - root5) / 2
. - Use these constants to apply the formula for finding the nth Fibonacci number:
((golden_ratio ** (steps + 1) - inv_golden_ratio ** (steps + 1)) / root5)
. - Convert the result into an integer which represents the total ways to climb the staircase.
This approach effectively harnesses a mathematical shortcut (Binet's Fibonacci Number Formula) to achieve a result without the need for iterative looping or recursion, thus optimizing performance by directly computing the result through mathematical expressions.
No comments yet.