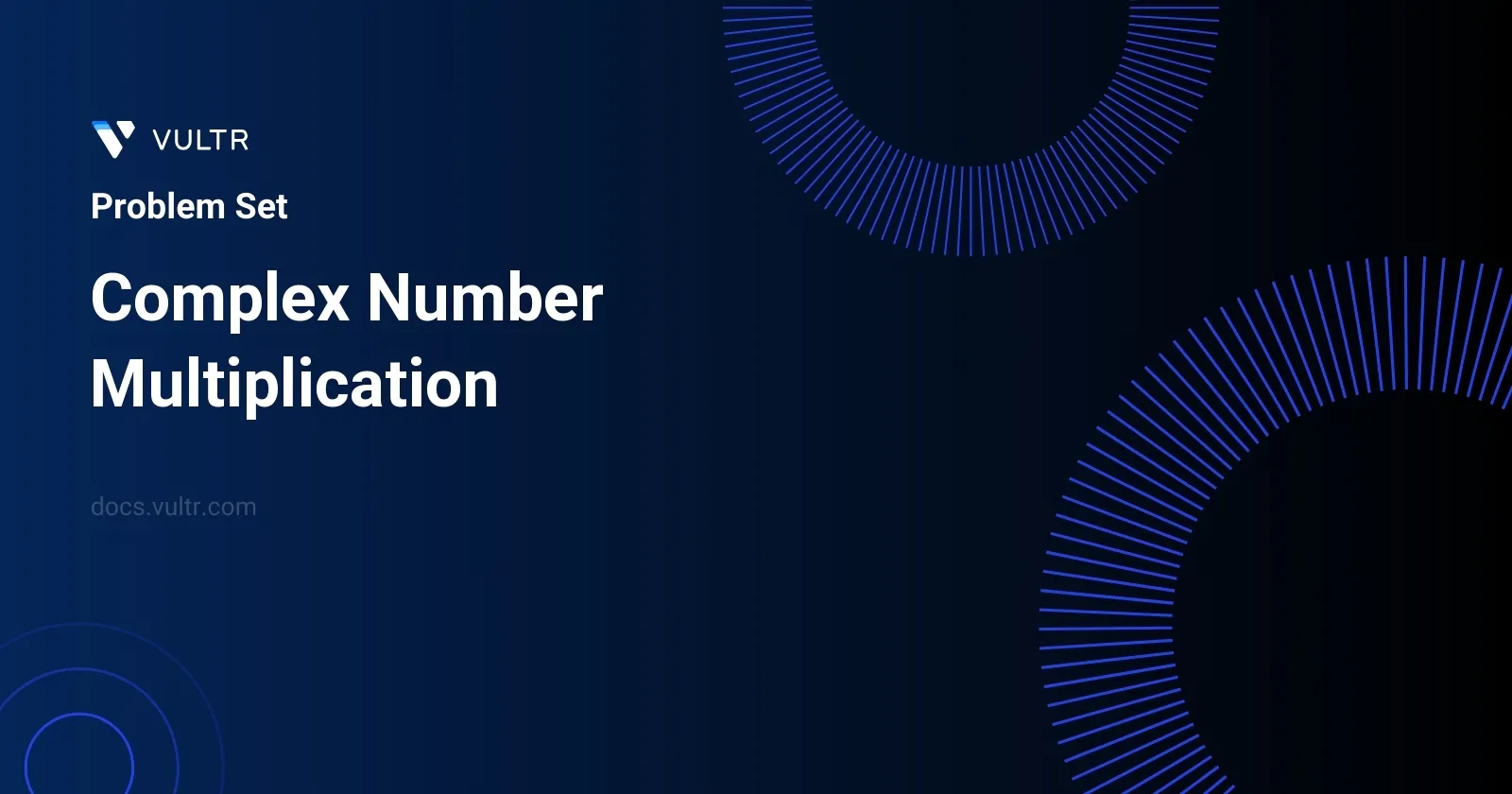
Problem Statement
In mathematics, a complex number is typically expressed as ( a + bi ), where ( a ) represents the real part and ( b ) the imaginary part, with ( i ) being the imaginary unit satisfying ( i^2 = -1 ). In this problem, we are provided with two complex numbers in string format, where the real and imaginary components are distinctly provided within each string. Each part—real and imaginary—ranges between -100 and 100.
Given these two strings each representing a complex number, your task will be to compute the product of these two numbers and represent the result as a string in the identical format, adhering strictly to the rules of complex number multiplication.
Examples
Example 1
Input:
num1 = "1+1i", num2 = "1+1i"
Output:
"0+2i"
Explanation:
(1 + i) * (1 + i) = 1 + i2 + 2 * i = 2i, and you need convert it to the form of 0+2i.
Example 2
Input:
num1 = "1+-1i", num2 = "1+-1i"
Output:
"0+-2i"
Explanation:
(1 - i) * (1 - i) = 1 + i2 - 2 * i = -2i, and you need convert it to the form of 0+-2i.
Constraints
num1
andnum2
are valid complex numbers.
Approach and Intuition
Understanding the operation and mechanics behind the multiplication of two complex numbers will guide us into crafting our solution. The given examples provide a glimpse into the logic needed:
Understanding Complex Number Multiplication:
For two complex numbers ( num1 = a + bi ) and ( num2 = c + di ):
The product can be computed using the formula: [ (a + bi)(c + di) = ac + adi + bci + bdi^2 ] Simplifying further as ( i^2 = -1 ): [ ac + adi + bci - bd = (ac - bd) + (ad + bc)i ]
This formula will help you to compute:
- The real component as ( ac - bd ).
- The imaginary component as ( ad + bc ).
Steps:
- Parse the input strings to extract ( a ), ( b ), ( c ), and ( d ).
- Apply the multiplication formula to compute the new components.
- Construct the resultant string in the format ( "real+imaginaryi" ).
This approach ensures that you are not only calculating the components correctly but also adhering to the specified output format. As indicated by the constraints, inputs will be properly formatted and within the specified range, so input validation beyond parsing is generally not required.
Solutions
- Java
public class Solution {
public String multiplyComplex(String num1, String num2) {
String parts1[] = num1.split("\\+|i");
String parts2[] = num2.split("\\+|i");
int realPart1 = Integer.parseInt(parts1[0]);
int imaginaryPart1 = Integer.parseInt(parts1[1]);
int realPart2 = Integer.parseInt(parts2[0]);
int imaginaryPart2 = Integer.parseInt(parts2[1]);
return (realPart1 * realPart2 - imaginaryPart1 * imaginaryPart2) + "+" + (realPart1 * imaginaryPart2 + imaginaryPart1 * realPart2) + "i";
}
}
The provided Java solution efficiently handles the multiplication of two complex numbers. The implemented method, multiplyComplex
, takes two strings representing complex numbers as input. These complex numbers are expected in the format "a+bi", where 'a' and 'b' are integers.
- Start by breaking down each complex number string into their real and imaginary components using the
split
method with regex that targets the '+' sign and the character 'i'. This produces an array where:- The first element is the real part.
- The second element, after excluding the 'i', is the imaginary part.
- Convert these string representations of the real and imaginary parts into integers.
- Apply the formula for complex number multiplication:
- Real part: (Real part of first number × Real part of second number) - (Imaginary part of first number × Imaginary part of second number).
- Imaginary part: (Real part of the first number × Imaginary part of the second number) + (Imaginary part of the first number × Real part of the second number).
- Construct the result as a string in the format "c+di", where 'c' and 'd' are results of the calculations for the real and imaginary parts, respectively, and return this string.
This method effectively calculates and returns the product of two complex numbers as a new complex number in string format.
No comments yet.