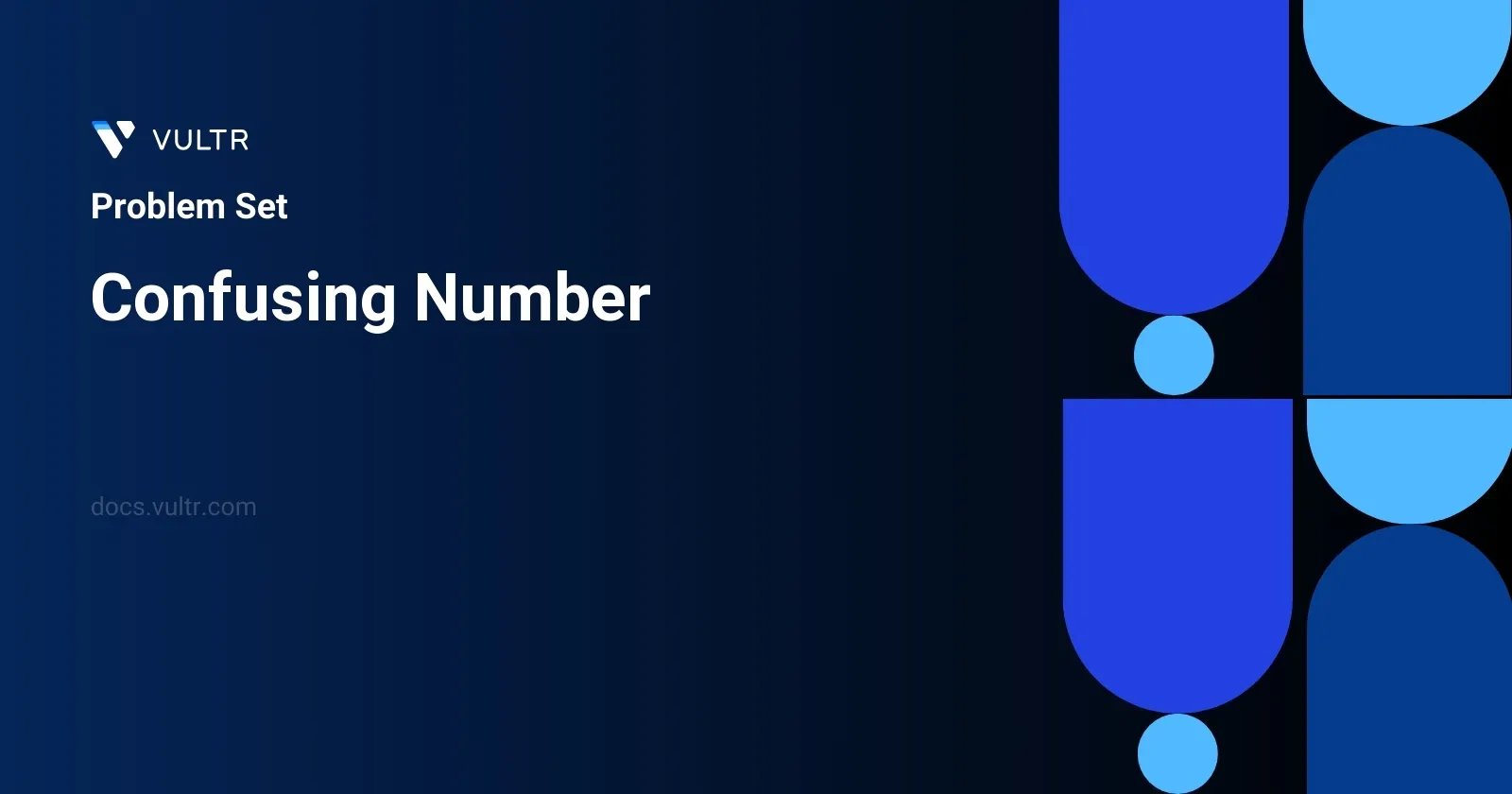
Problem Statement
The concept of a confusing number involves determining if a number becomes a different number when each of its digits is rotated by 180 degrees. Not all digits can be rotated to form valid digits using this rotation technique. The digits 0
, 1
, 6
, 8
, and 9
transform into other valid digits - they turn into 0
, 1
, 9
, 8
, and 6
, respectively, upon rotation. Conversely, the digits 2
, 3
, 4
, 5
, and 7
do not yield valid numbers when rotated by 180 degrees.
A number is defined as "confusing" if, after this rotation, it does not retain its original form and all the transformed digits remain valid. For example, the number 6
becomes 9
and does not equal its original form, thus making it a confusing number. However, the number 11
remains unchanged after rotation and therefore is not confusing. In this task, given any integer n
, the objective is to check whether it is a confusing number or not by following these rotation rules and return a boolean value (i.e., true
or false
).
Examples
Example 1
Input:
n = 6
Output:
true
Explanation:
We get 9 after rotating 6, 9 is a valid number, and 9 != 6.
Example 2
Input:
n = 89
Output:
true
Explanation:
We get 68 after rotating 89, 68 is a valid number and 68 != 89.
Example 3
Input:
n = 11
Output:
false
Explanation:
We get 11 after rotating 11, 11 is a valid number but the value remains the same, thus 11 is not a confusing number
Constraints
0 <= n <= 109
Approach and Intuition
To determine whether a given number n
is a confusing number, follow the below approach:
- Convert the number
n
to its string representation to access each digit individually. - Create a dictionary to map each rotatable digit to its corresponding digit post-rotation (e.g.,
{'6': '9', '9': '6', '0': '0', '1': '1','8': '8'}
). - Iterate through each digit of the number:
- Check if the digit is rotatable (i.e., it exists in the transformation dictionary).
- If any digit is not rotatable (not present in the dictionary), immediately return
false
as the number contains invalid digits post-rotation.
- Construct the rotated number by appending the transformed digits in reverse order (as rotating affects the sequence).
- Compare the newly formed number after all valid transformations to the original number.
- If the rotated number is different from the original and all digits are valid, return
true
, indicating it is a confusing number. - If the rotated number is the same as the original, return
false
, as the number is not confusing.
- If the rotated number is different from the original and all digits are valid, return
By following this plan, one can efficiently determine whether a number n
qualifies as a confusing number by handling the rotation and validation of each digit. This technique ensures that all conditions regarding the transformation and validity of digits are carefully observed, aligning with the problem constraints mentioned.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool isConfusing(int num) {
unordered_map<char, char> reverseMap = {{'0','0'}, {'1','1'}, {'6','9'}, {'8','8'}, {'9','6'}};
string transformed;
for (auto digit : to_string(num)) {
if (reverseMap.find(digit) == reverseMap.end()) {
return false;
}
transformed += reverseMap[digit];
}
reverse(begin(transformed), end(transformed));
return stoi(transformed) != num;
}
};
The "Confusing Number" problem involves determining if a number becomes different when rotated 180 degrees. The code solution provided is written in C++ and effectively solves this problem.
First, a mapping (using
unordered_map
) is set up where each digit maps to its counterpart when rotated 180 degrees. For instance, '6' becomes '9' and vice versa, while '0', '1', and '8' remain the same. Not all digits can be rotated to form other numbers, so digits like '2', '3', '4', '5', and '7' aren't included in the map.The
isConfusing
function starts by converting the integer into a string for easy manipulation of each digit. As it iterates over each digit, the function checks if the digit is in thereverseMap
. If a digit does not exist in this map, the function immediately returnsfalse
, indicating the number isn't confusing.If all digits are in the map, the function constructs a new string (
transformed
) by appending the rotated counterpart of each digit, effectively simulating the 180-degree rotation.After constructing the transformed string, it is reversed to replicate the effect of seeing the number from another angle or perspective.
Finally, the function examines if the integer value of the transformed string is different from the original number. If they are different, it returns
true
, marking the number as confusing; otherwise, it returnsfalse
.
This approach ensures that only numbers whose digits can all be rotated and which look different after the entire transformation process are identified as confusing. Utilizing string manipulation and map lookup provides an efficient way to check each digit individually and then aggregate the results to determine the final outcome.
class Solution {
public boolean isConfusingNumber(int number) {
// Map to hold digits and their rotations
Map<Character, Character> rotationMap = new HashMap<>() {{
put('0', '0');
put('1', '1');
put('6', '9');
put('8', '8');
put('9', '6');
}};
StringBuilder rotated = new StringBuilder();
// Convert number to string and process each digit
for (char digit : String.valueOf(number).toCharArray()) {
if (!rotationMap.containsKey(digit)) {
return false;
}
// Append the rotated value of the digit to 'rotated'
rotated.append(rotationMap.get(digit));
}
// Reverse and compare with original number
rotated.reverse();
return Integer.parseInt(rotated.toString()) != number;
}
}
The given Java solution checks if a number is confusing, defined by a number that when rotated 180 degrees becomes a different number. The method implemented, isConfusingNumber
, performs this check using a series of steps:
A
HashMap
namedrotationMap
is initialized to store valid digits and their corresponding rotations. The mapping includes:- '0' rotated to '0'
- '1' rotated to '1'
- '6' rotated to '9'
- '8' rotated to '8'
- '9' rotated to '6'
A StringBuilder
rotated
is used to construct the rotated version of the input number. The method iterates over each digit in the number, and for each digit:- Checks if the digit is present in
rotationMap
. If any digit is not present, the number cannot be a confusing number, and the method returnsfalse
. - Appends the rotated counterpart of the digit from
rotationMap
torotated
.
- Checks if the digit is present in
After processing all digits,
rotated
is reversed using theStringBuilder reverse
method, as the digits need to be in reverse order to represent the number seen from 180 degrees.Finally, the method compares the integer value of the reversed, rotated string to the original number. If they are not equal, then the number is confusing, and
true
is returned. Otherwise, the method returnsfalse
.
This method efficiently uses data structures and string manipulation to determine if a number is confusing per the defined criteria.
class Solution:
def isConfusing(self, number: int) -> bool:
# Mapping of valid digits and their rotational equivalents
rotational_map = {"0":"0", "1":"1", "8":"8", "6":"9", "9":"6"}
transposed_digits = []
# Analyze each digit and its possibility to be rotated
for digit in str(number):
if digit not in rotational_map:
return False
# Insert the mapped rotated digit into the list
transposed_digits.append(rotational_map[digit])
# Join digits to form the rotated number
transposed_digits = "".join(transposed_digits)
# If the rotated number reads differently backward, it is confusing
return int(transposed_digits[::-1]) != number
The "Confusing Number" solution implements a function isConfusing
to determine if a number becomes a different number when each of its digits is rotated 180 degrees.
- Start by creating a mapping of digits to their rotational equivalents. This includes mappings like '6' to '9' and '9' to '6', among others.
- Initialize an empty list
transposed_digits
to store the digits of the rotated number. - Convert the number to a string and iterate through each digit.
- If a digit does not have a rotational equivalent (i.e., it's not present in the
rotational_map
), the number cannot be a confusing number, and the function returnsFalse
. - For digits that can be rotated, look up their rotational equivalent in the
rotational_map
and append this value totransposed_digits
. - After processing all digits, join
transposed_digits
to form the rotated number represented as a string. - Convert the rotated number from string back to integer and compare it to the original number.
- Return
True
if the original number and the rotated number, when reversed, are not the same. This confirms that the number is confusing.
This Python function is efficient due to its use of string manipulation and dictionary mapping, avoiding more complex conditional structures.
No comments yet.