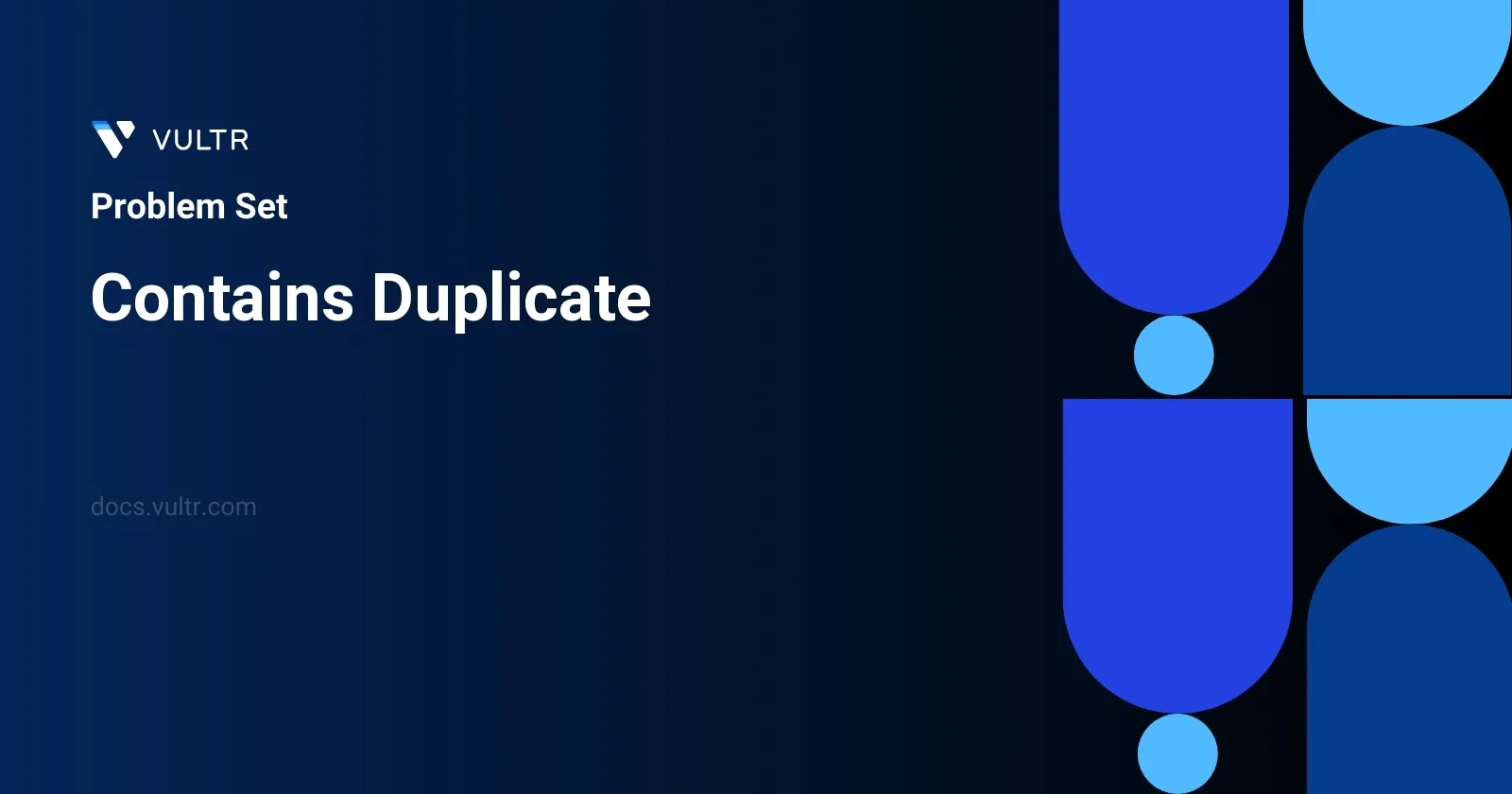
Problem Statement
The task is to determine whether an integer array nums
contains any duplicate elements. Specifically, you need to check if at least one value appears more than once within the array. If this condition is met, your function should return true
. Conversely, if each element in the array is unique and no duplications are found, the function should then return false
. This checks the nature of the array in terms of repetition of elements and assists in understanding the distribution of values in the dataset provided.
Examples
Example 1
Input:
nums = [1,2,3,1]
Output:
true
Explanation:
The element 1 occurs at the indices 0 and 3.
Example 2
Input:
nums = [1,2,3,4]
Output:
false
Explanation:
All elements are distinct.
Example 3
Input:
nums = [1,1,1,3,3,4,3,2,4,2]
Output:
true
Constraints
1 <= nums.length <= 105
-109 <= nums[i] <= 109
Approach and Intuition
The problem outlined involves checking for duplicate values in an integer array. The challenge simplifies to determining whether any element appears more than once. Let’s break down the approach based on the provided examples and constraints.
Naive Approach:
- One straightforward method could be to compare each pair of elements in the array.
- However, with an array length up to (10^5), this would result in substantial computation, making this method inefficient for larger arrays due to its (O(n^2)) time complexity.
Optimized Approach:
- Utilize a data structure that allows checks for existence in constant time, like a set.
- Iterate through each element of the array and before inserting it into the set, check if it is already present:
- If it is, return
true
because a duplicate exists. - If not, add the element to the set and continue.
- If it is, return
- If the iteration completes without finding duplicates, return
false
. - This method enhances the performance and ensures that you can check an element in constant time with a space complexity in the order of (O(n)) and time complexity also in (O(n)).
- Examples Analysis:
Example 1 (
nums = [1,2,3,1]
):- Here,
1
appears twice which is accurately detected by checking against existing elements in the set. - Output here is
true
.
- Here,
Example 2 (
nums = [1,2,3,4]
):- All elements are distinct with no repetitions, hence the output is
false
.
- All elements are distinct with no repetitions, hence the output is
Example 3 (
nums = [1,1,1,3,3,4,3,2,4,2]
):- Several numbers appear more than once e.g.,
1
,3
, and4
. A checking mechanism using a set quickly identifies these repetitions. - Output is
true
due to the multiple duplicates detected.
- Several numbers appear more than once e.g.,
Using a set for this problem is both intuitive and efficient given the constraints. It avoids unnecessary comparisons and scales well with larger inputs, ensuring quick detection of any repetitions in the array elements.
Solutions
- Java
public boolean hasDuplicate(int[] elements) {
HashSet<Integer> numbers = new HashSet<>(elements.length);
for (int num : elements) {
if (numbers.contains(num)) return true;
numbers.add(num);
}
return false;
}
This solution addresses the problem of determining whether a given array of integers contains any duplicate values. The implemented method, hasDuplicate
, utilizes Java's HashSet
collection to track the numbers that have been encountered.
- Initialize a
HashSet
namednumbers
, which benefits from constant time complexity for add and contains operations, making it ideal for this check. - Iterate over each integer in the provided
elements
array:- If the current integer is already present in the
HashSet
, returntrue
, indicating a duplicate exists. - Otherwise, add the integer to the
HashSet
.
- If the current integer is already present in the
- If the loop completes without finding a duplicate, return
false
.
This method ensures an efficient check with a time complexity of O(n), where n is the number of elements in the array, and it effectively handles arrays of any length.
No comments yet.