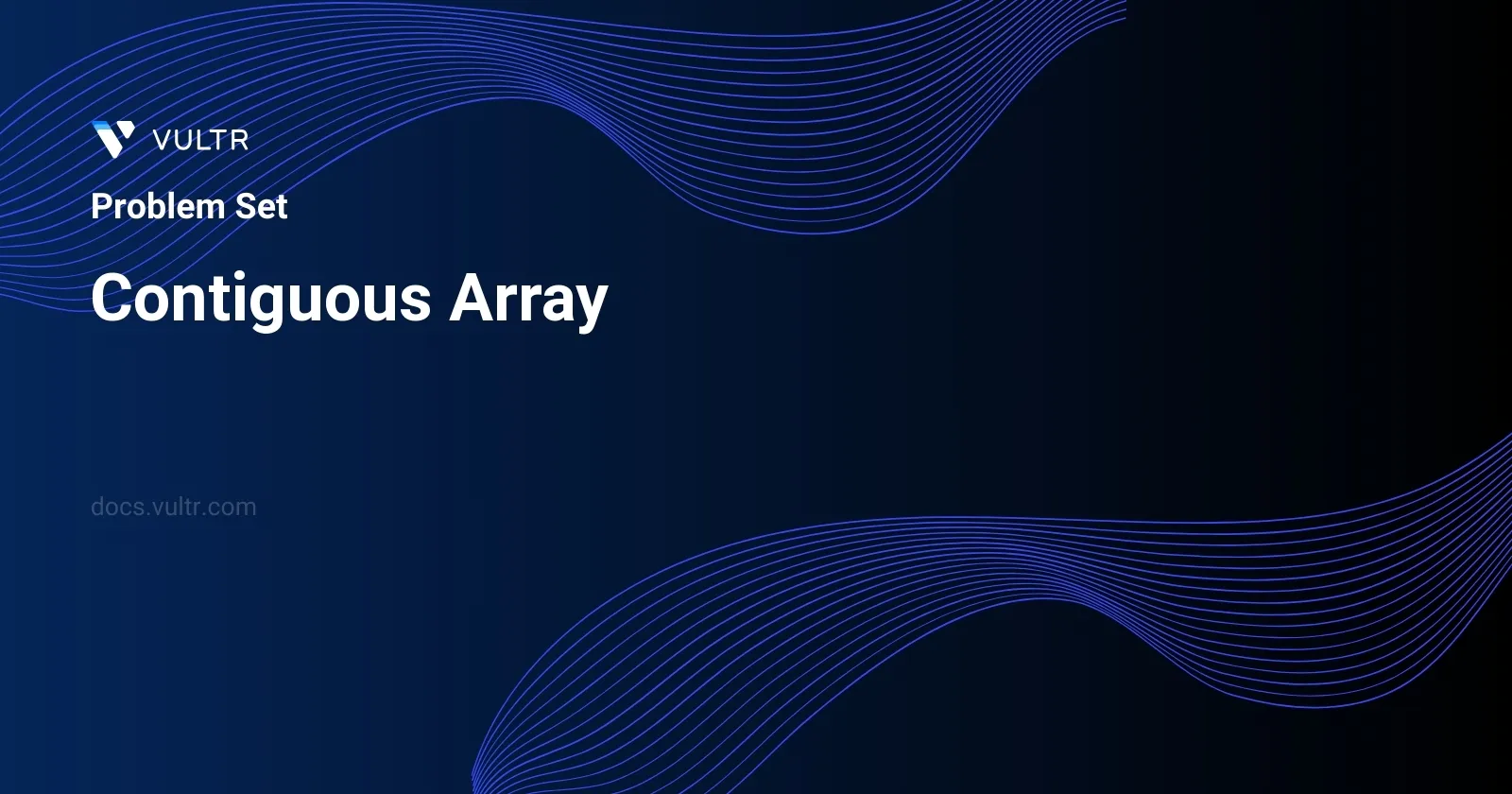
Problem Statement
In this task, we are presented with a binary array called nums
. Our goal is to determine the length of the longest contiguous subarray where the number of 0
s and the number 1
s are the same. This problem tests our ability to efficiently traverse and analyze subsections of an array to balance specified criteria.
Examples
Example 1
Input:
nums = [0,1]
Output:
2
Explanation:
[0, 1] is the longest contiguous subarray with an equal number of 0 and 1.
Example 2
Input:
nums = [0,1,0]
Output:
2
Explanation:
[0, 1] (or [1, 0]) is a longest contiguous subarray with equal number of 0 and 1.
Constraints
1 <= nums.length <= 105
nums[i]
is either0
or1
.
Approach and Intuition
Given the nature of our problem, solving it requires an understanding of subarray dynamics and the delicate balance between occurrences of elements. Here’s a systematic breakdown of potential approaches and intuitions drawn from the examples:
Understanding the Basic Idea:
- We need a subarray (continuous part of the original array) where the count of
0
s equals the count of1
s. This implies that for any length of the subarray, the number of1
s we add is exactly offset by the number of0
s.
- We need a subarray (continuous part of the original array) where the count of
Illustrative Examples Explanation:
- Example 1: Here,
nums = [0,1]
. This array itself is the largest subarray where the count of0
s and1
s are equal, giving us the maximum length of2
. - Example 2: In
nums = [0,1,0]
, the optimal subarrays could be[0,1]
or[1,0]
, both providing a balanced subarray with a length of2
.
- Example 1: Here,
Using a HashMap to Track Balances:
- A possible approach involves using a hashmap to keep track of the balance between
0
s and1
s as we iterate through the array. Here balance can be calculated by considering0
as-1
and1
as+1
. Hence, the balance changes as per the element we encounter. This balance helps us understand if and when the subarray returns to a previous state. - Specifically, the balance at each index
i
is defined as the number of1
s minus the number of0
s from the start to indexi
. If this balance repeats at indexj
, it means the subarray between these indices has an equal number of0
s and1
s.
- A possible approach involves using a hashmap to keep track of the balance between
Selecting the Right Data Structure:
- Use a map to store the first occurrence of each balance level. If the same balance level is reached again at a later index, then we can calculate the length of the subarray that lies between these repetitive balance indices.
Edge Cases:
- Single element arrays or arrays where all elements are
0
or all are1
can quickly be checked at the start as they will not contribute to a valid required subarray. This can enhance efficiency by reducing unnecessary computation.
- Single element arrays or arrays where all elements are
By considering these steps and underlying methodologies, the problem can be addressed systematically, ensuring that we efficiently find the required subarray without exhaustive search.
Solutions
- Java
public class Solution {
public int maxSubArrayLength(int[] nums) {
Map<Integer, Integer> indexMap = new HashMap<>();
indexMap.put(0, -1);
int maxLen = 0, balance = 0;
for (int index = 0; index < nums.length; index++) {
balance += (nums[index] == 1 ? 1 : -1);
if (indexMap.containsKey(balance)) {
maxLen = Math.max(maxLen, index - indexMap.get(balance));
} else {
indexMap.put(balance, index);
}
}
return maxLen;
}
}
The provided Java code addresses the problem of finding the maximum length of a contiguous subarray with an equal number of 0's and 1's. This approach efficiently uses a hashmap to track the first occurrences of balance values, where the "balance" tracks the difference between the count of 1's and 0's.
Here's a breakdown of how the code achieves this:
- A hashmap
indexMap
is initialized to store balance values and their corresponding indices. It starts by mapping a balance of 0 to an index of -1, covering the base case before the array begins. - A variable
maxLen
is initialized to keep track of the maximum length of any found subarray with equal numbers of 0's and 1's. - Another variable,
balance
, is used to keep a running difference between the number of 1's and 0's encountered as the array is iterated through. If a1
is encountered, the balance increases by 1; if0
is encountered, it decreases by 1. - As the loop progresses through the array:
- If the current
balance
has occurred before (found inindexMap
), it means a subarray with an equal number of 0's and 1's is found. UpdatemaxLen
by calculating the length of this subarray. - If this is the first occurrence of the current
balance
, store this balance with its index to reference in potential future matches.
- If the current
The function finally returns the value of maxLen
, which holds the length of the longest contiguous subarray fulfilling the condition.
To use this class and method:
- Ensure the class and method are accessible in the Java application or testing environment.
- Create an instance of the class
Solution
. - Call the method
maxSubArrayLength
passing the binary array (only containing 0's and 1's) as the parameter.
This implementation is efficient, achieving the solution in linear time complexity, O(n), where n is the length of the input array, due to the single pass and constant-time hashmap operations.
No comments yet.