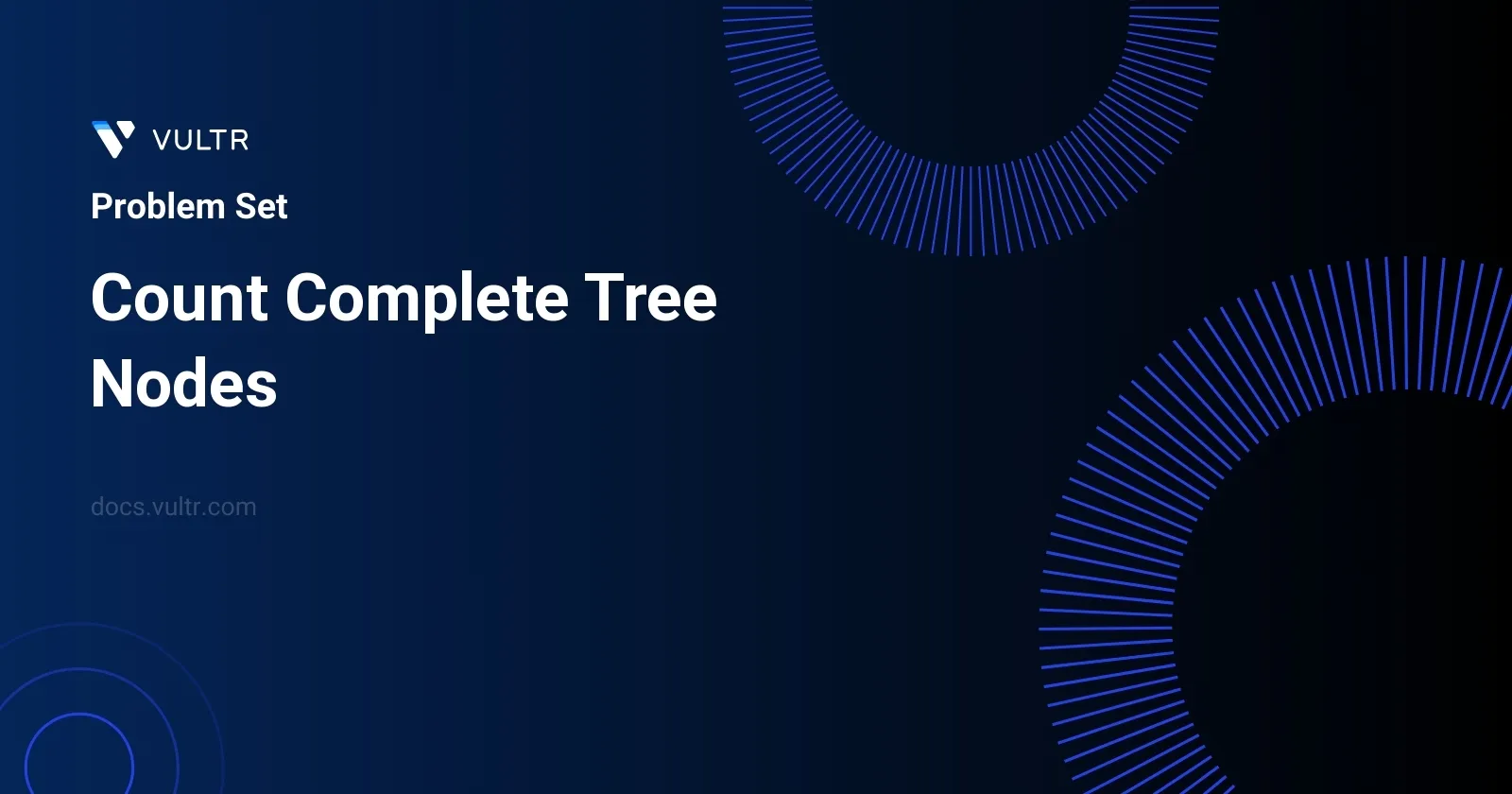
Problem Statement
This task involves formulating an algorithm to determine the number of nodes in a complete binary tree, given its root. In a complete binary tree, every level is fully populated except for potentially the last level, which must have all its nodes aligned to the left. The algorithm should efficiently compute the total node count, striving to achieve a time complexity lower than O(n)
, where n
represents the total number of nodes.
Examples
Example 1
Input:
root = [1,2,3,4,5,6]
Output:
6
Example 2
Input:
root = []
Output:
0
Example 3
Input:
root = [1]
Output:
1
Constraints
- The number of nodes in the tree is in the range
[0, 5 * 104]
. 0 <= Node.val <= 5 * 104
- The tree is guaranteed to be complete.
Approach and Intuition
The problem at hand is to count the nodes in a complete binary tree in less than linear time. Given the structured nature of a complete binary tree, straightforward traversal methods like breadth-first or depth-first (which inherently have O(n)
complexity) are not optimal. Here's a strategic approach to solve this efficiently:
Example Illustrations
Example 1:
Input: root = [1,2,3,4,5,6]
Output: 6
This input describes a complete binary tree with three levels. All the levels are fully filled, hence a direct count gives six nodes.
Example 2:
Input: root = []
Output: 0
This represents an empty tree. Consequently, the number of nodes is zero.
Example 3:
Input: root = [1]
Output: 1
This is a tree with just one node, which forms the root and thus there is only one node.
Intuitive Steps
- Check if the tree is empty, which directly yields a count of zero.
- Utilize the properties of the complete binary tree to determine tree height quickly by traversing left-child pointers until reaching a null.
- For counting the nodes especially in the last level:
- Apply binary search on the last level to determine how many nodes it contains. Convert potential node positions to their equivalent paths and verify these paths exist in the tree.
- Sum the nodes from all completely filled levels (which is straightforward to calculate using the height) with the node count obtained from the last level.
This approach efficiently narrows down the search space and leverages the structured nature of complete binary trees, which avoids unnecessary processing of each node.
Constraints Implications
- Since node values and array size are significantly large, optimizations beyond basic traversal are necessary to achieve sub-linear performance.
- The constraint that tree architecture must be complete simplifies certain assumptions and focuses efforts on counting terminal nodes smartly using binary search methodologies within the last level.
Solutions
- Java
- Python
class Solution {
// Calculate depth of tree
public int findDepth(TreeNode node) {
int depth = 0;
while (node.left != null) {
node = node.left;
depth++;
}
return depth;
}
// Verify if node at index exists
public boolean checkExistence(int index, int depth, TreeNode node) {
int low = 0, high = (int)Math.pow(2, depth) - 1;
int mid;
for (int level = 0; level < depth; level++) {
mid = low + (high - low) / 2;
if (index <= mid) {
node = node.left;
high = mid;
} else {
node = node.right;
low = mid + 1;
}
}
return node != null;
}
public int countNodes(TreeNode rootNode) {
if (rootNode == null) return 0;
int treeDepth = findDepth(rootNode);
if (treeDepth == 0) return 1;
int leftBound = 1, rightBound = (int)Math.pow(2, treeDepth) - 1;
int median;
while (leftBound <= rightBound) {
median = leftBound + (rightBound - leftBound) / 2;
if (checkExistence(median, treeDepth, rootNode)) leftBound = median + 1;
else rightBound = median - 1;
}
// Return total nodes calculated
return (int)Math.pow(2, treeDepth) - 1 + leftBound;
}
}
The provided Java code defines a solution to count the number of nodes in a complete binary tree. The implementation includes split functions to optimize the node count process through binary search, avoiding the need to traverse every node directly.
depth calculation: The
findDepth
function measures the depth of the tree by traversing from the root node to the deepest leftmost node. This ensures logarithmic time complexity relative to the height of the tree, which is optimal for balanced trees.existence check: The
checkExistence
function determines whether a node exists at a specified index for a given depth. Utilizing binary search on the tree level, it strategically navigates right or left based upon the mid-point calculations, thereby facilitating direct access to potential nodes without exhaustive visiting.counting nodes: The main method,
countNodes
, utilizes the aforementioned helpers to efficiently count nodes. Initially, it handles the edge case of a null tree by immediately returning zero. Using the depth obtained fromfindDepth
, it sets up a boundary for a binary search. The search narrows down the bounds to find the first nonexistent node index in the last level of the tree, therefore determining the count of existing nodes in less traversed paths.
This solution leverages logarithmic depth traversal combined with binary search over the last level of the tree, both contributing to significant performance gains particularly for large data sets where direct traversal would be computationally expensive. This method ensures an efficient and precise count of nodes in a complete binary tree.
class Solution:
def tree_depth(self, node: TreeNode) -> int:
depth = 0
while node.left:
node = node.left
depth += 1
return depth
def node_exists(self, index: int, depth: int, node: TreeNode) -> bool:
low, high = 0, 2**depth - 1
for _ in range(depth):
mid = low + (high - low) // 2
if index <= mid:
node = node.left
high = mid
else:
node = node.right
low = mid + 1
return node is not None
def countNodes(self, root: TreeNode) -> int:
if not root:
return 0
depth = self.tree_depth(root)
if depth == 0:
return 1
low, high = 1, 2**depth - 1
while low <= high:
mid = low + (high - low) // 2
if self.node_exists(mid, depth, root):
low = mid + 1
else:
high = mid - 1
return (2**depth - 1) + low
The given Python 3 code efficiently solves the problem of counting the total number of nodes in a complete binary tree. The structure of the code comprises a main class Solution
with three methods: tree_depth
, node_exists
, and countNodes
.
tree_depth(node)
: This method calculates the depth of the tree by traversing down the left child from the root until no left child is present. It returns the number of levels in the tree excluding the root level.node_exists(index, depth, node)
: This method determines whether a node exists at a specific index within the last level of the tree. It performs a binary search in the range of possible node indices at the deepest level, adjusting the search range based on whether the middle index node is found to be present or not.countNodes(root)
: This is the main method where:- The tree's depth is computed.
- If the tree has no depth (meaning only the root node exists or no nodes at all), an appropriate count is returned.
- If the tree has more depth, it performs a binary search on the last level to find the last node index, using the logic defined in the
node_exists
method. - The total number of nodes in the tree is then calculated by adding up the nodes from all levels except the last, with those identified in the last level of the tree.
This implementation is efficient because it optimizes the process of counting nodes in a complete binary tree through the use of binary search principles, reducing the potential overhead of a naive approach that would involve checking every node. This ensures high performance, especially pertinent for trees with a large number of levels.
No comments yet.