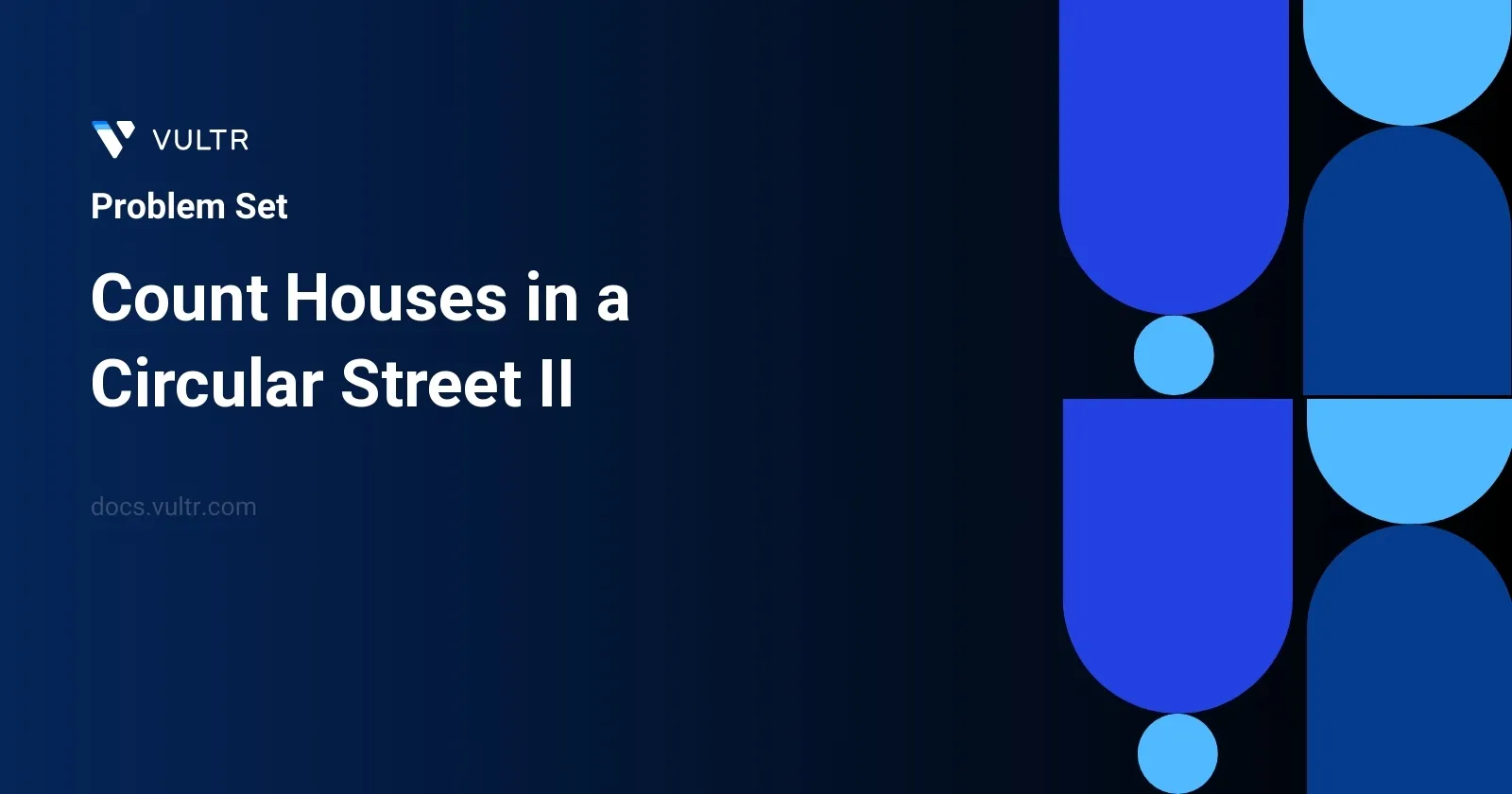
Problem Statement
You are provided with a street
object from a class named Street
, which models a circular street containing a series of houses, each house having a door which might be initially open or closed. Your role is to find out how many houses are present on this street. The maximum possible number of houses (k
) is known and is always greater than or equal to the actual count of the houses.
The Street
class offers three methods:
closeDoor()
- Closes the door of the house you are currently facing.isDoorOpen()
- Checks if the door of the house you are facing is open, returning true if it's open and false if it's closed.moveRight()
- Moves your position to the next house to the right on this circular street.
Given the circular nature of the street, moving to the right from the last house will bring you back to the first house. Using these class methods, you need to determine and return the total number of houses on the street.
Examples
Example 1
Input:
street = [1,1,1,1], k = 10
Output:
4
Explanation:
There are 4 houses, and all their doors are open. The number of houses is less than k, which is 10.
Example 2
Input:
street = [1,0,1,1,0], k = 5
Output:
5
Explanation:
There are 5 houses, and the doors of the 1st, 3rd, and 4th house (moving in the right direction) are open, and the rest are closed. The number of houses is equal to k, which is 5.
Constraints
n == number of houses
1 <= n <= k <= 105
street
is circular by definition provided in the statement.- The input is generated such that at least one of the doors is open.
Approach and Intuition
- Stand in front of the first house (you start at a house with an open door).
- Close the door of this house to mark it as visited.
- Move to the next house to the right.
- Continue moving to the right and closing doors until:
- You encounter a closed door, which indicates you have returned to the starting house.
- Count each house as you visit to get the total number of distinct houses.
By applying this traversal and marking strategy using the method closeDoor()
, you exploit the openness of at least one door (as guaranteed by the problem constraints) to differentiate unvisited versus revisited houses. This method ensures:
- Each house is counted exactly once.
- You stop counting when you return to the initially visited and closed house, exploiting the circular structure effectively.
This approach is efficient given the constraint that n <= k <= 105
, ensuring the process of traversing the street and counting houses completes in a reasonable time frame even at maximum limits.
Solutions
- Java
- Python
class Solution {
public int countOpenHouses(Street mainStreet, int maxSteps) {
// Index of the first open door
int firstOpenIdx = 0;
// Distance from first open door to another open door
int distance = 0;
// Current walk index
int stepIndex = 0;
// Found the first open door
boolean firstFound = false;
while (stepIndex <= 2 * maxSteps) {
// Check if the current door is open
if (mainStreet.isDoorOpen()) {
if (firstFound) {
distance = stepIndex - firstOpenIdx;
mainStreet.closeDoor();
} else {
firstOpenIdx = stepIndex;
firstFound = true;
}
}
mainStreet.moveRight();
stepIndex++;
}
return distance;
}
}
This solution involves solving the problem of counting the number of houses in a circular street using Java, focusing on the distance between the first two open houses within a specified range. The countOpenHouses
method within the Solution
class takes parameters mainStreet
, a customized object representing the street (presumably with methods like isDoorOpen()
, closeDoor()
, and moveRight()
), and maxSteps
, an integer indicating the maximum steps that can be considered from the starting point.
Here’s a breakdown of how the functions within the code work:
- Initialize an index
firstOpenIdx
and a distancedistance
, both initially zero, to store the position of the first open house and the distance between the first two open houses found. - Use
stepIndex
to track the number of steps taken, and a boolean flagfirstFound
to check the discovery of the first open house. - Iterate while the condition
stepIndex <= 2 * maxSteps
holds true, effectively ensuring any house within twice the maximum steps can be considered to account for the circular nature of the street. - Within this loop:
- Check if the current door is open using the
isDoorOpen()
method. - If the first open house has been found (i.e.,
firstFound
is true), compute the distance fromfirstOpenIdx
to the currentstepIndex
and then close this currently open door. - If no open house has been located yet, log the current index as
firstOpenIdx
and setfirstFound
to true.
- Check if the current door is open using the
- After walking through the street up to the allowable range, return the calculated
distance
indicating the distance between the first two open houses detected.
This method offers a specific utility in scenarios such as security systems, neighborhood watch efficiency, or even urban planning simulation where understanding the distribution of certain characteristics (like open houses in this case) along a circular path is critical. The function employs straightforward iteration and condition checking, leveraging the properties and methods of the provided mainStreet
object. Ensure to integrate error handling or consider cases where no open houses might be found within the given limits for broader application robustness.
class Solution:
def countHouses(self, street: Optional['Street'], num: int) -> int:
# Track distance to the initial open door found
initial_open_door = 0
# Distance from the current door to the initial open door
distance_from_initial = 0
# Current step in our traversal
current_step = 0
# Indicates if the initial open door has been found
initial_found = False
while current_step <= 2 * num:
# Check if the current door is open
if street.isDoorOpen():
# If the initial door has been encountered before, record distance and close the door
if initial_found:
distance_from_initial = current_step - initial_open_door
street.closeDoor()
# Mark the first open door encountered
else:
initial_open_door = current_step
initial_found = True
# Move to the next house and increment the step counter
street.moveRight()
current_step += 1
return distance_from_initial
In this solution, you're working with a problem of counting the number of houses between the first open door encountered and the next subsequent open door, given a circular street layout implemented in Python. The provided Python class Solution
includes a method countHouses
that takes two parameters: street
, which is an instance of a Street
class, and num
, an integer representing the total number of houses on the street. This method efficiently determines the distance between two open doors on this circular street.
Detailed Steps:
Initialize several variables:
initial_open_door
to store the position of the first open door encountered.distance_from_initial
to store the distance to the next open door encountered after the initial.current_step
to keep track of the current position on the street during traversal.initial_found
as a boolean to note when the first open door has been found.
Implement a while loop that will continue until
current_step
exceeds twice the value ofnum
to ensure a complete scanning of the street considering its circular nature.Inside the loop:
- Check if the current door is open using
street.isDoorOpen()
. - If an open door is found and
initial_found
isTrue
, updatedistance_from_initial
to the difference betweencurrent_step
andinitial_open_door
and then invokestreet.closeDoor()
. - If it's the first open door found (i.e.,
initial_found
isFalse
), updateinitial_open_door
withcurrent_step
, and setinitial_found
toTrue
.
- Check if the current door is open using
Move to the next house using
street.moveRight()
and incrementcurrent_step
by 1.The function returns
distance_from_initial
, providing the distance between the first and the next open door encountered in the traversal.
This solution effectively leverages a single traversal to compute the desired distance, considering the circular nature of the street by allowing a loop around it up to twice the total number of doors. This approach ensures that the method will handle edge cases such as when the first open door is towards the very end of the list and the next one is towards the beginning.
No comments yet.