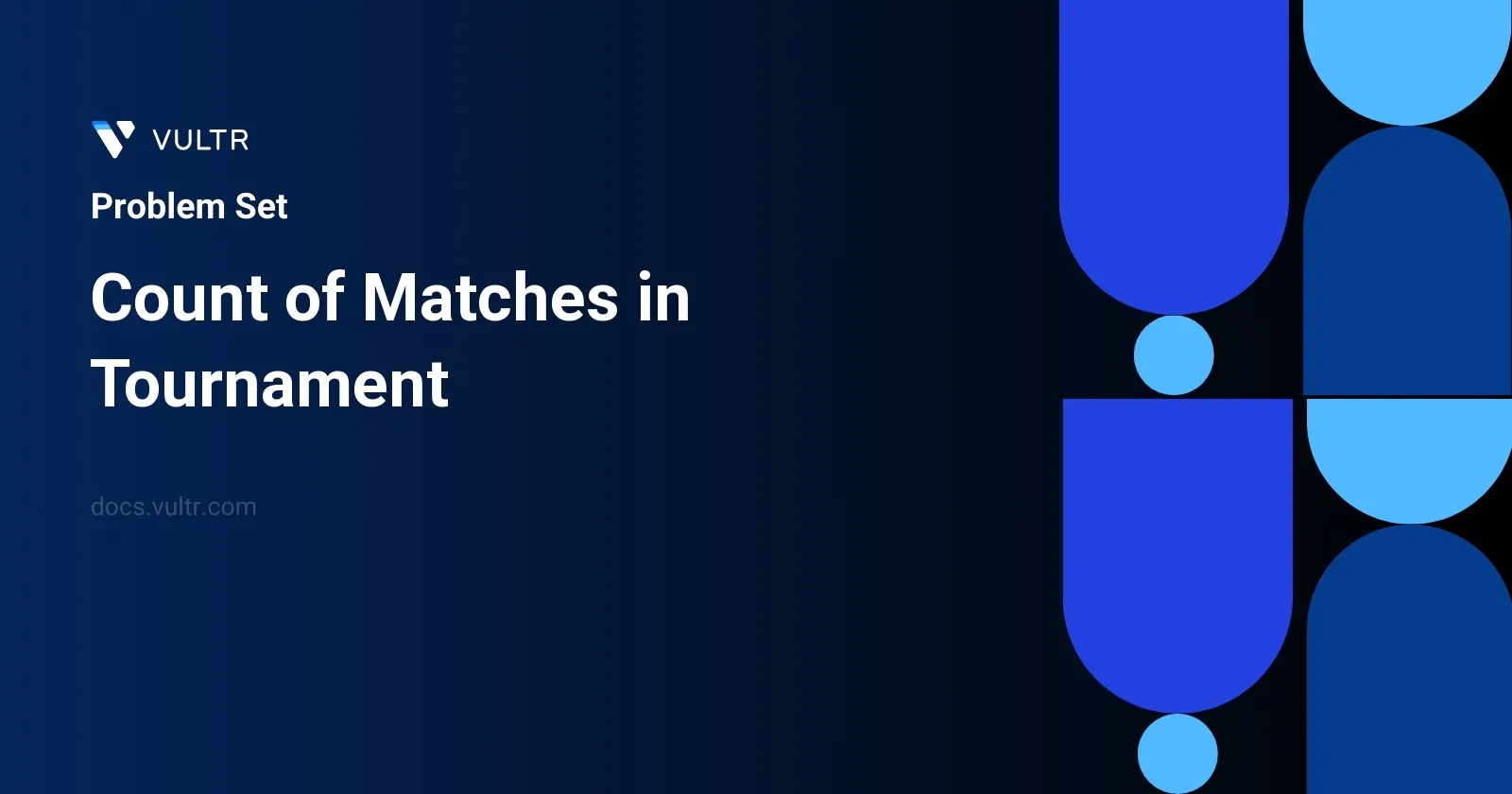
Problem Statement
In this challenge, you are provided with an integer n
, representing the number of teams participating in a unique tournament. The tournament follows a set of specific rules based on the parity (oddness or evenness) of the teams:
- For even numbers of teams: Each team is paired with another, resulting in
n / 2
matches. Consequently,n / 2
teams progress to the next stage. - For odd numbers of teams: One team randomly skips to the next round without competing, while the remaining teams are paired. This results in
(n - 1) / 2
matches, and(n - 1) / 2 + 1
teams moving to the next round.
Your task is to determine how many total matches are played by the time a single winner is determined in the tournament.
Examples
Example 1
Input:
n = 7
Output:
6
Explanation:
Details of the tournament: - 1st Round: Teams = 7, Matches = 3, and 4 teams advance. - 2nd Round: Teams = 4, Matches = 2, and 2 teams advance. - 3rd Round: Teams = 2, Matches = 1, and 1 team is declared the winner. Total number of matches = 3 + 2 + 1 = 6.
Example 2
Input:
n = 14
Output:
13
Explanation:
Details of the tournament: - 1st Round: Teams = 14, Matches = 7, and 7 teams advance. - 2nd Round: Teams = 7, Matches = 3, and 4 teams advance. - 3rd Round: Teams = 4, Matches = 2, and 2 teams advance. - 4th Round: Teams = 2, Matches = 1, and 1 team is declared the winner. Total number of matches = 7 + 3 + 2 + 1 = 13.
Constraints
1 <= n <= 200
Approach and Intuition
Given the mechanics of the tournament, the key observation is that in each round, the total number of teams decreases until only one champion remains. The main insight is that teams are effectively halved in each round, making the tournament's progression logarithmic in nature relative to the initial number of teams.
Let's decompose the process using the provided examples:
Analyzing Example Scenarios:
Example 1 (
n = 7
teams)- Round 1: 7 teams (odd) compete — 3 matches occur, and 4 teams advance.
- Round 2: Now with 4 teams (even) — 2 matches occur, and 2 teams advance.
- Round 3: Finally, 2 teams (even) compete — 1 match occurs, declaring the winner.
- Total matches = 3 (Round 1) + 2 (Round 2) + 1 (Round 3) = 6 matches.
Example 2 (
n = 14
teams)- Round 1: 14 teams (even) compete — 7 matches occur, with 7 teams advancing.
- Round 2: 7 teams (odd) continue — 3 matches occur, and 4 teams advance.
- Round 3: With 4 teams (even) left — 2 matches occur, leaving 2 teams.
- Round 4: The final 2 teams compete — 1 match occurs to find the winner.
- Total matches = 7 (Round 1) + 3 (Round 2) + 2 (Round 3) + 1 (Round 4) = 13 matches.
From these examples, we observe that:
- Each reduction in the number of teams is accounted as a match played.
- The sequence of matches directly correlates to progressively eliminating half the teams, rounded up when the count is odd.
This systematic elimination approach reflects the efficient and predictable operation to determine the total number of matches required to find a tournament winner solely based on the initial count of participating teams.
Solutions
- C++
- Java
- Python
class Solution {
public:
int countMatches(int teams) {
return teams - 1;
}
};
The given solution in C++ is designed to determine the number of matches played in a tournament where every game eliminates one team. The process of elimination continues until only one team remains, implying a tournament structured in a knockout format.
In this approach:
- Define a class called
Solution
. - Implement the
countMatches
function which accepts an integerteams
, representing the number of teams participating in the tournament. - The function simply calculates the total number of matches by subtracting 1 from the total number of teams. This is based on the principle that for
n
teams,n-1
matches are needed for there to be one winner, as each match eliminates exactly one team.
Assuming this represents a simple and direct calculation specific to the problem of finding the number of matches needed in a knockout tournament, the code is remarkably efficient with a constant time complexity O(1), as it directly returns the result based on the input without any iterative or recursive operations.
This method correctly handles tournaments of any size, assuming a minimum of 2 teams are needed for a match to occur.
class Solution {
public int totalMatches(int teams) {
return teams - 1;
}
}
In the given Java solution for calculating the count of matches in a tournament, the method totalMatches(int teams)
efficiently calculates the total number of matches required to determine a winner in a knockout tournament format. The solution leverages a simple mathematical principle: in a knockout tournament where each match eliminates one team, the total number of matches played is always one less than the number of teams. Thus, the function returns teams - 1
. This approach ensures that the method executes in constant time, O(1), with immediate calculation and return of the result, guaranteeing optimal performance for any number of teams.
class Solution:
def totalMatches(self, teams: int) -> int:
return teams - 1
Problem Title: Count of Matches in Tournament
Language: Python3
Code Explanation:
The code defines a method totalMatches
within the Solution
class that calculates the number of matches played in a knockout tournament where there is one winner and every match eliminates one team. The method accepts one parameter, teams
, which represents the total number of teams participating in the tournament. To determine the number of matches required for such a tournament, subtract one from the total number of teams. This logic is based on the principle that every match results in the elimination of one team, hence to find a single winner, the number of matches that need to take place is exactly one less than the number of participating teams.
Example:
- If the input for
teams
is 4, the method will return 3. This is because three matches are needed to determine a winner from four teams (first round: two matches, second round: one match).
Why this works:
Every match in a knockout style tournament directly results in one team being eliminated, reducing the number of teams that proceed to the next round. Continue this until only one team, the winner, remains. Thus, for N
teams, N-1
matches are necessary and sufficient to conclude the tournament with a definitive winner.
No comments yet.