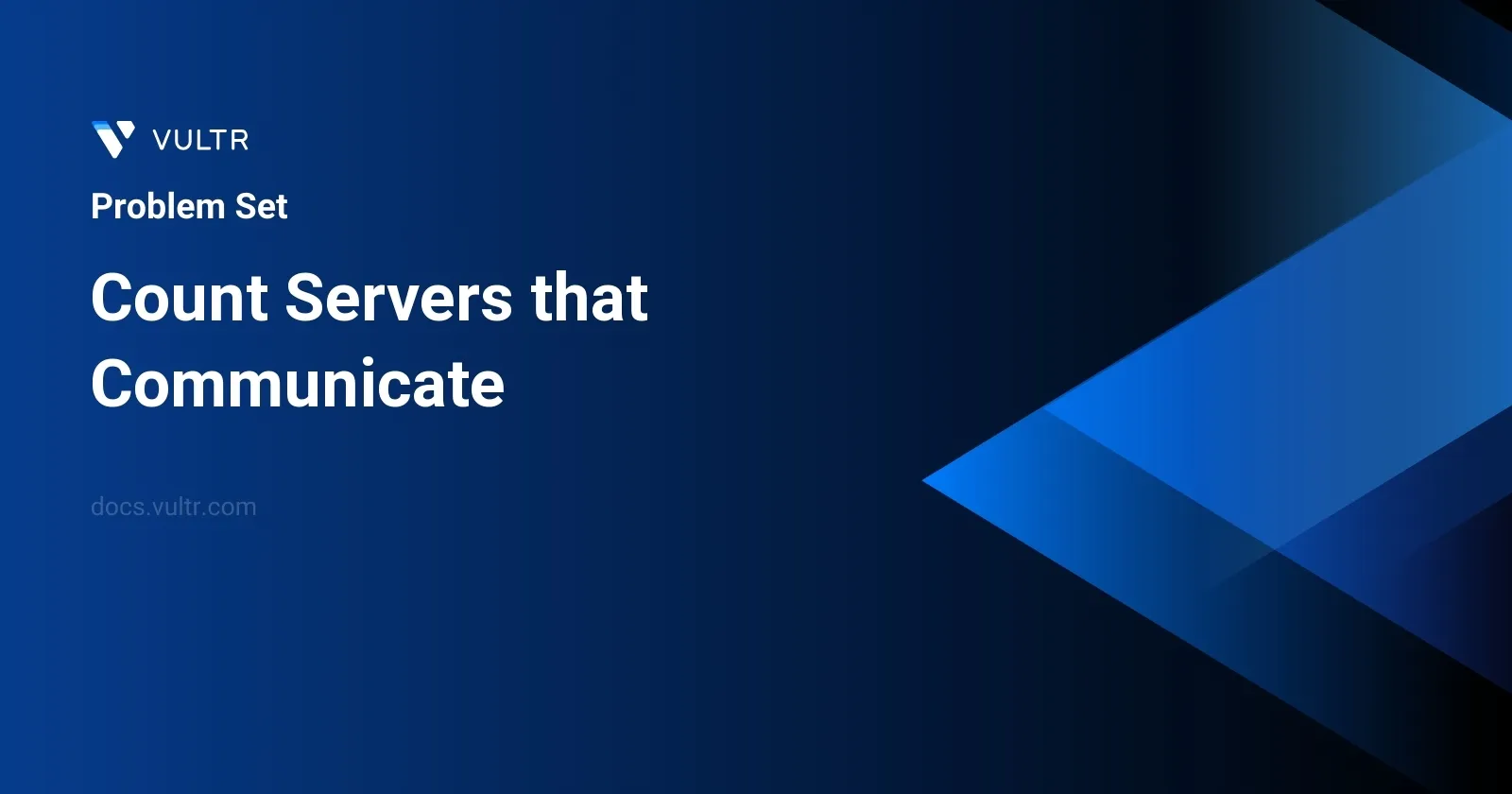
Problem Statement
In a given server center which can be visualized as a matrix (where each cell can either have a server denoted by 1
or nothing denoted by 0
), two servers are considered to be able to communicate if they are positioned in the same row or the same column. The challenge posed is to determine how many servers are capable of such communication given this matrix setup. The end goal is to return the count of servers that are not isolated;
i.e., those that can communicate with at least one other server. This scenario is modeled by a m * n
integer matrix referred to as grid
.
Examples
Example 1
Input:
grid = [[1,0],[0,1]]
Output:
0
Explanation:
No servers can communicate with others.
Example 2
Input:
grid = [[1,0],[1,1]]
Output:
3
Explanation:
All three servers can communicate with at least one other server.
Example 3
Input:
grid = [[1,1,0,0],[0,0,1,0],[0,0,1,0],[0,0,0,1]]
Output:
4
Explanation:
The two servers in the first row can communicate with each other. The two servers in the third column can communicate with each other. The server at right bottom corner can't communicate with any other server.
Constraints
m == grid.length
n == grid[i].length
1 <= m <= 250
1 <= n <= 250
grid[i][j] == 0 or 1
Approach and Intuition
The task at hand involves identifying servers that are capable of "communicating" or connecting with one another based on their positioning within the same row or column. To approach this problem efficiently, it can be broken down into several clear steps:
Initialize Counters for Rows and Columns:
- Initialize two arrays (or hash maps) to count servers in each row and in each column. Let these be
rowCount[]
andcolCount[]
.
- Initialize two arrays (or hash maps) to count servers in each row and in each column. Let these be
First Sweep - Count Servers:
- Traverse the entire grid. For each cell containing a server (
grid[i][j] == 1
):- Increment the respective counters
rowCount[i]
andcolCount[j]
. - This initial sweep allows us to record the total number of servers in each row and column.
- Increment the respective counters
- Traverse the entire grid. For each cell containing a server (
Second Sweep - Identify Communicating Servers:
- Traverse the grid a second time. For each server (
grid[i][j] == 1
), check the counters:- If
rowCount[i] > 1
orcolCount[j] > 1
, it implies that this server is not isolated and can communicate with another server in its row or column. - Tally up such servers to get the total count of communicating servers.
- If
- Traverse the grid a second time. For each server (
The efficiency of this approach is supported by its O(m*n) time complexity, where m
and n
are the dimensions of the grid. This is because each server is processed twice: once for counting and once for verifying communication possibilities. This method ensures that even larger grids can be handled within a reasonable time frame.
By using row and column counters, the solution is both intuitive and efficient, allowing us to solve the problem with just two full scans of the grid. This approach takes full advantage of the constraints given, particularly the binary nature of the grid (only 0
s and 1
s) and the upper limit on grid dimensions (250x250
).
Solutions
- C++
- Java
- Python
class Solution {
public:
int serverCounter(vector<vector<int>>& matrix) {
int row = matrix.size(), col = matrix[0].size();
int countCommServers = 0;
for (int i = 0; i < row; ++i) {
int serverCount in Row = 0;
int firstServerCol = -1;
// Finding servers in the current row
for (int j = 0; j < col; ++j) {
if (matrix[i][j]) {
if (serverCountInRow == 0) {
firstServerCol = j;
}
serverCountInRow += 1;
}
}
// Determine if servers in this row can communicate
bool canTalk = (serverCountInRow > 1);
// Checking for communication possibility for a lonely server
if (!canTalk) {
for (int k = 0; k < row; ++k) {
if (k != i && matrix[k][firstServerCol]) {
canTalk = true;
break;
}
}
}
// Add to total if communication is possible
if (canTalk) {
countCommServers += serverCountInRow;
}
}
return countCommServers;
}
};
The provided C++ solution addresses the problem of counting servers that can communicate in a grid. The grid is represented as a 2D vector where each element can be either 0 (no server) or 1 (server present). The function serverCounter
expects this grid as input and returns the number of servers that are able to communicate.
Here's a breakdown of the steps involved in the implementation:
Initialize variables:
row
andcol
are initialized to store the dimensions of the matrix.countCommServers
is initialized to keep track of the count of communicating servers.
Iterate through each row of the matrix:
- For each row, maintain a count of servers (
serverCountInRow
) and the column index of the first server found (firstServerCol
).
- For each row, maintain a count of servers (
Check within row:
- Traverse each column in the current row to count how many servers are present and note the position of the first server.
Assess communication ability:
- After counting, determine if servers within the same row can communicate based on whether there are more than one server in that row (
serverCountInRow > 1
). - If a single server is found in a row, check other rows to see if there's another server in the same column, allowing cross-row communication.
- After counting, determine if servers within the same row can communicate based on whether there are more than one server in that row (
Update communicating servers count:
- If communication is established either within the same row or across rows, add the count of servers in that row to
countCommServers
.
- If communication is established either within the same row or across rows, add the count of servers in that row to
Return result:
- Finally, the function returns the count of servers that can communicate.
This solution effectively uses nested loops and a logical determination of communication paths to solve the problem efficiently within the constraints of the grid matrix.
class Solution {
public int calculateCommunicatingServers(int[][] networkGrid) {
int totalRows = networkGrid.length, totalColumns = networkGrid[0].length;
int countOfCommunicableServers = 0;
for (int row = 0; row < totalRows; ++row) {
int numServersInRow = 0;
int lastFoundServerColumn = -1;
// Count servers in this row and note the column of the first server found
for (int col = 0; col < totalColumns; ++col) {
if (networkGrid[row][col] == 1) {
if (numServersInRow == 0) {
lastFoundServerColumn = col;
}
numServersInRow++;
}
}
// Determine if the current row of servers can communicate
boolean isCommunicableRow = (numServersInRow != 1);
// If row has one server, check other rows for any server in the same column
if (!isCommunicableRow) {
for (int otherRow = 0; otherRow < totalRows; ++otherRow) {
if (otherRow != row && networkGrid[otherRow][lastFoundServerColumn] == 1) {
isCommunicableRow = true;
break;
}
}
}
// Add to communicable servers if this row's servers can communicate
if (isCommunicableRow) {
countOfCommunicableServers += numServersInRow;
}
}
return countOfCommunicableServers;
}
}
This Java solution tackles the problem of identifying servers that can communicate in a grid (represented as a 2D array) where each cell with a value of 1 represents a server.
- The method
calculateCommunicatingServers
receives a 2D integer array namednetworkGrid
as input. - Two integer variables,
totalRows
andtotalColumns
, store the total number of rows and columns innetworkGrid
respectively. - Another integer,
countOfCommunicableServers
, initiated to zero, is used to keep track of the total number of servers that can communicate. - The solution iterates over each row and determines how many servers (
numServersInRow
) it contains and records the column index of the last found server (lastFoundServerColumn
). - A server is considered communicable if it shares its row or its column with at least one other server.
- For each row:
- If only one server is found in that row, the code further checks other rows to determine if any server resides in the same column (
lastFoundServerColumn
). - If communicability condition is satisfied either through row or column sharing, the number of servers in that row is added to
countOfCommunicableServers
.
- If only one server is found in that row, the code further checks other rows to determine if any server resides in the same column (
- Finally, the method returns the total count of servers that can communicate.
This approach efficiently captures the communicability of servers by exploiting grid traversal and condition checks to ensure that every server that can communicate is counted accurately.
class Solution:
def calculateCommServers(self, grid: List[List[int]]) -> int:
row_count = len(grid)
col_count = len(grid[0])
comm_servers = 0
for row_index in range(row_count):
num_servers = 0
col_track = -1
# Find servers in the current row and track the first server's index
for col_index in range(col_count):
if grid[row_index][col_index] == 1:
if num_servers == 0:
col_track = col_index
num_servers += 1
# Determine if servers in this row can communicate
communicates = (num_servers != 1)
# Check for communication from other rows in the same column
if not communicates:
for i in range(row_count):
if i != row_index and grid[i][col_track] == 1:
communicates = True
break
# Increment count based on communication ability
if communicates:
comm_servers += num_servers
return comm_servers
The given Python program defines a method to count the number of servers that can communicate among themselves within a same row or column of a grid. The grid is represented by a 2D list, where 1
represents a server and 0
represents an empty space.
Here's a breakdown of the solution's approach:
Initialize counter variables for the number of rows and columns, as well as for the total communicating servers.
Iterate through each row. For each row:
- Initialize an internal counter to track the number of servers in that row and a variable to keep a reference to the first server's column index (if any).
- Traverse through the columns of that row to count servers and record the index of the first server found.
After counting, check if there is more than one server in the current row. This indicates potential communication within the row.
If only one server is found in a row, check other rows for a server in the same column—this establishes vertical communication possibilities.
If communication (either horizontal within the row, or vertical across rows from the same column) is verified, add the number of servers in the current row to the total count of communicating servers.
Return the count of communicating servers.
This solution efficiently checks each server's communication ability by only considering relevant rows and columns and increases the counter upon confirming communication capability. This minimizes unnecessary computations and enhances performance, especially for larger grids.
No comments yet.