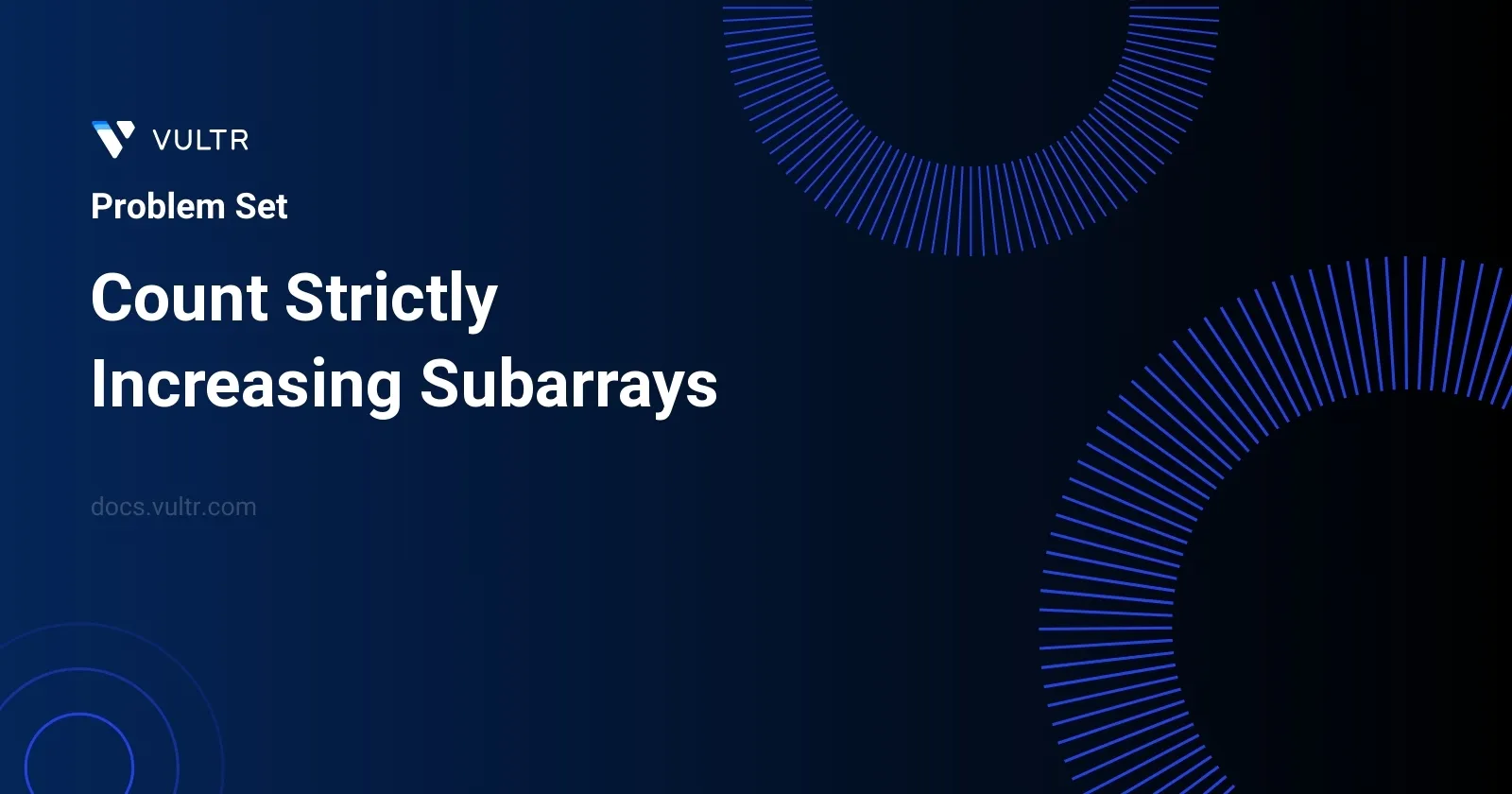
Problem Statement
In this task, we are given an array of positive integers named nums
. Our goal is to determine the total number of contiguous subarrays within nums
that exhibit a strictly increasing sequence. Each element of the array nums
participates in the formation of subarrays, where a subarray is defined as a continuous slice of the original array.
Examples
Example 1
Input:
nums = [1,3,5,4,4,6]
Output:
10
Explanation:
The strictly increasing subarrays are the following: - Subarrays of length 1: [1], [3], [5], [4], [4], [6]. - Subarrays of length 2: [1,3], [3,5], [4,6]. - Subarrays of length 3: [1,3,5]. The total number of subarrays is 6 + 3 + 1 = 10.
Example 2
Input:
nums = [1,2,3,4,5]
Output:
15
Explanation:
Every subarray is strictly increasing. There are 15 possible subarrays that we can take.
Constraints
1 <= nums.length <= 105
1 <= nums[i] <= 106
Approach and Intuition
To solve the problem of counting strictly increasing subarrays, let's delve into the intuition and the approach based on given examples and constraints:
Detailed Steps
Understanding Edge Cases: Consider small cases like
[1]
,[1, 2]
and[1, 2, 1, 3]
. Each offers insight on how to handle single elements (always a valid subarray), pairs (valid if in increasing order), and the reset of count when the sequence isn't strictly increasing.Initial Observations from Examples:
- Example 1:
{1, 3, 5, 4, 4, 6}
illustrates a reset in increasing pattern at elements5
and4
, and again at4
and4
. This calls for a mechanism to continuously check and reset when the sequence breaks. - Example 2:
{1, 2, 3, 4, 5}
being entirely strictly increasing, provides the sum of all possible subarrays, which is the maximum possibility for an array of its nature.
- Example 1:
Functionality to Catch Subarrays:
- Start with each element considering it as a potential beginning of a new subarray.
- Move forward and count as long as the next element is greater than the current one.
- When the sequence breaks (or ends), sum up all possible subarrays starting from the first element of the current strictly increasing sequence. This could be calculated using the formula for the sum of first
n
natural numbers, wheren
is the length of the current sequence (because every element can be the start of a new subarray).
Edge Case Handlings:
- Single element arrays always have exactly one increasing subarray (the element itself).
- Arrays of length two require a simple comparison.
Given the constraints (1 <= nums.length <= 105
and 1 <= nums[i] <= 106
), the solution needs to efficiently handle large inputs, making the approach of continuously checking and summing subarrays optimal without necessitating a double loop to explicitly evaluate every possible subarray.
Solutions
- C++
- Java
class Solution {
public:
long long countIncreasingSubsequences(vector<int>& elements) {
long long totalSubsequences = 0;
for (int index = 0; index < elements.size(); index++) {
long lengthOfSubsequence = 1;
while (index + 1 < elements.size() && elements[index] < elements[index + 1]) {
lengthOfSubsequence++;
index++;
}
totalSubsequences += (lengthOfSubsequence * (lengthOfSubsequence + 1)) / 2;
}
return totalSubsequences;
}
};
The given C++ solution provides a method to count the number of strictly increasing subarrays within an array of integers. This solution is encapsulated within a class named Solution
, which features a public method countIncreasingSubsequences
taking a vector of integers as its argument.
The method implements an efficient approach using a single scan of the input array:
- Initialize a
long long
variabletotalSubsequences
to store the result. - Loop through each index
index
of the arrayelements
. - For each element, calculate the length of the strictly increasing subsequence starting from this index.
- As long as the next element is greater than the current, increment the length and the index.
- The number of subsequences that can be formed with a strictly increasing subsequence of length
n
is given by(n * (n + 1)) / 2
. This formula is derived from the sum of the firstn
natural numbers. - Add the computed number of subsequences to
totalSubsequences
.
The function finally returns totalSubsequences
, representing the total count of strictly increasing subsequences within the input array. Remember, the return value captures possible large results using a long long
integer to prevent overflow issues associated with large input sizes.
class Solution {
public long countSubarrays(int[] array) {
long totalSubarrays = 0;
for (int index = 0; index < array.length; index++) {
long currentLength = 1;
while (index + 1 < array.length && array[index] < array[index + 1]) {
currentLength++;
index++;
}
totalSubarrays += (currentLength * (currentLength + 1)) / 2;
}
return totalSubarrays;
}
}
This solution aims to count the number of strictly increasing subarrays within a given array in Java. The primary approach taken involves iterating through the array while continuously checking for sequences where subsequent elements are strictly greater than their predecessors.
Here's how the solution works:
- Start by initializing a variable
totalSubarrays
to zero, which will track the count of all valid subarrays. - Iterate through each element of the array using a for loop.
- For each element, initialize a
currentLength
variable to 1 to represent the minimum length of any subarray (an element itself). - Use a nested while loop to check if the current element is less than its next element. If it is:
- Increment the
currentLength
which tracks the length of the current strictly increasing sequence. - Also increment the
index
to avoid rechecking the same elements.
- Increment the
- After exiting the while loop, calculate the number of subarrays that the identified sequence can form using the formula ( \text{currentLength} \times (\text{currentLength} + 1) / 2 ), and add this value to
totalSubarrays
. - Return the
totalSubarrays
as the result which represents the total count of strict increasing subarrays.
Key considerations:
- The use of a single loop with a nested while ensures that each element is part of only one strictly increasing sequence calculation which optimizes the performance.
- The formula ( \text{currentLength} \times (\text{currentLength} + 1) / 2 ) efficiently computes the sum of the first
n
natural numbers, which corresponds to the number of subarrays formed by 'n' consecutive strictly increasing numbers. - Make sure to handle edge cases such as arrays with no consecutive increasing numbers, very large arrays, or arrays of uniform elements effectively within this framework.
This implementation provides a clear and efficient method to count increasing subarrays, ensuring an optimal solution with a time complexity nearly linear relative to the size of the input array, i.e., ( O(n) ), where n
is the number of elements in the array.
No comments yet.