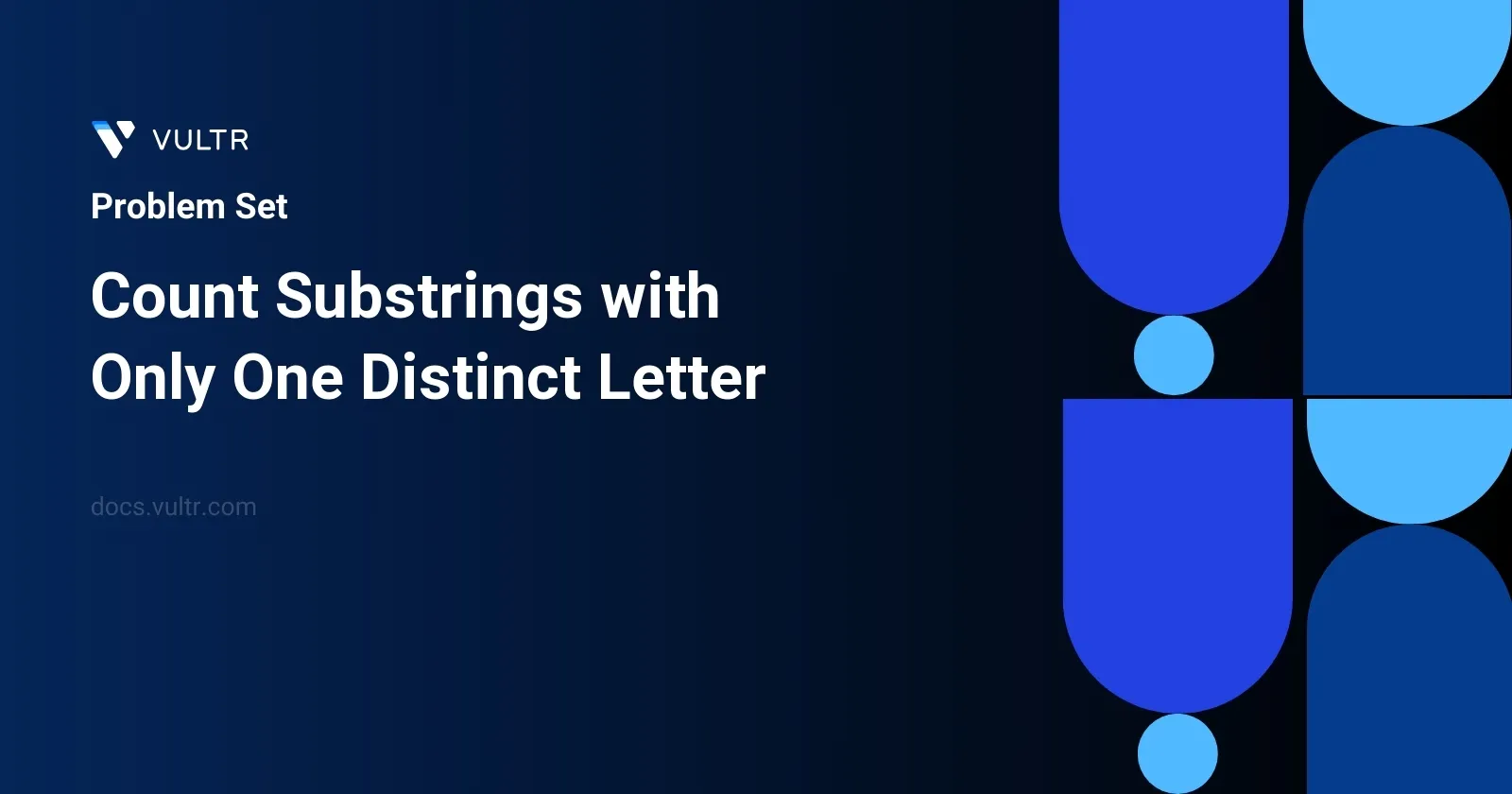
Problem Statement
The challenge presented is to count the total number of substrings within a given string s
such that each substring contains exactly one unique character. This specifically focuses on identifying substrings where all characters are identical. Each substring counts independently based on its occurrence, even if they are of the same set of characters but at different positions or lengths within the main string.
Examples
Example 1
Input:
s = "aaaba"
Output:
8
Explanation:
The substrings with one distinct letter are "aaa", "aa", "a", "b". "aaa" occurs 1 time. "aa" occurs 2 times. "a" occurs 4 times. "b" occurs 1 time. So the answer is 1 + 2 + 4 + 1 = 8.
Example 2
Input:
s = "aaaaaaaaaa"
Output:
55
Constraints
1 <= s.length <= 1000
s[i]
consists of only lowercase English letters.
Approach and Intuition
To solve the problem, it's essential to understand substrings and how we might systematically count these specific kinds.
- Iterate through the string
s
to find continuous blocks (segments) where the character does not change. - For each segment of the same character, calculate the number of possible substrings. If a segment has a length
n
, the number of such substrings can be computed as the sum of the firstn
natural numbers (which isn*(n+1)/2
). - Accumulate the counts for each character segment to get the total number of substrings for
s
.
Breakdown
- Initialization: Start with a total count of 0.
- Traverse the string: As you iterate, if a character is the same as the previous one, extend the current segment. If not, calculate possible substrings from the previous segment and reset counters for the new segment.
- Character Segment Calculation: For a sequence of character length,
l
, use the formulal*(l+1)/2
to add to the total count. - Edge Case Handling: Ensure to calculate the contribution of the last segment after the loop because it might not trigger a calculation in the loop.
Illustration
Using example 2 where s = "aaaaaaaaaa"
:
- The entire string is a single segment of length 10.
- Calculate the substrings for this segment: [ \frac{10 \times (10 + 1)}{2} = 55 ]
- The result of 55 shows the complete substrings count for 10 consecutive 'a's.
Solutions
- Java
- Python
class Solution {
public int sumOfRepeatingChars(String str) {
int sum = 1, consecutive = 1;
for (int index = 1; index < str.length(); index++) {
if (str.charAt(index) == str.charAt(index - 1)) {
consecutive++;
} else {
consecutive = 1;
}
sum += consecutive;
}
return sum;
}
}
The given Java program is designed to calculate the number of substrings where all characters are the same in a given string. It achieves this by iterating through the string and counting the length of consecutive characters that are identical. Each time it encounters a character that matches the previous character, it increases the count of consecutive characters. Otherwise, it resets this count to one. The sum of these counts over the entire string gives the total number of such substrings. The method sumOfRepeatingChars
returns this sum. Here's how the code implements this:
- Initialize two integer variables,
sum
andconsecutive
, to 1.sum
holds the cumulative number of valid substrings, andconsecutive
tracks the length of the current sequence of identical characters. - Iterate through the string starting from the second character.
- For each character, check if it matches the previous character. If it does, increment the
consecutive
variable. If not, resetconsecutive
to 1. - Add the current value of
consecutive
tosum
with each iteration. - After the loop completes, return the value of
sum
, which is the total count of substrings with identical consecutive characters.
class Solution:
def computeTotalLetters(self, string: str) -> int:
overallSum = 1
consecutive = 1
for index in range(1, len(string)):
if string[index] == string[index - 1]:
consecutive += 1
else:
consecutive = 1
overallSum += consecutive
return overallSum
The solution provided is a Python 3 implementation for counting the sum of the lengths of all substrings in a given string that have only one distinct letter. Follow the approach below to understand the solution’s logic:
- The function
computeTotalLetters
takes a single string as an argument. - Initialize
overallSum
to 1, which will keep the count of total substrings with identical letters. Start with 1 to account for the first character trivially being a substring. - Set
consecutive
to 1, which tracks the length of the current consecutive substring with identical letters. - Iterate through the string starting from the second character:
- Compare each character with the previous character.
- If they match, the current character continues a substring of identical characters (
consecutive
increment by 1). - If they differ, reset
consecutive
to 1 as a new set of identical letters starts. - Add the value of
consecutive
tooverallSum
during each iteration; this adds the number of new substrings formed by adding the current letter.
- Once the loop completes, return
overallSum
, which is the total count of all substrings in which all characters are the same.
This function effectively and efficiently calculates the desired total by leveraging the nature of consecutive identical characters in substrings. The use of a simple loop and accumulative counting ensures optimal performance.
No comments yet.