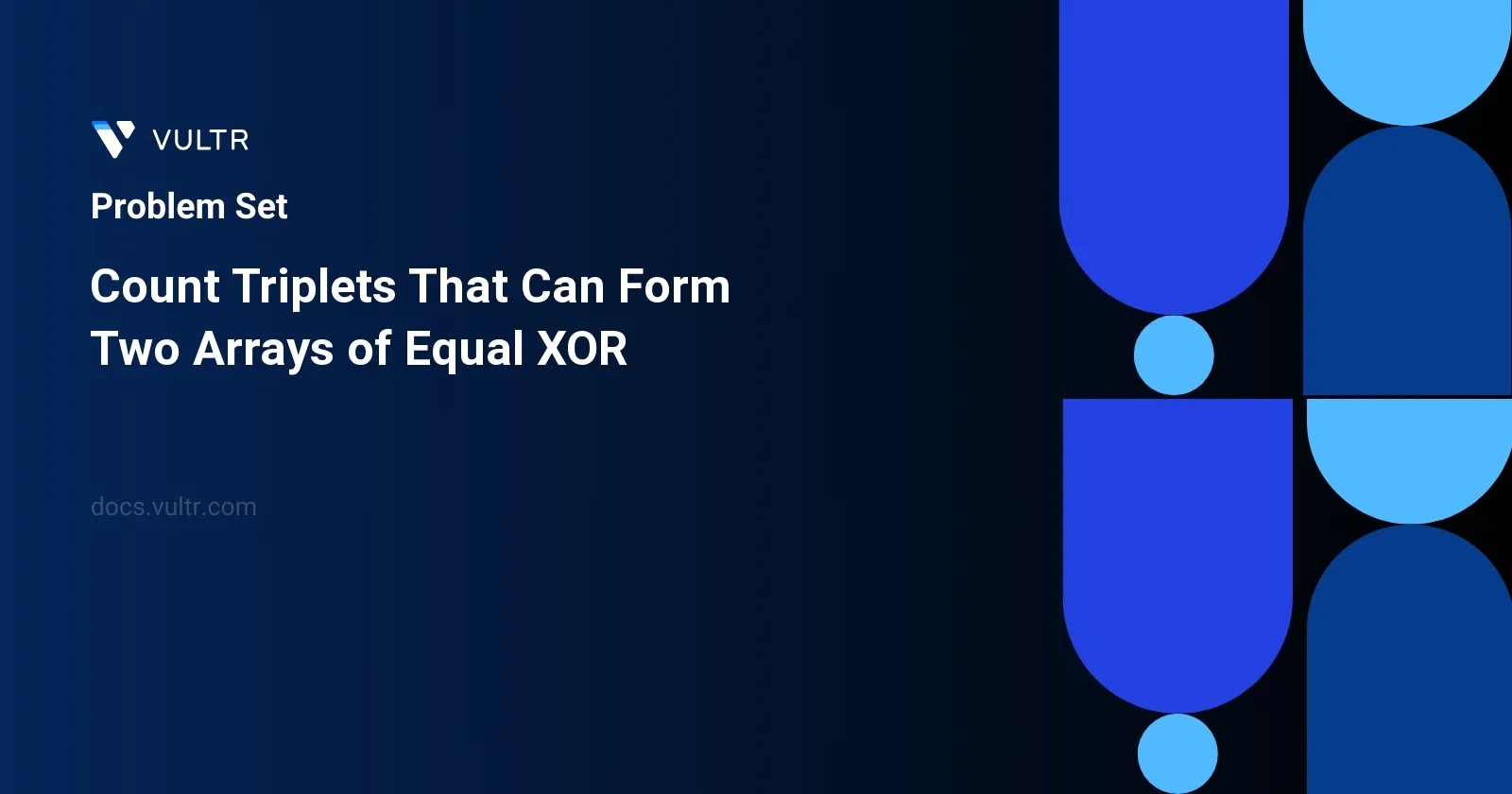
Problem Statement
In this task, we are given an array of integers named arr
. We need to determine the count of specific triplets within this array. These triplets consist of indices (i, j, k)
that satisfy the condition 0 <= i < j <= k < arr.length
. For each triplet, we define two values, a
and b
, based on the bitwise XOR operation (^
). a
is calculated as the XOR of all elements from index i
to j-1
, and b
is derived from the XOR of elements from index j
to k
. The goal is to find the number of such triplets where a
equals b
.
Examples
Example 1
Input:
arr = [2,3,1,6,7]
Output:
4
Explanation:
The triplets are (0,1,2), (0,2,2), (2,3,4) and (2,4,4)
Example 2
Input:
arr = [1,1,1,1,1]
Output:
10
Constraints
1 <= arr.length <= 300
1 <= arr[i] <= 108
Approach and Intuition
The intention is to identify the number of triplets (i, j, k)
such that the XOR from i
to j-1
equals the XOR from j
to k
. A brute-force approach could be to use three nested loops to check each combination, but that would be inefficient with larger arrays. Based on the constraints:
- Understand that XOR is cumulative and associative, which can simplify the computation utilizing a prefix XOR array.
- Construct a prefix XOR where the value at each index
i
is the XOR of all elements from the start of the array up toi
. - A useful property of XOR is that for any segment of the array
l to r
, the XOR can be computed asprefixXOR[r] ^ prefixXOR[l-1]
. - Using the prefixXOR, reduce the complexity of the solution by using only a dual loop. The first loop would fix the end index
k
, and an inner loop would iterate for possible values ofj
. - Each possible
(j, k)
combination suggests a potential indexi
, such that the segmentsi to j-1
andj to k
have the same XOR: effectively, requiringprefixXOR[j-1] == prefixXOR[k]
given the starting index 0. - Use a dictionary or hashmap to efficiently count and quickly look up occurrences of each XOR value at indices, which helps in computing the required triplet count dynamically.
Following this approach helps us overcome the brute-force limitations and exploits properties of the XOR operation to gain efficiency.
Solutions
- C++
- Java
- Python
class Solution {
public:
int countTriplets(vector<int>& numbers) {
int length = numbers.size();
int tripletCount = 0;
int cumulativeXOR = 0;
unordered_map<int, int> xorCountMap = {{0, 1}}, xorIndexSumMap;
for (int index = 0; index < length; ++index) {
cumulativeXOR ^= numbers[index];
tripletCount += xorCountMap[cumulativeXOR]++ * index - xorIndexSumMap[cumulativeXOR];
xorIndexSumMap[cumulativeXOR] += index + 1;
}
return tripletCount;
}
};
The provided C++ solution efficiently counts the number of triplets within an array numbers
, such that two subarrays formed by those triplets have the same XOR. This solution utilizes cumulative XOR calculations to identify equal XOR subarrays efficiently without having to recalculate XOR for every possible subarray.
- Initialize
length
as the size of thenumbers
vector. - Use
tripletCount
to track the count of valid triplets. cumulativeXOR
represents the XOR from the start of the vector up to the current processing index.- Two hash maps,
xorCountMap
andxorIndexSumMap
, are used:xorCountMap
stores how many times a particular XOR result has appeared.xorIndexSumMap
keeps track of the summation of indices where each XOR result has appeared.
- Loop through each element of the
numbers
vector:- Compute cumulative XOR up to the current index.
- If the cumulative XOR has been seen before, calculate potential triplets by subtracting the pre-recorded index sum from
index * count of previous occurrences
. - Update the maps to reflect the current state.
- Finally, return
tripletCount
which now contains the number of valid triplet indices.
This approach avoids the naive method of checking each possible triplet explicitly, thus providing a more optimized solution using hashmap to exploit properties of XOR in constant time operations.
class Solution {
public int findTriplets(int[] data) {
int length = data.length;
int tripletCount = 0;
int xorSum = 0;
// Hashmaps for tracking prefix XOR values and their indices
Map<Integer, Integer> frequencyMap = new HashMap<>();
frequencyMap.put(0, 1);
Map<Integer, Integer> sumMap = new HashMap<>();
// Processing each element in the array
for (int index = 0; index < length; ++index) {
// Current prefix XOR calculation
xorSum ^= data[index];
// Computing the current potential triplets count contribution
tripletCount +=
frequencyMap.getOrDefault(xorSum, 0) * index -
sumMap.getOrDefault(xorSum, 0);
// Updating maps for encountered XOR prefix
sumMap.put(xorSum, sumMap.getOrDefault(xorSum, 0) + index + 1);
frequencyMap.put(xorSum, frequencyMap.getOrDefault(xorSum, 0) + 1);
}
return tripletCount;
}
}
The given problem titled "Count Triplets That Can Form Two Arrays of Equal XOR" is implemented in Java. The presented solution defines a method findTriplets
that counts such triplets in an array data
where the XOR of elements between two positions i
and j
is equal to the XOR of elements between positions j+1
and k
.
- Initialize an integer variable
tripletCount
to count the number of valid triplets. - Maintain a prefix XOR sum
xorSum
to keep track of XOR up to the current index in the array. - Use two hashmaps:
frequencyMap
to store the frequency of occurrence of each XOR sum.sumMap
to store the sum of indices where each XOR sum is encountered.
The core idea is to iterate through the array and for each element:
- Update the
xorSum
with the XOR of the current element. - Calculate the potential contributions to
tripletCount
from the currentxorSum
by checking how many timesxorSum
has appeared before the current index and where it appeared. - Store the frequency of the current
xorSum
infrequencyMap
and the sum of indices insumMap
.
The method finally returns tripletCount
indicating the number of triplets fulfilling the condition. The solution employs efficient use of hashmaps to store pre-computed values, reducing the complexity compared to a naive approach that checks all possible triplets explicitly.
class Solution:
def countTriplets(self, array: List[int]) -> int:
n = len(array)
triplet_count = 0
xor_sum = 0
# Using dictionaries to store counts and totals based on XOR results
count_tracker = defaultdict(int)
count_tracker[0] = 1
sum_tracker = defaultdict(int)
# Looping over the array elements
for index in range(n):
# XOR accumulation
xor_sum ^= array[index]
# Count triplets contribution from this position
triplet_count += count_tracker[xor_sum] * index - sum_tracker[xor_sum]
# Update maps with the current XOR and indices
sum_tracker[xor_sum] += index + 1
count_tracker[xor_sum] += 1
return triplet_count
This Python 3 solution tackles the problem of counting triplets in an array such that they can equate to two arrays of equal XOR. The approach leverages dictionary structures to efficiently compute the desired counts without explicitly considering all possible triplet combinations. Here’s a clear breakdown of how the solution operates:
Initialize Variables:
xor_sum
is used to hold the cumulative XOR of array elements as the array is processed element by element. Atriplet_count
starts at zero and increments based on certain conditions discussed below.Use Two Dictionaries:
count_tracker
keeps a running tally of how many times each XOR result has appeared.sum_tracker
captures the sum of indices where each XOR result appeared.
Iterate Through the Array: For each element in the array, update the
xor_sum
.Count Contributions: For the current XOR result, calculate contributions to the triplet count. This is achieved by the formula where contributions are added based on previous appearances (
index
times the count fromcount_tracker
minus the sum stored insum_tracker
).Update Mappings: Increase counters in both dictionaries for tracking future iterations.
Return the Result: The accumulated
triplet_count
provides the number of valid triplet indices that meet the prompt’s condition.
Efficiency Considerations: This solution leverages the linear complexity of array traversal alongside dictionary operations, which theoretically are average-case constant time, leading to significant efficiency improvements over brute-force solutions.
This code effectively eliminates the need for nested loops through clever use of cumulative property storage and leveraging data structures like dictionaries, which are inherently more efficient for these types of look-up operations. Thus, you achieve both clarity and performance.
No comments yet.