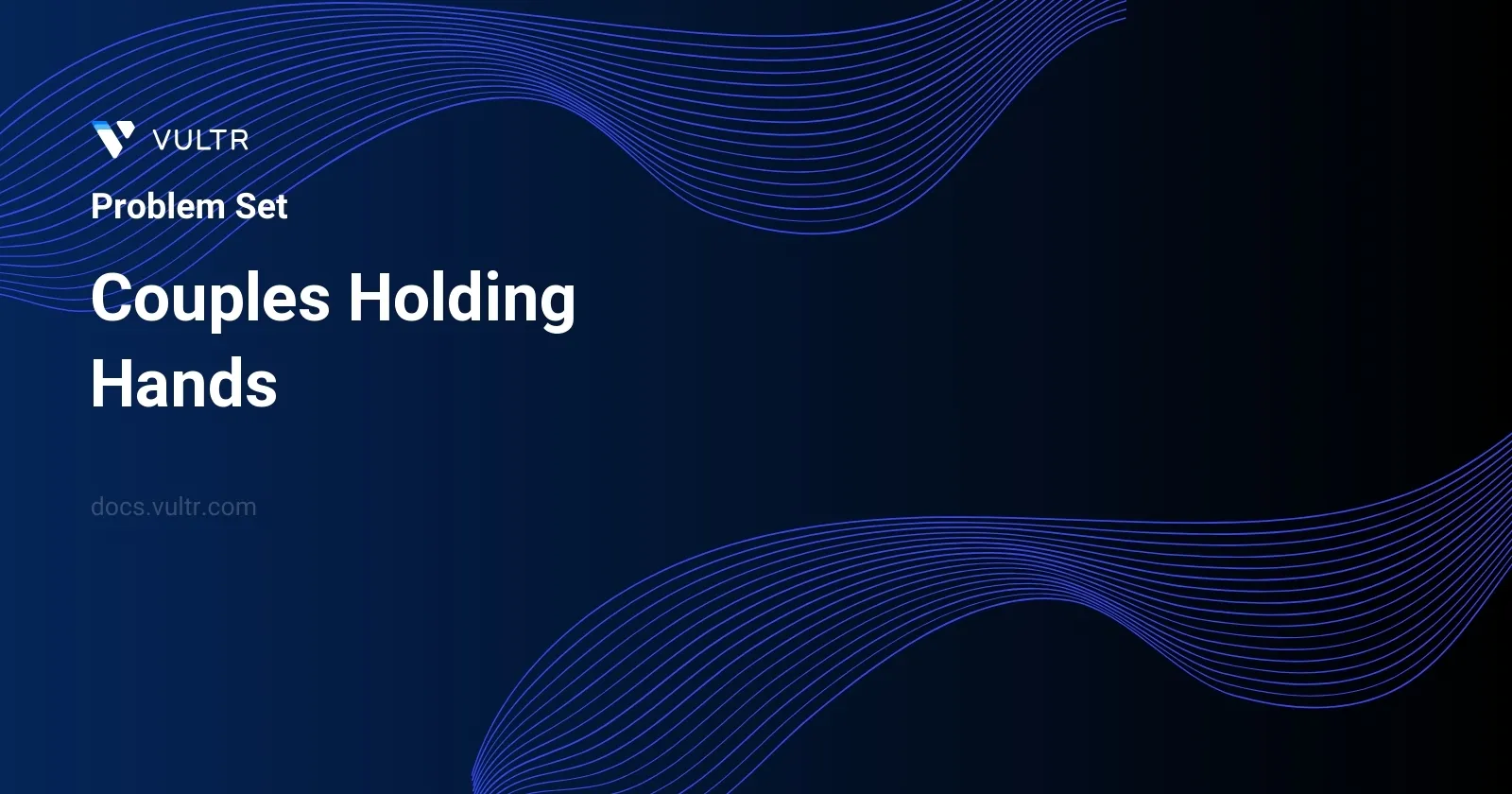
Problem Statement
In this problem, we are given n
couples that are sitting in 2n
seats, arranged in a linear row. Each person in these seats is identified uniquely by an integer, and they are coupled in a sequential manner, meaning the first couple is represented as (0, 1)
, the second as (2, 3)
, and so on until (2n-2, 2n-1)
.
Our objective is to determine the minimum number of swaps required so that all couples are seated next to each other. A swap is defined as two individuals standing up and exchanging their seats. This situation is modeled with an array named row
, where row[i]
gives the ID of the person sitting in the i-th
seat.
Examples
Example 1
Input:
row = [0,2,1,3]
Output:
1
Explanation:
We only need to swap the second (row[1]) and third (row[2]) person.
Example 2
Input:
row = [3,2,0,1]
Output:
0
Explanation:
All couples are already seated side by side.
Constraints
2n == row.length
2 <= n <= 30
n
is even.0 <= row[i] < 2n
- All the elements of
row
are unique.
Approach and Intuition
The primary challenge is to rearrange the array such that each sequential pair (2i, 2i+1)
for all i
from 0
to n-1
are couples according to their IDs. We need to make these arrangements by swapping any two individuals, striving to achieve this with the minimum number of swaps.
To approach this:
- First, understand the current arrangement by mapping each individual to their current position.
- Next, iterate through the
row
array, and for each odd-indexed position ensure it's coupled with the right partner (as couples are in sequential order). - If the current person at an odd-indexed position isn't paired with the correct partner, find the correct partner elsewhere in the array, and perform a swap.
- Every valid swap accounts for correctly positioning a couple. Continue this process until all individuals are correctly paired with their partners.
This greedy swapping ensures that each swap contributes directly to placing a couple together, contributing to the goal of minimising the swap count.
Example 1
- Input:
row = [0,2,1,3]
- Output:
1
- Explanation: The pair
(2, 3)
is separated by0
. By swapping2
(at index1
) and1
(at index2
), we couple(0, 1)
and(2, 3)
correctly with just one swap.
Example 2
- Input:
row = [3,2,0,1]
- Output:
0
- Explanation: Here, all couples
(2, 3)
and(0, 1)
are already positioned next to each other. Therefore, no swaps are required.
From these examples, it's clear that the correct identification of misplaced individuals and their partners and the subsequent swap is central to efficiently solving this problem.
Solutions
- Java
class Solution {
public int swapCouples(int[] seats) {
int swaps = 0;
for (int i = 0; i < seats.length; i += 2) {
int partner = seats[i];
if (seats[i+1] == (partner ^ 1)) continue;
swaps++;
for (int j = i+1; j < seats.length; j++) {
if (seats[j] == (partner ^ 1)) {
seats[j] = seats[i+1];
seats[i+1] = partner ^ 1;
break;
}
}
}
return swaps;
}
}
The Java solution for the problem "Couples Holding Hands" utilizes an array to track seated couples and determines the minimum number of adjacent pairs that need to be swapped to ensure each couple sits together.
The method
swapCouples
, accepts an integer arrayseats
where the positions of individuals are marked sequentially.The process iterates over the array in steps of two since each couple ideally occupies two adjacent seats.
For each pair in the seats array (represented by indices
i
andi+1
):Determine if the person at index
i+1
is the correct partner of the individual at indexi
. This is checked using the bitwise XOR operation,partner ^ 1
, wherepartner
is the value atseats[i]
.If the partner is incorrect, increment the swap counter
swaps
.Locate the correct partner in the rest of the array and perform a swap, ensuring the couple sits together.
The loop search ensures that for each couple starting on an even index
i
, the partner sitting next to them is swapped correctly if they are not already seated together.The method returns the count of swaps required to arrange all pairs correctly.
In summary, the solution effectively manipulates array indices and values by applying basic operations and searches to couple the individuals correctly with a minimum number of adjacent swaps.
No comments yet.