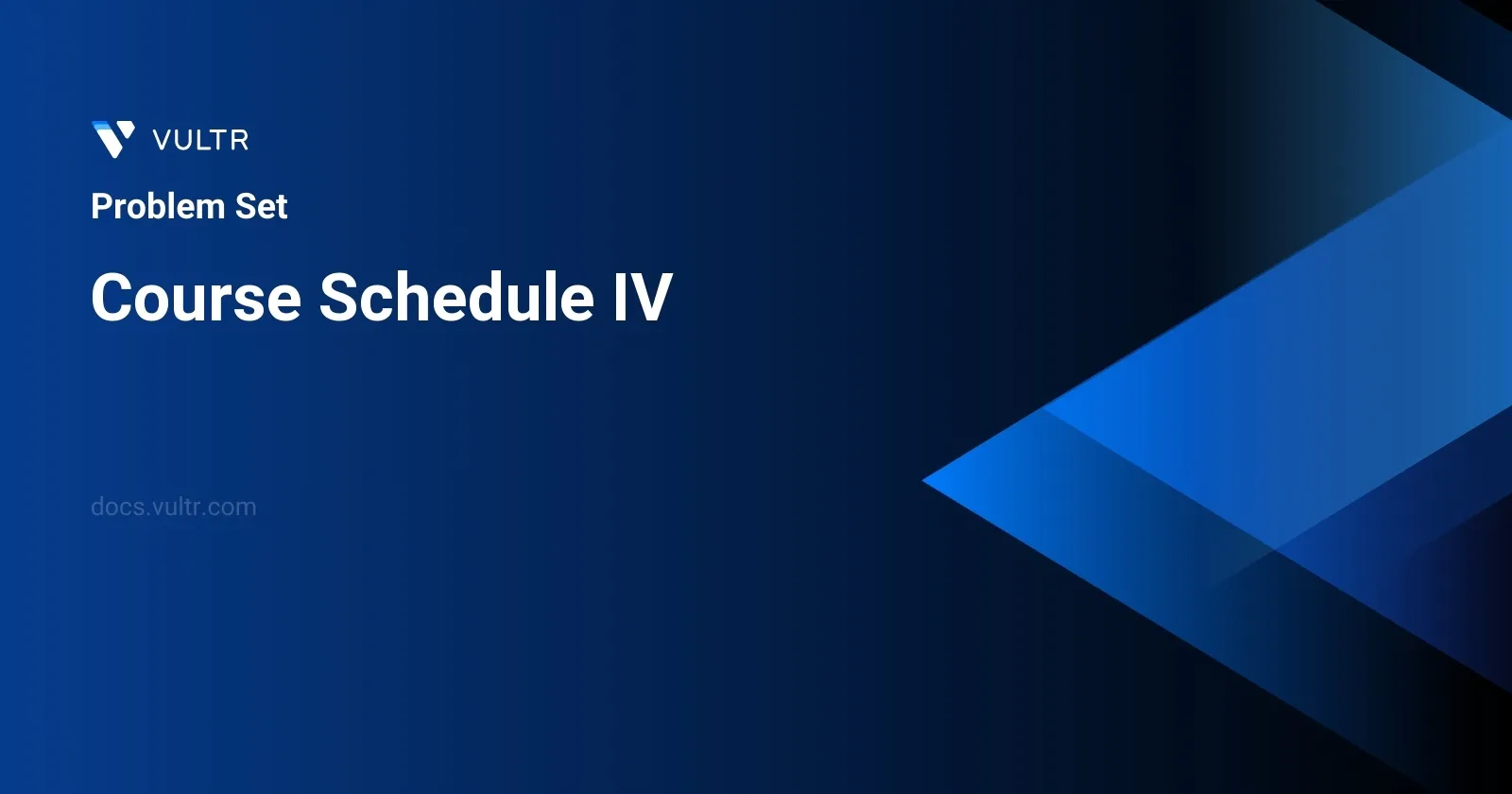
Problem Statement
In the educational setting of this problem, you are required to complete certain courses to fulfill a degree requirement, and each course may have other courses as prerequisites. Specifically, let's say there are numCourses
courses, uniquely identified from 0
to numCourses - 1
.
The requirements are defined in an array prerequisites
, where each element, [ai, bi]
, denotes that you must take course ai
before you can enroll in course bi
.
Furthermore, the relationship created by these prerequisites can be multilayered or indirect. For example, if a
is a prerequisite for b
and b
is a prerequisite for c
, then implicitly a
is also a prerequisite for c
.
The main task here is to answer several queries about these course relationships, which are provided in the array queries
. Each query, [uj, vj]
, asks whether course uj
is a prerequisite for course vj
. The expected output in response to the queries is a boolean array where each element corresponds to whether the queried relationship exists.
Examples
Example 1
Input:
numCourses = 2, prerequisites = [[1,0]], queries = [[0,1],[1,0]]
Output:
[false,true]
Explanation:
The pair [1, 0] indicates that you have to take course 1 before you can take course 0. Course 0 is not a prerequisite of course 1, but the opposite is true.
Example 2
Input:
numCourses = 2, prerequisites = [], queries = [[1,0],[0,1]]
Output:
[false,false]
Explanation:
There are no prerequisites, and each course is independent.
Example 3
Input:
numCourses = 3, prerequisites = [[1,2],[1,0],[2,0]], queries = [[1,0],[1,2]]
Output:
[true,true]
Constraints
2 <= numCourses <= 100
0 <= prerequisites.length <= (numCourses * (numCourses - 1) / 2)
prerequisites[i].length == 2
0 <= ai, bi <= numCourses - 1
ai != bi
- All the pairs
[ai, bi]
are unique. - The prerequisites graph has no cycles.
1 <= queries.length <= 104
0 <= ui, vi <= numCourses - 1
ui != vi
Approach and Intuition
Graph Representation of Prerequisites: To efficiently answer the prerequisite checks, you can represent the given prerequisites as a directed graph. Here, each course is a node and a directed edge from node
ai
to nodebi
indicates thatai
is a prerequisite forbi
.Processing the Graph with Depth-first Search (DFS) or Breadth-first Search (BFS): To determine if there exists a path from course
uj
to coursevj
(i.e., ifuj
is a prerequisite forvj
), one can perform a search starting at courseuj
. If you can reachvj
, then the answer istrue
, otherwise it’sfalse
.Using Transitive Closure: A more preemptive approach uses the concept of transitive closure in graph theory, which can preprocess the information to quickly answer any queried relationship. Specifically, this involves computing a matrix which answers whether a path exists between each pair of nodes (courses) or not.
Handling Disconnected Graphs: Since some courses may not have any prerequisites, the graph can be disconnected. This means it's possible that some courses are not reachable from others, and this needs to be taken into account to avoid erroneous conclusions.
Edge Cases:
- Where no prerequisites are defined (
prerequisites = []
), each query should return false since no course is related to another. - In scenarios where
prerequisites
forms a long chain or deep trees, ensuring that the search doesn't exceed the allowed time is crucial, making the choice of search algorithm or strategy critical.
- Where no prerequisites are defined (
Through these approaches, the system devised can respond accurately and efficiently to the prerequisite queries, reflecting the complexities and dependencies among the courses in a structured educational framework.
Solutions
- C++
- Java
- Python
class Solution {
public:
vector<bool> prerequisitesCheck(int coursesCount,
vector<vector<int>>& preconditions,
vector<vector<int>>& courseQueries) {
vector<vector<bool>> prerequisiteMatrix(coursesCount,
vector<bool>(coursesCount, false));
for (auto pre : preconditions) {
prerequisiteMatrix[pre[0]][pre[1]] = true;
}
for (int k = 0; k < coursesCount; k++) {
for (int i = 0; i < coursesCount; i++) {
for (int j = 0; j < coursesCount; j++) {
// Transitive closure update.
prerequisiteMatrix[i][j] = prerequisiteMatrix[i][j] ||
(prerequisiteMatrix[i][k] && prerequisiteMatrix[k][j]);
}
}
}
vector<bool> results;
for (auto query : courseQueries) {
results.push_back(prerequisiteMatrix[query[0]][query[1]]);
}
return results;
}
};
The provided C++ code defines a function within a class Solution
that determines if specific courses are prerequisites for others based on given preconditions. The function prerequisitesCheck
involves the following steps:
Initialize a 2D boolean matrix
prerequisiteMatrix
sized according to thecoursesCount
, setting all values initially tofalse
. This matrix represents whether a course at index i is a prerequisite for a course at index j.Populate this matrix using a given list of
preconditions
. Each precondition is a vector where the first element is a prerequisite for the second element; this relationship is marked astrue
in the matrix.Utilize the Floyd-Warshall algorithm to compute the transitive closure of the prerequisites matrix. This determines whether a sequence of prerequisite relationships exists between any two courses, updating the matrix to reflect these extended relationships.
Process each query in
courseQueries
to determine if one course is a prerequisite for another by checking the final state ofprerequisiteMatrix
.Push the result (true or false) into a results vector based on the queried relationships from
courseQueries
.Return the results vector, which contains boolean values corresponding to each query, indicating whether the first course in the query is a prerequisite for the second course based on both direct and indirect preconditions.
public class Solution {
public List<Boolean> canTakeCourses(
int totalCourses,
int[][] courseDependencies,
int[][] courseQueries
) {
boolean[][] directPrerequisite = new boolean[totalCourses][totalCourses];
for (int[] coursePair : courseDependencies) {
directPrerequisite[coursePair[0]][coursePair[1]] = true;
}
for (int middleCourse = 0; middleCourse < totalCourses; middleCourse++) {
for (int startCourse = 0; startCourse < totalCourses; startCourse++) {
for (int endCourse = 0; endCourse < totalCourses; endCourse++) {
directPrerequisite[startCourse][endCourse] =
directPrerequisite[startCourse][endCourse] ||
(directPrerequisite[startCourse][middleCourse] &&
directPrerequisite[middleCourse][endCourse]);
}
}
}
List<Boolean> results = new ArrayList<>();
for (int[] query : courseQueries) {
results.add(directPrerequisite[query[0]][query[1]]);
}
return results;
}
}
This solution addresses the problem of determining if specific courses can be taken given a list of course prerequisites. The Java implementation utilizes a graph represented by a 2D Boolean array (directPrerequisite
) to track direct and indirect prerequisites among courses.
- Initialize a 2D array
directPrerequisite
where each element[i][j]
is set totrue
if coursei
is a direct prerequisite for coursej
. - Iterate over the provided
courseDependencies
array to populate thedirectPrerequisite
array based on the direct relationships given. - Use the Floyd-Warshall algorithm to compute transitive closures within the graph. This loop:
- Goes through each course as a possible intermediate step (
middleCourse
). - Then iterates for each possible starting (
startCourse
) and ending course (endCourse
). - Updates
directPrerequisite[startCourse][endCourse]
totrue
if there exists a path throughmiddleCourse
that makesstartCourse
a prerequisite forendCourse
.
- Goes through each course as a possible intermediate step (
After building the complete transitive closure:
- Initialize an ArrayList
results
to store Boolean values. - For each pair of courses in
courseQueries
, checkdirectPrerequisite
to determine if the second course can be taken after completing the first course. - Return the
results
list indicating for each query pair whether the second course is accessible given the prerequisites.
This approach effectively queries prerequisite relationships in constant time after an initial computation cost, making it efficient for multiple queries.
class Solution:
def isPrerequisiteExists(
self,
totalCourses: int,
prereq: List[List[int]],
courseQueries: List[List[int]],
) -> List[bool]:
directPrereq = [[False] * totalCourses for _ in range(totalCourses)]
for p in prereq:
directPrereq[p[0]][p[1]] = True
for k in range(totalCourses):
for i in range(totalCourses):
for j in range(totalCourses):
directPrereq[i][j] = (
directPrereq[i][j] or (directPrereq[i][k] and directPrereq[k][j])
)
results = []
for query in courseQueries:
results.append(directPrereq[query[0]][query[1]])
return results
The Python3 solution provided facilitates determining if certain courses are prerequisites for others within a set schedule. The code addresses the task through the following steps:
Initialize a two-dimensional list called
directPrereq
to store boolean values, set toFalse
by default, which represent whether a course is a direct prerequisite of another course.Update the
directPrereq
matrix based on the given list of prerequisites. If a specific coursea
is a prerequisite for courseb
, then the respective cell in the matrix for these courses is set toTrue
.Use the Floyd-Warshall algorithm to extend the direct prerequisite relations to indirect relationships. This involves three nested loops which iteratively update the
directPrereq
matrix to reflect whether a transition through an intermediate course establishes a prerequisite relationship.For each query in
courseQueries
, check thedirectPrereq
matrix to see if the first course is a prerequisite for the second course in the query list. The result for each query is then appended to the results list.Return the list of boolean results corresponding to each query, indicating whether the first course in each query is a prerequisite for the second one.
This solution effectively transforms the initial list of direct prerequisites into a comprehensive map of all (direct and indirect) prerequisite relationships, allowing quick look-up for the queries.
No comments yet.