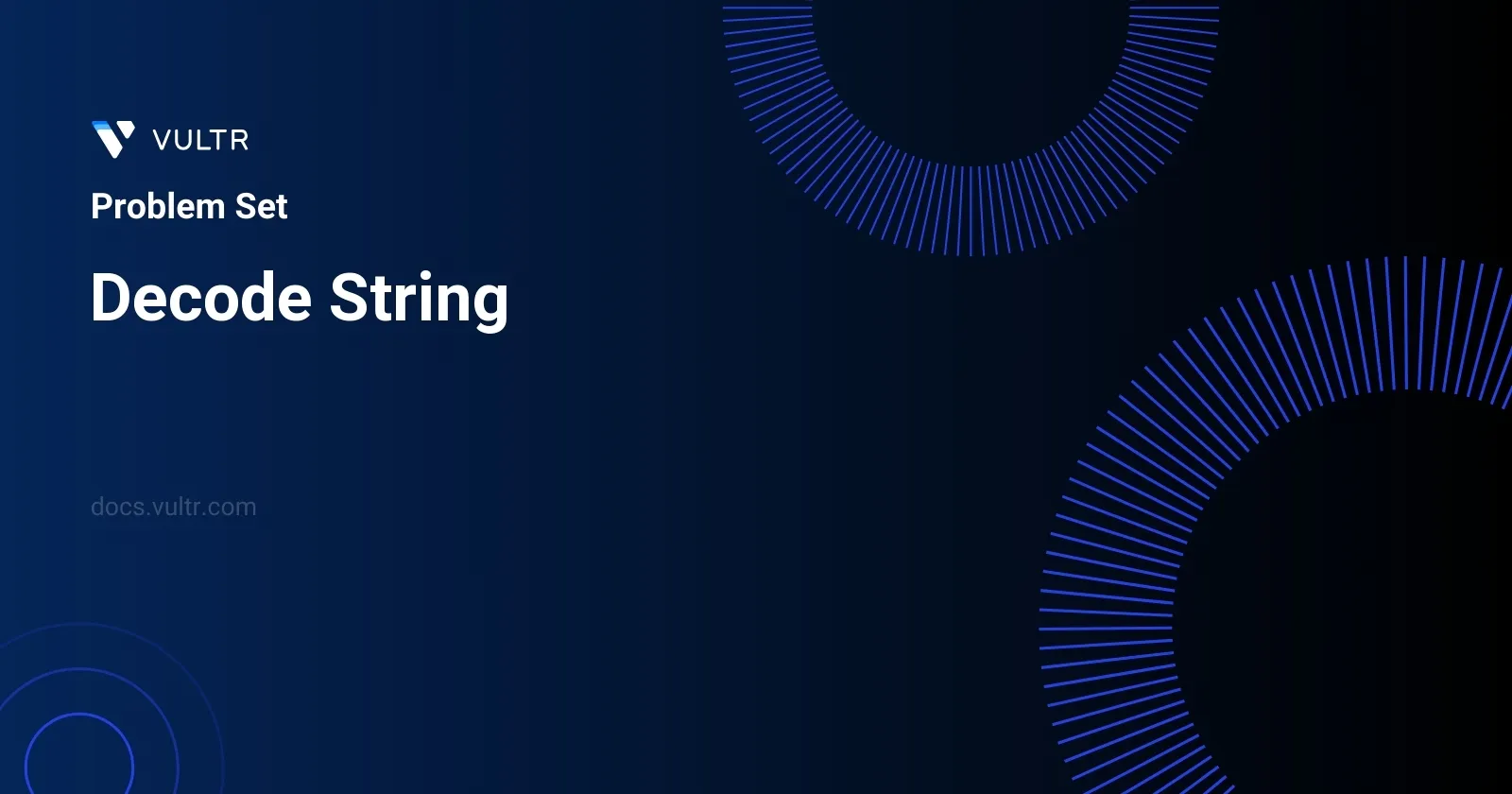
Problem Statement
In this problem, we are provided with an encoded string where the encoding follows a specific pattern: k[encoded_string]
. The encoded string within the square brackets should be repeated k
times, where k
is a positive integer. The challenge is to decode this string according to the aforementioned rule. The input string lacks any irregular formatting—it does not contain any unnecessary whitespace, all square brackets are correctly paired, and it only uses digits to denote repetition counts. Moreover, the original message (before encoding) does not feature any numerical characters; numbers appear exclusively as repeat-specifiers. Each encoded string will also adhere to constraints ensuring outputs that are feasible to handle, both in length and complexity.
Examples
Example 1
Input:
s = "3[a]2[bc]"
Output:
"aaabcbc"
Example 2
Input:
s = "3[a2[c]]"
Output:
"accaccacc"
Example 3
Input:
s = "2[abc]3[cd]ef"
Output:
"abcabccdcdcdef"
Constraints
1 <= s.length <= 30
s
consists of lowercase English letters, digits, and square brackets'[]'
.s
is guaranteed to be a valid input.- All the integers in
s
are in the range[1, 300]
.
Approach and Intuition
To decode the given encoded strings, we can follow a systematic approach that involves:
- Using a stack data structure to handle the nested and sequential pattern described by the encoding rule. We utilize this stack to build the decoded string as we progress through the input.
- Iterating through the input string character by character, handling different types of characters (digits, letters, and brackets) appropriately:
- For digits: These signify the number of times the following string should be repeated. Multiple digits in sequence should be combined to form the complete multiplier (e.g., "12" should be interpreted as 12).
- For letters: These are the base characters of our strings that will need to be repeated as per the preceding digit.
- For left bracket '[': This denotes the start of a new repeat sequence. We might need to remember the current context (current string and current multiplier) by pushing them onto a stack.
- For right bracket ']': This denotes the end of a repeat sequence. Here, we pop the top of the stack to get our last context, repeat the string encoding within the brackets as specified by the preceding number, and then continue building our string.
- Continuously building and updating the resultant string based on the operations connected with each encountered character.
- By the time we reach the end of the string, the stack should be back to its initial state, and we will have our fully decoded string ready to return.
This method efficiently manages the nested nature of the encoding and can straightforwardly handle even complex nested encoded patterns, as illustrated by the example inputs provided. The constraints ensure the procedure executes swiftly within acceptable limits, making this a robust solution for all provided test cases.
Solutions
- C++
- Java
class Solution {
public:
string unrollString(string str) {
int pos = 0;
return helper(str, pos);
}
string helper(const string& str, int& pos) {
string rebuilt;
while (pos < str.length() && str[pos] != ']') {
if (!isdigit(str[pos]))
rebuilt += str[pos++];
else {
int num = 0;
// calculate num while digit appears
while (pos < str.length() && isdigit(str[pos]))
num = num * 10 + str[pos++] - '0';
// skip '[' character
pos++;
string inner = helper(str, pos);
// skip ']' character
pos++;
while (num-- > 0)
rebuilt += inner;
}
}
return rebuilt;
}
};
This solution in C++ deals with decoding a string where certain parts of the string are enclosed in brackets []
with a number prefix. The number indicates how many times the string within the brackets should be repeated. The input to the function unrollString
is a string that you want to decode, and it returns the decoded string.
- The
unrollString
function initializes the position and calls the helper functionhelper
. - Inside the
helper
function, an empty stringrebuilt
is initialized to start constructing the decoded version of the sub-part of the string it currently processes.
The process unfolds as follows:
- Iterate character by character in the
str
array until you encounter a closing bracket]
or exhaust the string. - If the current character is not a digit, simply append it to
rebuilt
. - If the character is a digit, compute the full number that represents how many times the subsequent string pattern should repeat.
- After computing the repetition number, ignore the open bracket
[
and recursively invokehelper
to process the string enclosed within the brackets. - After getting the decoded string from the recursive call, skip the closing bracket
]
. - Append the decoded string to
rebuilt
as many times as specified by the number calculated earlier.
The recursion effectively handles nested patterns by processing innermost strings first, gradually working outwards, accurately maintaining the sequence and the number of repetitions necessary at each level. The function finally returns the fully decoded string once all nested patterns have been processed.
This methodology is space-efficient as it utilizes a reference to the position and string constructs to decode segments without duplicating arrays or strings unnecessarily.
class Solution {
int position = 0;
String decode(String str) {
StringBuilder output = new StringBuilder();
while (position < str.length() && str.charAt(position) != ']') {
if (!Character.isDigit(str.charAt(position)))
output.append(str.charAt(position++));
else {
int multiplier = 0;
while (position < str.length() && Character.isDigit(str.charAt(position)))
multiplier = multiplier * 10 + str.charAt(position++) - '0';
position++; // Skip the '['
String innerDecoded = decode(str);
position++; // Skip the ']'
while (multiplier-- > 0)
output.append(innerDecoded);
}
}
return output.toString();
}
}
The Java program provided offers a solution for decoding a specially formatted string that includes numbers followed by square brackets. These numbers determine how many times the sequence inside the brackets should be repeated. Below is a summary of how the code solves this problem and how each part of the code contributes to the solution:
Class and Method Structure: A class named
Solution
includes a method calleddecode
which takes a stringstr
as input and uses a recursive approach to decode it.Variables: There is one instance variable
position
used to keep track of the current index in the input string during decoding.Main Decoding Loop:
- Non-Digit Characters: Directly appends any character, that is not a digit, to a
StringBuilder
namedoutput
. - Digit Handling: When a digit is encountered, it calculates the entire number (which might be multi-digit) and this represents the multiplier indicating the number of times to repeat the string inside the subsequent square brackets.
- Recursive Decoding:
- Skips the opening bracket '[' by incrementing
position
. - Calls itself recursively to decode the string inside the brackets. This ensures nested patterns are decoded correctly.
- After the closing bracket ']', the decoded string from the recursive call is appended to
output
as many times as specified by the multiplier.
- Skips the opening bracket '[' by incrementing
- Non-Digit Characters: Directly appends any character, that is not a digit, to a
Termination Condition: The main decoding loop continues until
position
reaches the end of the string or encounters a closing bracket]
, which helps in managing nested structures.Returning the Result: Once the loop completes, the method returns the fully decoded string built in the
output
.
This solution efficiently handles nested and multi-layered encoded strings using recursion. Each recursive call processes another level of encoding, allowing the method to handle complex patterns like 3[a2[c]]
intuitively, by resolving 2[c]
first then repeating the resolved string as specified.
No comments yet.