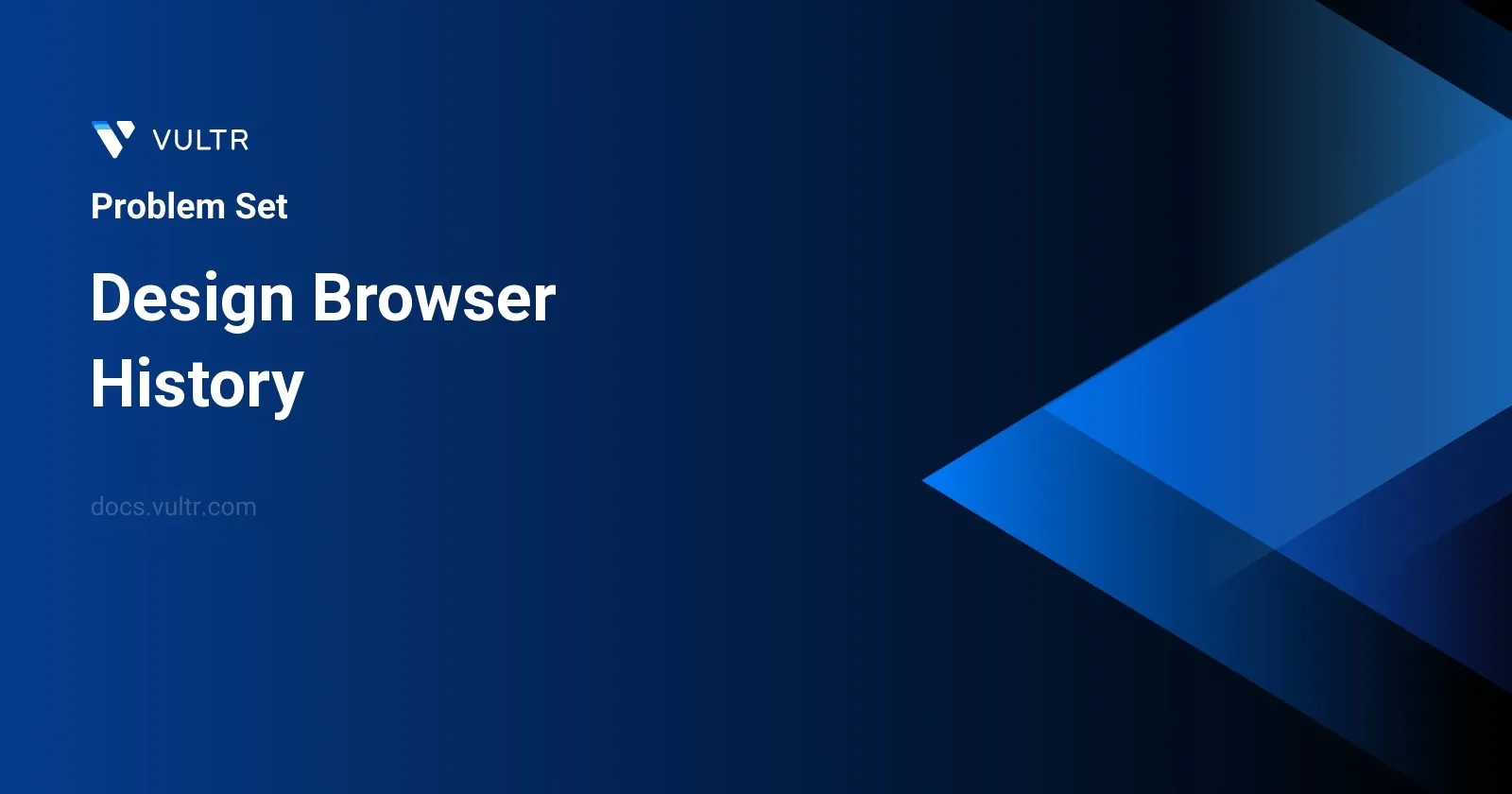
Problem Statement
In this scenario, you are working with a simple web browser that supports basic navigation functionalities in a single tab. The capabilities you need to implement include visiting a new URL from the current one, moving backward in the visitation history by a certain number of steps, and moving forward through the same history by a specified number of steps. The class BrowserHistory
should be designed with methods to handle these functionalities:
BrowserHistory(string homepage)
: This initializes the browser with the given homepage URL.void visit(string url)
: This method should allow the browser to visit a new URL. The forward history from the current page gets cleared as a new URL is visited.string back(int steps)
: This retrieves the URL after moving back a given number of steps in the history. Should the desired steps exceed available history, it returns the furthest possible.string forward(int steps)
: This retrieves the URL after advancing forward by the specified steps. If the desired forward movement exceeds the tracked forward history, it returns as far forward as possible.
The objective is to manage the browser's history effectively, ensuring that movements forward and backward adjust the current position and potential future movements accordingly.
Examples
Example 1
Input:
["BrowserHistory","visit","visit","visit","back","back","forward","visit","forward","back","back"] [["vultr.com"],["google.com"],["facebook.com"],["youtube.com"],[1],[1],[1],["linkedin.com"],[2],[2],[7]]
Output:
[null,null,null,null,"facebook.com","google.com","facebook.com",null,"linkedin.com","google.com","vultr.com"]
Explanation:
BrowserHistory browserHistory = new BrowserHistory("vultr.com"); browserHistory.visit("google.com"); // You are in "vultr.com". Visit "google.com" browserHistory.visit("facebook.com"); // You are in "google.com". Visit "facebook.com" browserHistory.visit("youtube.com"); // You are in "facebook.com". Visit "youtube.com" browserHistory.back(1); // You are in "youtube.com", move back to "facebook.com" return "facebook.com" browserHistory.back(1); // You are in "facebook.com", move back to "google.com" return "google.com" browserHistory.forward(1); // You are in "google.com", move forward to "facebook.com" return "facebook.com" browserHistory.visit("linkedin.com"); // You are in "facebook.com". Visit "linkedin.com" browserHistory.forward(2); // You are in "linkedin.com", you cannot move forward any steps. browserHistory.back(2); // You are in "linkedin.com", move back two steps to "facebook.com" then to "google.com". return "google.com" browserHistory.back(7); // You are in "google.com", you can move back only one step to "vultr.com". return "vultr.com"
Constraints
1 <= homepage.length <= 20
1 <= url.length <= 20
1 <= steps <= 100
homepage
andurl
consist of '.' or lower case English letters.- At most
5000
calls will be made tovisit
,back
, andforward
.
Approach and Intuition
To understand the approach required to simulate the browser history, let's analyze the example provided:
Initialization:
- The browser is initialized at "vultr.com".
Visiting URLs:
- Visits are registered sequentially from "google.com" to "youtube.com", each time clearing forward navigation possible from the current page.
Moving Back:
- It's then asked to move back first by 1 step from "youtube.com" to "facebook.com", and then another step back lands it at "google.com".
Moving Forward:
- Moving forward by 1 step from "google.com" brings it back to "facebook.com".
More Visiting:
- Visiting "linkedin.com" from "facebook.com" clears any forward history entirely.
Attempts to Move Forward:
- Any attempt to move forward beyond this point results in staying on "linkedin.com" because there are no forward entries.
Moving Back Further:
- Moving back by 2 steps from "linkedin.com" takes the browser to "google.com" through "facebook.com".
- An attempt to move back by 7 steps from "google.com" brings it only back to the homepage "vultr.com", which is the furthest back it can go.
From the example, the critical operational details emerge:
- Navigating the website: Each visit should dynamically adjust the history stack.
- Bounds of navigation: Both the
back
andforward
operations are bound by the current position in the history and the extremities of the history list (either the beginning or the most recent page).
The design of BrowserHistory
can effectively be achieved using a list to keep track of visited URLs and an index pointer to the current URL. The operations adapt the current position pointing to handle the navigation requests within the constraints of available history.
Solutions
- C++
- Java
- JavaScript
- Python
class NavigationHistory {
vector<string> history;
int currentIdx, lastIdx;
public:
NavigationHistory(string startPage) {
history.push_back(startPage);
currentIdx = 0;
lastIdx = 0;
}
void visit(string url) {
currentIdx += 1;
if (history.size() > currentIdx) {
history[currentIdx] = url;
} else {
history.push_back(url);
}
lastIdx = currentIdx;
}
string back(int steps) {
currentIdx = max(0, currentIdx - steps);
return history[currentIdx];
}
string forward(int steps) {
currentIdx = min(lastIdx, currentIdx + steps);
return history[currentIdx];
}
};
The problem requires defining a simple browser history navigator in C++, where users can visit new pages and navigate forwards or backwards within their browsing history.
The implemented solution, NavigationHistory
, uses a vector<string>
to store URLs of the sites visited. Two pointer integers, currentIdx
and lastIdx
, track the current position and the furthest page visited, respectively. Here's a breakdown of how the class functions:
Upon initialization with a
startPage
, the history vector includes this page, and both indicescurrentIdx
andlastIdx
are set to 0, pointing to the starting page.The
visit
method allows navigation to a new URL. The current index increments to point to the new page location. If this index exceeds the current length of the history vector, the URL is appended to the history. ThelastIdx
updates to reflect the most recent page.The
back
method enables backward navigation by a given number of steps. It adjusts thecurrentIdx
to ensure it doesn’t fall below zero (which would be out of history bounds), then returns the URL at the new current index.The
forward
method manages forward navigation, checking thatcurrentIdx
does not exceedlastIdx
. It adjusts thecurrentIdx
appropriately and returns the URL of that position.
This solution ensures efficient tracking and accessing of navigation history, providing basic functionalities similar to web browser history mechanisms. Ensure robust testing, particularly for edge cases like attempting to go back or forward beyond the bounds of the history list.
class WebNavigation {
ArrayList<String> history;
int current, end;
public WebNavigation(String startPage) {
history = new ArrayList<String>(Arrays.asList(startPage));
current = 0;
end = 0;
}
public void visit(String page) {
current += 1;
if (history.size() > current) {
history.set(current, page);
} else {
history.add(page);
}
end = current;
}
public String back(int steps) {
current = Math.max(0, current - steps);
return history.get(current);
}
public String forward(int steps) {
current = Math.min(end, current + steps);
return history.get(current);
}
}
This solution describes the implementation of a browser history feature using Java, where a user can navigate through their web history either back or forward. The class WebNavigation
maintains the user’s browsing history and provides methods to navigate through this history conveniently.
The primary components declared in the
WebNavigation
class are:history
: an ArrayList that stores the URL of web pages visited.current
: an integer index pointing to the current page in the history.end
: an integer marking the end of the history list after the latest visit or deletion of forward history.
On initializing the
WebNavigation
object with a starting page, thehistory
ArrayList is initialized with the start page, and bothcurrent
andend
are set to 0.The
visit
method allows adding a new page to the browser history:- It first increments the
current
. - If the current index is less than the size of the history, it replaces the existing entry; otherwise, it adds the new page to the list.
- It updates
end
to the newcurrent
, effectively erasing any forward history.
- It first increments the
The
back
function takessteps
as an argument and moves thecurrent
index backwards without going below 0, ensuring the user doesn’t navigate back past the beginning of history. It then returns the page at the newcurrent
.Similarly, the
forward
function takessteps
as an argument and advances thecurrent
index forward, capped by theend
index which ensures the user cannot navigate forward past their most recently visited page.
Overall, this class provides a structured way to handle browsing history similar to typical web browsers, allowing users to go back and forward through their history based on their navigation actions.
var WebNavigation = function(startPage) {
this.pages = [startPage];
this.currentIndex = 0;
this.maxIndex = 0;
};
WebNavigation.prototype.goTo = function(page) {
this.currentIndex++;
if (this.pages.length > this.currentIndex) {
this.pages[this.currentIndex] = page;
} else {
this.pages.push(page);
}
this.maxIndex = this.currentIndex;
};
WebNavigation.prototype.goBack = function(steps) {
this.currentIndex = Math.max(0, this.currentIndex - steps);
return this.pages[this.currentIndex];
};
WebNavigation.prototype.goForward = function(steps) {
this.currentIndex = Math.min(this.maxIndex, this.currentIndex + steps);
return this.pages[this.currentIndex];
};
This JavaScript code implements a simple Web Navigation system where a user can navigate through their browser history, similar to how typical web browsers handle tab history navigation.
Initialize the browser history with:
WebNavigation(startPage)
: Initializes the navigation system starting atstartPage
. This function sets up the tracking for pages visited and the current index in the browsing history.
Navigate to a new webpage:
goTo(page)
: Directs the browser to a new page. This method increments the current index, updates or adds the new page to the history, and adjusts the maximum index reached in the history.
Move backward through your browser history:
goBack(steps)
: Moves back a certain number of steps in the browser history. The function usesMath.max
to prevent the index from going below 0.
Move forward through your browser history:
goForward(steps)
: Moves forward a number of steps in the browser history. It usesMath.min
to ensure the index does not exceed the furthest page visited.
Both goBack(steps)
and goForward(steps)
return the webpage at the current index after the operation, which allows the user to see the page they navigated to.
class BrowserHistory:
def __init__(self, homepage: str):
self.history = [homepage]
self.current, self.end = 0, 0
def visit(self, url: str) -> None:
self.current += 1
if len(self.history) > self.current:
self.history[self.current] = url
else:
self.history.append(url)
self.end = self.current
def back(self, steps: int) -> str:
self.current = max(0, self.current - steps)
return self.history[self.current]
def forward(self, steps: int) -> str:
self.current = min(self.end, self.current + steps)
return self.history[self.current]
The provided Python solution involves simulating a simplified browser history with three primary functions: visit
, back
, and forward
. Here's how each function operates:
Initialization (
__init__
):- Initiate with the
homepage
setting the starting page of the browser history.
- Initiate with the
visit
(Visiting a new URL):- Increments the current index and updates/adds the URL to the history list. Also resets the end point to the current index, effectively removing forward history after a new visit.
back
(Navigating backwards):- Decrements the current index by a given number of steps but does not go below zero. Returns the URL at the adjusted index.
forward
(Navigating forwards):- Increments the current index bounded by the end index and returns the URL at the new index.
This basic browser history mechanism effectively uses Python lists to manage URLs and simulate browsing forward and backward through previously visited sites based on user actions.
No comments yet.