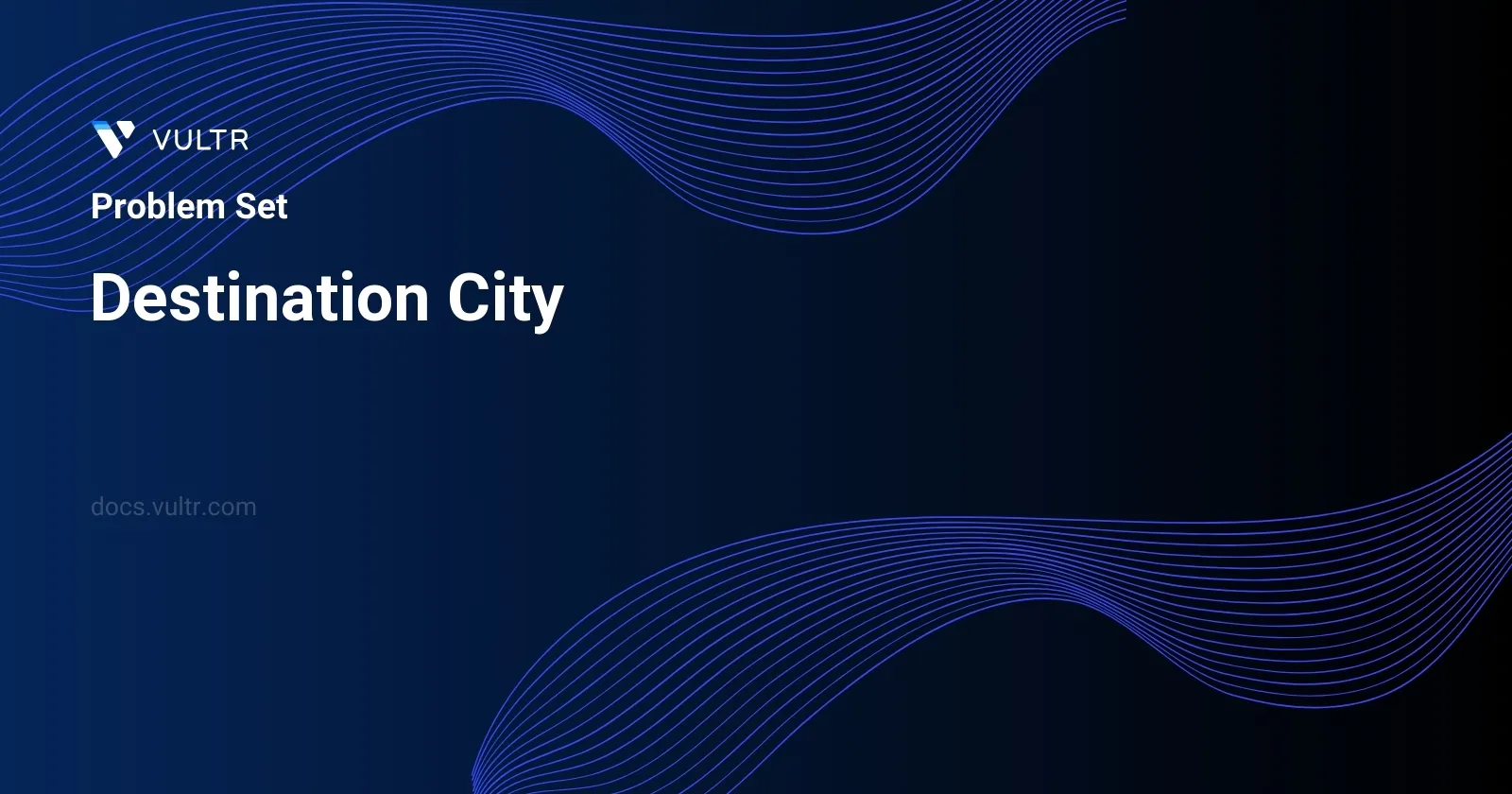
Problem Statement
In this task, you are given an array of direct paths represented as pairs, where each pair [cityAi, cityBi]
indicates a direct route from cityAi
to cityBi
. Your goal is to determine which city is the ultimate destination of this sequence of trips. The destination city is identified as the one which does not have any other city listed as its destination in the provided array of paths. Essentially, you have to find a city from which no outgoing paths are present in the given list. This problem is set in a graph context without loops, ensuring a straightforward path leading to a unique endpoint city.
Examples
Example 1
Input:
paths = [["London","New York"],["New York","Lima"],["Lima","Sao Paulo"]]
Output:
"Sao Paulo"
Explanation:
Starting at "London" city you will reach "Sao Paulo" city which is the destination city. Your trip consist of: "London" -> "New York" -> "Lima" -> "Sao Paulo".
Example 2
Input:
paths = [["B","C"],["D","B"],["C","A"]]
Output:
"A"
Explanation:
All possible trips are: "D" -> "B" -> "C" -> "A". "B" -> "C" -> "A". "C" -> "A". "A". Clearly the destination city is "A".
Example 3
Input:
paths = [["A","Z"]]
Output:
"Z"
Constraints
1 <= paths.length <= 100
paths[i].length == 2
1 <= cityAi.length, cityBi.length <= 10
cityAi != cityBi
- All strings consist of lowercase and uppercase English letters and the space character.
Approach and Intuition
To find the destination city, consider the following approach:
- Track all cities that are starting points (
cityAi
) and all cities that are endpoints (cityBi
) in separate lists or sets. - The destination city is simply the city that appears in the endpoint list but never appears in the starting point list. This approach leverages the problem's guarantee that the path structure forms a line without loops.
Example Analyses from Provided Examples:
Example 1:
- Paths:
[["London","New York"],["New York","Lima"],["Lima","Sao Paulo"]]
- Start Cities:
London, New York, Lima
- End Cities:
New York, Lima, Sao Paulo
Sao Paulo
is the only city among end cities that does not appear as a start city, hence it is the destination.
- Paths:
Example 2:
- Paths:
[["B","C"],["D","B"],["C","A"]]
- Start Cities:
B, D, C
- End Cities:
C, B, A
- Only
A
appears solely in the endpoint list, making it the destination.
- Paths:
Example 3:
- Paths:
[["A","Z"]]
- Start Cities:
A
- End Cities:
Z
Z
does not appear as a start city, therefore it's identified as the destination.
- Paths:
This approach systematically processes the paths to single out the destination city based on the properties of the graph formed by the paths.
Solutions
- C++
- Java
- Python
class Solution {
public:
string findDestinationCity(vector<vector<string>>& routes) {
unordered_set<string> citiesWithDeparture;
for (auto& route : routes) {
citiesWithDeparture.insert(route[0]);
}
for (auto& route : routes) {
string target = route[1];
if (citiesWithDeparture.find(target) == citiesWithDeparture.end()) {
return target;
}
}
return "";
}
};
This solution involves finding the destination city from a given list of routes. Each route is represented as a vector of strings, where the first string is the departure city and the second is the destination city. The solution utilizes C++ and the standard library's unordered_set to efficiently determine the unique destination city that does not act as a departure city for any other route.
- Start by defining a class
Solution
with a public member functionstring findDestinationCity(vector<vector<string>>& routes)
. - Create an unordered_set named
citiesWithDeparture
to store cities that are departure points. - Loop through the routes array using a range-based for loop to populate the
citiesWithDeparture
set with the first city of each route (departure city). - Iterate again over the routes to check each destination city:
- For each destination city in the route list, check if it is not present in the
citiesWithDeparture
set. - If a city is found that does not exist in the
citiesWithDeparture
, return this city as it does not serve as a departure city and thus, is the final destination. - If no such city is found (though the problem context suggests there should be one), return an empty string.
- For each destination city in the route list, check if it is not present in the
This method ensures an efficient check by taking advantage of the fast look-up times of unordered sets, concluding with a solution that meets the problem's requirements.
class Solution {
public String findDestinationCity(List<List<String>> travelPaths) {
Set<String> origins = new HashSet<>();
for (List<String> path : travelPaths) {
origins.add(path.get(0));
}
for (List<String> path : travelPaths) {
String destination = path.get(1);
if (!origins.contains(destination)) {
return destination;
}
}
return "";
}
}
The provided Java solution determines the destination city from a list of travel paths. The approach employs two main data structures: a Set
called origins
and a nested List
called travelPaths
. Each entry in travelPaths
is assumed to consist of two elements — the starting city (origin) and the ending city (destination).
- Initialize a
Set
namedorigins
to store each travel path's origin city. This helps in checking if a city is a starting point for any route. - Iterate through each path in
travelPaths
:- Add the origin city of the current path to the
origins
set.
- Add the origin city of the current path to the
- Traverse the travel paths a second time:
- For each path, check if its destination city is not in the
origins
set. - If a city appears exclusively as a destination (i.e., it is not found in the
origins
set), then that city is identified as the final destination. The function then returns this city.
- For each path, check if its destination city is not in the
Given the nature of the problem, if a city is not a point of origin for any path, it must be the endpoint of the series of travels. Thus, the algorithm effectively identifies the city where the journey will end based on the provided routes. In scenarios where no distinct endpoint exists, an empty string is returned as per the code. The solution provides an efficient means to pinpoint the ending city, ensuring that only cities that have never been used as a starting point are potential endpoints.
class Solution:
def findDestination(self, routes: List[List[str]]) -> str:
sources = set()
for path in routes:
sources.add(path[0])
for path in routes:
destination = path[1]
if destination not in sources:
return destination
return ""
The provided Python solution addresses the problem of finding a destination city that you cannot travel from to any other city, given a list of city pairs representing direct paths between two cities. The solution operates in two main phases:
- Create a set named
sources
to track all cities that serve as a starting point. - Iteratively populate the
sources
set with the first city from each city pair in theroutes
input list.
After collecting all source cities, the code performs the following steps:
- Traverse each path in the
routes
list. - Check if the destination city (second city in each path) is not part of the
sources
set. - If a city appears only as a destination and never as a source, the code returns this city, as it is the ultimate destination city where no further travel is possible.
If no such destination city exists that isn’t a source city in any route, the function returns an empty string. This solution effectively identifies the endpoint in a series of directed paths by leveraging set operations for efficient lookup.
No comments yet.