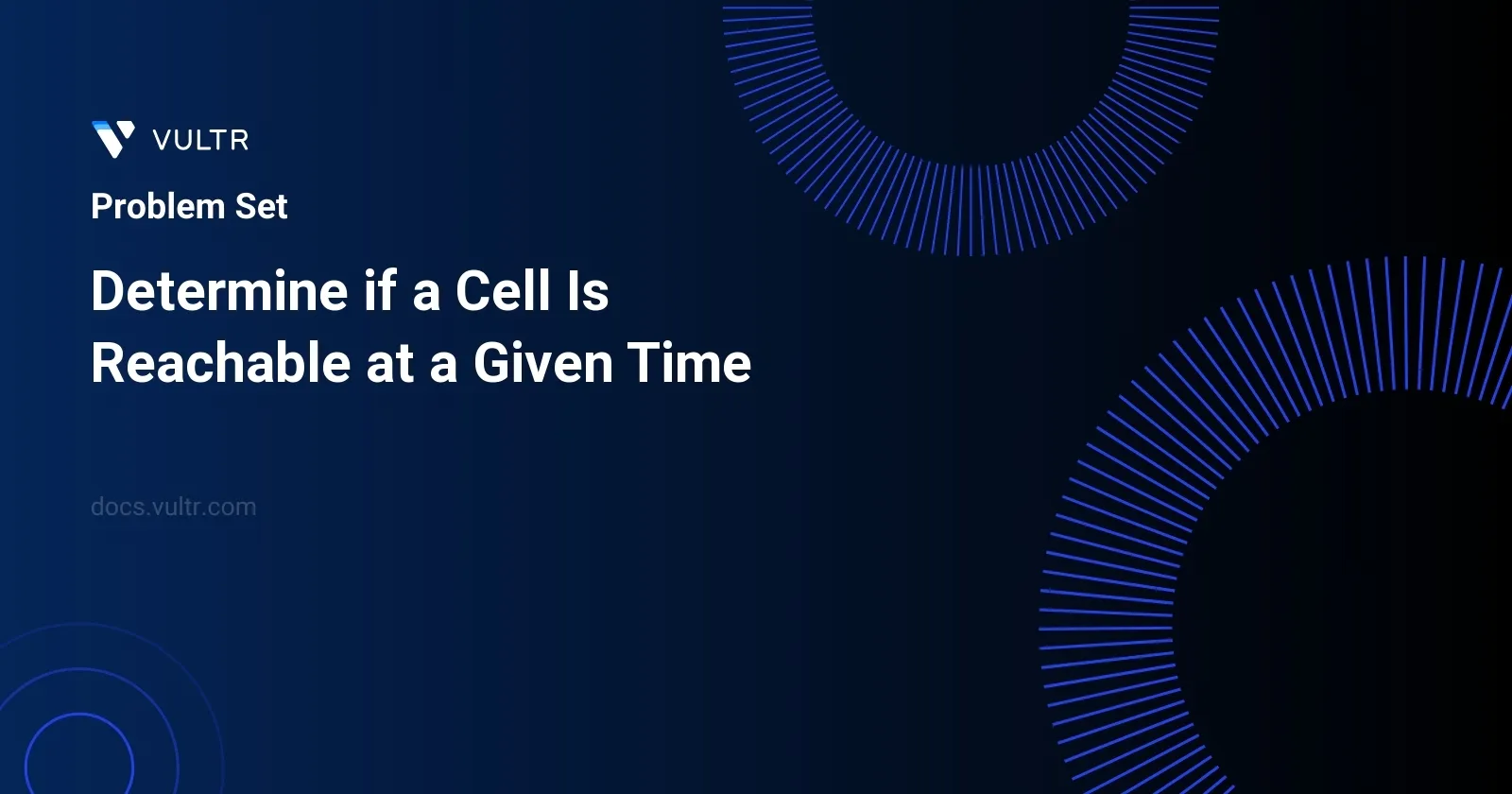
Problem Statement
The task is to determine whether it's possible to exactly reach a destination point (fx, fy)
from a starting point (sx, sy)
in an infinite 2D grid after exactly t
seconds. At each second, movement to any of the eight adjacent cells surrounding the current location is possible. A pivotal consideration is that each move takes one exact second, and you are allowed to visit the same cell multiple times. The decision to be made is whether you can land on (fx, fy)
right at the t
-th second.
Examples
Example 1
Input:
sx = 2, sy = 4, fx = 7, fy = 7, t = 6
Output:
true
Explanation:
Starting at cell (2, 4), we can reach cell (7, 7) in exactly 6 seconds by going through the cells depicted in the picture above.
Example 2
Input:
sx = 3, sy = 1, fx = 7, fy = 3, t = 3
Output:
false
Explanation:
Starting at cell (3, 1), it takes at least 4 seconds to reach cell (7, 3) by going through the cells depicted in the picture above. Hence, we cannot reach cell (7, 3) at the third second.
Constraints
1 <= sx, sy, fx, fy <= 109
0 <= t <= 109
Approach and Intuition
To begin solving the problem, it's necessary first to determine the Manhattan distance between the start
(sx, sy)
and the finish(fx, fy)
positions. The Manhattan distance provides the minimum steps required to travel between two points when movement is restricted to grid lines.- The calculation is based on the formula:
min_steps = abs(fx - sx) + abs(fy - sy)
.
- The calculation is based on the formula:
Using this minimum number of steps (
min_steps
), approximate if it's feasible to make these steps within the given timet
.- If
min_steps
is more significant thant
, it's impossible to reach(fx, fy)
in time because we don't have enough seconds. - If
min_steps
is less than or equal tot
, further considerations take effect.
- If
Account for the number of additional moves needed if
min_steps
is less thant
. To land exactly on(fx, fy)
aftert
seconds:- The additional moves (
t - min_steps
) should allow one to formulate a sequence that ultimately permits an exact(fx, fy)
landing att
seconds. - These excess moves, because every extra move requires a returning move to keep you in a possible path to reach the destination exact after
t
moves, must be such that they are compensated by a subsequent reversing or nullifying move.
- The additional moves (
A crucial observation:
- As the movement forms a Manhattan grid and moving back and forth or up and down between two cells doesn't change the net distance covered,
t
must have the same parity (even or odd) asmin_steps
. This ensures that any surplus time can be used effectively to simulate stationary movement (moving to a cell and moving back) which does not change the position but consumes time.
- As the movement forms a Manhattan grid and moving back and forth or up and down between two cells doesn't change the net distance covered,
To determine reachability:
- Calculate
min_steps
- Compare
min_steps
witht
to see if it's feasible to even consider reaching(fx, fy)
- Check if
t - min_steps
, if even or odd, matches the evenness or oddness of the path length. This ensures that you can use any extra time without ending up away from(fx, fy)
.
Understanding through examples:
- For Example 1 with inputs
(sx = 2, sy = 4, fx = 7, fy = 7, t = 6)
: The minimum steps to reach(7, 7)
from(2, 4)
are 8 (5+3), but one can manipulate movements using remaining negative surplus moves to remain stationary, effectively using the time and reaching the destination exactly att = 6
. - For Example 2 with inputs
(sx = 3, sy = 1, fx = 7, fy = 3, t = 3)
: The minimum steps are 6 (4+2), and with only 3 seconds available, there's no tactical way to negate the extra moves required.
This concept leverages grid properties and parity characteristics of even and odd numbers to ascertain exactness in movement and timing, key to solving the problem efficiently and correctly.
Solutions
- C++
- Java
- C
- JavaScript
- Python
class Solution {
public:
bool canReachDestination(int startX, int startY, int finalX, int finalY, int time) {
int deltaX = abs(startX - finalX);
int deltaY = abs(startY - finalY);
if (deltaX == 0 && deltaY == 0 && time == 1) {
return false;
}
return time >= max(deltaX, deltaY);
}
};
This code snippet defines a method in C++ to determine whether it is possible to reach a target cell in a matrix from a given starting position within a specified time. The function canReachDestination()
receives five parameters: the starting coordinates (startX
, startY
), the destination coordinates (finalX
, finalY
), and the available time
to reach the destination. The approach primarily revolves around calculating the absolute differences between the corresponding coordinates of the start and final positions (deltaX
and deltaY
). The condition checks:
- If the starting position coincides with the destination and only one unit of time is allowed, the function returns
false
. - In all other scenarios, the function checks whether the given time is greater than or equal to the maximum of
deltaX
anddeltaY
.
This ensures flexibility in movement pattern, prioritizing the maximum required steps in any direction to conclude if the destination is reachable within the specified duration.
class Solution {
public boolean canReachDestination(int startX, int startY, int endX, int endY, int time) {
int deltaX = Math.abs(startX - endX);
int deltaY = Math.abs(startY - endY);
if (deltaX == 0 && deltaY == 0 && time == 1) {
return false;
}
return time >= Math.max(deltaX, deltaY);
}
}
The problem "Determine if a Cell Is Reachable at a Given Time" involves finding whether one can reach a destination (cell) from a starting point within a certain number of moves or time units in a grid. The solution is implemented in Java.
In this Java solution, the canReachDestination
method calculates whether the destination is reachable from the start position within the given time. Utilize the Math.abs()
function to compute the absolute differences in the x and y coordinates between the start and destination points, labeled as deltaX
and deltaY
, respectively.
The method first checks if the starting point is the same as the destination and the time is exactly 1. This specific condition returns false, which is a special case where the move isn't possible due to constraints probably intended to handle cases where staying in place costs a move.
For other general cases, compare the maximum of deltaX
and deltaY
to the available time. Return true if the time is greater than or equal to the maximum of these deltas, indicating that moving horizontally or vertically or both in any sequence could cover the distance in the given time.
Therefore:
- Determine the absolute differences between start and destination coordinates with
Math.abs()
. - Verify if starting at the destination with time equals 1 falls under no movement allowed.
- Return true if time supports at least the longest single-axis movement derived from the maximum of
deltaX
anddeltaY
.
bool can_arrive(int start_x, int start_y, int finish_x, int finish_y, int time_given){
int delta_x = abs(start_x - finish_x);
int delta_y = abs(start_y - finish_y);
if (delta_x == 0 && delta_y == 0 && time_given == 1) {
return false;
}
return time_given >= (delta_x > delta_y ? delta_x : delta_y);
}
This solution in C determines if it's possible to reach a cell (defined by its coordinates) from a starting point within a given time frame. The function can_arrive
accepts coordinates for the starting (start_x
, start_y
) and finishing positions (finish_x
, finish_y
), along with the time_given
.
The approach utilizes the Manhattan distance between the start and end points by calculating the absolute differences between their respective coordinates (delta_x
and delta_y
). The conditional check returns:
false
if both starting and ending points are the same and the time given is 1 - which under default conditions might incorrectly returntrue
.true
if thetime_given
is greater than or equal to the maximum ofdelta_x
anddelta_y
. This ensures checking the minimum time required to either shift entirely horizontally or vertically to reach the target cell.
Overall, this function effectively calculates if a direct move from the start to the finish within the stipulated time is feasible, assuming a unit step takes equivalent time horizontally or vertically.
var canReachDestination = function(startX, startY, finishX, finishY, time) {
var deltaX = Math.abs(startX - finishX);
var deltaY = Math.abs(startY - finishY);
if (deltaX === 0 && deltaY === 0 && time === 1) {
return false;
}
return time >= Math.max(deltaX, deltaY);
};
This JavaScript function, canReachDestination
, checks whether a destination cell in a grid can be reached from a starting position within a given time. The function takes as inputs the coordinates of the start (startX
, startY
) and finish (finishX
, finishY
) positions, along with the time
expected to reach the destination.
- Calculate the absolute differences between the start and finish coordinates for both X and Y dimensions using
Math.abs
. Store these differences indeltaX
anddeltaY
. - An initial condition checks if the start position is the same as the finish position and the provided time is exactly 1, in which case it returns
false
. - For all other cases, compare the provided time with the maximum of
deltaX
anddeltaY
values. If the time is greater than or equal to this value, returntrue
, indicating that reaching the destination is possible within the given time. Otherwise, returnfalse
.
This approach ensures that distance constraints are respected relative to the time allowed, effectively solving the problem of reachability in a grid environment based on Manhattan distance constraints.
class Solution:
def canReachInTime(self, start_x: int, start_y: int, end_x: int, end_y: int, time: int) -> bool:
dx = abs(start_x - end_x)
dy = abs(start_y - end_y)
if dx == 0 and dy == 0 and time == 1:
return False
return time >= max(dx, dy)
This Python solution addresses the problem of determining if a cell in a grid can be reached from a starting position within a given time. The canReachInTime
method takes starting coordinates (start_x
, start_y
), ending coordinates (end_x
, end_y
), and the maximum time allowed (time
). The method calculates the Manhattan distance between the start and end positions using the absolute differences dx
and dy
. If both dx
and dy
are zero and the time is only a single step, then reaching the cell isn't possible and it returns False
. Otherwise, the method checks if the time provided is greater than or equal to the maximum of dx
and dy
. If so, the cell is reachable, and the function returns True
; otherwise, it returns False
. This approach ensures that the shortest path in terms of grid steps is considered.
No comments yet.