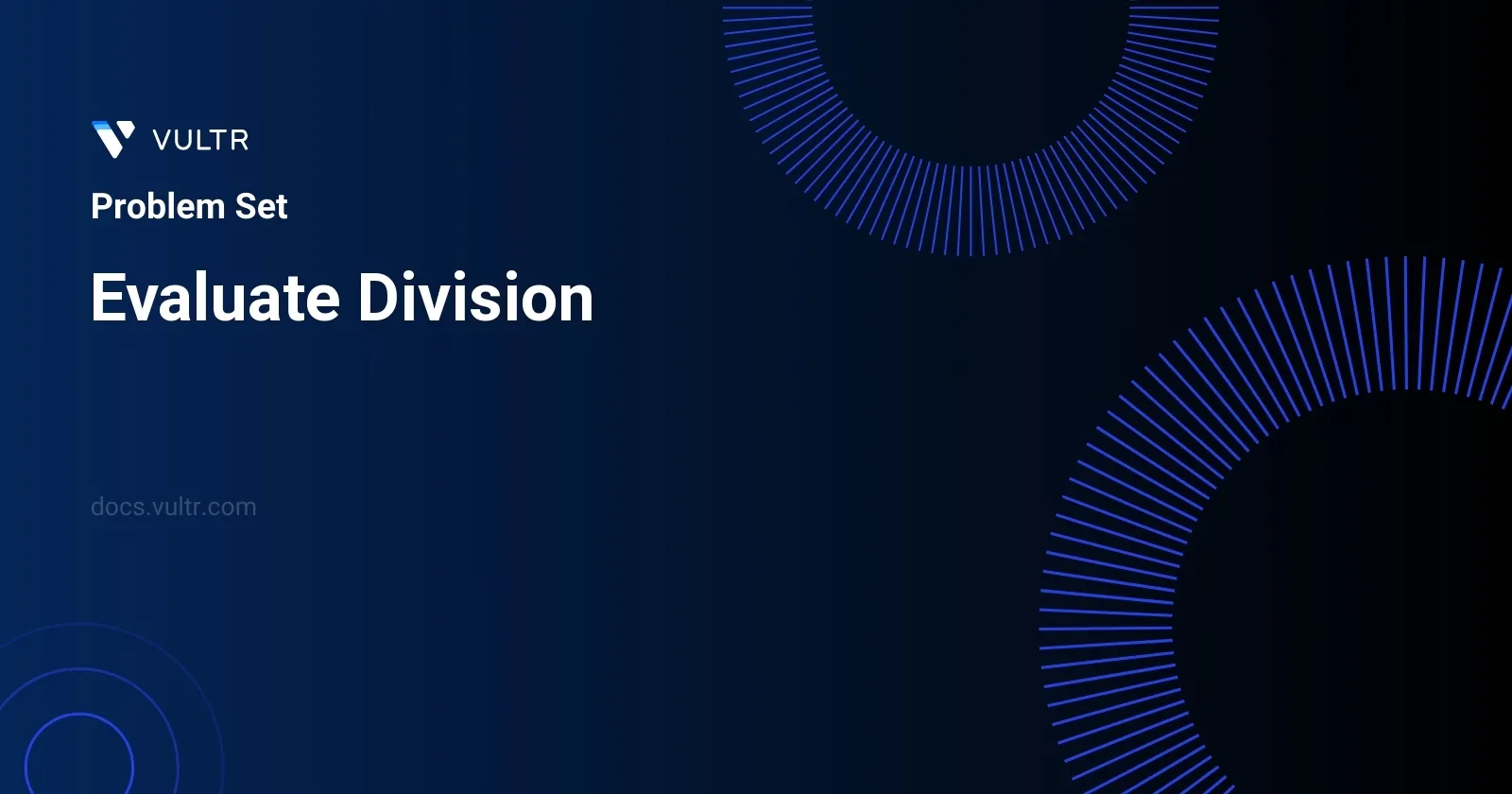
Problem Statement
In this problem, you are provided with two sets of data:
- An array of equations represented by pairs of variables, where each pair correlates to an equation depicting the division of the first variable by the second variable, alongside an array of values that specify the result of these divisions.
- Queries that require a set of results based on the given equations.
Each equation pair and its corresponding value concretely establish the relationship between two variables in the format Ai / Bi = values[i]
. Each variable (Ai
or Bi
) is denoted by a string. You are tasked with responding to several queries, each seeking the result of a division between two specified variables.
You need to compute the results for these queries based on the established relationships from the given equations. If a result cannot be conclusively determined based on the given data, the function should return -1.0
.
The main challenge lies in determining the results utilizing the transitive relationships derived from the equations. For example, if the equations establish relationships between a
and b
, as well as b
and c
, you may also need to deduce the relationship between a
and c
.
It's important to remember:
- The input data is always valid, and thus won't directly cause execution errors such as zero division.
- Variables not defined in the equations have no established relationships, meaning their division result is undefined, equating to
-1.0
.
Examples
Example 1
Input:
equations = [["a","b"],["b","c"]], values = [2.0,3.0], queries = [["a","c"],["b","a"],["a","e"],["a","a"],["x","x"]]
Output:
[6.00000,0.50000,-1.00000,1.00000,-1.00000]
Explanation:
Given: *a / b = 2.0*, *b / c = 3.0* queries are: *a / c = ?*, *b / a = ?*, *a / e = ?*, *a / a = ?*, *x / x = ?* return: [6.0, 0.5, -1.0, 1.0, -1.0 ] note: x is undefined => -1.0
Example 2
Input:
equations = [["a","b"],["b","c"],["bc","cd"]], values = [1.5,2.5,5.0], queries = [["a","c"],["c","b"],["bc","cd"],["cd","bc"]]
Output:
[3.75000,0.40000,5.00000,0.20000]
Example 3
Input:
equations = [["a","b"]], values = [0.5], queries = [["a","b"],["b","a"],["a","c"],["x","y"]]
Output:
[0.50000,2.00000,-1.00000,-1.00000]
Constraints
1 <= equations.length <= 20
equations[i].length == 2
1 <= Ai.length, Bi.length <= 5
values.length == equations.length
0.0 < values[i] <= 20.0
1 <= queries.length <= 20
queries[i].length == 2
1 <= Cj.length, Dj.length <= 5
Ai, Bi, Cj, Dj
consist of lower case English letters and digits.
Approach and Intuition
The solution to this problem involves constructing a graphical representation of the equations where each variable is a node and the division value forms the weight of the directed edge between the nodes. This method uses concepts from graph theory to solve the problem efficiently.
Steps:
Construct a directed graph:
- Variables in the equations act as nodes.
- An equation
Ai / Bi = value
introduces a directed edge fromAi
toBi
with weight equal tovalue
. - To enable inverse lookups, add an additional edge from
Bi
toAi
with the weight1 / value
.
For each query
(Cj, Dj)
, determine ifCj
can be reached fromDj
using any path in the constructed graph:- If
Cj
orDj
or both do not exist in the graph, the result is-1.0
since they are undefined based on the equations. - If they exist, use depth-first search (DFS) or breadth-first search (BFS) to find a path from
Cj
toDj
and calculate the cumulative product of the weights along this path.
- If
Graphical traversal for path finding:
- Using BFS, start from node
Cj
, traverse to all reachable nodes. IfDj
is reached, the cumulative product of the traversal gives the required division value. - A failure to reach
Dj
signifies no discernible relationship based on the given equations, returning-1.0
.
This approach fully explores the potential relationships between variables as represented by the graph structure, taking into account both direct and derived relationships, ensuring a comprehensive solution to the queries based on the dataset provided.
Solutions
- Java
- Python
class Solution {
public double[] calculateRatios(List<List<String>> equations, double[] values,
List<List<String>> queries) {
HashMap<String, Pair<String, Double>> groupMap = new HashMap<>();
// Building the map
for (int i = 0; i < equations.size(); i++) {
List<String> equation = equations.get(i);
String numerator = equation.get(0), denominator = equation.get(1);
double quotient = values[i];
mergeGroups(groupMap, numerator, denominator, quotient);
}
// Evaluating each query
double[] outcomes = new double[queries.size()];
for (int i = 0; i < queries.size(); i++) {
List<String> query = queries.get(i);
String numerator = query.get(0), denominator = query.get(1);
if (!groupMap.containsKey(numerator) || !groupMap.containsKey(denominator))
outcomes[i] = -1.0;
else {
Pair<String, Double> numeratorGroup = locate(groupMap, numerator);
Pair<String, Double> denominatorGroup = locate(groupMap, denominator);
String numeratorRoot = numeratorGroup.getKey();
String denominatorRoot = denominatorGroup.getKey();
Double numeratorWeight = numeratorGroup.getValue();
Double denominatorWeight = denominatorGroup.getValue();
if (!numeratorRoot.equals(denominatorRoot))
outcomes[i] = -1.0;
else
outcomes[i] = numeratorWeight / denominatorWeight;
}
}
return outcomes;
}
private Pair<String, Double> locate(HashMap<String, Pair<String, Double>> groupMap, String item) {
if (!groupMap.containsKey(item))
groupMap.put(item, new Pair<String, Double>(item, 1.0));
Pair<String, Double> entry = groupMap.get(item);
// Correcting inconsistencies dynamically
if (!entry.getKey().equals(item)) {
Pair<String, Double> newGroup = locate(groupMap, entry.getKey());
groupMap.put(item, new Pair<String, Double>(
newGroup.getKey(), entry.getValue() * newGroup.getValue()));
}
return groupMap.get(item);
}
private void mergeGroups(HashMap<String, Pair<String, Double>> groupMap, String num, String denom, Double ratio) {
Pair<String, Double> numEntry = locate(groupMap, num);
Pair<String, Double> denomEntry = locate(groupMap, denom);
String numRoot = numEntry.getKey();
String denomRoot = denomEntry.getKey();
Double numWeight = numEntry.getValue();
Double denomWeight = denomEntry.getValue();
if (!numRoot.equals(denomRoot)) {
groupMap.put(numRoot, new Pair<String, Double>(denomRoot,
denomWeight * ratio / numWeight));
}
}
}
The Java solution provided tackles the problem of evaluating the division results for given queries based on a list of known equations and their corresponding values. The solution implements a Union-Find data structure with path compression and balancing to answer the division queries efficiently.
- The equations and values initialize a
HashMap
with key-value pairs representing divisions and their results. Each variable in the equation is treated as a node in a graph. - The key part of the solution is to maintain a correctly updated structure where each node points to its parent node and the ratio to the parent node is updated accordingly. This is done through the
mergeGroups
method. - For each query, two main operations occur: locating the group's root for both the numerator and the denominator using the
locate
method, which updates the group relationship on-the-fly to ensure that all links point directly to the root (path compression). - Comparison of the roots for the numerator and denominator:
- If they are different, the answer is -1.0, representing that the division cannot be evaluated given the input equations.
- If they are the same, the division result is computed as the ratio of the weight of the numerator node to the weight of the denominator node.
- The result for each query is stored in an array and returned.
By leveraging the principles of the Union-Find structure, the solution efficiently manages to perform and retrieve the required calculations dynamically, ensuring that each variable is directly connected to its group representative, thereby reducing the complexity of finding the root to almost constant time in practice, thanks to path compression. All of this is managed while keeping the space complexity in check with the use of a simple hash map for the Union-Find structure. This approach guarantees efficient handling of complex query sets with varying dependencies.
class Solution:
def computeEquations(self, equations: List[List[str]], values: List[float], queries: List[List[str]]) -> List[float]:
group_mapping = {}
def locate(node_id):
if node_id not in group_mapping:
group_mapping[node_id] = (node_id, 1)
parent_id, node_factor = group_mapping[node_id]
if parent_id != node_id:
superior_parent, parent_factor = locate(parent_id)
group_mapping[node_id] = (superior_parent, node_factor * parent_factor)
return group_mapping[node_id]
def merge(node1, node2, value_ratio):
parent1, factor1 = locate(node1)
parent2, factor2 = locate(node2)
if parent1 != parent2:
group_mapping[parent1] = (parent2, factor2 * value_ratio / factor1)
for (numerator, denominator), value in zip(equations, values):
merge(numerator, denominator, value)
results = []
for (numerator, denominator) in queries:
if numerator not in group_mapping or denominator not in group_mapping:
results.append(-1.0)
else:
parent_num, factor_num = locate(numerator)
parent_denom, factor_denom = locate(denominator)
if parent_num != parent_denom:
results.append(-1.0)
else:
results.append(factor_num / factor_denom)
return results
The "Evaluate Division" solution employs the union-find algorithm to solve division equations represented by ratios and to handle queries based on those equations. The code is structured in Python, using nested functions within the Solution
class to maintain a clean and logical flow. The process is as follows:
- Initialize a
group_mapping
dictionary to keep track of each node’s parent and its relative factor. - Define a
locate
function that performs path compression. This function ensures that every node directly points to its representative parent while keeping the relative multiplication factor updated correctly. - Implement a
merge
function to union two nodes with their given division value. This function updates the root parent and recalculates the multiplication factor for the representative parent. - Iterate through each equation in the list and merge the nodes according to the given values.
- Process each query by first checking if the nodes exist in
group_mapping
. If either node is missing, append-1.0
to the results list indicating an impossible query. - For existing nodes, find their representative parents. If they belong to different groups, append
-1.0
. Otherwise, calculate the division result by dividing their respective factors and append the result to the list. - Return the list of results.
The union-find structure efficiently manages the merging and locating operations, making the code effective for complex datasets. This approach ensures that each query is processed in nearly constant time, once the initial unions are formed, thereby providing a very efficient solution to the problem statement.
No comments yet.