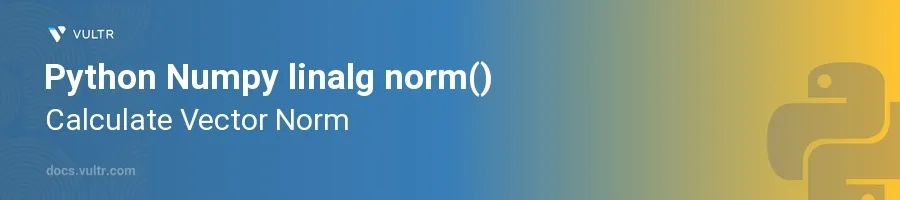
Introduction
The numpy.linalg.norm()
function in Python is a powerful tool provided by the NumPy library, primarily used to calculate the norm of a vector or matrix. This method supports various norms, making it extremely versatile for scientific computing. Norm calculations are fundamental in numerous mathematical and engineering computations, particularly in operations involving vectors and matrices.
In this article, you will learn how to leverage the numpy.linalg.norm()
function to compute vector norms. Discover how to apply this function in different scenarios and understand the impact of various norm types on the computation.
Calculating Norms of Vectors
Calculate the Euclidean Norm (L2 Norm)
Import the NumPy library.
Define a vector for which the norm is to be calculated.
Use the
norm()
function to compute the Euclidean norm.pythonimport numpy as np vector = np.array([3, 4]) norm = np.linalg.norm(vector) print(norm)
This code snippet calculates the Euclidean norm (also known as L2 norm) for the vector
[3, 4]
. The result is5.0
, which is the straight-line distance from the origin to the point represented by the vector.
Compute the Manhattan Norm (L1 Norm)
Set up a vector whose Manhattan norm you wish to find.
Using
numpy.linalg.norm()
, specify theord=1
parameter to calculate the L1 norm.pythonimport numpy as np vector = np.array([3, -4]) norm = np.linalg.norm(vector, ord=1) print(norm)
Here, the Manhattan norm (or L1 norm) for the vector
[3, -4]
is calculated. The L1 norm sums the absolute values of the components, resulting in7.0
.
Use the Infinity Norm
Prepare a vector to calculate the infinity norm.
Specify
ord=np.inf
within thenorm()
function to execute this computation.pythonimport numpy as np vector = np.array([-3, 7, 5]) norm = np.linalg.norm(vector, ord=np.inf) print(norm)
For the vector
[-3, 7, 5]
, the infinity norm calculates the maximum absolute value among the vector's components, which is7.0
in this case.
Conclusion
The norm()
function from the numpy.linalg
module is essential for calculating various types of norms for vectors and matrices. Whether it's the straightforward Euclidean norm, the summative Manhattan norm, or the maximum-seeking infinity norm, this function is equipped to handle them efficiently. By mastering the numpy.linalg.norm()
function, streamline complex mathematical tasks involving vector and matrix operations, ultimately refining analytical capabilities and computational accuracy in Python projects.
No comments yet.