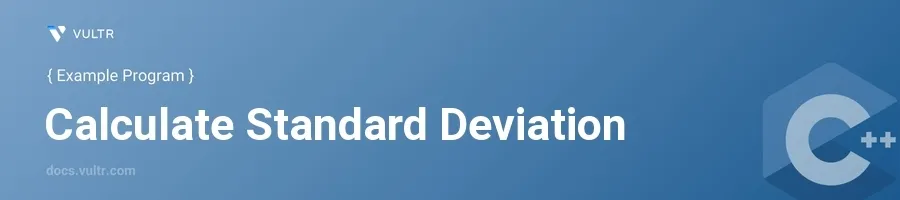
Introduction
Standard deviation is a crucial statistical measure that quantifies the amount of variation or dispersion in a set of data values. In C++, calculating standard deviation involves a series of computational steps including mean calculation, variance summation, and, ultimately, the square root of the variance.
In this article, you will learn how to implement a C++ program to calculate the standard deviation for a series of numbers. You will follow through a detailed explanation of the necessary calculations and see practical examples where these concepts are applied using C++ code.
Understanding Standard Deviation
Theory Behind Standard Deviation
- Calculate the mean (the average of the numbers).
- Compute the variance for each number (subtract the mean and square the result).
- Calculate the average of these squared differences (this is the variance).
- Get the square root of the variance to find the standard deviation.
Example Calculations
Consider a dataset: {4, 9, 11, 12, 17}
First, calculate the mean:
[ \text{Mean} = \frac{(4 + 9 + 11 + 12 + 17)}{5} = 10.6 ]
Next, compute variances and then the average:
[ \text{Variances} = [(4-10.6)^2, (9-10.6)^2, (11-10.6)^2, (12-10.6)^2, (17-10.6)^2] ]
Calculate the mean of variances and then the square root to find the standard deviation.
Implementing Standard Deviation in C++
Simple Implementation of Standard Deviation
Start by including necessary standard libraries for input-output operations and mathematical functions.
cpp#include <iostream> #include <vector> #include <cmath> // For the sqrt() function
Use a function
calculateSD
that takes a vector of numbers and returns their standard deviation:cppdouble calculateSD(const std::vector<int>& data) { double sum = 0.0; double mean, standardDeviation = 0.0; for (int value : data) { sum += value; } mean = sum / data.size(); for (int value : data) { standardDeviation += pow(value - mean, 2); } return sqrt(standardDeviation / data.size()); }
This function computes the mean of the dataset, then sums the squared differences from the mean, and finally returns the square root of the average of these squared differences.
Main Program to Demonstrate Usage
Define the
main()
function where you create a list of values, calculate, and output the standard deviation:cppint main() { std::vector<int> data = {4, 9, 11, 12, 17}; double SD = calculateSD(data); std::cout << "Standard Deviation = " << SD; return 0; }
Running this program will calculate and display the standard deviation of the numbers in the
data
vector.
Conclusion
Calculating the standard deviation in C++ involves several mathematical operations that C++ handles efficiently through its standard libraries. By following the described program structure, you effortlessly calculate the standard deviation for any dataset provided. Implementing such statistical calculations allows for deeper data analysis and understanding in your C++ projects with direct applications in fields ranging from finance to machine learning. Apply the provided program and adjust it to fit larger datasets or integrate it into complex data analysis software.
No comments yet.