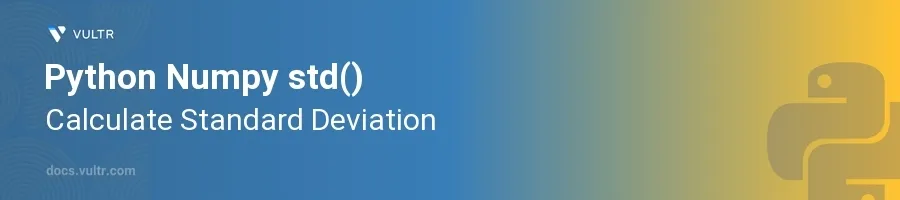
Introduction
The std()
function in the Numpy library is a versatile tool designed for computing the standard deviation, a critical statistical metric used widely across data analysis, science, and engineering to quantify the amount of variation or dispersion in a set of data points. Understanding how to use this function is essential for tasks that involve data normalization, optimization, and error analysis.
In this article, you will learn how to effectively utilize the std()
function to calculate the standard deviation in various contexts. Explore how to use this function with different data types, consider the impact of parameters that alter its behavior, and apply it to real-world data analysis scenarios.
Understanding Standard Deviation
Standard deviation is a measure that is used to quantify the amount of variation or dispersion a set of data points has from the average (mean) of the data. In simpler terms, it shows how much the data varies from the mean.
Key Concepts of Standard Deviation
- A low standard deviation indicates that the data points tend to be close to the mean.
- A high standard deviation indicates that the data points are spread out over a larger range of values.
Using Numpy's std() Function
Numpy's std()
function calculates the standard deviation of an array-like data structure. This section covers the basics and dives deeper into more complex applications.
Calculating Basic Standard Deviation
Import the Numpy library.
Create an array or a list of numerical data.
Apply the
std()
function to compute the standard deviation.pythonimport numpy as np data = [4, 8, 15, 16, 23, 42] stddev = np.std(data) print("Standard Deviation:", stddev)
This code snippet calculates the standard deviation of the data list. It helps determine how spread out the numbers are in the list.
Impact of the ddof
Parameter
Understand that
ddof
stands for Delta Degrees of Freedom. The default value is0
.Set the
ddof
to1
to use the sample standard deviation formula instead of the population standard deviation.pythonsample_stddev = np.std(data, ddof=1) print("Sample Standard Deviation:", sample_stddev)
Changing
ddof
to 1 adjusts the divisor during calculation fromN
(number of elements) toN-1
, which gives an unbiased estimator of the variance for a sample.
Standard Deviation on Multidimensional Arrays
Create a 2D array.
Use the
axis
parameter to specify the axis (0 for columns, 1 for rows) along which the standard deviation should be calculated.pythonmatrix = np.array([[1, 2], [3, 4], [5, 6]]) col_stddev = np.std(matrix, axis=0) row_stddev = np.std(matrix, axis=1) print("Column-wise Standard Deviation:", col_stddev) print("Row-wise Standard Deviation:", row_stddev)
Specifying the axis helps in finding the standard deviation across the specified dimension of the array.
Practical Applications of Standard Deviation
Beyond basic statistical analysis, the standard deviation is vital in fields like finance, quality control, and physics. Some practical applications include:
- In finance, it is used to measure the volatility of stock prices.
- In quality control, determining how much manufacturing processes deviate from performance standards.
- In physics, it can indicate the precision of measurements.
Using Standard Deviation in Data Normalization
Normalize datasets using the mean and standard deviation to standardize data before applying machine learning models.
pythonnormalized_data = (data - np.mean(data)) / np.std(data) print("Normalized Data:", normalized_data)
This snippet shows a common method of data preprocessing to ensure each feature contributes equally to the analysis, particularly important in machine learning algorithms sensitive to feature scaling.
Conclusion
Mastering the std()
function in Numpy enhances your abilities in statistical analysis by providing the tools to quantify data dispersion efficiently. Apply this function across various data sets and scenarios to gain deeper insights and make more informed decisions in your data-driven projects. By following the examples and applications discussed, you bolster not only your data analysis toolkit but also your capability to handle and interpret complex datasets effectively.
No comments yet.