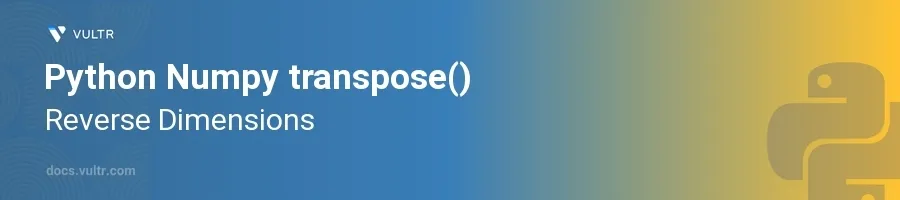
Introduction
The transpose()
function in NumPy is an essential tool for data manipulation and analysis, especially when dealing with arrays of multiple dimensions. This function reverses or permutes the axes of an array according to a specified tuple of axes, making it a powerful utility for reshaping and transforming data to fit the needs of various algorithms and operations.
In this article, you will learn how to leverage the transpose()
function effectively in different contexts. Explore its application in reversing the dimensions of matrices, swapping axes, and the nuances of using it with higher-dimensional data structures.
Understanding the transpose() Function
Basic Usage of transpose()
Import the NumPy package.
Create a two-dimensional array.
Apply the
transpose()
function without any additional arguments.pythonimport numpy as np array_2d = np.array([[1, 2], [3, 4]]) transposed_array = array_2d.transpose() print(transposed_array)
This example demonstrates the simplest form of transposition, where a 2x2 matrix's rows and columns are interchanged. The output would display the matrix with flipped axes.
Using transpose() with Arguments
Understand that
transpose()
can accept a tuple of integers representing the new permutation of axes.Define a three-dimensional array.
Specify an axes tuple to rearrange the dimensions.
pythonimport numpy as np array_3d = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]]) transposed_array = array_3d.transpose((1, 0, 2)) print(transposed_array)
In this example, the axes are rearranged where the second axis (index 1) becomes the first, the first axis (index 0) becomes the second, and the third axis (index 2) remains in place. This flexibility in specifying the axes order allows for complex data manipulation tasks.
Advanced Applications of transpose()
Integrating transpose() with Linear Algebra Operations
Apply the transpose to perform matrix computations.
Combine it with functions like
np.dot()
for matrix multiplication.pythonimport numpy as np matrix_a = np.array([[1, 2], [3, 4]]) matrix_b = np.array([[5, 6], [7, 8]]) product = np.dot(matrix_a, matrix_b.transpose()) print(product)
Matrix multiplication often requires that the dimensions of the matrices be aligned correctly, and transposing one of the matrices can ensure these requirements are met.
Using transpose() in Image Processing
Recognize the use of
transpose()
in image data manipulation.Rearrange the dimensions of an image array as needed by certain image processing libraries or techniques.
pythonimport numpy as np image_data = np.random.rand(100, 100, 3) # Simulate a 100x100 RGB image transposed_image = image_data.transpose((1, 0, 2)) print(transposed_image.shape)
By transposing the image data of dimensions
(Height, Width, Channels)
to(Width, Height, Channels)
, you adapt the array structure to different processing algorithms or visualization frameworks that might expect a specific axes order.
Conclusion
The transpose()
function in NumPy offers a significant advantage in data manipulation, enabling the reordering of axes for arrays of any shape and size. Whether you're working with two-dimensional matrices or complex multi-dimensional data arrays, understanding how to apply transpose()
to reverse or permute axes appropriately empowers you to handle data more effectively in Python. Harness these techniques to simplify data transformation tasks and enhance your numerical computation projects.
No comments yet.