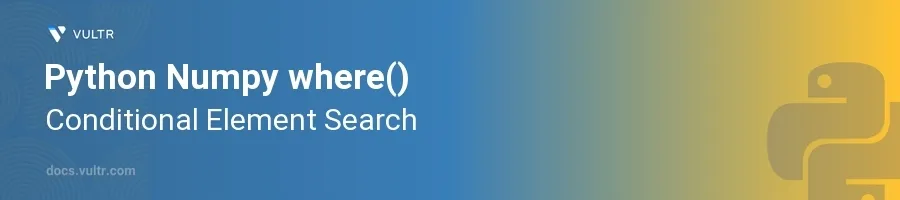
Introduction
The numpy.where()
function is a versatile tool in the Python numpy library, primarily used to locate elements or indices in an array that meet certain conditions. It is immensely useful in data processing where decisions need to be based on criteria, helping to extract or manipulate data efficiently.
In this article, you will learn how to utilize the numpy.where()
function to perform conditional searches in arrays. Explore the various ways to apply conditions, handle compound conditions, and leverage numpy.where()
in practical data analysis scenarios.
Basic Usage of numpy.where()
Finding Indices of Elements that Meet a Condition
Import numpy and create an array.
Use
numpy.where()
to find indices of elements that satisfy a specific condition.pythonimport numpy as np data = np.array([1, 2, 3, 4, 5, 6, 7, 8]) result = np.where(data > 4) print("Indices where data is greater than 4:", result)
In this code,
numpy.where()
checks the conditiondata > 4
and returns the indices where this condition isTrue
.
Using Conditions to Filter Data
Create an array based on the results from
numpy.where()
.Extract elements based on the found indices.
pythonfiltered_data = data[result] print("Filtered data:", filtered_data)
This snippet extracts the elements from
data
using the indices found bynumpy.where()
, displaying the data that meets the condition.
Handling Compound Conditions
Applying Multiple Conditions
Define an array with more complex criteria.
Use logical operators like
&
(and) and|
(or) to combine conditions.pythonresult = np.where((data > 2) & (data < 6)) print("Indices with data between 2 and 6:", result)
This example searches for values that are greater than 2 and less than 6. The result shows indices of
data
where these conditions are bothTrue
.
Using Conditions with Multiple Arrays
Create additional arrays.
Compare elements across these arrays using
numpy.where()
.pythona = np.array([1, 2, 3, 4, 5]) b = np.array([5, 4, 3, 2, 1]) result = np.where(a < b) print("Indices where a < b:", result)
Here, array
a
is compared to arrayb
element-wise, locating indices where the conditiona < b
holds true.
Practical Applications
Replacing Elements Based on Condition
Use
numpy.where()
to substitute certain elements in an array.Specify a replacement for elements that meet the condition.
pythonmodified_data = np.where(data > 4, data, -1) print("Data with replacements:", modified_data)
This operation replaces elements in
data
where the conditiondata > 4
is not met with-1
, while keeping the original values which meet the condition.
Using numpy.where() in Data Analysis
Analyze arrays to extract or modify data effectively in a dataset.
Apply conditions relevant to the analysis to filter or change records.
pythonconditions_applied = np.where((data % 2 == 0) | (data > 5)) print("Filtered indices for analysis:", conditions_applied)
This example demonstrates the use of
numpy.where()
to identify indices for data analysis, considering data that is either even or greater than 5.
Conclusion
The numpy.where()
function in Python offers a robust way to perform conditional element searches within arrays, enabling efficient and selective data handling. From simple condition checks to complex analyses involving multiple conditions and arrays, numpy.where()
enhances data manipulation capabilities in numpy. Whether modifying, replacing, or extracting data, utilizing the techniques discussed here streamlines processes in data analysis and ensures your projects are more dynamic and responsive to conditions. Adopt this function to make your data workflows more powerful and flexible.
No comments yet.