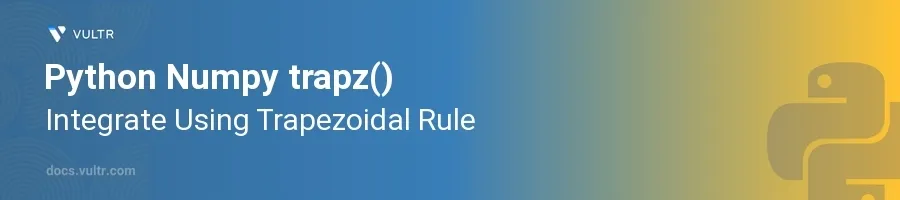
Introduction
The numpy.trapz()
function in Python leverages the trapezoidal rule to compute the definite integral of an array's data points. This method is a cornerstone in numerical integration, providing a straightforward approach to approximate the integral of data that is sampled discretely. In situations where an analytical solution to an integral is cumbersome or impossible, numerical methods like the trapezoidal rule come in handy, especially in fields such as physics, engineering, and economics.
In this article, you will learn how to harness the trapz()
function effectively. Explore its applications through several examples that cover basic integration of arrays, use with unevenly spaced data, and integration along specific axes in multidimensional arrays.
Basic Integration with trapz()
Computing the Integral of a Simple Array
Import the NumPy library.
Define an array representing y-values of a function over evenly spaced x-values.
Compute the integral using
numpy.trapz()
.pythonimport numpy as np y = np.array([0, 1, 2, 3, 4]) integral = np.trapz(y) print("Integral:", integral)
This example calculates the integral of the y-values assuming they are spaced at unit intervals. Since no x-values are specified, it defaults to unit spacing.
Integration Over a Specified Range of x-values
Specify the x-values corresponding to each y-value.
Pass both the y-values and the x-values to the
numpy.trapz()
function.pythonx = np.array([0, 1, 2, 3, 4]) integral = np.trapz(y, x) print("Integral:", integral)
Here, the
trapz()
function uses the x-values provided to determine the intervals over which to compute the integral.
Integration with Unevenly Spaced Data
Calculating Integrals with Non-Uniform Spacing
Understand that the trapezoidal rule can handle irregular intervals.
Define an array of x-values that are not evenly spaced.
Use these irregular x-values for numerical integration.
pythonx_non_uniform = np.array([0, 0.5, 1.5, 3, 4]) integral_non_uniform = np.trapz(y, x_non_uniform) print("Integral with non-uniform x-values:", integral_non_uniform)
In this example, the non-uniform x-values represent the varying intervals at which the data points are sampled.
numpy.trapz()
calculates the area of trapezoids of different widths accordingly.
Advanced Applications
Integration Along a Specific Axis in Multidimensional Data
Grasp that
numpy.trapz()
can integrate along any given axis in a multidimensional array.Create a 2D array where each row might represent a different function or dataset.
Set the axis parameter to determine the axis along which to integrate.
pythondata = np.array([[0, 1, 2], [2, 3, 4], [4, 5, 6]]) integral_axis_0 = np.trapz(data, axis=0) integral_axis_1 = np.trapz(data, axis=1) print("Integral along axis 0:", integral_axis_0) print("Integral along axis 1:", integral_axis_1)
This scenario involves a 2D array where integration is performed along each column (
axis=0
) and each row (axis=1
) separately.
Conclusion
The numpy.trapz()
function provides a robust method for numerical integration using the trapezoidal rule. It is well-suited for tasks ranging from simple to complex integrations, particularly when dealing with sampled or discrete data that are irregularly spaced. By mastering trapz()
, programming efficiency and the ability to handle diverse analytical tasks in scientific computing, data analysis, and beyond are significantly enhanced. Implement these techniques to simplify and speed up numerical integration processes in your data-driven projects.
No comments yet.