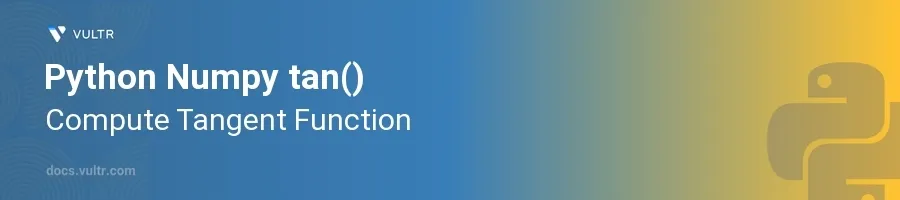
Introduction
The tan()
function in Python's NumPy library computes the tangent of a given angle. This trigonometric function is essential in fields such as engineering, physics, and mathematics, where angles and their properties are fundamental. As part of the broader array of mathematical tools provided by NumPy, tan()
plays a critical role in performing vectorized operations across arrays, optimizing calculations over individual elements, and significantly boosting computational efficiency.
In this article, you will learn how to use the tan()
function to compute the tangent of angles in both degrees and radians. You will also explore practical applications involving array operations with the tan()
function. By mastering this tool, you enhance your capabilities in handling complex mathematical computations using Python.
Computing Tangent for Single Values
Convert Degrees to Radians
Recognize that NumPy's
tan()
function expects the angle in radians.Convert degrees to radians using the
deg2rad()
or direct multiplication bypi/180
.pythonimport numpy as np degrees = 45 radians = np.deg2rad(degrees) tan_value = np.tan(radians) print("Tangent of 45 degrees:", tan_value)
This code converts 45 degrees into radians and computes the tangent. The tangent of 45 degrees is 1 (or very close to 1, depending on the precision).
Use Direct Radian Values
Apply the
tan()
function directly if the angle is already in radians.pythonradians = np.pi / 4 # π/4 radians is 45 degrees tan_value = np.tan(radians) print("Tangent of π/4 radians:", tan_value)
Here, π/4 radians is equivalent to 45 degrees, and the tangent is calculated directly.
Applying tan() on Arrays
Compute Tangent for an Array of Angles
Use the
tan()
function to compute the tangent for each angle in an array of radian values.pythonradians_array = np.array([0, np.pi/6, np.pi/4, np.pi/3, np.pi/2]) tan_values = np.tan(radians_array) print("Tangent values:", tan_values)
This code snippet computes the tangent for an array of angles: 0, π/6, π/4, π/3, and π/2 radians. The results are stored and printed as an array of tangent values.
Handle Large Arrays Efficiently
Utilize the vectorized nature of NumPy's
tan()
to handle large-scale computations efficiently.pythonlarge_radians_array = np.linspace(0, 2*np.pi, 1000) # 1000 angles from 0 to 2π tan_values = np.tan(large_radians_array) print("Tangent values for 1000 angles:", tan_values[:5], "...") # Display first 5 for brevity
In this example, tangent values are computed for 1000 angles evenly spaced between 0 and 2π. This demonstrates
tan()
's ability to efficiently handle large arrays, typical in scientific computing.
Practical Applications
Creating Wave Patterns
Explore the use of the
tan()
function in generating wave patterns, a common requirement in signal processing.pythonx = np.linspace(-2*np.pi, 2*np.pi, 1000) y = np.tan(x) # Visualization code would typically follow here to plot `y` vs `x`.
By calculating the tangent for a range of x-values, you generate a wave pattern which can be visualized using libraries like matplotlib.
Conclusion
The tan()
function from NumPy is a versatile tool for computing tangent values in Python, coping effectively with both single values and large arrays. Understanding how to convert degrees to radians, applying the function on arrays, and utilizing its results in practical scenarios like wave pattern generation empowers you to perform efficient and accurate trigonometric calculations. Leverage the tan()
function in your projects to handle complex mathematical tasks with ease. This knowledge not only enhances computational efficiency but also broadens the application scope of your programming skills.
No comments yet.