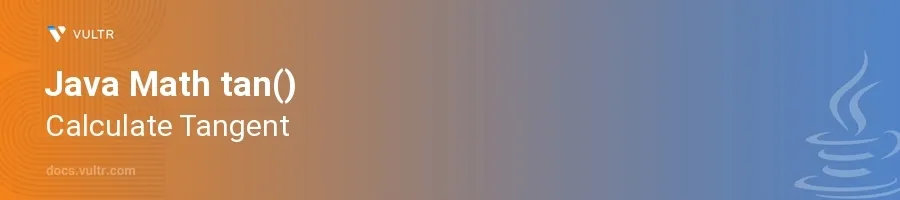
Introduction
The Math.tan()
method in Java is a part of the Java Math class and is used to calculate the trigonometric tangent of an angle, provided in radians. This method is crucial in geometrical calculations, simulations, and any applications where trigonometry plays a role.
In this article, you will learn how to effectively utilize the Math.tan()
function to calculate the tangent of various angles. Discover the comprehensive utilizations of this method across different programming scenarios while ensuring precise trigonometric calculations.
Using Math.tan() Method
Calculate Tangent of a Specific Angle
Convert the angle from degrees to radians as
Math.tan()
requires the angle in radians.Use
Math.tan()
to find the tangent of the angle.javadouble degrees = 45; // Angle in degrees double radians = Math.toRadians(degrees); // Convert to radians double tangent = Math.tan(radians); // Compute tangent System.out.println("Tangent of " + degrees + " degrees is: " + tangent);
This code snippet calculates the tangent of 45 degrees. Conversion of degrees to radians is handled by
Math.toRadians()
, ensuring that the input toMath.tan()
is correctly formatted.
Handle Extreme Angles
Be vigilant in using angles that could lead to significant calculation errors.
Apply
Math.tan()
to angles like 90 degrees which are undefined in tangent operations and observe the output.javadouble degrees90 = 90; // 90 degrees angle double radians90 = Math.toRadians(degrees90); // Convert to radians double tangent90 = Math.tan(radians90); // Compute tangent of 90 degrees System.out.println("Tangent of 90 degrees is: " + tangent90);
The tangent of 90 degrees tends to infinity, and depending on the precision and the computation limits of the system, it might return a very large number. This output provides deep insights into system behavior for critical values.
Experiment with Negative Angles
Understand that negative angles are also valid inputs and can be useful in certain scenarios.
Calculate the tangent of a negative angle using the same method.
javadouble negDegrees = -45; // Negative angle double negRadians = Math.toRadians(negDegrees); // Convert to radians double negTangent = Math.tan(negRadians); // Compute tangent of negative angle System.out.println("Tangent of " + negDegrees + " degrees is: " + negTangent);
For a -45 degrees angle, this code snippet effectively calculates the negative tangent, demonstrating the method's flexibility with negative inputs.
Advanced Usage of Math.tan()
Periodicity Considerations
Remember that the tangent function is periodic with a period of ( \pi ) (180 degrees).
Use this property for optimization by reducing the input angle modulo 180 degrees before conversion and calculation.
javadouble degrees = 450; // An angle greater than 360 degrees double adjustedDegrees = degrees % 360; // Adjust for periodicity double radians = Math.toRadians(adjustedDegrees); // Convert to radians double tangent = Math.tan(radians); // Calculate tangent System.out.println("Tangent of " + degrees + " degrees is (periodic adjustment): " + tangent);
Adjusting for periodicity avoids unnecessary recalculations and can improve computational efficiency, making use of the cyclic nature of the tangent function.
Conclusion
The Math.tan()
method in Java is a versatile tool for computing the tangent of angles provided in radians. By converting degrees to radians, handling edge cases, and optimizing with periodicity, harness this function's full potential in your Java applications. Whether for simple angle computations or complex trigonometric calculations, Math.tan()
offers a straightforward approach to achieving precise results in any project requiring trigonometric functionality. Implement these techniques to ensure your calculations are both accurate and efficient.
No comments yet.