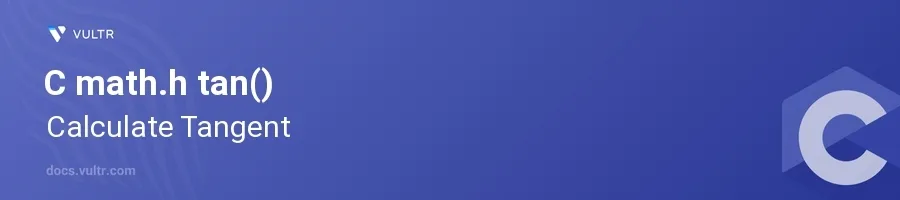
Introduction
The tan()
function from the C Standard Library's <math.h>
header file is an essential tool for calculating the tangent of an angle provided in radians. This function is widely used in various applications that involve trigonometric calculations, from engineering simulations to computer graphics transformations.
In this article, you will learn how to accurately utilize the tan()
function to compute the tangent of various angles. Explore practical examples that demostrate how to integrate this function into your C programming projects efficiently.
Utilizing tan() in C
Calculate Tangent of an Angle
Include the
<math.h>
header to access thetan()
function.Initialize a variable to store the angle in radians.
Call
tan()
with this angle.Output the result using
printf()
.c#include <math.h> #include <stdio.h> int main() { double angle = M_PI / 4; // Angle in radians (45 degrees) double tangent = tan(angle); printf("The tangent of 45 degrees is: %f\n", tangent); return 0; }
This example calculates the tangent of 45 degrees (π/4 radians). The output should closely approximate
1.000000
, since the tangent of 45 degrees is 1.
Handling Angles That Result in Undefined Tangents
Recognize that angles like π/2 (90 degrees), 3π/2 (270 degrees), etc., lead to undefined tangents.
Implement error checking before using the result if your application might encounter such angles.
c#include <math.h> #include <stdio.h> #include <errno.h> #include <fenv.h> int main() { double angle = M_PI / 2; // 90 degrees double tangent = tan(angle); if (fetestexcept(FE_INVALID)) { printf("Undefined tangent encountered.\n"); } else { printf("The tangent of 90 degrees is: %f\n", tangent); } return 0; }
In this script, error-checking mechanisms detect if the operation leads to an undefined result, handling the particular scenario gracefully.
Explore Tangent Values for Diverse Angles
Use a loop to compute and print the tangent for a range of angles.
c#include <math.h> #include <stdio.h> int main() { for (double angle = 0; angle <= M_PI; angle += M_PI / 4) { double tangent = tan(angle); printf("Tangent of %f radians is %f\n", angle, tangent); } return 0; }
This code sequentially calculates the tangent for angles 0, π/4, π/2, 3π/4, and π radians, thus covering an interesting spectrum from 0 to 180 degrees.
Conclusion
Harness the power of the tan()
function in the C programming language to compute tangents of angles efficiently. With proper initialization and error checking, you can integrate this functionality into a wide array of mathematical and practical tasks. Make sure you handle angles leading to undefined results to avoid runtime errors in critical applications. By following the examples illustrated, you are equipped to enhance your programs with precise trigonometric calculations.
No comments yet.