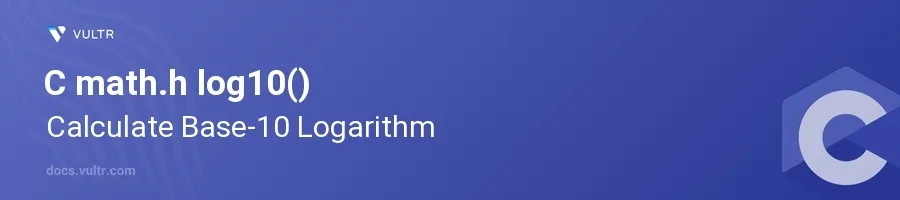
Introduction
The log10()
function from C's math library is a widely used mathematical function for calculating the base-10 logarithm of a number. This function is essential in scientific computing, engineering, and any domain where logarithmic scales or decibels are utilized for data measurement and analysis.
In this article, you will learn how to effectively use the log10()
function in various programming scenarios. You'll explore its fundamental application in calculating the logarithm for positive numbers and managing different edge cases, including negative numbers and zero.
Utilizing log10() in C
Calculating Logarithm of Positive Numbers
Include the math header and standard input-output headers in your code.
Initialize a positive number.
Call
log10()
to compute the logarithm.Print the result.
c#include <stdio.h> #include <math.h> int main() { double number = 100.0; double result = log10(number); printf("The base-10 logarithm of %.2f is %f\n", number, result); return 0; }
In this example, the logarithm of
100.0
is calculated usinglog10()
. The function returns2.0
since10^2 = 100
.
Handling Special Cases
Logarithm of Zero
Understand that the logarithm of zero is not defined in the real number system and results in negative infinity in C.
Execute
log10()
on zero.Observe the result.
cdouble zero_log = log10(0); printf("The base-10 logarithm of 0 is %f\n", zero_log);
This code will output negative infinity, denoted typically by
-inf
in C, indicating an undefined or unbounded result.
Negative Numbers
Know that the logarithm of a negative number is not defined in the real number system and typically results in a NaN (Not a Number) error.
Attempt computing the logarithm of a negative number.
Check the output.
cdouble negative_log = log10(-10); printf("The base-10 logarithm of -10 is %f\n", negative_log);
Computing the base-10 logarithm of
-10
generates a NaN result because logarithms of negative numbers are not defined in the field of real numbers.
Conclusion
The log10()
function is a versatile tool in the C programming language for computing the base-10 logarithm. Mastery of this function opens the door to more intricate calculations in various technical fields that use logarithmic measurements. Implement the techniques discussed, keeping in mind how the function behaves with non-positive numbers to ensure your code handles all possible input scenarios effectively.
No comments yet.