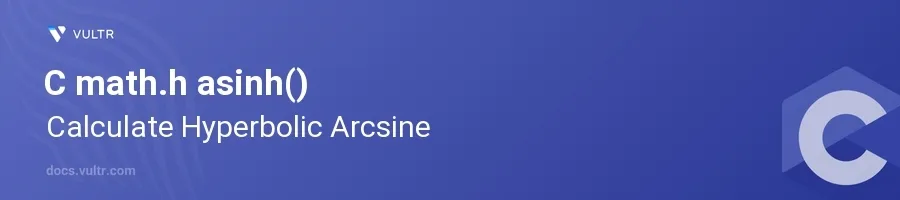
Introduction
The asinh()
function in C is part of the <math.h>
library and calculates the hyperbolic arcsine of a given number. This function is crucial for computations involving hyperbolic functions, typically seen in engineering, physics, and hyperbolic geometry.
In this article, you will learn how to effectively use the asinh()
function for computing the hyperbolic arcsine values of different types of numbers, including positive, negative, and zero. Each example will provide insight into handling typical scenarios where hyperbolic arcsine calculations are necessary.
Understanding the asinh() Function
Basic Usage of asinh()
Include the
<math.h>
library in your program.Declare a variable to store the number whose hyperbolic arcsine you need to calculate.
Call the
asinh()
function and store the result.c#include <math.h> #include <stdio.h> int main() { double number = 1.0; double result = asinh(number); printf("The hyperbolic arcsine of %f is %f\n", number, result); return 0; }
In this code,
asinh()
calculates the hyperbolic arcsine of1.0
. The result is then printed to the console.
Calculating asinh() for Various Numbers
Implement
asinh()
for different values to see how the function behaves across a range of inputs, including negative numbers and zero.c#include <math.h> #include <stdio.h> int main() { double numbers[] = {-1.0, 0.0, 1.0, 2.0}; int i; for (i = 0; i < 4; i++) { double result = asinh(numbers[i]); printf("The hyperbolic arcsine of %f is %f\n", numbers[i], result); } return 0; }
This snippet calculates the hyperbolic arcsine for an array of numbers:
-1.0
,0.0
,1.0
, and2.0
. The results are printed sequentially, showcasing howasinh()
processes various types of input.
Special Cases Handling
Check the behavior of
asinh()
when providing extreme values and special floating-point numbers such asINFINITY
.c#include <math.h> #include <stdio.h> int main() { double vals[] = {1e-10, 1e10, INFINITY}; int i; for (i = 0; i < 3; i++) { double result = asinh(vals[i]); printf("The hyperbolic arcsine of %f is %f\n", vals[i], result); } return 0; }
Here, the code demonstrates how
asinh()
handles extremely small numbers (1e-10
), very large numbers (1e10
), andINFINITY
. This helps understand the range and limitations of the function.
Conclusion
The asinh()
function from the math.h
library in C is essential for computing the hyperbolic arcsine of a number. Understanding how to use this function efficiently with a variety of numeric inputs enables you to handle calculations pertinent to fields like physics and engineering where hyperbolic angles frequently arise. By following the examples provided, you can handle basic to advanced scenarios involving the asinh()
function effectively.
No comments yet.