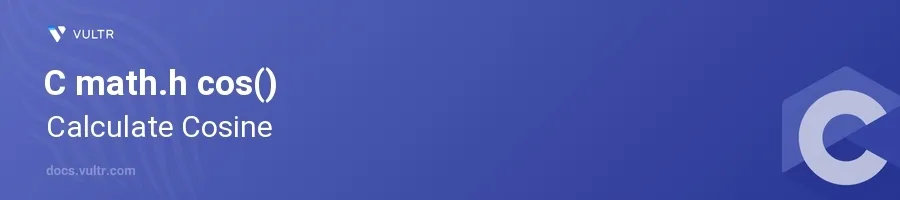
Introduction
The cos()
function from the C standard library's math.h
header file computes the cosine of a given angle, which must be specified in radians. This mathematical function is crucial in various applications such as engineering, physics, and computer graphics, where trigonometric calculations are necessary.
In this article, you will learn how to effectively utilize the cos()
function in practical programming scenarios. Understand how to convert degrees to radians and explore examples demonstrating the function's usage in different contexts.
Understanding cos()
Basics of cos()
Include the
math.h
header at the beginning of your program to usecos()
.Provide the angle in radians to
cos()
to calculate the cosine value.c#include <math.h> #include <stdio.h> int main() { double angle = M_PI / 4; // 45 degrees in radians double result = cos(angle); printf("Cosine of 45 degrees: %f\n", result); return 0; }
This snippet calculates the cosine of 45 degrees. Note that
M_PI
is a constant frommath.h
representing π, and π/4 radians equals 45 degrees.
Converting Degrees to Radians
Often, angles are given in degrees, but
cos()
requires radians.Convert degrees to radians using the formula: radians = degrees * (π / 180).
c#include <math.h> #include <stdio.h> double degrees_to_radians(double degrees) { return degrees * (M_PI / 180); } int main() { double degrees = 60; double radians = degrees_to_radians(degrees); double result = cos(radians); printf("Cosine of 60 degrees: %f\n", result); return 0; }
This code includes a function
degrees_to_radians()
to handle the conversion. It then calculates the cosine of 60 degrees.
Practical Example: Using cos() in a Project
Apply
cos()
to simulate real-world phenomena such as oscillations.Combine
cos()
with other mathematical functions for more complex calculations.c#include <math.h> #include <stdio.h> int main() { for (int t = 0; t <= 360; t += 10) { double radians = degrees_to_radians(t); double displacement = 10 * cos(radians); printf("Displacement at %d degrees: %f units\n", t, displacement); } return 0; }
This code models the harmonic motion of a point moving with a maximum amplitude of 10 units, showing the displacement for each 10-degree increment over one full cycle (360 degrees).
Conclusion
The cos()
function from the C math.h
library is indispensable for computing cosine values, critical in fields requiring trigonometric operations. Mastering the conversion between degrees and radians and integrating cos()
into applications can greatly enhance the mathematical capabilities of your programs. Implement the examples provided to ensure precision in your computational tasks and expand them to suit more complex scenarios in your projects.
No comments yet.